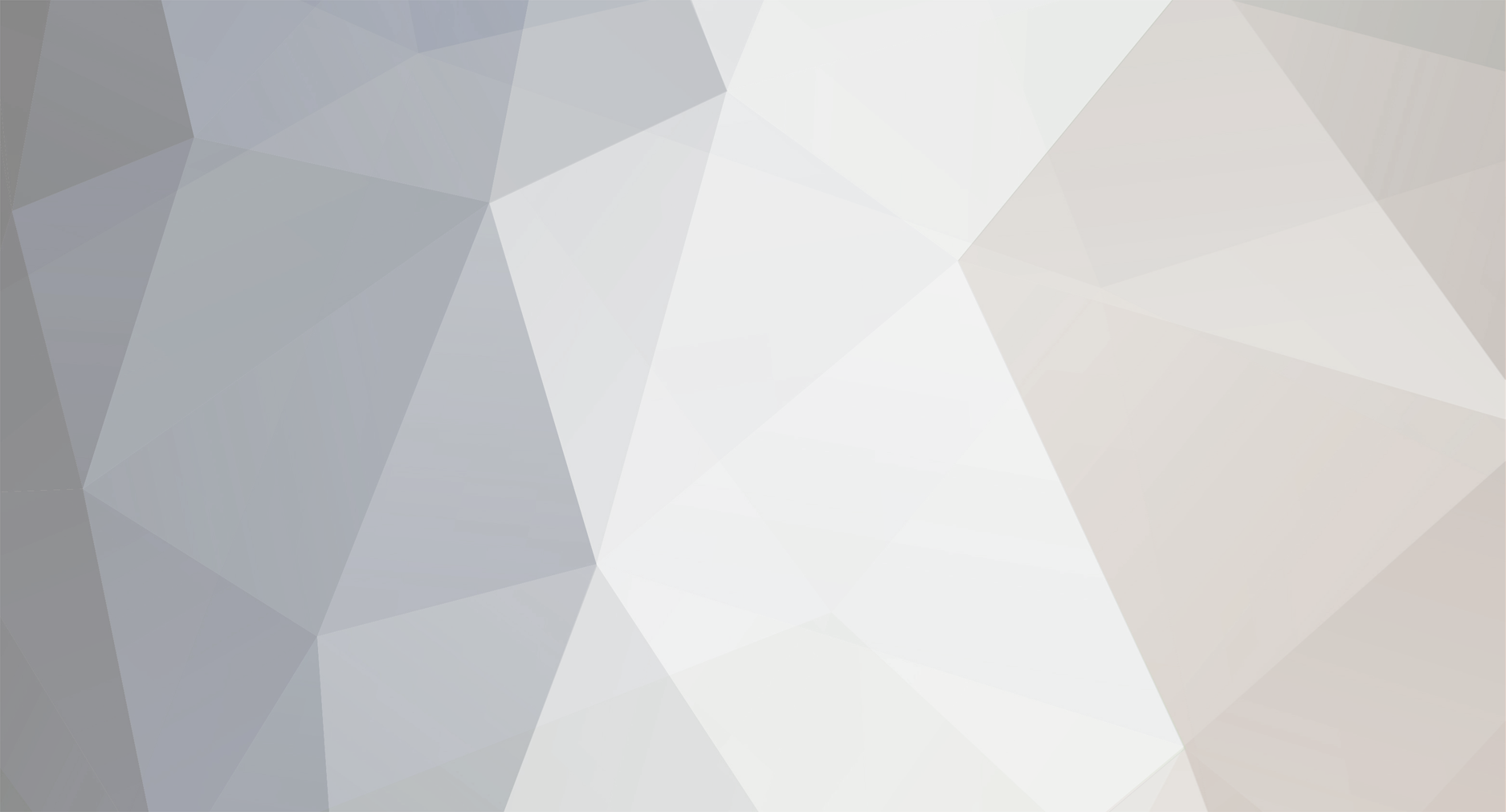
rwhite35
Members-
Posts
159 -
Joined
-
Last visited
Everything posted by rwhite35
-
i need help woth my form validation [;s
rwhite35 replied to Michael_Baxter's topic in PHP Coding Help
Couple items. Where you have error reporting, you could one or other, they're both doing the same thing. The way the variables are being defined is odd. I'm not sure that's valid code. use a simple syntax that's common. $lname = ""; To exit your script on a failed condition, use: if (empty($_POST["lname"])) { $lnameErr = "Last Name is required"; exit(); } else { ... } Start with those changes and see where that gets you. But to answer your question directly, use exit() or die() to end the script. then something like $lnameErr = "Last Name Required."; header ("Location error.php?err=$lnameErr"); //send to previous or error reporting page exit(); -
Stacking function within ternary initial expression
rwhite35 replied to rwhite35's topic in PHP Coding Help
@mac_gyver, excellent point. I hadn't thought about that. @Barand, for the sake of completeness, I'll give that try. Thanks you both. -
I thought I would add this topic since I'm in need of an answer and don't see much ternary used in code examples. Using a ternary operator to assign a file path to a local variable. The path string is conditional, depending on which page id was passed to the script. Once the $_GET variable is evaluated, I like to unset these variables as a personal "memory maintenance" preference (even though garbage collection is automatic). Would prefer to only call unset( ) if $_GET[pageid] is set. The second code block makes the issue clearer. Here is the code I've tried. It generates an error. $fpath = (unset(isset($_GET['pageid']))) ? "path/to/file2.txt" : "path/to/file1.txt"; //error Parse error: syntax error, unexpected 'unset' (T_UNSET) /* for comparison purpose, does same thing as above * but works as expected because ifelse construct * allows for stacking functions within a code block. * where ternary seems to have a problem with unset(). */ if (isset($_GET['pageid'])) { $fpath = "path/to/file2.txt"; unset($_GET['pageid']); } else { $fpath = "path/to/file1.txt"; }
-
If you are using PDO or even mysqli, I would suggest turning on error reporting so you see what errors are being thrown. Take a look at this query where its doing something similar with a sub query running before insert statement. $query = "INSERT INTO gali_img ( galc_id, gali_name, gali_type, gali_display, gali_active, gali_date ) VALUES ( ( SELECT galc_id FROM galc_cat ORDER BY galc_id DESC LIMIT 1 ), :galiName, :galiType, :galiDisplay, :galiActive, :galiDate)"; Notice that the sub query is the first VALUES item. Hope that helps. BTW I'm using a PDO driver here, and the :galiName are alias binders for each field.
