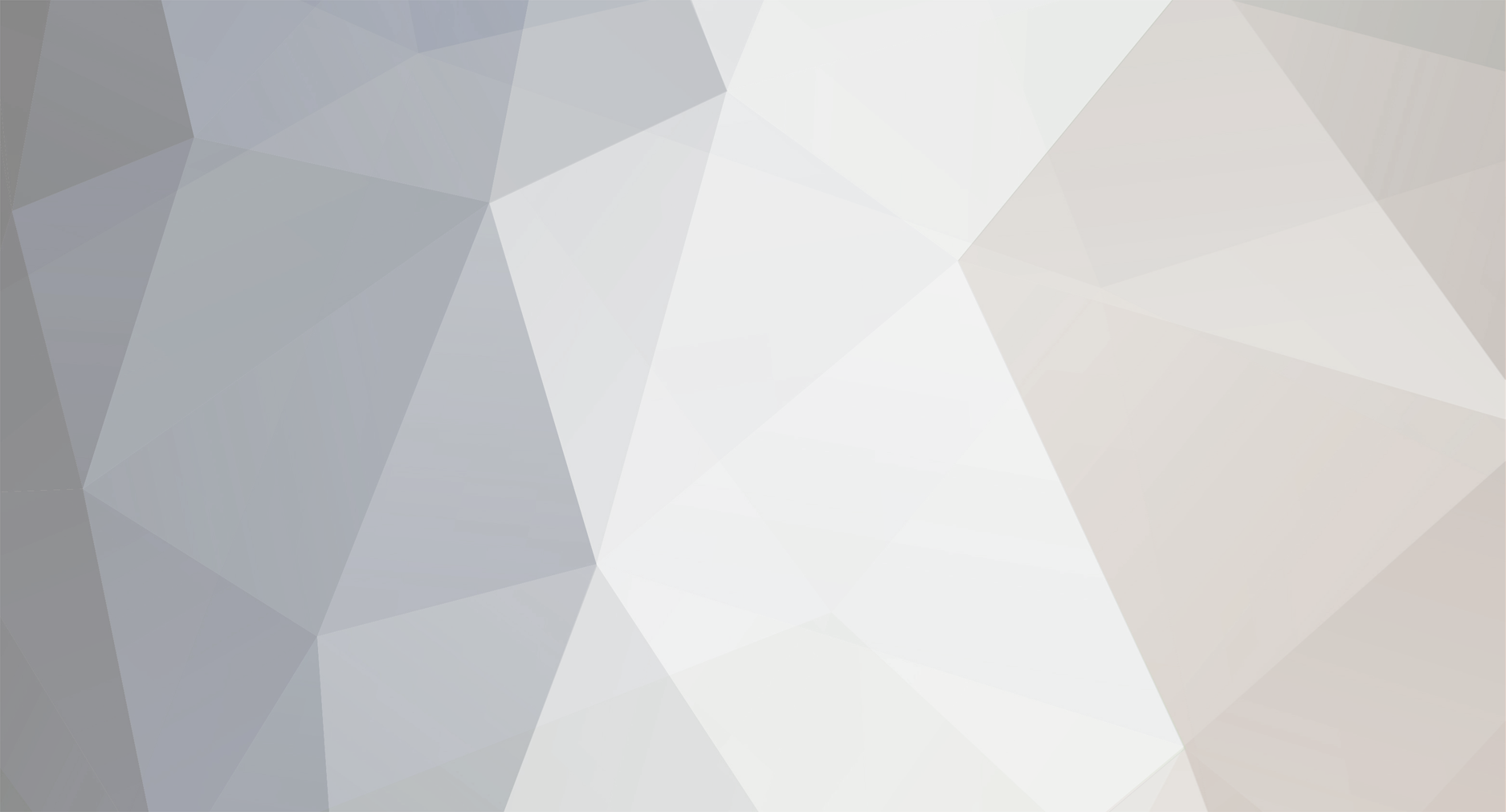
nethnet
Members-
Posts
284 -
Joined
-
Last visited
Everything posted by nethnet
-
It is saying you haven't selected a database. You need these two lines of code to initiate a database session: <?php $link = mysql_connect("server","username","password"); mysql_select_db("database",$link); ?> It's best to put that in a file called db.php and include it on every page. You'll have to change all the values in quotes to your actual values.
-
That warning is telling you that $berekenen is not of type RESOURCE like it should be in order to be used in the mysql_fetch_array() function. Most likely, it is of type BOOLEAN, more specifically FALSE because the query failed. Add this for debugging purposes and see if a mysql error is returned: <?php $berekenen = mysql_query($query) or die("Query error: " . mysql_error()); ?>
-
Sorry, I wasn't reading this very carefully.. You have everything set in your $_SESSION variables that you will need on your pages, right? So on a page like members.php where you are checking to see if they are logged in, have this: <?php include 'db.php'; $username = $_SESSION['username']; $user_pass = $_SESSION['user_pass']; if((!$username) || (!$user_pass)){ echo "<center />Please enter Username and Password! <br />"; include 'login.php'; exit(); } ?> A better way would be to have a PHP script with the above code named something like login_check.php, and for any page that you want to make sure the user is logged in, just put this at the top: <?php include "login_check.php"; ?> Theo
-
It should be level_usr = 'User'. Don't forget quotes.
-
Try this: <?php $sql = "SELECT ZWI_TSJ_uitslag FROM `poule a` WHERE user_id = \"{$row['id_usr']}\""; $query = mysql_query($sql) or die("Error in the query: " . mysql_error()); ?> If that does not work, try echoing $sql to ensure that the query is exactly what it should be.
-
You're not passing the data along to members.php at all... instead of using header("Location: ..."), why don't you just use include "members.php"; and remove the line include "db.php"; That way you have full access to all the variables you use in checkuser.php
-
The $_POST array is empty according to what you just said. Check your form to make sure the data is being passed along to this script.
-
Wow, nevermind... found my mistake almost immediately after posting, haha... I forgot braces around my foreach loop....
-
So it's getting late and I feel like I'm too tired to catch my simple mistake here, so I'm posting it for all to take a stab at. Basically what this script is supposed to do is select all of the tutorials from my database and organize them into categories. The final result would look something like this, with the number in each category in parentheses: AJAX (23) MySQL (40) PHP (65) RSS (7) XML (12) You get the idea... So the problem here is that my script is pulling the necessary information from the database, but for some reason it isn't populating my $two_dim array (which is supposed to keep track of the counts of different categories). <?php $sql = "SELECT * FROM tutorials"; $query = mysql_query($sql); $two_dim = array(); while($result = mysql_fetch_object($query)){ $category = explode(",",$result->keywords); foreach($category as $cat) // echo "$cat, "; $two_dim[$cat]++; } // echo "<p>"; // print_r($two_dim); // echo "</p>"; ?> The commented lines are lines I added in to debug... Currently there is just one record in the table, and it's value for "keywords" is "PHP,XML,RSS,MySQL", so the resulting array should look like: Array ( [code=php:0] => 1, [xml] => 1, [RSS] => 1, [MySQL] => 1 ) However, when I uncomment the last three commented lines to see what my array contains I only see: Array ( [MySQL] => 1 ) For some reason only the last value from the exploded array is being put into the new array to track counts... So then I added the first commented line to see if $cat was working properly, and when it runs it prints out "PHP, XML, RSS, MySQL, " like expected... I don't understand what is going on here, and it has to be something simple as this is a very simple script... Thanks in advance, Theo
-
If you aren't outputting anything with the script, then you will get a blank page... Is the mail being sent/the information being put into the database??
-
You could make that into a JS function and put it into a .js file that you include in every page in the HTML head, or you could just leave it like that in a file and use include() to show it on every page.
-
Show us the rest of the code.
-
You could do a lot of things. They say it's best to start small-scale and work your way up, but I disagree. When I started using PHP I started with a full-scale MMORPG. It took forever to do, but I learned so much from that one project. You could do something like a personal blog, where you can experiment with pretty much anything new and interesting that comes your way. Forums like this are always a good learning experience. Or you could venture to the PHP Freelancing forum and see if there is anyone who is looking for a small non-paid team to work on a large project. Working with other people will be immensely beneficial, as well. It's really up to you and whatever you find interesting. Theo
-
Where is $id being set?
-
Try adding this just for debugging purposes: if(mail($email1, $subject, $message, "From: Draconic Quest<webmaster@draconicquest.com>,\nX-Mailer: PHP/" . phpversion())) echo "Success! Email was sent!"; else echo "There was an error and the mail was not sent!"; If you see the success message when you run your script, then just give it some time, perhaps the email isn't being delivered immediately, or check your spam box. Theo
-
What you're trying to do (and I may be wrong, but I'm pretty sure I'm not) is unfeasible, as PHP is a server-side language and JS is client-side. Therefore, all of your PHP is parsed before there is any output to the browser, while JS is actually part of the output. If all you're doing is getting the time, do what schilly recommended, as use the PHP function time(). Theo
-
Show us what is being output as well as the contents of your array.
-
Appending .txt worked to see the source... and I don't exactly understand why it's not working for you when it works for me in both Opera and Firefox.
-
$specialties=explode(",",$row['specialties']); $max = count($specialties); $count = 0; foreach($specialties as $fs){ $count++; if($count == $max) echo "$fs"; else echo "$fs, "; }
-
The beauty of foreach loops... <?php $headers = get_headers($url); foreach($headers AS $key => $value) echo "[$key]: $value<br />"; ?> Theo
-
That's what happens when two people reply at the same time time time...
-
They function essentially the same way, the difference betwwen them being how they return errors. If the included file isn't found or is not able to be included, PHP will throw a warning and continue running the rest of the script. If the required file isn't found or is not able to be included, PHP will throw a fatal error and stop executing the script. Information in the manual: require() include() Also look at the following: require_once() include_once() Theo
-
[SOLVED] php/javascript time?? or system/server time??
nethnet replied to DonPatricio's topic in PHP Coding Help
Maybe if you're developing locally... but if you use the time() function online it will give you the server time, and it will have absolutely nothing to do with the time on your computer. BTW, you don't have to worry about different users timezones affecting what is being put in the database. When you do the INSERT query, just use the MySQL now() function, which is also server-based. <?php $sql = "INSERT INTO table (all, your, basic, fields, here, insert_time) VALUES ($all, $your, $basic, $values, $here, now())"; $query = mysql_query($sql) or die("Error inserting record."); ?> Theo -
<p class="comments align-right clear"> This is just a <p> tag with 3 classes (I don't think that part of the CSS was included in this thread.. sorry about that.. I assure you it's in my stylesheet though). I realized I was using <span> incorrectly, but it yielded the results I wanted in Opera, and I was hoping there was some way to get it to work the same way in IE and other browsers (i.e. where the black border runs through the middle of the images). Anyway, I'm going to switch it to a list like you suggested, and see if I can tweak that to get what I want. Thanks for the help, I'll post again if I get more frustrated Theo
-
[SOLVED] Using a server side include and php together
nethnet replied to candleman98's topic in PHP Coding Help
I'd suggest looking into virtual(). This is a function only supported when PHP is running as an Apache v2.0 or later module. Theo