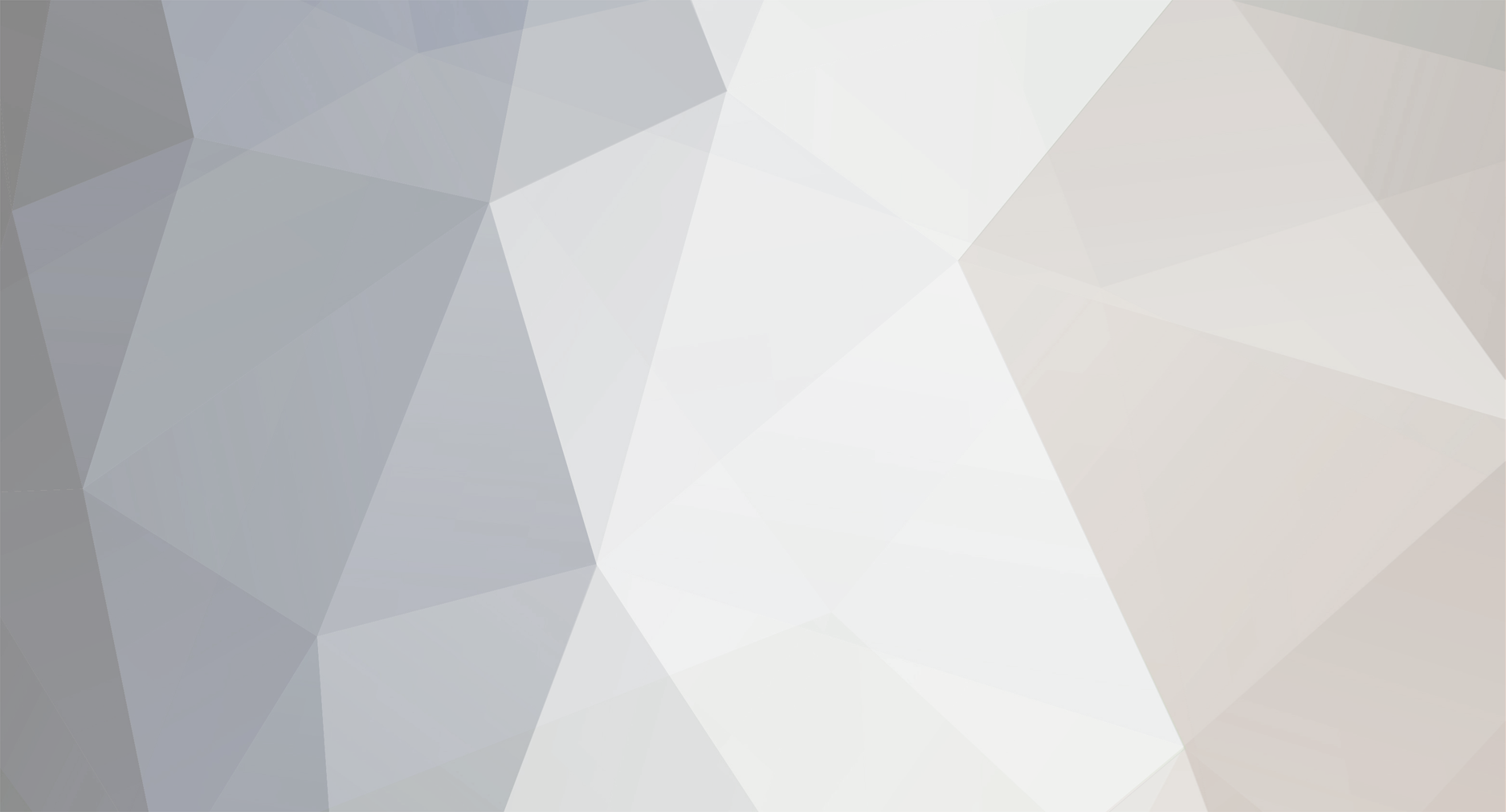
White_Lily
-
Posts
531 -
Joined
-
Last visited
-
Days Won
1
Posts posted by White_Lily
-
-
it is. and the "Go To Login" button is an image rather than text, so all you have to do is a little extra styling.
-
how are you storing the downloads? (file names specifically)?
-
is the one on my site what you were looking for or not?
-
Can I just ask, why does it HAVE TO use associative arrays and foreach loops?
-
It's not really about what we think, it's about what you or the client wants, if you want a pop-up - have a pop-up, if you dont want a pop-up - then dont have one.
-
Actually, it does help, because if its what he wants, then I can give him code for it.
Whereas if its not exactly what he wants then posting the code would be a waste of time.
-
An example of what the OP wants is on the Social Network link in my signature, fill out the form and a green pop-up box will appear saying "Go To Login" meaning that you have successfully registered to the site.
-
If you want multiple errors to appear should there be more than one fault in the form submitted, try using something that displays the errors in a list, like this:
<?php $connect = mysqli_connect("localhost", "web113-social-1", "innov8er", "web113-social-1"); if(!$connect){ die('Connect Error (' . mysqli_connect_errno() . ') '. mysqli_connect_error()); } include "conf.php"; include "functions.php"; $error = array(); foreach($_POST as $key => $value){ if(empty($value) && $value == "undefined") $error[] = "Please fill in any blank fields. ".$key.":".$value; } $name = mysqli_real_escape_string($connect, $_POST["name"]); $email = mysqli_real_escape_string($connect, $_POST["email"]); $username = mysqli_real_escape_string($connect, $_POST["username"]); $password = mysqli_real_escape_string($connect, $_POST["password"]); $confirmPassword = mysqli_real_escape_string($connect, $_POST["confirmPassword"]); $getData = select("*", "users", "username = '$username'", NULL, 1); $data = mysqli_fetch_assoc($getData); if($username == $data["username"]) $error[] = "That username is already taken, please choose another."; if($email == $data["email"]) $error[] = "That email address is already in use."; if($password != $confirmPassword) $error[] = "The passwords you entered do not match."; if(strlen($username) < 6) $error[] = "That username is too short, it should be at least 6 characters long."; if(strlen($username) > 30) $error[] = "That username is too long, it should be no longer than 30 characters."; if(!preg_match('/^[a-zA-Z0-9]+$/', $username)) $error[] = "Invalid username, there should be no spaces or special charaters, please choose another."; if(!preg_match('/^(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{6,}$/', $password)) $error[] = "Invalid password, there should be 1 uppercase and lowercase letter, 1 digit, it must also be a minimum of 6 characters, and a maximum of 30 charaters."; if(count($error) == 0){ if(!mkdir($GLOBALS["siteRoot"]."social/users/".$username)) $error[] = "Could not create your account, either try again later, or contact the webmaster."; if(!mkdir($GLOBALS["siteRoot"]."social/users/".$username."/avatars")) $error[] = "Could not create your account, either try again later, or contact the webmaster."; if(!mkdir($GLOBALS["siteRoot"]."social/users/".$username."/images")) $error[] = "Could not create your account, either try again later, or contact the webmaster."; if(!mkdir($GLOBALS["siteRoot"]."social/users/".$username."/images/albums")) $error[] = "Could not create your account, either try again later, or contact the webmaster."; } if(count($error) > 0){ for($i = 0; $i < count($error); $i++) echo "<li class=\"error\">".$error[$i]."</li>"; } $password = hash('sha256', $password); if(count($error) == 0){ $regUser = insert("users", "first_name, username, password, directory, email", "'$name', '$username', '$password', '".$GLOBALS["siteRoot"]."social/users/".$username."', '$email'"); if($regUser) echo 'correct'; } ?>
and before anyone starts to disagree: yes the foreach($_POST as $key => $value) DOES check to see if the form is empty, should you not believe me click the social network development link in my signature and click "Sign Up" and you will see that it works.
-
I will work on one and get back to you then.
-
Thats because its an example, profile.php isnt created yet, but what im asking is, is that process what you were looking for?
-
I figured it out, it was the $connect variable being undefined, how I don't know, but all I did was re-type the connection details re-uploaded the file, and the error disappeared and data from the database showed.
-
Go to the forum development link in my signature, and click "Sign In".
The username and pass is:
user: Innov8er
pass: Dr4g0nhe4rt
-
The part where the pop-up box lets the user know something is wrong, or their login was correct is done by using php & aJax together.
I can give you an example if it will be useful to you?
-
Which would most likely suit your site? I find plain text to be boring and not very eye-catching - so a jQuery pop-up box would be better, you could also go the extra mile and make the registration and login forms pop-up boxes aswell, and use jQuery / aJax to validate the forms.
-
have you also considered letting people choose their own background? (either have a range of "default" backgrounds, or allow users to upload there own?
-
Just a quick question, you have a "Find Friends" tab, which at the moment seems to list all members, but where is the search bar?
-
Okay, so I looked back at php.net to see what error checking they use...
<?php $connect = mysqli_connect("localhost", "username", "password", "database"); if(!$connect){ die('Connect Error (' . mysqli_connect_errno() . ') '. mysqli_connect_error()); } echo 'Success... ' . mysqli_get_host_info($connect) . "\n"; ?>
Guess what... Still getting an Undefined $connect error... dispite manually typing in the connection settings.
-
To check if a a post variable is empty you could just use:
<?php foreach($_POST as $key => $value){ if(empty($value)) $error = "Please fill out all fields of the form"; } ?>
-
-
Notice: Undefined variable: connect in/home/sites/janedealsart.co.uk/public_html/social/inc/functions.php on line 17
Actually Jessica - yes it is producing an undefined variable error.
-
-
Hi, I have ahd a slight problem with the deprecation of mysql_*() functions, and so are swapping them for mysqli_*() functions, how I have come across an error which states that $connect is undefined.
<?php $GLOBALS["host"] = "localhost"; $GLOBALS["user"] = "web113-social-1"; $GLOBALS["pass"] = "********"; $GLOBALS["database"] = "***************"; $connect = mysqli_connect($GLOBALS["host"], $GLOBALS["user"], $GLOBALS["pass"], $GLOBALS["database"]); ?>
I have compared this against php.net's examples, and this code looks almost exact. I dont know what the problem is. Any help would be appreciated.
- Lily
-
Oh okay sorry, misread that bit >_<
-
you could make the password itself more secure and just not do it in plain text, but thats all you can do really.
-
Although this makes things more difficult and a lot more learning for you, I think most forms should have client AND server side validation. (client side being jQuery & aJax, server-side being PHP), client side is useful so that your users dont have to waste time refilling the form out because theyve got a wrong input, jQuery and aJax validate their inputs as they are typing/clicking.
-
Regardless, the database still won't like some characters.
Login form text help
in PHP Coding Help
Posted
Okay, I put the files you need into a .zip folder for you:
http://janedealsart.co.uk/template/uploads/51015b6445b3c.zip