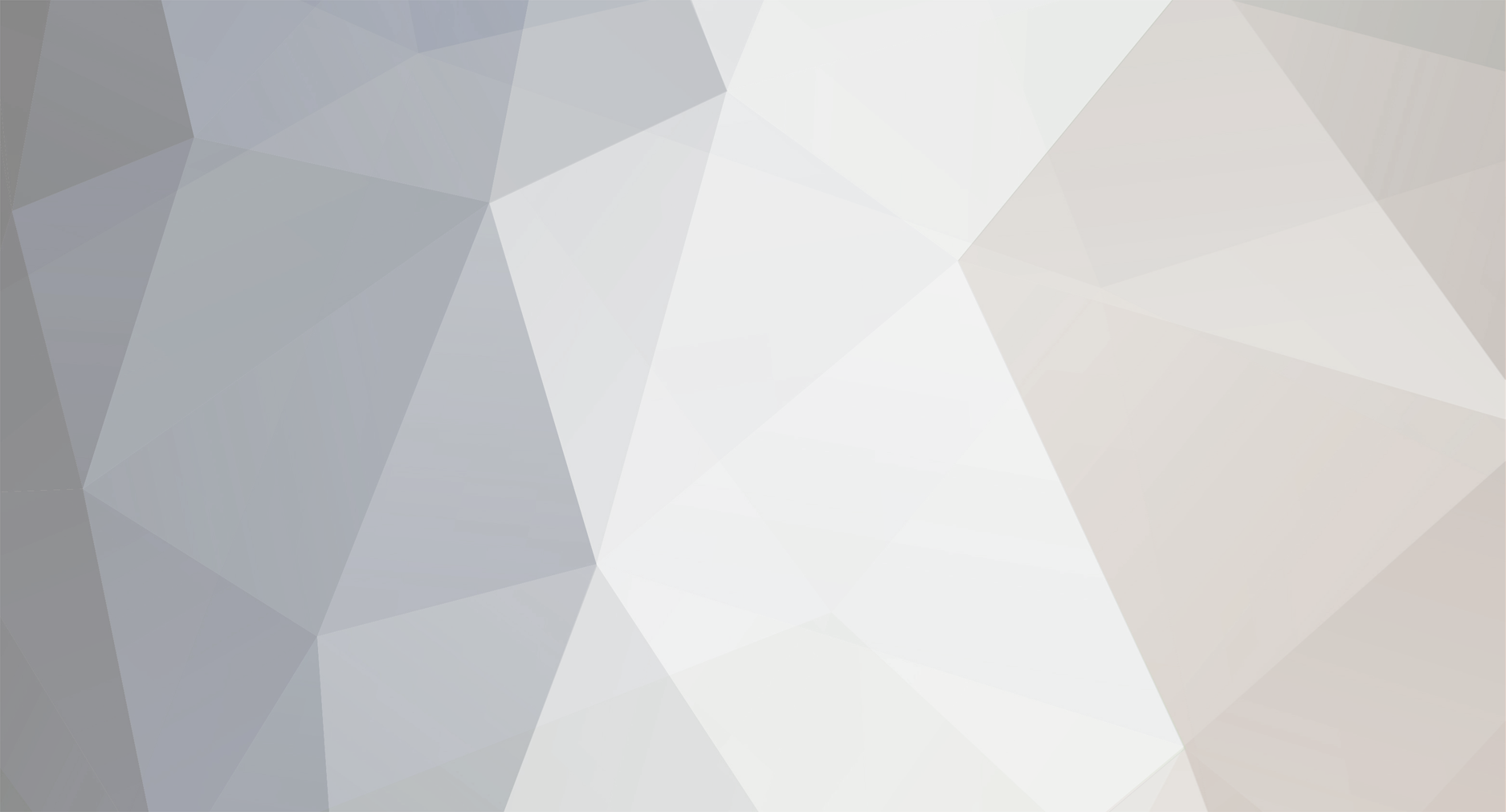
rwhite35
Members-
Posts
159 -
Joined
-
Last visited
Everything posted by rwhite35
-
Okay, here's a question for the collective conscience that is PHP Freaks. How do you overcome a serious (or even moderate) case of Developers Block... I mean I'm hang'en round forums, answering posts, playing my guitar, talking to the walls, you name it. Just can't seem to get past a particular juncture in an development process. It's not a syntactical issue and I have a road map and all that best practice stuff... Its a motivation issue. You get about 5K + lines of code in to a project and POW... You hit a wall... Any and all suggestions are appreciated and will likely be at least considered as viable options.
