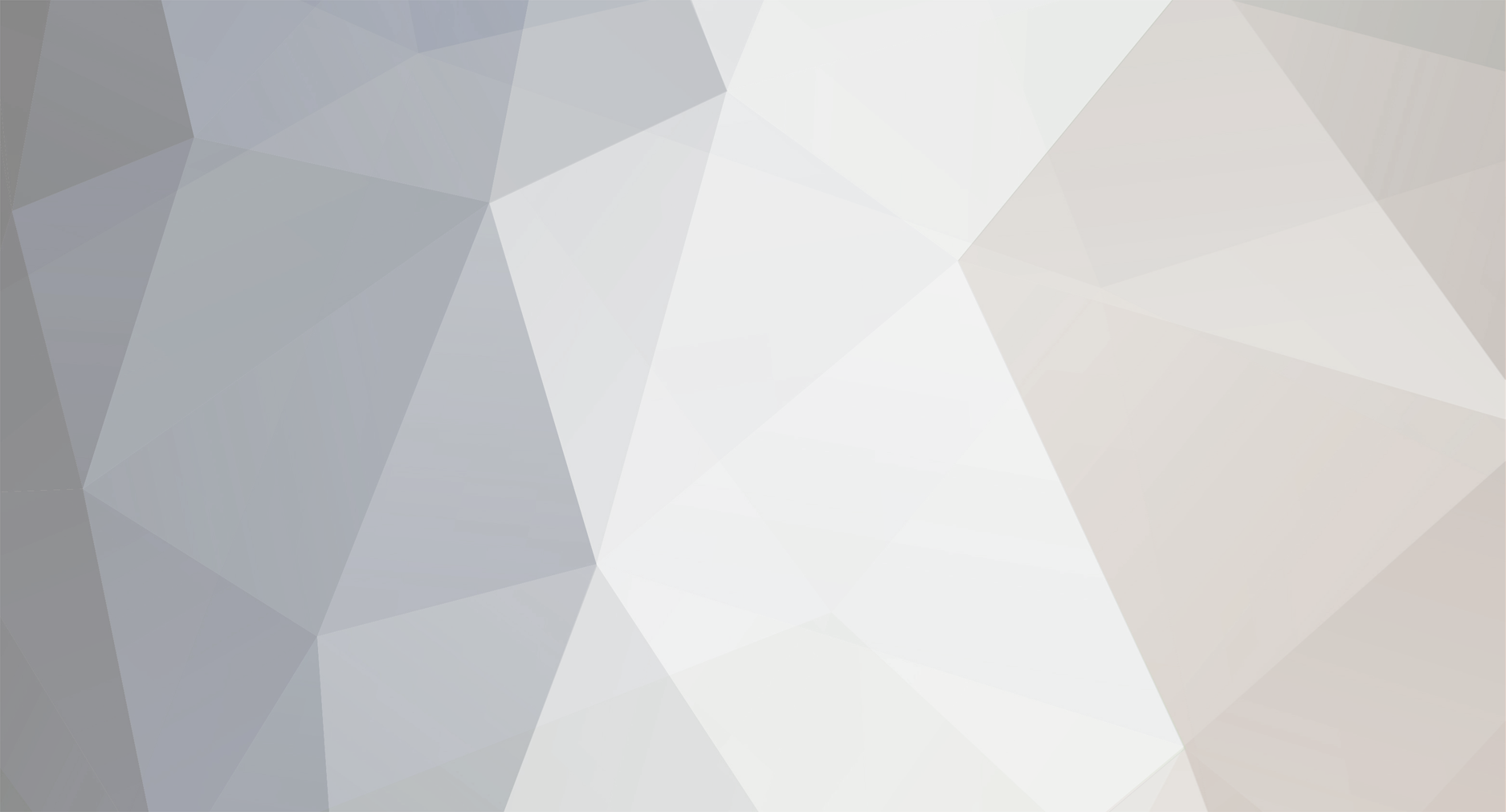
White_Lily
-
Posts
531 -
Joined
-
Last visited
-
Days Won
1
Posts posted by White_Lily
-
-
Also, you will want to make sure that a user is logged in on the pages you only want members to view. i.e:
<?php if(empty($_SESSION["user"])){ // If the is no username session set, redirect the viewer to the login page or whatever page you want. header("Location: login.php"); } ?>
-
you could also use php to see if banner.jpg exists in the given directory, if so, show the banner, if not show some text instead.
-
The script below is my upload script that renames files upon upload, however im sure it can be manipulated to rename files in a directory!
<?php if(!empty($_POST["upload_file"])){ $file = explode(".", $_FILES["file_upload"]["name"]); $extension = strtolower($file[1]); if(!empty($file[1])){ if(in_array($extension, array('jpg','jpeg', 'gif', 'png', 'bmp', 'doc', 'pdf', 'docx', 'mp4', 'zip', 'mov', 'mpg', 'wmv', '3gp'))){ $filepath = $_FILES["file_upload"]["tmp_name"]; $filename = uniqid().".".$file[1]; $target = $_SERVER["DOCUMENT_ROOT"]."template/uploads/".$filename; if(move_uploaded_file($filepath, $target)){ $document = str_replace("_", " ", $file[0]); $document = str_replace("-", " ", $document); $url = $GLOBALS["siteUrl"]."uploads/".$filename; $putFileInfo = insert("files", "file_name, file_url, file_original, new_file", "'$document', '$url', '".$_FILES["file_upload"]["name"]."', '$filename'"); if($putFileInfo){ $filePass = "File was successfully uploaded."; }else{ $fileErr = "File could not be uploaded: ".mysql_error(); } }else{ $fileErr .= "Could not move file."; } }else{ $fileErr .= "That is not an accepted file extension for the $page."; } }elseif(empty($file[1])){ $fileErr = "No file selected."; } } ?>
-
or a better question, what is your code? do you have an error reporting file such as php.ini? try dying any queries with mysql_error() and if they are wrong, you will receive an error?
