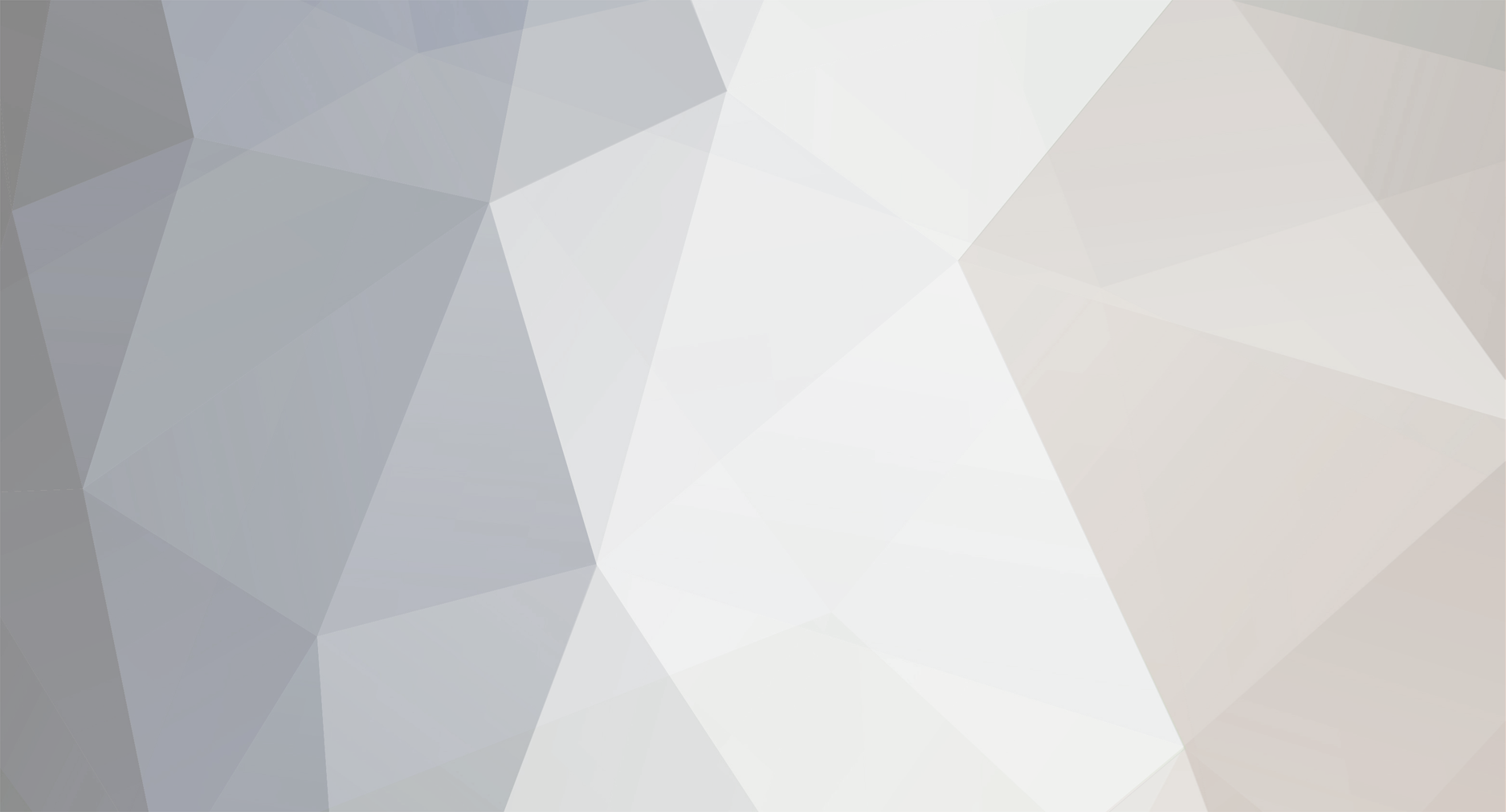
White_Lily
-
Posts
531 -
Joined
-
Last visited
-
Days Won
1
Posts posted by White_Lily
-
-
you could type out the value of $_SERVER["DOCUMENT_ROOT"] into a $GLOBALS[""] variable? eg:
<?php $GLOBALS["file_root"] = "/Projects/cms/5113/htdocs2"; ?>
this way only you can change and nothing else
-
okay, its working now not entirely sure what went wrong, i implemented the changes you suggested, refreshed the page - nothing happened, so i re-uploaded and now it is working... anyway, thanks for your help :-)
-
d'yah what all I want to do is get the jQuery to calculate the minimum and maximum length of the users entered string, if its less than 6 then show an error, if its more than 30 show an error, anything inbetween is acceptable... thats all i wanna do.
-
so the input field needs the id of usernameLength, not the div?
-
by implenting the changes and uploading the document then running it on the live site.
the site im working on is the Forum Development in my signature, if you go there and "Sign In" at the top of the page, youll notice nothing happens, and both chrome and firebug are complaining about .length being "Undefined"
-
that doesnt seem to work either
-
So it calculates the number of elements, not the number of characters in a user entered string?
-
Yeah I have the latest jQuery from jquery.com's website and it is included, but like I said the code above worked one moment then a few hours later I go to try and work on it and it doesnt work at all.
-
Hi, I have a new problem in that I managed to get my validation working last night, but because it was getting close to 4am, I decided it was time for bed, I woke up a few hours later to work on the script some more, and when I go to my site to see where I left off, I find that the script no longer works, but it did just before I went to bed?
Here is the code I typed out:
<script type="text/javascript"> $(function(){ var $submit = $("div.submitBtn input"); var $required = $(".required"); function containsBlanks(){ var blanks = $required.map(function(){ return $(this).val() == ""; }); return $.inArray(true,blanks) != -1; } function isValidEmail(email){ return email.indexOf("@") != -1; } function requiredFields(){ if(containsBlanks() || $("#usernameLength input").val().length < 6 || $("#usernameLength input").val().length > 30/* || !isValidEmail($("#email").val())*/) $submit.attr("disabled","disabled"); else $submit.removeAttr("disabled"); } $("#registerOverlay span").hide(); $("#signIn").click(function(){ $("div.popOverlay").fadeIn("slow"); $("div#registerOverlay").fadeIn("slow"); }); $("div.popOverlay").click(function(){ $(this).fadeOut("slow"); $("div#registerOverlay").fadeOut("slow"); }); $("#registerOverlay input").focus(function(){ $(this).next().fadeIn("slow").css({ background: "pink", border: "1px solid red" }); }).blur(function(){ $(this).next().fadeOut("slow"); }).keyup(function(){ // check all required fields requiredFields(); }); $("#usernameLength input").keyup(function(){ //check string length of username if($("#usernameLength input").val().length < 6) $(this).next().removeClass("pass").css({ background: "pink", border: "1px solid red" }); else if($("#usernameLength input").val().length > 30) $(this).next().removeClass("pass").css({ background: "pink", border: "1px solid red" }); else $(this).next().removeClass("error").css({ background: "lightgreen", border: "1px solid green" }); }); /*$("#email").keyup(function(){ // check for valid email if(isValidEmail($(this).val())) $(this).next().removeClass("error").addClass("pass"); else $(this).next().removeClass("pass").addClass("error"); });*/ requiredFields(); }); </script>
The error that Google Chromes "Inspect Element" is giving me is:
"Uncaught TypeError: Cannot read property 'length' of undefined"
I've searched google but have not found a solution relevant to my problem.
Any help is appreciated
-
I have solved this, the classes were conflicting with eachother, so i had to set validation styles as element styles rather than class styles.
-
You could also just learn jQuery and write one yourself. That way you will understand 100% of it, be able to edit and debug it, and you will know what to edit and what not to edit when it comes to making particular changes.
What I can do is have a go at making a small menu (like yours) myself with code that I will write, and post it here if I succeed and find its cross-browser compatible. Okay?
-
Hi I have written a custom script to validate a form that I am using, the problem I am having is that i have written the code so that classes are applied at particular stages of the validation depending on what the user is editing.
The code:
<script type="text/javascript"> $(function(){ var $submit = $("div.submitBtn input"); var $required = $(".required"); var $userLength = $("#usernameLength input").val().length; function containsBlanks(){ var blanks = $required.map(function(){ return $(this).val() == ""; }); return $.inArray(true,blanks) != -1; } function isValidEmail(email){ return email.indexOf("@") != -1; } function requiredFields(){ if(containsBlanks() || $("#usernameLength input").val().length < 6 || $("#usernameLength input").val().length > 30/* || !isValidEmail($("#email").val())*/) $submit.attr("disabled","disabled"); else $submit.removeAttr("disabled"); } $("#registerOverlay span").hide(); $("#signIn").click(function(){ $("div.popOverlay").fadeIn("slow"); $("div#registerOverlay").fadeIn("slow"); }); $("div.popOverlay").click(function(){ $(this).fadeOut("slow"); $("div#registerOverlay").fadeOut("slow"); }); $("#registerOverlay input").focus(function(){ $(this).next().fadeIn("slow"); }).blur(function(){ $(this).next().fadeOut("slow"); }).keyup(function(){ // check all required fields requiredFields(); }); $("#usernameLength input").keyup(function(){ //check string length of username if($("#usernameLength input").val().length < 6) $("#usernameLength span").removeClass("pass").addClass("error"); else if($("#usernameLength input").val().length > 30) $("#usernameLength span").removeClass("pass").addClass("error"); else $("#usernameLength span").removeClass("error").addClass("pass"); }); /*$("#email").keyup(function(){ // check for valid email if(isValidEmail($(this).val())) $(this).next().removeClass("error").addClass("pass"); else $(this).next().removeClass("pass").addClass("error"); });*/ requiredFields(); }); </script>
The actual problem that I am having is that the addClass for the usernameLength span isnt working, well its applying the class, just not the styles, im not entirely sure why so I guessed it had something to do with this jQuery script. If you have any suggestions its much appreciated :-)
-
Do what beney said up until the last point (which is wrong).