- 5 replies
-
- programming
- applications
-
(and 1 more)
Tagged with:
-
Object Oriented Programming was tough to get a handle on at first. But then once you get it, it will make development and maintenance SOOOOOO much simpler. Here is simple class that returns yesterdays day and date based on today. Its silly, but has all the machinery for class and objects. <?php class whenWasYesterday { public $today; //class property, assign date input public $resultOut; //method variable for output public function __construct($t) { //constructor assigns input to properties $this->today = $t; //this property now has global scope } /* method to output class result calls a private class method with $this which means "this instance" of the class. @return string, for output to browser */ public function returnYesterday() { echo "And yesterday was ".$this->calcYesterday(); } /* method to calculate yesterday, only the class can modify this. @param int, $this->today, timestamp @returns string, example Thursday June 2013 */ private function calcYesterday() { if(!empty($this->today)){ $resultOut = date('l F Y',($this->today - 86400)); }else{ $resultOut = "What happened to the time?"; } return $resultOut; } } ?> <!DOCTYPE html> <html lang="en"> <meta charset="UTF-8" /> <head> <script javescript> //define this moment var now = new Date(); </script> <?php //PHP is server side, so this now is actually sooner than the JS now... $now = time(); $phpObj = new whenWasYesterday($now); ?> </head> </body> <p>Today is <script language="javascript">document.write (now);</script><br> <?php print $phpObj->returnYesterday()."<br>\n"; ?> So there you have it!</p> </body> </html>
-
Have a look at this post, where I've given a simplified PDO example.
-
This is where PDO really excels. You can make multiple queries on the database with little performance cost. PDO is a prepared query statement that you apply variable data to with each query. So you have a table of airline codes (SWA, DAL, TAN), Your query would look something like this: $sql = "SELECT COUNT(*) AS Nr, SUBSTR(Callsign, 1, 3) as Airline FROM {$dbprefix}Reports WHERE PilotID=? AND CallSign=? GROUP BY Airline ORDER BY Nr DESC LIMIT 5"; Each time through your would bind the $userid to PilotID and the $airlineCode to CallSign column(not sure of you tbl structure). The results set then would be only the records with matching $airlineCodes. The results set can then be assigned to multi-dim array as Kicken suggest. The array would look something like: array ( [0] => ( [SWA] => ( [0] => swa123 [1] => swa345 [2] => swa1524 ) ) [1]=> ( [DAL] => ( [0] => dal321 [1] => dal543 ) ) ) Where the outer array [0] would be each result set.
-
Oops sorry, use array_key_exists, not in_array. Was going off the top of my head. So: if (array_key_exists($key, $_POST)) { //returns true on a match
-
So this looks like a method in a larger class, correct? Depending on how you are passing the start the end timestamps will determine what kind of looping function you can use. If you were to pass a parameter like this: <?php //set by the user $params = array($_POST['timestampS'],$_POST['timestampE']); $yourObj = new yourClass($params); $yourObj->interfaceMethod(); Then inside your class: public function interfaceMethod() { foreach ($this->params as $k=>$v) { // set $this->params with a constructor so it has global scope $result = $this->Leave($k); //where $k is either timestampS or timestampE } return $result; } You'll then change the Leave method to something like private function Leave($key){ //private function Leave(){ if (in_array($key, $_POST) { //returns true on a match //if(isset($_POST["Leave"]) && !empty($_POST['timestampS']) && !empty($_POST['timestampE'])) { The rest can remain largely the same, except where youve changed the table and query ti reflect the change in start and end columns. This is all pseudo code, but what important is you need a method that loops through your parameters and calls the private method Leave with each timestamp. Hope that helps.
-
adding to an array with each recursive function
rwhite35 replied to 1internet's topic in PHP Coding Help
Look in to variable scoping. The array outside your function has one structure and the array inside your function another. Also, check out PDO or mysqli instead of mysql_. Here's you code: <?php define("DB_HOST", "localhost"); define("DB_NAME", "databasename"); define("DB_UNAME", "dbusername"); define("DB_UPWORD", "dbuserpassword"); $structure = array(); $x = array(22,23); print_r($x); try{ $_conn = new PDO('mysql:host='.DB_HOST.';dbname='.DB_NAME.'', DB_UNAME, DB_UPWORD); } catch (PDOException $e){ print "Error: ".$e->getMessage()."<br>"; die(); } function runStructure($value, $_conn, $array) { $query = "SELECT `parent_id` FROM `categories` WHERE `categories_id`=?"; $stmt = $_conn->prepare($query); $stmt->bindParam(1,$value); $stmt->execute(); $row=$stmt->fetch(PDO::FETCH_ASSOC); $array[]=$row['parent_id']; //echo $row['parent_id']; return $array; } foreach($x as $k=>$v) { $structure = runStructure($v,$_conn,$structure); } print_r($structure); ?> Couple things to note. The database connection is outside the function. This way you only have one db connection. Two, the $x variable should be an array of all your id you want to query. Finally, you see at the bottom, I'm looping through each $x array element and calling the function on the individual nodes. This code block works here: Try it out. -
I'll use OOP style when I have a repetitive pattern that will be used on multiple pages. One way to think of Classes would be functions on steroids. A popular choice for OOP are database connection and query. Where the connection and query machinery are contained inside a class. Then you can pass the class parameters specific to a pages data pull. The result back is an object (array or value) that can be manipulated and accessed like an array or variable. Another use to try when learning OOP is HTML form generation. If you have a multi-step process and want to present specific fields to certain users, a simple form class for each user is handy. There are a lot of OOP tutorials out there, specially for database interaction. Good Luck!
-
Dude, you need to eat that elephant one byte at a time... Have you tried anything? And if so, where are you getting stuck or what happens that isn't supposed to? Break you process down in to steps and build your output from there. Then you can test each step in the process.
-
You'll want to run a query like: $count_result=$mysqli->query("SELECT count(*) FROM tbl_name"); which will return the total row count (ie 100) then you can divide that by the number of results per page. Once you have that you can run additional queries using: SELECT SQL_CALC_FOUND_ROWS * FROM tbl_name LIMIT $start, $perpage where the starting record is the next record after the last query (say 20 per page). First time through $start = 0 and $perpage = 20. The next time through, $start = 21, $perpage = 20. Sorry left out a critical part, $count_result is used to define $perpage. So in the 100/20 example, you would generate 5 pages with 20 results each.
-
I would suggest you drill your question down to a specific problem with a specific routine or algorithm. What have you tried so far and is this script generating any errors or results... I think we'll be more a help to you if you can keep it as simple as possible.
-
New rows not working, overwriting last entry in cell.
rwhite35 replied to FrankieGee's topic in PHP Coding Help
Hi Frank, Taking a look at your file, couple suggestions that might help you debug (there's a few). Enable Firebug on your Firefox browser, click the Consule tab and re-run your script. You'll discover a few Javascript errors. For PHP you can setup an error log that will wright your custom errors out to a text file. I like to do this wherever I'm trying to visualize whats going on under the hood. The following code is how you would use this: <?php //log custom error messages $log_path = "/path/to/your/log/file/dev_log_messages.txt"; $log_tstamp = date('Y-m-d G:H:s',time()); //then later in your code blocks $someVariable = "Test String"; error_log($log_tstamp." the test string is ".$someVariable."\n",3,$log_path); Using this technique will help you identify values or result that don't display to the browser window, but are critical to the process success. Couple overall thoughts, ideally, you want your PHP code block above your HTML code block. Specially since you are using sessions, the PHP session header needs sent before anything else. <?php session_start(); //some php code ?> <html> //some javascript and html </html> Sorry this doesn't answer your question directly, but in the long run, it will help you code better and faster. -
What was the error?
-
I should add to this and say if you have already ran the PHP code and output the page, then any client side functionality (like hiding checkboxes) should be handled on the client side. If on the next step, you only want to show the checkboxes that were not (or were) selected, then you would have another PHP code block on the next page to display what you want. Does that make sense?
-
You might want to try a JQuery approach. <script type="text/javascript" src="http://code.jquery.com/jquery-latest.js"></script> <script type="text/javascript"> $(document).ready(function() { $('input[type=checkbox]').click(function(){ $('input[type=checkbox]').hide(); //alert("That was clicked"); }); }); I only tried it with on checkbox, but this should give you some direction.
-
You're going to run in to the Same Origin Policy which basically restricts server A remote code from running on server B. API's and SDK get around this policy by using keys and single source signon schemes. You can load a remote page in an <iframe> tag, however that code can't make runtime instructions without some special setup (reverse proxy server).