-
Since the web is "stateless", you really only have two choices. database or flat file. I prefer writing data to XML(flat file), specially when the public has the ability to add comments... Usually for blog post and other long form text. And save discrete data to a database. That being said, your XML could store the count as Barand suggest (retrieve/tally/rewrite) or as new element in the XML. The XML might then look like: //pseudo XML code <tally> <count id="1"> <click>1</click> <button>red</button> <date>2015-02-05</date> </count> <count id="2"> <click>1</click> <button>blue</button> <date>2015-02-10</date> </count> //continues on... <count id="15"> <click>1</click> <button>red</button> <date>2015-02-28</date> </count> </tally> It would be a simple matter of tallying the ticks from each <count><click> element. That's an alternative approach since this is a thought experiment.
-
Why not assign the xml object to an array. I've updated my code using your CURL, so in affect CURL should be returning the same thing for either call. $curl = curl_init(); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); curl_setopt($curl, CURLOPT_URL, 'http://example.com/fake/path/to/my.xml'); curl_setopt($curl, CURLOPT_HTTPGET, true); curl_setopt($curl, CURLOPT_HEADER, false); curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false); $curl_response= curl_exec($curl); //returns string curl_close($curl); var_dump($curl_response); $xml= new SimpleXMLElement($curl_response); //converts string to xml object $array['blog'] = $xml->blog; //assign object to array var_dump($array); echo "The headline is: ".$array['blog']->thead; //access individual elements //Outputs //var_dump array array (size=1) 'blog' => object(SimpleXMLElement)[2] public '@attributes' => array (size=1) 'id' => string '1' (length=1) public 'tdate' => string '10th Jan 2014' (length=13) public 'thead' => string 'From Marketing' (length=14) public 'tpost' => string 'You are currently ...' (length=14) The headline is: From Marketing
-
can you attach the XML file? Something in the XML is formed different then what my answer is expecting.
-
If I'm following your logic. when the web user clicks link 1, you want to load "include/page1.php". PHP is a server side technology. In order to run the that pages code, your either have to reload or user a asynchronous programming model like AJAX. AJAX can execute code in the background. Look into JQuery $.ajax as possible solution.
-
Can you please describe all that this code does?
rwhite35 replied to Chrisj's topic in PHP Coding Help
L1 creates a condition where if the variable $error is not set, run the code block that follows L2 assign the temporary file name (stored in /tmp) to a local variable $uploadFile L3 concatinates (joins together) several variables to make a machine generated name for the thumbnail L4 moves the file from /tmp to /uploads and assigns the file the $thumbnail name, just created. L5 - L8 is only run if an $error is set. L8 copies an image file already on the server and into the upload folder. This is so there is some image if the $_FILES upload process fail Check out conditional statement, which is a construct of PHP (actually every programming language). Hope that helps. -
Sorry for the second post, wanted to edit the original. I'm opening an XML locally, but how I'm accessing the array elements are what you're looking for. $xml_path = simplexml_load_file("path/to/mylocal.xml"); foreach($xml_path as $key=>$value) { $array{$key} = $value; } echo "<pre>"; print_r($array); echo "</pre>"; echo "this is the headline element: ".$array['blog']->thead; /* output Array ( [blog] => SimpleXMLElement Object ( [@attributes] => Array ( [id] => 1 ) [tdate] => 10th Jan 2014 [thead] => From Marketing [tpost] => New marketing rules for our network. ) ) this is the headline element: From Marketing */
-
Give this a try foreach ($xml as $key=>$value) { $array{$key} = $value; } That should give you an associative array where the xml->node is the key and its values the value. Note: your creating XML. You could also check out asXML()
-
Trigger a function again after AJAX Success
rwhite35 replied to emmontenegro's topic in Javascript Help
Also look at the promise interface which is similar to an after expression in looping constructs. $.ajax({ //do some stuff here }) /* promise interface */ .done (function(data) { //do some other stuff }); -
Can you post any source? Also do you have error_reporting(E_ALL) somewhere at the top of the script. Perhaps you're getting notices and warning. But without some more details, its anybodies guess.
-
Hello Mohan, look at AJAX. Here is a code example: /* assign vars, $text_id is a PHP var */ var txtid = <?php echo htmlspecialchars(json_encode($text_id), ENT_NOQUOTES); ?>; var set_url = processPost.php //script to process user submit /* post data to script that processes submit */ $.ajax({ type: "POST", url: set_url, data: {pos_data: txtid}, contentType: 'application/x-www-form-urlencoded; charset=UTF-8' }) /* post execution ui feedback (promise interface) */ .done (function(data) { if(console && console.log) { console.log("AJAX success: " + data); } }) .fail(function(obj,status,error) { alert("AJAX Error:" + obj.error); }) .always(function(){ //you may not want this location.reload(); }); //UI feedback on success alert("Change set! Click Save File to create a PDF"); NOTE: This is inside a JQuery code block with some PHP earlier in the same script. Use this as a learning path for your project.
-
Ugh! Dyslexia is a real b***h when you write code for a living... I was thrown off by thinking it was an issue with godaddy/Windows and failed to look at the simplest things first... Thanks for the assist!
-
So this is unexpected. If $host is assigned as a class property, but the db user name and password remain constants defined in SITE_CONF script, it will all work... And while this isn't how I want to do it, its a work-around that will have to do. class datapull { /* class properties */ var $host; protected function conn() { require '../SITE_CONF.php'; //defined $host $this->host=$host; try { $DB = new PDO($this->host,DB_UNAME,DB_UPWORD); //mixed attributes, properties and const $DB->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); return $DB; } catch( Exception $e ) { $mes = "L226: projectGallery_class.php Caught Exception --- "; $mes .= $e->getMessage(); $mes .= (empty($this->host)) ? " host sting empty!" : null; error_log($mes); //uses system error report } }
-
additional testing: //changed line 10 in first code, get_defined_constants returns 1 - not a list of constants=value $mes .= (defined('DB_HOST')) ? " host string ".DB_HOST : print_r(get_defined_constants(true)); From this, it would seem that the constants are NOT defined for datapull() class. BTW, this is a class being called from an interface->sub class->datapull::conn(). All the machinery is working if I hard code the credential as datapull() class properties. But then portability goes down and I would need to touch several scripts to make the solution work.
-
Thanks Mac Gyver, Okay working off that premise I'm revising my original code to include the (module specific) configuration file: protected function conn() { require '../SITE_CONF.php'; //site wide user config includes db connection credentials try { $DB = new PDO(DB_HOST,DB_UNAME,DB_UPWORD); $DB->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); return $DB; } catch( Exception $e ) { $mes = "L226: projectGallery_class.php Caught Exception --- "; $mes .= $e->getMessage(); $mes .= (defined('DB_HOST')) ? " host string ".DB_HOST : " host empty."; error_log($mes); //uses system error report } } SITE_CONF.php is also included in the viewer page - index.php. So I'm getting an already defined, but then in my defined() conditions, you'll see "host empty". PHP Notice: Constant DB_NAME already defined in D:\path\to\domain\SITE_CONF.php on line 66 PHP Notice: Constant DB_UNAME already defined in D:\path\to\domain\SITE_CONF.php on line 67 PHP Notice: Constant DB_UPWORD already defined in D:\path\to\domain\SITE_CONF.php on line 68 PHP Notice: Constant BD_HOST already defined in D:\path\to\domain\SITE_CONF.php on line 71 PHP Notice: Use of undefined constant DB_HOST - assumed 'DB_HOST' in D:\path\to\domain\portfolio\lib\projectGallery_class.php on line 220 L226: projectGallery_class.php Caught Exception --- invalid data source name host empty. PHP Fatal error: Call to a member function setAttribute() on a non-object in D:\path\to\domain\portfolio\lib\projectGallery_class.php on line 243 Finally, here is how the constants are being defined in SITE_CONF.php: /* * DB Credentials * godaddy requires database name and user name the same string */ define("DB_NAME","nameString"); define("DB_UNAME","nameString"); define("DB_UPWORD","passwordString"); define("BD_HOST","mysql:host=nameString.db.1234567.hostedresource.com;dbname=nameString"); Thanks in advance for any help! I appreciate the assist.
