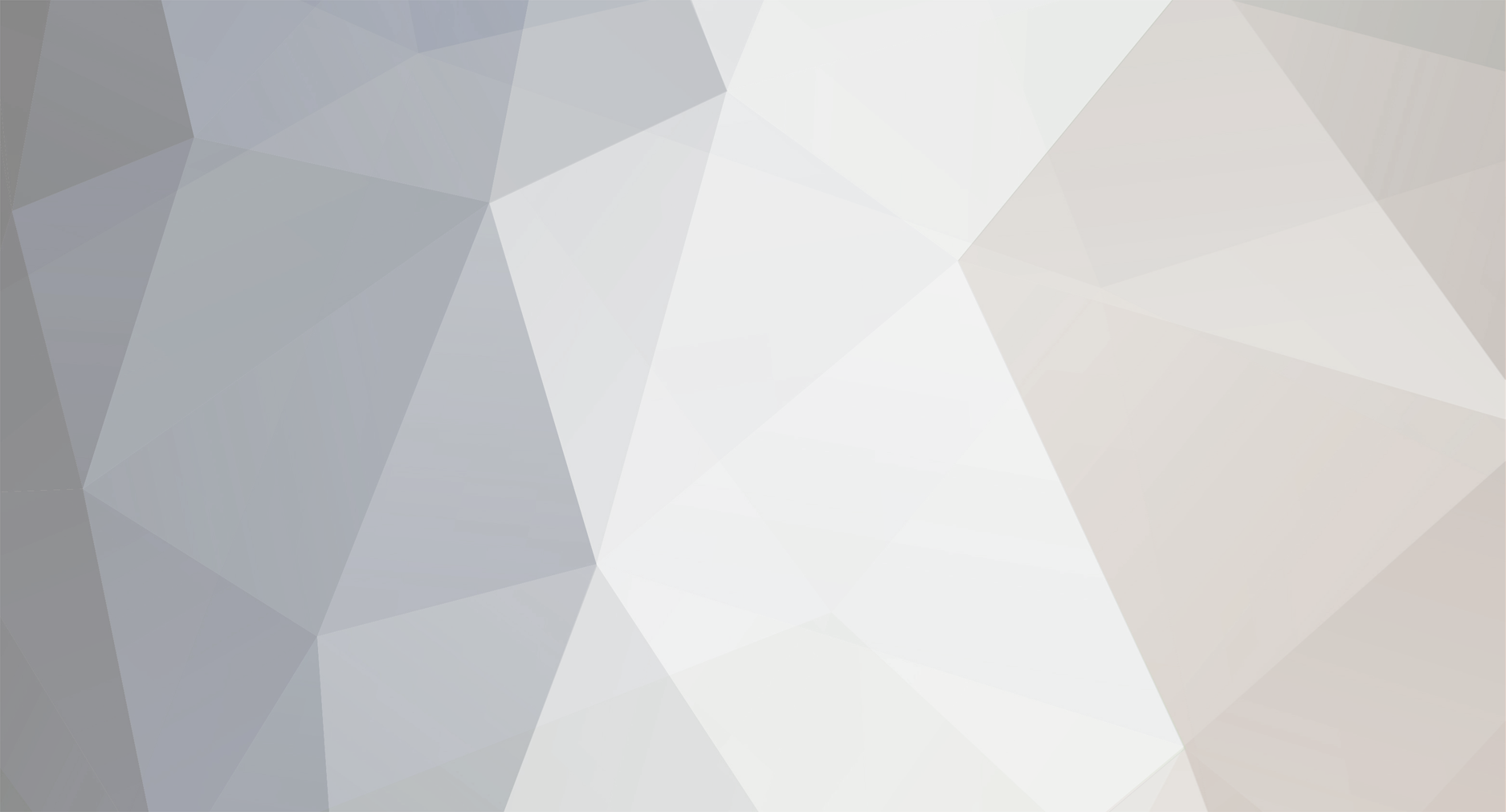
davidannis
Members-
Posts
627 -
Joined
-
Last visited
-
Days Won
2
Everything posted by davidannis
-
Earlier I posted about how to best deal with a calculated field in MySQL and was advised not to store the value in the database. So, I took my calculated value out of the tables. I can select based on the calculated value SELECT ... right_count, wrong_count, right_count + wrong_count AS Total_Count, right_count / (right_count + wrong_count) AS Right_Percent ... but now I want to UPDATE based on Right_Percent. The old code was: UPDATE leitner_vcard_boxes SET box=box+1 last_reboxed_date='$today' where box<7 AND last_reboxed_date<'$cutoff_date' and right_percent<='$score' The only way I can think of updating is something like: UPDATE leitner_vcard_boxes SET box=box+1 last_reboxed_date='$today' WHERE box<7 and last_reboxed_date<'$cutoff_date' AND Right_Percent IN (SELECT right_count, wrong_count, right_count / (right_count + wrong_count) AS Right_Percent FROM leitner_vcard_boxes WHERE Right_Percent,='$score'); Will using a subquery even work? Is there a better way? To further complicate things, I loop through 5 score/cutoff date pairs (so that a lower score gets reboxed faster than a higher score). I imagine it would be more efficient to try to combine it all into a single query. $cutoff_scores=array(25=>7,50=>14,75=>28,90=>40); foreach ($cutoff_scores as $score => $rebox_interval){ $today=date('Y-m-d'); $interval=$rebox_interval.' days'; $cutoff_date=date_sub($today, date_interval_create_from_date_string($interval)); $query="UPDATE leitner_vcard_boxes SET box=box+1 last_reboxed_date='$today' where box<7 and last_reboxed_date<'$cutoff_date' and right_percent<='$score'"; $result= mysqli_query($link, $interval); }
-
That is a broad question. Do you want to link bank accounts? Do you want to maintain a balance for each user? There are legal issues. Paypal got banking licenses in Europe. How do you intend to get money in and out of your system? You need to use Ajax. If you Google "search completion ajax" you'll find examples.
-
2 rows 'found', but only data for 1 row returned
davidannis replied to stubarny's topic in PHP Coding Help
Is the code you show in a bigger loop? The error message says line 26 but it is about 8 lines in. -
I think what you want is: $start = $_GET['start']; // value="1994" $end = $_GET['end']; for ($x=$start ; $x<=$end; $x++){ $years .= 'T'.$x.'Year' ; }
-
Storing SQL Selects in a table and running them in PHP
davidannis replied to bazianm's topic in PHP Coding Help
I'm thinking use a delimiter to pick out the variables. You can then do something like this: <?php $var='id'; $id='123'; $select='SELECT * FROM mytable WHERE id=\'~id~\''; $answer= str_replace(('~'.$var.'~'), $$var ,$select, $count); echo $answer; ?> You can pass str_replace an array of values to replace multiple variables in one pass. -
Storing SQL Selects in a table and running them in PHP
davidannis replied to bazianm's topic in PHP Coding Help
You can use str_replace like this: <?php $var='$id'; $id='123'; $select='SELECT * FROM mytable WHERE id=\'$id\''; $answer= str_replace($var, $id, $select, $count); echo $answer; ?> There must be more elegant solutions with preg_match to make sure you get variables that are whole words ($apple not treated as $a.'pple') and I'm guessing with $$ -
Storing SQL Selects in a table and running them in PHP
davidannis replied to bazianm's topic in PHP Coding Help
$id = "somevalue"; $lcSQL = "SELECT * from ListTable where id = '$id'"; $result=mysqli_query($link, $query); if ($result){ $row=mysqli_fetch_assoc($result); $result=mysqli_query($link, $row['stored_query']); //do stuff here }else{ //handle error here echo 'No stored query for that id'; } -
Paypal also has a PDT service. PDT is faster, but less reliable than IPN because IPN retries after failures and PDT does not. The best approach if you want to give immediate access is to use PDT and have an IPN listener to catch the successful payments that PDT misses.
-
Form does not accept characters like é etc.
davidannis replied to kimikai's topic in PHP Coding Help
While I am all for not reinventing the wheel I used a Pear package http://pear.php.net/package/Mail in a lot of places, but it is no longer being maintained and now I'm going to have to go back and replace and retest. Pieces of that code are depricated and those pieces were written that way largely (I think) to support features that I never used and probably never will. I also hate debugging when I am using code as a black box. So, sometimes it is just easier to write it myself, know that I understand it, and know that I will not have complications from features that I don't need.- 12 replies
-
Form does not accept characters like é etc.
davidannis replied to kimikai's topic in PHP Coding Help
$message="This is a multi-part message in MIME format. --_1_$boundary Content-Type: multipart/alternative; boundary=\"_2_$boundary\" --_2_$boundary Content-Type: text/plain; charset=\"iso-8859-1\" Content-Transfer-Encoding: 7bit $message --_2_$boundary--"; if ($attachment!='') { $message.=" --_1_$boundary Content-Type: application/octet-stream; name=\"$filename\" Content-Transfer-Encoding: base64 Content-Disposition: attachment $attachment --_1_$boundary--"; }- 12 replies
-
Form does not accept characters like é etc.
davidannis replied to kimikai's topic in PHP Coding Help
Try wrapping the part that sends the attachment in an if if ($attachment!=''){ $message .= STUFF YOU ALREADY HAVE HERE } instead of having it included in the message every time.- 12 replies
-
Form does not accept characters like é etc.
davidannis replied to kimikai's topic in PHP Coding Help
In your email header you use a different encoding. Content-Type: text/plain; charset=\"iso-8859-1\" I would try to make the encoding consistent.- 12 replies
-
Form does not accept characters like é etc.
davidannis replied to kimikai's topic in PHP Coding Help
No, that shouldn't be the problem. They must be before the form is displayed so the correct character set should be submitted.- 12 replies
-
Form does not accept characters like é etc.
davidannis replied to kimikai's topic in PHP Coding Help
You need to allow a character set that supports those characters in your html header Instructions are here. http://www.w3.org/International/O-charset- 12 replies
-
All of the records in a box in this case happen to have the same pos value (part of speech) but in many cases that won't be true.
-
I am trying to locate an array in an array of arrays: $card_pos = array_search($target, $_SESSION['leitner']['boxes'][($box - 1)]); if ($card_pos !== false) { // Do my stuff here }echo '<pre> oh no -- didn\'t find '; print_r($target); echo "in ";print_r ($_SESSION['leitner']['boxes'][($box + 1)]);echo '<br>leitner';print_r ($_SESSION['leitner']); echo '</pre>'; but I don't find the target in the array though it is clearly there as you can see in the output: oh no -- didn't find Array ( [pos] => v5g [tense] => agerimasu ) in Array ( [0] => Array ( [pos] => v5g [tense] => agerimasu ) ) leitnerArray ( [boxes] => Array ( [1] => Array ( ) [2] => Array ( ) [3] => Array ( [0] => Array ( [pos] => v5g [tense] => agaru ) [1] => Array ( [pos] => v5g [tense] => agarimasu ) [2] => Array ( [pos] => v5g [tense] => agarimasen ) [3] => Array ( [pos] => v5g [tense] => agaranai ) [4] => Array ( [pos] => v5g [tense] => ageru ) [5] => Array ( [pos] => v5g [tense] => ageranai ) [6] => Array ( [pos] => v5g [tense] => agerimasen ) [7] => Array ( [pos] => v5g [tense] => agerimasend ) [8] => Array ( [pos] => v5g [tense] => agereba ) [9] => Array ( [pos] => v5g [tense] => agareba ) [10] => Array ( [pos] => v5g [tense] => agatte ) [11] => Array ( [pos] => v5g [tense] => agette ) ) [4] => Array ( [0] => Array ( [pos] => v5g [tense] => agerimasu ) ) [5] => Array ( ) ) [denom_total] => Array ( [1] => 0 [2] => 0 [3] => 36 [4] => 40 [5] => 40 ) [denom_max] => 40 )
-
Actually, looking at it again the problem goes a little deeper. If I assume (which I did) that learner_id, student_id, and id are all the same thing and he changed the name to be consistent then it doesn't matter that line 7 doesn't return the id because it only returns a result if the WHERE clause is true (if the id and password pair match). So, he wouldn't need to retrieve it -- he already has it and has validated it because he's matched the user supplied id with the password. However, when he sets it on line 16 he uses student_id which could be different and when he updates the date he's using _GET with yet another name for the id. If the form is using the POST method _GET is going to be empty. To make the script work, you need to name the id one thing and use that name everywhere and you need to use either GET or POST, trying to use both is just going to result in a mess. I agree that the code is very messy and would advise starting over.
-
I think the OP is doing the 2 of the 3 things you point out, just in an unorthodox manner. He retrieves the ID on line 7 and stores it on line 16. He just calls the ID 3 different things - learner_id, user_id, and student_id which makes it confusing. So, now all the OP needs to do is capitalize the first letter of location in the header on line 23, remove the part of the URL after the .php (no ?id=...) and get the id from $_SESSION in Student_Home.php. It would be a bonus if the naming of the id was the same everywhere.
-
On line 19 you use $GET. Do you mean to use $_GET? Also, put the variable to be evaluated in double quotes inside curly braces {$_GET[id]} (like you do on line 23 where it is not needed because that is not an array element). On line 23 you use $student_id but I don't see where you set that variable.
-
Looks like you want to loop through the votes first then loop through the values.
-
Creating Triggers in PhpMyAdmin (Beginner Questions)
davidannis replied to davidannis's topic in MySQL Help
Thank you. That answer is extremely helpful. -
Bought my 12 year old a book on Unity coding yesterday. He's worked through the first 6 chapters and is now trying to teach the 9 year old neighbors. -proud papa
-
I have created a flashcard program to teach Japanese vocabulary. I want to add sound files from native speakers so I have created a page with a recorder that 1. displays a word (on load) 2. allows the native speaker to record the word (on press of a button) 3. uploads the recording to the server (on press of another button) I wrote the word display as a separate php script so that I could use Ajax to just change the word being recorded, so that I would not need to reload the entire page every time the speaker switched to a new word. The js that gets the word to be recorded is: function ajaxRequest(){ //declare the variable at the top, even though it will be null at first var req = null; //modern browsers req = new XMLHttpRequest(); //setup the readystatechange listener req.onreadystatechange = function(){ //right now we only care about a successful and complete response if (req.readyState === 4 && req.status === 200){ //inject the returned HTML into the DOM document.getElementById('cardData').innerHTML = req.responseText; }; }; //open the XMLHttpRequest connection req.open("GET","nextcard.php",true); //send the XMLHttpRequest request (nothing has actually been sent until this very line) req.send(); }; The section that uploads the file is: $('#send').click(function(){ $.jRecorder.sendData(); } and it gets the filename from another script: $.jRecorder( { host : 'http://localhost:10088/NewFolder/japanese/jrecorder/acceptfile.php?filename=hello.wav' The problem that I have is that when I get each word I want to change the name of the file being uploaded. It all works and if I reloaded the page each time I could do this: $.jRecorder( { host : 'http://localhost:10088/NewFolder/japanese/jrecorder/acceptfile.php?filename=<?php echo $word.'_'.$reader_id;?>.wav' but I want to stay on the Ajax path. So, I think that I need to convert the newcard program to return json with something like: $response=array(filename=>$word.$reader_id, card_data=>$card_stuff); echo json_encode($response); but once I get the json back I don't see how I get the filename into the script that sets the upload url and make that script re-execute. Is there a reasonable way to do that?
-
No, old ugly code is still legacy code in my opinion.
-
I believe there is a small typo here. Make sure that you have a space between Location: and http://