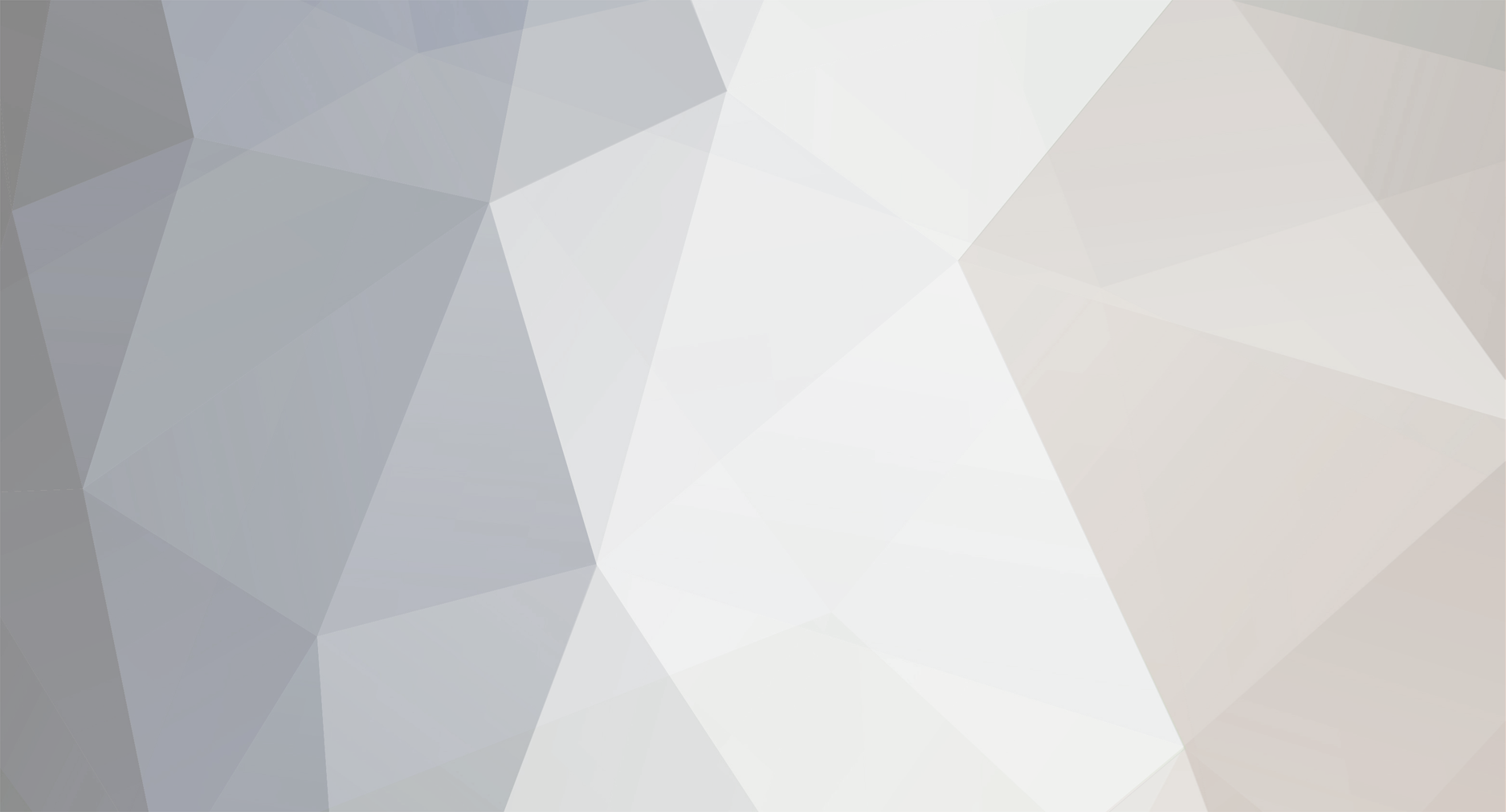
rwhite35
Members-
Posts
159 -
Joined
-
Last visited
Everything posted by rwhite35
-
creating a Link to customers page using search
rwhite35 replied to moosey_man1988's topic in PHP Coding Help
If you want to create the links server side, the script that produced the result needs to have that logic. If you want to create links on the client side, you'll need to look at some JQuery or Javascript functionality. Personally, I prefer the server side logic. Can you post the code that creates the page result, specifically the code block for each result? -
textbox items display when listbox item selected in php form
rwhite35 replied to sathish16's topic in PHP Coding Help
What have you tried? Please share some code and any errors you are getting. Otherwise, we can't be much use to you. -
I would like to add to QuickOldCar, not everything requires OO either. Unless you are building some large framework or need an application interface when working with a team of developers, OO might be overkill. Functional Programming is the better choice when you need server side processing on "trivial" (relative to project scope) functionality. That said, OO is important and should eventually be in your learning path.
-
Alternatively, if the values of A and B are not variable, you can run something like: SELECT C_fld FROM test WHERE A_fld + B_fld=260; Where 260 is the sum of A and B.
-
Hey All, Need to pass the current window width to a PHP class which creates a slide show presentation. The presentation image sizes need calculated for all devices including mobile and smart phone (mobile web). Problem is, PHP is server side and doesn't care about the windows width. I'm using Javascript to acquire the window width and pass that value to PHP - before calling the class that create the presentation. My workaround is using Output Buffering: <php ob_start(); //starts buffering ?> <script> var wwidth = screen.width; document.write(wwidth): //output </script> <?php $windowwidth = ob_get_clean(); //works error_log("Window width is: ".$windowwidth); //continue on with the rest of the script ?> I dislike ducking in and out of PHP like this. And I'm not able to use Javascript XMLHttpRequest because I need the size before the class is called, on the current page. Any alternative suggestions? Thanks in advance
- 1 reply
-
- ob_start
- xmlhttprequest
-
(and 1 more)
Tagged with:
-
What happens if you have to add two or three more users? You might want to reconsider your database schema. One table for your users, one table for the leads rotation. In that way you separate the data into manageable concepts. Then when you lookup who had the last lead, you could prioritize a rotation with some simple logic. If 5 was the last to get a lead, then start the rotation from the top. Good luck.
-
You can use JSON encoding to pass variables from PHP to Javascript. I prefer a JS Object as apposed to a variable. <script> var coordObj = new Object; coordObj.postcode = <?php echo htmlspecialchars(json_encode($postcode), ENT_NOQUOTES); ?>; coordObj.latitude = <?php echo htmlspecialchars(json_encode($latitude), ENT_NOQUOTES); ?>; coordObj.longitude = <?php echo htmlspecialchars(json_encode($longitude), ENT_NOQUOTES); ?>; </script> Then to access your JS object, included later one in the same page render (this is not asynchronous). <script> var postcode = coordObj.postcode; var latitude = coordObj.latitude; var longitude = coordObj.longitude; //now do the rest of your stuff </script>
-
hiding a menu option based on user role. Not WP
rwhite35 replied to laflair13's topic in PHP Coding Help
Something else to consider. You're using your class like a glorified function. The class should return an object, like a car or your user... Then in your login script, you can assign values to the session. Generally, speaking if you have "privileged" content, you would probably want to store a way of ID'ing the user. Example: the public might be 1, admin 2, superadmin 3. Pseudo table: user_name | user_cred public | 1 admin | 2 sadmin | 3 So the login script would change this way: if(isset($_POST['login'])) { $email = filter_var($_POST['email'],FILTER_SANITIZE_EMAIL); $password = filter_var($_POST['password'],FILTER_SANITIZE_STRING); $userObj = new Users(); $credentials = $userObj->login($email, $password); } if($credentials) $_SESSION['loggedin'] = $credentials['user_cred']; Then in your class: if($stmt->num_rows == 1) { $credentials = $stmt->fetch_assoc(); } return $credentials; //prototype Array([email]=>string, [password]=>string, [user_cred]=>int) Now your have a means to evaluate conditions where users with different loggedin values can access different things. Hope that help. -
Capture user information and store in Database
rwhite35 replied to rajeshkr's topic in PHP Coding Help
Assuming your data is stored in a MySQL database, check out BETWEEN clause. Also it would probably help if you had a specific questions. Your question is broad and covers a lot of machinery. -
assuming $row_count holds the actual count, you want something like: if ($i == $row_count) { echo "</tr><tr>"; $row_count = $row_count + 7; } This is doing a couple things. first it creates a counter ($i). before you start your foreach loop, add this line: $i=0; once inside the foreach loop, your want to evaluate the if statement above. If the increment count is is equal to the row_count, add a return. Then to continue the incremental count, and still inside your foreach, increment the counter by one, for each loop. Put it all together, should look something like. $row_count = 7; $i=0; foreach($images as $image){ echo "<td><a href=" . $image['galleryurl'] . " target=\"new\"><img src=" . $image['gallerythumb'] . " height=\"160\" width=\"160\" alt=\"" . $image['gallerydesc'] . "\"></a></td>"; if ($i == $row_count) { echo "</tr><tr>"; $row_count = $row_count + 7; } $i++; } Note I've overloaded the variable $row_count, this way $row_count will have the value 7, 14, 21, 28 etc.. Give that a try.
-
The error reporting looks like your being denied SMTP because you're not logged in. Do you have access to sendmail and have you tried setting that instead of SMTP? Reason for delay in error message is because your mail server is set to try and send your mail and fails after a set period of time. Also, your sending the test from you - to you... That email never leave your machine, those are handled differently then mail to remote address. Try another outlook.com address, outside your development server, if you can.
-
This may seem obvious, but it has't been mentioned. Have you turned on SMTP error reporting? /* * $mail->SMTPDebug = 0; disable debugging, 0 is the default) * $mail->SMTPDebug = 1; echo errors and server responses * $mail->SMTPDebug = 2; echo errors, server responses and client messages */ $mail->SMTPDebug = 1; I'm not as familiar with PHPMailer, but with other classes/frameworks, you can pass additional headers. That may be where you want to research, specifically for MS mail. BTW, I've used part of your ajax script to update on of my own. I like how your handling post submit UI feedback. Good luck
-
@tobimichigan Yeah, there is ten to the power of 2 ways to skin a cat.... I use JQuery and AJAX and have done it both ways... Any case, check lines 82 and 92, I think you need: contentType: 'application/x-www-form-urlencoded; charset=UTF-8'
-
L42 in DBManipulate.php, I don't see where your form has an action="#" or method="post" which would trigger the processing.
-
instance object best practice (for optimization)
rwhite35 replied to rwhite35's topic in Application Design
Ignace, thanks for the reply. I plan to do some benchmarking (if I can get to it) this week. I'll post any results here. This occurs often in large scale projects (over 50K lines of code), but it seems like its more preference than a hard fast rule. That's my sense of it anyway. Thanks again, I appreciate your insights. -
Q: Should instancing an object be held off until needed - or - instanced early and held in memory until (it may/or may not) be called? Here is an example of the type situation where an custom error reporting class is instanced only when some test has failed. The error reporting is for authorized users running an application from protected sub sections(RESTish solution). /* * check form input and $_FILES upload * if test fails, instance errorwrapper::factory object and call errorReport method * testfilesize function, compares file size limit, set pass1 to true on success * testfiletype function, compares file to allowed MIME types, set pass2 to true on success * svalidate returns array $filteres, sanitized input on success, error message(s) on fail * param boolean $pass[1,2], set initial test condition * param int MAX_FILE_SIZE, constant defines max file size allowed. */ $pass1 = false; $pass2 = false; if(isset($_FILES)) { $ftype = $_FILES['img_file']['type']; $fsize = $_FILES['img_file']['size']; testfilesize($pass1,MAX_FILE_SIZE,$fsize); testfiletype($pass2,$ftype); } if($pass1 == false) { $erparams=array('cat'=>3,'src'=>"addform_control",'ref'=>"addform",'fault'=>"over file size limit"); $errObj=errorwrapper::factory($erparams); $errObj->errorReport(); exit(); } elseif ($pass2 == false) { $erparams=array('cat'=>3,'src'=>"addform_control",'ref'=>"addform",'fault'=>"illegal file type"); $errObj=errorwrapper::factory($erparams); $errObj->errorReport(); exit(); } /* * process form input, all fields checked before returning filteres * could report multiple errors, mode sets level of sanitization. */ $filteres = svalidate($_POST,$mode=1); if($filteres['exit']==1) { foreach($filteres['err'] as $err) { $mes .= $err."\n"; } $erparams=array('cat'=>2,'src'=>"addfo",'ref'=>"list_viewer",'fault'=>$mes); $errObj=errorwrapper::factory($erparams); $errObj->errorReport(); exit(); } else { //continue processing here, error checking over } In this case, the object $errObj is only instanced on a failed condition. However, $errObj could be instantiated before the conditional test and only calls the method when there is a failed condition. It seems like "six to one half dozen" but these applications are large and complex, so I'm looking to squeeze out any worthwhile optimization. Thanks for considering the question.
-
How to insert a multiple textbox with the same name
rwhite35 replied to Murtaza66's topic in PHP Coding Help
Check out this post here. You're looking to batch all 15 forms in to one transaction. Also read ConNix comment on prepared statements. Prepared statements will automatically optimize your POST data and it is more secure then what you have. -
How to insert a multiple textbox with the same name
rwhite35 replied to Murtaza66's topic in PHP Coding Help
To answer your question more directly. Here is one way to access your post variables. $producten = $_POST['Producten'][0]; //would be the first producton select item -
How to insert a multiple textbox with the same name
rwhite35 replied to Murtaza66's topic in PHP Coding Help
First, use mysqli instead of mysql for your database functionality. Next write a simple script to output your data on post. I think you'll see post data differently then you're expecting. /* form.php script */ <form action="seepostdata.php" method="post"> ... form fields, etc Then in your seepostdata.php script: /* output post array to viewer */ echo "<pre>"; print_r($_POST); echo "</pre>"; This should output something like Array ( [Producten] => array ( [0]=>"first option selection", [1]=>"next option selection", [2]=>"etc etc" ) [ProdOms] => array ( [0]=>"first string", [1]=>"another string", [2]=>"etc etc" ) ) Then copy paste that output to a text file and save it for reference. It is very difficult to visualize a multidimensional array. Which is being created when you name your fields like name="Poducten[]". Good luck. -
In addition to CroNix, look in to PDO transactions. If you have a situation where you're making multiple inserts or updates, it best to use transactions to batch process your queries. Using your example: $queue = array( "INSERT INTO companies (brands) VALUES('Toyota')", "INSERT INTO companies (brands) VALUES ('Volkswagen')" ); //keep adding your inserts try { $dbh = new PDO(DB_HOST, DB_UNAME, DB_UPWORD, array(PDO::ATTR_PERSISTENT => true)); } catch (Exception $e) { die("Unable to connect: ".$e->getMessage()); } try { $dbh->beginTransaction(); foreach ($queue as $query) { $dbh->exec($query); } $dbh->commit(); } catch (Exection $e) { $dbh->rollBack(); echo "Query execution failed".$e->getMessage(); } It seems like more lines of code and more complicated, but it is safer, better and more efficient.
-
This is why I shouldn't visit forums... Now I'm curious as to how to solve your problem... Looking around a bit and you may want to consider a framework like Yii. It allows machine-to-machine interaction is a RESTful manner (behind the scenes). I would characterize my second answer as "Web Services" on the cheap... Reading in a flat file. But a web services framework may be the better solution. At any rate, I'm going to build a similar prototype which will satisfy my curiosity -- thanks to your post.
-
Okay, so if I understand the logic, the user is on their mobile device (iPhone/Android) and they've logged in through the OS web interface (UIKit for example). Once login or register scripts are done, that memory is automatically released. There isn't a 'persistent' state or Automatic Reference Counting(ARC) like with iOS. I think you would need to instance an object inside your app, store the token in memory there, and perhaps re query the database. PHP is stateless. Alternatively, maybe you could write the token to a flat file and compare the token stored in iOS memory to the one written in the text file. Just thinking how I might approach the problem.
-
Input looks good. PDO good, hashing looks good. Unless I'm missing it, I don't see where you're assigning the token to a session variable. Something like: session_start(); $_SESSION['authorized'] = $token; Then on subsequent pages, you would check your users token to insure they're authorized to run the script. That would save you multiple trips to the database in order to check the token there.
-
i need help woth my form validation [;s
rwhite35 replied to Michael_Baxter's topic in PHP Coding Help
Good to know, learn something new everyday. -
New project, question regarding one required spec
rwhite35 replied to steelcitydev's topic in PHP Coding Help
If you want to use PHP (server side tech) to query the db, you'll need to reload the page. Otherwise you're stuck with a complicated JS solution. I think the most efficient strategy would be to cut the video up into :30 second segments, each having its own file. Then you can absolutely control the web users flow through the training session. Including when they have to exit and come back at a later date/time. This way, you store their progress and they can pick up where they left off.