-
Requinix, I was afraid it may be some configuration issue with GoDaddy/Windows environment. As mentioned before, my PHP Windows / GoDaddy hosting knowledge is limited. I'll research that direction though. Thanks for the reply.
-
Hello All, Have a GoDaddy MySQL PHP:PDO hosting questions. Using a database class to make script queries. Unfortunately, I'm required to use GoDaddy Windows hosting. However, I'm a Linux kind of guy. Here's the issue. The viewer script includes database credentials defined as constants, in a file outside the root directory. Typically, these constants are available to all sub processes including class objects. Everything works as expected on development server. The error message I get with version using constants: L226: projectGallery_class datapull Caught Exception --- invalid data source name with host string userDBName.db.6482519.hostedresource.com;dbname=userDBName However when I deploy to GoDaddy's production server, the constants are defined - but empty. Here is the example code. /* works on Linux */ class datapull { var $host; // not assigned here var $userName; // not assigned here var $userPass; // not assigned here protected function conn() { try { $DB = new PDO(DB_HOST,DB_UNAME,DB_PASS); $DB->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); return $DB; } catch( Exception $e ) { $mes = "L226: datapull::conn() Caught Exception --- "; $mes .= $e->getMessage(); error_log($mes); } } public function dbquery($qqueue) { $DB = (!isset($DB)) ? $this->conn() : $DB; /* some query stuff here */ return $result } The modified class for GoDaddy Windows class datapull { var $host = “mysql:host=userDBName.db.1234567.hostedresource.com;dbname=userDBName”; var $userName = “userDBName”; //godaddy user and database naming convention var $userPass = “userPWDString”; //user password protected function conn() { try { $DB = new PDO($this->host,$this->userName,$this->userPass); $DB->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); return $DB; } catch( Exception $e ) { $mes = "L226: datapull::conn() Caught Exception --- "; $mes .= $e->getMessage(); error_log($mes); } } public function dbquery($qqueue) { $DB = (!isset($DB)) ? $this->conn() : $DB; /* some query stuff here */ return $result }
-
Rather then passing sensitive data between scripts using $_GET vars, I would suggest setting a session variable. Note the session_start() in both scripts. /* SCRIPT 1 * initial script instantiates user id var * db query gets the users name and id, then assigns it to a session var */ session_start(); //later in code after db query... $_SESSION['user']['userID'] = $userId; $_SESSION['user']['userName'] = $userName; Now the next script that requires the users ID /* SCRIPT 2 * assign users name and id to local variables * now script 2 has the name and id */ session_start(); $userId = $_SESSION['user']['userID']; $userName = $_SESSION['user']['userName'];
-
Also take a look at url encoding which help setup the $_GET variables you plan to pass along to the next script. Specially for strings.
-
Actually I think OP is confusing the word "user" in this instance. In your original post, the "user" being referred to is between server (i.e. apache) and a client-server (mysql). Where a (privileged) "user"could be root or admin or some other entity you setup when creating the database. Later in your second post, I believe you are thinking about a "user" as in a web user who is filling in some form from a web page. Or some entity/person who has authorization to run queries against the database though your scripts. Like updating a table. The connection is owned by the client-server (like MySQL), but the person doing the action (filling in a form and submitting it) is simply an un-priviledge web user. MySQL is a client-server to your web user who has authorization to use do some task, like updating a table. Thats how I'm reading this thread anyway.
-
Take a look at usort (user defined sort). Because you're working with an object, you'll probably need to create an algorithm for sorting the bid/ask. Plus you're overloading the variables, I'm assuming that is your intention. so $btce_bids is equal to $array['bid'][0][0] and overwrites $array->bids. This looks like something Bitcoin. Is it?
-
Also you may want to consider a library like PHPMailer. https://github.com/Synchro/PHPMailer . Personally I user Pear Mail, but I think PHPMailer is better supported. Good luck.