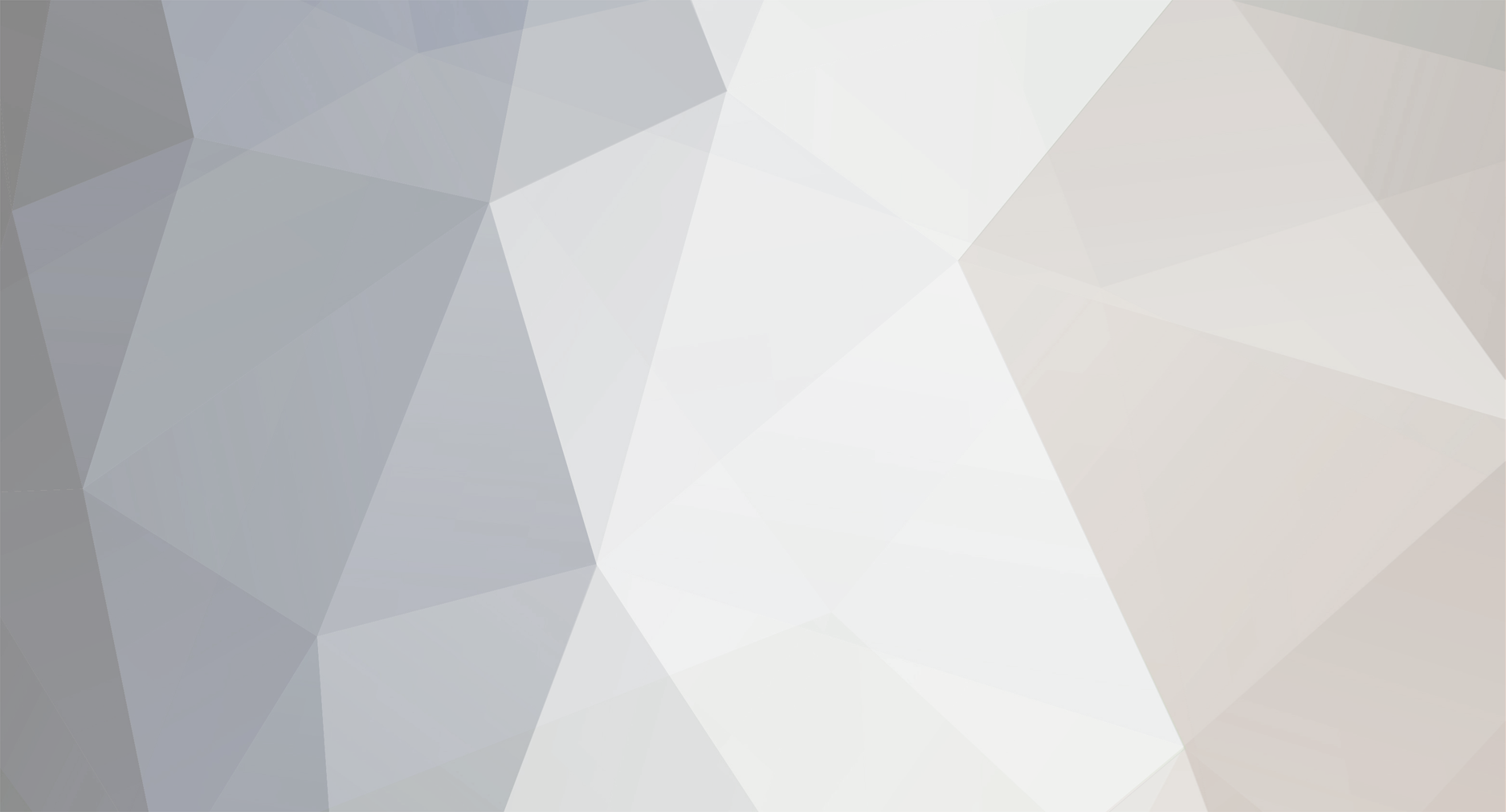
JD*
Members-
Posts
230 -
Joined
-
Last visited
Everything posted by JD*
-
You can also use a switch conditional which makes things a little cleaner: <?php //added these to test them, uncomment one //$result = "Swinging Ship"; //$result = "Roller Coaster"; //$result = "Ice Blast"; switch($result) { case "Swinging Ship": $go_to_page = "Swing.php"; break; case "Roller Coaster": $go_to_page = "Roller.php"; break; case "Ice Blast": $go_to_page = "Ice.php"; break; default: $go_to_page = "./"; //a default location to go? break; } echo "<a href='$go_to_page'>$result</a>" ?>
-
The only other way I can think of would be to use sessions, where you would have code at the top of every page to initiate a session and check to see if the session is set for price ($_SESSION['ad_price']) and if not, query a database for the current value, then set the session. It's pretty much the same thing as doing an include. Of course, you could do the same thing by reading a text file on your server instead, but at the end of the day, it's all the same; the value has to come from somewhere, and if you want it to be centrally located, you're going to have to tell all of your pages where to find that information
-
noob needs help.. no errors, but code not functioning
JD* replied to phpnoob123's topic in PHP Coding Help
Ok, so replace this: if(isset($_POST['submit'])) { copy_zip_file(); extract_zip_file(); } function copy_zip_file(){ $old = 'test.zip'; $new = '/folders/$folderName/test.zip'; copy($old, $new) or die("Unable to copy $old to $new."); } with this if(isset($_POST['submit'])) { if(exec("cp ".$old." ".$new)) { extract_zip_file(); } else { die("Unable to copy $old to $new"); } } See if that works. -
noob needs help.. no errors, but code not functioning
JD* replied to phpnoob123's topic in PHP Coding Help
Try doing the copy with exec instead (assuming you're on a unix hosting system, cp) and see if that works. If so, your host may have disabled the copy command http://php.net/manual/en/function.exec.php -
Change your \n to <br /> and you should be in business
-
In that case, it sounds like this should be directed to the javascript forum, as it can all be done via those scripts.