-
Okay how about this: Step One, first time through ($_POST would not be set): initialLoad.php $host = "xxxxxxx"; $port = 'xxxx'; $dbname = "xxxx"; $db_username = "xxxx"; $db_password = "xxxxx"; $dsn = "sqlsrv:Server=$host,$port;Database=$dbname;"; // Connect $db = new PDO($dsn, $db_username, $db_password); try { $sql = 'SELECT * FROM MainJobDetails WHERE JobNo = ?'; $q = $db->prepare($sql); $q->execute(array($_POST['jobnumber'])); // Return results while($row = $q->fetch(PDO::FETCH_ASSOC)) { echo '<h2>Scan the barcode on the label or click "Local Delivery"</h2>'; echo '<form id="update" action="processInput.php" method="post">'; echo '<table class="results"><tr><th>'; echo 'Customer'; echo '</th><th>'; echo 'Description'; echo '</th><th>'; echo 'Consignment #'; echo '</th><th>'; echo 'Local Delivery'; echo '</th></tr><tr><td>'; echo $row['InvoiceCustomerName']; echo '</td><td>'; echo $row['JobDesc']; echo '</td><td>'; echo '<input type="text" name="consignment" id="consignment"/>'; echo '</td><td>'; echo '<input type="checkbox" name="local" id="local"/>'; echo '</td></tr></table><br />'; echo '<button type="submit" id="updatebutton" class="blue button"/>Update details</button></form>'; return; } } catch(PDOException $e) { echo $e->getMessage(); } Now process the input: processInput.php: session_start(); if($_SERVER['REQUEST_METHOD'] === 'POST'){ $_SESSION['InvCustName']['cons'] = $_POST['consignment']; $_SESSION['InvCustName']['local']=$_POST['local']; } if(!empty($_SESSION['invCustName']['local']) { //do some additional processing like location redirect to the next step } else { //send them to an alternate step since they didn't check off the local box. } Using this strategy, you can better control the users flow through whatever your process might be. Hope that makes more sense. I've left out most of the HTML output for brevity.
-
your trying to concatinate two variables but using a logic operator. Changes this: $userName = filter_input(INPUT_POST, "userName") && $bName = filter_input(INPUT_POST, "bName"); To this: $userName = filter_input(INPUT_POST, "userName").",".$bName = filter_input(INPUT_POST, "bName"); //or just $userName = filter_input(INPUT_POST, "userName").$bName = filter_input(INPUT_POST, "bName"); or as boompa suggest $userName = filter_input(INPUT_POST, "userName"); $bName = filter_input(INPUT_POST, "bName"); echo $userName."".$bName;
-
PHP file: test_php.php <?php echo "Hello World"; ?> from the command line prompt: yourname$ php test_php.php stndout: Hello World
-
Change session_is_registered to $_SESSION['myusername']. session_register, session_is_registered(), and session_unregister(). are all deprecated. To assign value to your session, use $_SESSION['myusername'] = "your string";
-
I would suggest looking at one of PHP's Image manipulation libraries. Personally, I prefer GD, but there are others. You would output one set of ten cards, then make that in to a JPEG, PNG or PDF template. You could then send the job to the printer and each print out would be identical. If each business card is a different individual's contact info, then you would run the first ten > print and then run the next ten and so on. Your program would have to keep track of the last card ran, but that would be the most effecient approach, at least in my mind.
-
In a Model Control Viewer methodology, you should be handing each step to a separate script. That way you can keep track of where you're in the registration process. At the end of the first step, I would write your POST data to a sesssion variable(assumes you scrub the POST input first). Then in subsequent steps you can add to that session data. In the final step, you then process the session variable however you choose.
-
Trying to insert variables inside an EOD statement
rwhite35 replied to jbegreen's topic in PHP Coding Help
Hi, it looks like you're trying to use a HEREDOC syntax. Your script would look like this: <?php $var1 = $_POST['someInput']; $var2 = $_POST['moreInput']; $output = <<<EOF This would be any text. to add your variables, you would $var1 and $var2. Then on the last line of text, make sure you close the HEREDOC. EOF; //close your script ?> <doctype html> <html lang=en> <head><title>Try Me</title></head> <body> <?php //now print out to display print($output); ?> </body> </html> try that. -
Grab or get the email address and given name.
rwhite35 replied to aj123cd's topic in PHP Coding Help
Using the following may help you visualize your array better: echo "<pre>" print_r($your_array); echo "</pre>"; Then to access your data, you would need to loop over the outter array then any sub arrays using foreach($your_array as $value).