Use:
<?php $sql = "query"; $result = mysql_query($sql); // This is where beney is wrong //Use: $data = mysql_fetch_array($sql); foreach($data as $row){ //continue with your code } ?>
-
The only reason I can think of as to why its doing that is because your echoing the $_GET[""] function, which pulls specified data from the url so that you can use it inside your script. The only solution here is to find that $_GET function and delete it, tbh, i think thats all you can do lol.
-
When i write forms, and need to check for empty fields, I use this code for it:
$validateArray = array("name" => "text", "email" => "email"); foreach($validateArray as $key => $value) { if($value == "text") $($key) = htmlspecialchars(mysql_real_escape_string($_POST[$key])); else $$key = mysql_real_escape_string($_POST[$key]); if(empty($$key)) { } }
-
I am constantly having to write registration and login scripts for clients, and therefore I prefer simple ways of comparing usernames.
This is the code i use to get a taken name, and display an error if the user tries to use a taken name:
$compare = select("users", "user_username", NULL, NULL, 1) or die(mysql_error()); // my select function is a custom and so you should swap this for a query or your personal select function. $use = mysql_fetch_assoc($compare); if($username == $use["user_username"]){ $error .= "<li class='error'>That username is already in use. Choose another.</li>"; }
-
Barand, by drop down menu he means when you hover ( or click on some sites o.O ) then a sub menu appears. Like on Facebook when you click the settings button at the top of your profile, it then displays a drop down menu with more settings to view, personally however I have always found drop down menus a problem.
Try this google search and you might find what you are looking for:
http://www.google.co...iw=1280&bih=933
Note: Most drop down menus are not supported by some browsers (namely: IE) so it might be worth looking into something that is supported by all browsers. The google link is for pure css menus, if you something with a bit os style to it, you might find jQuery menus better, again though, these are not supported in all browsers.
-
<?php $echo = $getFig["news_content"]; //change this to the content variable you are using if(strlen($echo) <= 30){ $bar = $echo; }if(strlen($echo) > 30){ $bar = substr($echo, 0, 31 /* if you change this, you must change the 30s in the above to be one less then this number as shown */)."<ahref=\"--fileLink--\">Read More...</a>"; } echo htmlspecialchars($bar); ?>
I used this to shorten my news feeds for one of my sites, works perfectly!
-
Your posts on your provided thread also state to adjust the time it takes to fill the form out, problem with this though is that people will have to take some time to think of a username... so im not sure if the time method would work...
-
yeah lol sorry. Marcus, a question about your alternative (lol) to captchas, shouldnt $_POST["start_time"]; be $_SESSION["start_time"];?? or am i to actually have another field in my form a with the time in it?
-
@cyberRobot; I have added an expression which i commonly use for passwords which basically says that you have to have 1 uppercase letter, 1 digit, and 1 lowercase letter and a minimum of 6 characters. and i have added the stuff about the labels and inputs
@cyber & marcus; I will have a look at thos captcha examples and will post later what I have done.
-
You keep saying the mysql_real_escape_string() is done too early, but it still checks them if they are empty and displays an error if they are empty... so im not extirely sure what your saying. However, feel free to see for yourself with the forum developnent link in my signature thing.
-
The part where i check if all fields have data is that the script does not come up with a whole bunch of errors (hopefully making it easier on the user :/ )
Use of data is whats planned based on what the client wants and how (s)he wants to use it, it is also based on how much they have in their budget which allows me to plan how much work i can do with what they give me.
-
1) What other validation should be done? just saying that there isn't any doesn't really help me much... i.e: should i be using expressions on certain inputs?
2) Not entirely sure if you noticed, but every variable needs a value for it to continue with the checks and user creation, hence the "&&" after every !empty().
3) What do you suggest I do about the insert query then? (I'm not a mind-reader unfortunately)
4) No data is redundant in my table. It will all get used. (Believe it or not but before I build my sites i plan what the site will use and how any data will be used)
I have changed the select query to only select the username field.
CSV Import Page
in Application Design
Posted · Edited by White_Lily
Try this:
http://www.google.co...iw=1280&bih=933
Also to add to Christian's comment, data within a CSV could also be "unclean" such as characters that PHPMyAdmin (or whatever database your using) won't like.