-
SocialCloud, I have validated my submit button the way mdahlke suggested, and it works in every browser I have tested. (IE 6+, Mozilla, Opera, Safari and Chrome)
-
that just makes it more difficult lol. after i have the design done im putting the category html inside a while loop, making the .group .last element useless unless i use JS (which im trying to avoid so far so good).
-
Okay... :last-of-type doesn't even exist...
-
is :last-of-type compatible accross all browsers (even IE 7+)?
-
i changed the CSS to "groups" and it still does not do anything.
-
The CSS is not working in all browsers.
HTML:
<div class="groupBox"> <div class="groupHeading"> Heading 1 </div> <div class="group"> <div class="title-2"> <div class="catHeading"> Category 1 </div> <div class="cateDescription"> This is category description 1 </div> </div> <div class="countTopics"> <b>Topic Count:</b><br> <font>( 345 )</font> </div> <div class="clear"></div> </div> <div class="group"> <div class="title-2"> <div class="catHeading"> Category 2 </div> <div class="cateDescription"> This is category description 2 </div> </div> <div class="countTopics"> <b>Topic Count:</b><br> <font>( 545 )</font> </div> <div class="clear"></div> </div> <div class="clear"></div> </div>
-
Hi, I have a problem with my CSS as it is displaying the border-bottom property regardless of the :last-child element on the next style block.
.groupCategories { display: block; border-bottom: 1px solid #666666; } .groupCategories:last-child { border: 0px; }
Any ideas?
-
Try:
$sql = 'SELECT `BikeCode`, `Model`, `Price` FROM `Bike` WHERE `BikeCode` = '.$id; $result = $db->query($sql) or die("Select query does not work!<br><br><br>".mysql_error());
-
Well then they aren't the same lol
-
If they are the same, then please explain why enabling the php.ini file does not display errors on one Internal Server Error, whereas HTTP 500 does display errors?
I know what die; does, im just saying that I don't usually need it.
-
Ah, I have figured it, i forgot to write "Location: ..."; and also needed the die; for some reason... (don't usually need die;)
-
It wasn't a HTTP 500... Re-read my post and you'll see its an "Internal Server Error", nothing about http 500...
-
it can't be the select function because most of the site usues it and they all work fine and done the same way as above.
-
val.php... where all the validation for the site goes.
the user enters data into the login area on the home page, which then gets passed through val.php (above) which if successful redirects to profile.php...
-
Hi, I have a problem in which I have validation that allows a user to sign into their account if they didn't provide an email address upon registration (which when they do provide an email address they get sent a generated password in their welcome email).
Once they manage to sign in without the password they will get directed to a page where they will be asked to create one.
However when this process happens it does not log them in, it just comes up with an Internal Server Error.
My code is below:
if(!empty($_POST["login"])){ $logUser = $_POST["logUser"]; $logPass = $_POST["logPass"]; if(empty($logUser)){ $logErr .= "<li class='error'>You can't sign in without providing your registered username.</li>"; } $grabUserData = select("username, password", "users", "username = '$logUser'"); $data = mysql_fetch_assoc($grabUserData); if($data["username"] != $logUser){ $logErr .= "<li class='error'>Invalid username</li>"; } if(empty($logPass) && $data["password"] == "da39a3ee5e6b4b0d3255bfef95601890afd80709"){ session_start(); $_SESSION["UserProfile"] = $logUser; $_SESSION["UserID"] = $data["user_id"]; header("profile.php"); } }
Any ideas would be useful.
- Lily
(I don't want posts about the security of my processing code... if I wanted opinions on this I would ask.)
-
Since clearly help can't be given, I have done things a bit differently and managed to get the same result. Therefore this topic is solved.
-
Hi, I have a problem where my while loop isn't displaying what I have asked it to display, everything works except for this while loop and I can't see what the problem is code below:
while (false !== ($entry = readdir($handle)) && $fileGet = mysql_fetch_assoc($getfile)) { if ($entry != "." && $entry != "..") { $listFile = '<td>'.$entry.'</td>'; echo '<tr>'; echo '<td>'.$fileGet["file_name"].'</td>'; echo $listFile; echo '<td>'.$fileGet["file_url"].'</td>'; echo '<td><a href="delete-file.php?id='.$fileGet["file_id"].'&file='.$fileGet["file_original"].'">Delete</a> </td>'; echo '</tr>'; } }
Any Ideas? Thanks - Lily
-
Actually Javascript isn't easier as you would have to make sure the javascript works accross all major browsers. Especially IE7 as it seems that one is most problematic.
-
as SocialCloud said, AccountCreation.php shouldn't have to be complex, after all you said it is dealing with $_POST variables so surely it is only checking them for empties, maybe a few text patterns, and depending on your site content and what the users profile is maybe even directory creations? these should all be able to be put into a function without any problems. the only changes you would have to make is how it outputs any messages from validation.
-
UM...... Whats the actual problem? If you want people to physically help you with developing this then you to re-post this in the free-lance section or ask a mod to move it.
Secure Registration
in PHP Coding Help
Posted
Hey,
I have written a secure registration form, and I have wondered could could be done to make even more secure. (I want to try and avoid capchas if possible.)
Here is my current code: