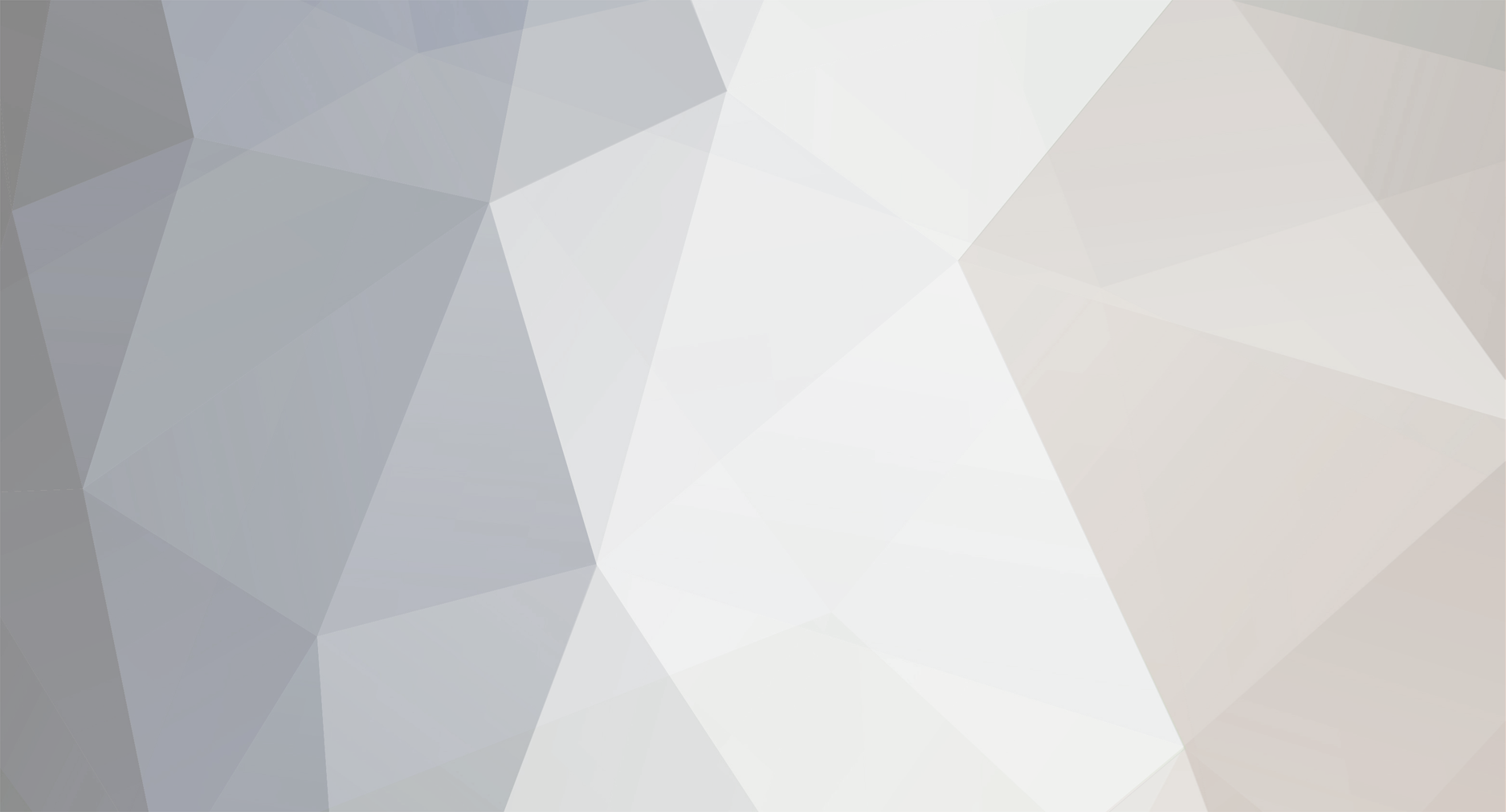
nethnet
Members-
Posts
284 -
Joined
-
Last visited
Everything posted by nethnet
-
[SOLVED] Problems With Larger Number Calulations
nethnet replied to dlebowski's topic in PHP Coding Help
Hrmm, actually, I just ran your original code and it worked fine for me.. no problems. I don't know why it's adding up different for you. <?php ini_set(display_errors, TRUE); $ItemTotalPrice=90000; $TaxTotal=900; $CashPremiumDue=4545.00; $CashTotalPaid=0; $GrandTotalNoPremium = ($ItemTotalPrice + $TaxTotal) + $CashPremiumDue; $AllPaymentTotal = $CashTotalPaid; $amountdue = number_format(($GrandTotalNoPremium - $AllPaymentTotal), 2, '.', ','); echo $amountdue; ?> And this is what I see on the page: 95,445.00 Apparently the float vs. integer issue isn't even a problem here... I guess that just applies to strings. Try copying what is written above exactly and see if you still get 90,904.00 in your variable. Theo -
Just realized that in my first post I put ORDER BY topic_id ASC. The ASC should be DESC if you want the highest number. The other way will give you the lowest number.
-
"LIMIT 1" will return only one row from the database, and "ORDER BY topic_id DESC" will put all of the rows in reverse numerical order based on topic_id, so using them together will give you just one row, that being the highest topic_id...
-
<? $sql = "SELECT topic_title, topic_first_poster_name, topic_id, forum_id, topic_poster FROM phpbb_topics ORDER BY topic_id ASC LIMIT 1"; $info = mysql_query($sql) or die("Couldn't run the query."); ?>
-
<? $level = @$_GET['level']; ?>
-
The function nl2br() will replace all new lines with <br /> tags, but if you need to put the content inside <p> tags, then you could try the following: <?php $raw_content = $_GET['textbox']; $lines = explode("\r\n",$raw_content); $formatted = array(); foreach ($lines AS $line){ if (!empty($line)) $formatted[] = "<p>" . $line . "</p>"; } ?> The $formatted array will then contain each line from the form inside <p> tags. Also, this will work if the content is coming from a <textarea> because the form uses both \r and \n for new lines. If this doesn't work, play around with "\r", "\n", and "\r\n" to see which works if you aren't working with a <textarea>. Theo
-
[SOLVED] Problems With Larger Number Calulations
nethnet replied to dlebowski's topic in PHP Coding Help
The problem is with the decimal in your variable $CashPremiumDue. When you perform mathematical operations in PHP with variables, by default, those variables are converted to integers if they are not already. Therefore, since $CashPremiumDue is type float instead of type integer, PHP is recognizing it and treating it the same way it would a string (because of the decimal). When you add or subtract strings in PHP, only the first character in the "string" is evaluated, so really PHP is recognizing this: <?php $GrandTotalNoPremium = (90000 + 900) + 4; ?> Obviously that's not what you want, so try something like this instead: <?php $GrandTotalNoPremium = ($ItemTotalPrice + $TaxTotal) + (int)$CashPremiumDue; ?> Or just drop the .00 if it's always zero. -
So I've been developing my website for a while now, and I use Opera web browser to test it, and I just recently realized that this was a bad idea (well, maybe not bad, but naive). Sure enough, as soon as I opened it in IE I noticed an error in how I want part of my layout to be displayed. It's correct when viewed in Opera, but in IE the heights of my SPAN tags are not how I want them to be. I realize that most of you don't have Opera and IE to view my page in and see for yourself, so I'm including screenshots of what's going on here. In Opera, the correct way: In IE, the INcorrect way: <div id="main"> <h1>nethnet.com desktop</h1> <div class="center"> <span class="desktop"><img src="images/repository.png" /> Repository</span> <span class="desktop"><img src="images/tutorials.png" /> Tutorials</span> <span class="desktop"><img src="images/news.png" /> News</span> <span class="desktop"><img src="images/documentation.png" /> Documentation</span> </div> <p><strong>Nethnet.com</strong> is a free online archive of lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat.</p> <p>Lorem ipsum dolor sit amet, consectetuer adipiscing elit, sed diam nonummy nibh euismod tincidunt ut laoreet dolore magna aliquam erat</p> <p>Consectetuer adipi elitagna veni!</p> <p class="comments align-right clear"> <a href="index.html">Read more</a> : <a href="index.html">comments(3)</a> : 1.25.08 </p> </div> The CSS that corresponds to what is going on here is: /* main column */ div.center { text-align: center; } #main { float: right; margin: 0; padding: 0; width: 545px; } #main h1 { margin: 10px 0; padding: 4px 0 4px 8px; font-size: 105%; color: #FFF; text-transform: uppercase; background-color: #CC0000; letter-spacing: .5px; } #main span.desktop { margin: 5px 5px 5px 5px; padding: 5px 5px 5px 5px; font-size: 105%; font-weight: bold; border: 1px solid #333; height: 20px; } This is probably something really simple, but how do I get the border of the SPAN style to run through the images like it does by default in Opera? Thanks in advance for any help. Regards, Theo
-
Are the variables used to define the fields inside $msg even set anywhere? Most likely they are all empty to begin with... Is there another script that gives them values?
-
echo php vars in javascript new window function
nethnet replied to xjasonx's topic in PHP Coding Help
You cannot interpolate variables inside single quotes. Try something like this: echo "window.open(\"nwindow.php?id=$id&title=$title\",\"newwindow\",config=\"height=500,width=400\")"; Use double quotes when you contain variables inside an echo statement, or concatenate, or as I did above, escape other double quotes. -
PHP MYSql help - Album was not added due to an error
nethnet replied to mortonfc's topic in PHP Coding Help
Change this: echo'Album was not added due to an error'; To this: echo "Album was not added due to an error. Query: " . $query; That way we can see what the exact query is. -
getting started with PHP/the easiest question in this forum (probably)
nethnet replied to MNSarahG's topic in PHP Coding Help
Do you have error reporting turned off? If you ran that script, you should have an output no matter what, since both the if and else statements output text. If you have error reporting turned off then perhaps your script is encountering a fatal error and not executing fully, and you aren't being told of it. Check your error logs or set error reporting to on. -
getting started with PHP/the easiest question in this forum (probably)
nethnet replied to MNSarahG's topic in PHP Coding Help
Try something like this: $bool = mysql_query($query); if ($bool == TRUE) echo "The query was executed successfully."; else echo "There was an error with the MySQL query."; That way we at least have some output to help us see if the query is being executed properly. -
It looks much better than it did last time I looked at it. I'm still not sure if the green bar at the top matches the scheme of the website, however. I think it would look better if you made it a gray.
-
You could keep track of how many times the logo was viewed in a flat text file if you don't want to use a database. Although, if this is sensitive material, you should be aware that the text file won't be secure.
-
Combine them into one query. "UPDATE users SET activated=1 AND accesslevel=1 WHERE uid='$uid'"
-
You'll need to do this in activate.php. When the activation script is run, have it update the database with this: $id = $_REQUEST['uid']; mysql_query("UPDATE users SET accesslevel = 1 WHERE id = $id"); You'll have to change "id" to whatever you call the user id in your database.
-
How to make my software Limited to one domain?
nethnet replied to ahzulfi's topic in PHP Coding Help
If you think it's hidden well enough, I suppose that is a possible way to do it.. although, if someone wants to use the script bad enough without getting a license, that's not going to stop them from finding it, trust me. I haven't really dealt with this sort of thing before, but I imagine you could omit a few lines of code that are necessary for the script to operate properly (lines that can't be replicated by anyone who just views the source) and put them in a script that is executed off of your own server. That script could then run any form of validation you desired, since you would have complete control over it. I'm not sure if that would even work or not, or even if it's what your looking for in a solution, but it's worth a shot. Theo -
First of all, center it. If you are going to center the footer, center the content. Also, there are some issues with the scrollbars implemented for the content section. I'm viewing the site in Opera 9.21 and they are just kind of floating around the page. One of them is lost behind the google ads. The logo hurts my eyes because of the aliasing regarding the text. Try softening the edges of the letters out, or blending them into the background a bit. Also, I was unable to do anything after logging in. I'm not sure if there is anything interactive yet, but it just greeted me and popped up the same welcome message from before. It also doesn't validate. It's a good start, but it needs some work.
-
Try starting off with the PHP Freaks Tutorial archive. There's a wealth of information there for a new PHP programmer, so I'm sure you will find it very helpful. It's accessible from the main page, or just click on the link: http://www.phpfreaks.com/tutorials.php If you are serious about PHP, I'd recommend buying a good book or two. Amazon.com offers a wide selection of good books. It's good to have high ambitions with PHP, but you should be aware that if you are completely new to the language (or programming in general), then it will probably take a decent amount of time, effort, and frustration before you'll be ready to tackle a full-scale project like a forum system. Best of luck, Theo
-
You should post in the freelancing forum if you want someone to customize a code for you.
-
Noobie Stuff: Very Basics Explained Easily
nethnet replied to Pudgemeister's topic in PHP Coding Help
It can be a string like he had. The problem is that he has "==" instead of "=" like it should be. Change those double equals to single equals. It's returning an error because of the SQL syntax. -
A submit button only sends data within that form. There is no data in your form and therefore nothing is being sent.
-
Noobie Stuff: Very Basics Explained Easily
nethnet replied to Pudgemeister's topic in PHP Coding Help
Like GingerRobot said, your functions should not be in quotes. What you are doing, essentially, is setting $num_1 and $row_1 equal to strings, not the functions you wish to perform. So if you were to echo $row_1, the output would be "mysql_fetch_assoc($result_1)". Then, you attempt to echo $row_1 as if it were an array (by using the key 'mining_depot'). This would work if $row_1 were an array, but it's a string as you have it, and when you try to echo a string using a key you get different results. For example: $string = "Random string"; echo $string[0]; echo $string[1]; echo $string[2]; echo $string[7]; You would get: "ran " (because you are echoing the letter in slot 0, 1, 2, and then 7 [the space]). You are using a word as your key, "mining_depot", and therefore you are telling PHP to "echo the letter amonst string $row_1 in slot number 'mining_depot'". As you can tell, this doesn't make sense, and PHP is outputting an 'm' instead. The reason why the m is showing up is because PHP is automatically converting the string 'mining_depot' to an integer (PHP does this when you provide a string when only an integer may be used). When telling PHP to echo a letter in a string like you are doing, only an integer may be used, and 'mining_depot' is being converted to 0. PHP.net will give you good information on type changes like this (string to integer in our case). The 0 is then being used as the slot number, and that letter happens to be 'm' (because that's the first letter in "mysql_fetch_assoc($result_1)"). So, yeah, take those quotes off and it should work. I just wanted you to get a better understanding of what was happening. Good luck! nethnet -
I guess it would depend on how much you feel you would benefit from formal schooling in web design. Do you already feel like you're on the same level or superior to graduates of that program? If so, I would say it wouldn't be worth it. Web design "certification" isn't generally used as a criteria that clients would look at anyway. If you have a nice portfolio, enough to convince them that is, then you don't have to worry about pieces of paper hanging in your office. It all depends on your personal comfort level with professional web design.