-
I'm assuming that you're going to be sending this to a script on the server itself, yes? If so, you can probably write some javascript that would add the return value to a hidden form value and then submit the form, which can then process.
-
You're most of the way there, check this against your and see if you can get it to work: $result = mysql_query("SELECT password FROM Accounts WHERE Username = '".$_POST['Username']."'") or die(mysql_error()); if(mysql_num_rows($result) != 1) { echo "No such user exists"; unset($_POST); exit(); } if(md5($_POST['Password']) == mysql_result($result, 0, "Password")) { $_SESSION['loggedin'] = yes; $_SESSION['User'] = $usr; $_SESSION['message']= "You have successfully logged in " . $usr; } else { $_SESSION['message'] = "Username and password do not match."; UNSET($_POST); } Try not to use $_REQUEST, it's better to use $_POST and $_GET
-
Actually, in your games table you'll probably have a few columns, but at the least you'll have ID, name, category_id and link. Then, in your category table, you'll have ID and category (or whatever you want to call it). Then, your category_id in the games tables will reference the ID in the category table. In your PHP, when you do your select, it would look something like this: $games = mysql_query("SELECT g.name, c.category, g.link FROM games g, categories c WHERE g.category_id = c.ID") or die(mysql_error()); The above assumes that your tables are named games and categories. Once you do that, you can loop through the results and print out a link tag around the name of the game.
-
Sure. You can do it like any other operation, just add in your code right before you remove their session/cookie/whatever you use to track the login.
-
Use nl2br on the insert/update to change your \r\n to <br>s. You can then do a str_replace on the way out if you want to replace your <br>s with \r\n
-
So.... I take it that means it's not possible?
-
I wasn't really sure how to phrase the subject and I'm not sure if this can be done, but lets see: I have yearly data that is stored like this: Name | Agreed --------|---------- Joe | 2009-2010,2010-2011 Mary | 2009-2010 What I'm trying to do is make a query for a drop down list that will say "Please choose a year range" and I want to avoid hand coding it, but rather list only those years that have already been entered by people. Since each date isn't stored in a separate column I don't think I can do group by. Anyone have some thoughts on if it can be accomplished?
-
Or, if it's not something that needs to be secure, pass it to the URL
-
Ok, here's some updated code for you <? $album=basename($_GET['album']); $dirname = "uploader/photo/$album/"; $images = scandir($dirname); $ignore = Array(".", ".."); $start = (!empty($_GET['start']) ? $_GET['start'] : 0); $prev = ($start >= 20 ? $start - 20 : 0); $end = $start + 20; $i = 0; foreach($images as $curimg){ if(in_array($curimg, $ignore)) continue; if(($i >= $start) && ($i < $end)) { echo '<a href="uploader/photo/'.$album.'/'.$curimg.'" rel="lightbox">'.$curimg.'</a> '; } $i++; } echo '<br /><br /><a href="?album='.$album.'&start='.$prev.'">Previous Page</a> | <a href="?album='.$album.'&start='.$end.'">Next Page</a>'; ?> A few notes: When you use the inline conditional switch ($start variable, I'm not sure if that's what it's called but it's what I call it), you set your variable first, then feed it the conditional. Got rid of the $next variable, unnecessary Condensed your if check for the $ignore part Put all HTML in single quotes - yes, you have more quotes in echoing your variables, but it's easier to port to a plain HTML page for page construction without having to find/replace all " to \" You still need to put in some checks to see if there are files for a valid next/previous link to show up (right now they show up regardless and the pages will be blank/break)
-
What you're going to want to do is before the code that echos, get the number of files in your directory. Take that number, divide by 20 and you'll get the number of pages you need to have access to (the old <- 1 2 3 4 -> type of nav). Then, you'll have to make it so that each link will have an action/id assigned to it, so that when the page loads, it checks which portion is should load. Finally, when you start to show the pictures, you can use the number you're on to tell it where to start the array pointer, and set up a counter so that it will only loop through 20 images.
-
You need to do a return in your function: function array2fieldtest ($data) { //var_dump($data) ; //$element_count = count ($data) ; foreach ($data as $ky) { $key = key ($data) ; $keys[] = $key ; next ($data) ; } $fields = 'id, ' . implode (', ', $keys) ; return nl2br ("$fields\n") ; }
-
It sounds like you just need to have a column in a user database called "scrip" that, when they do actions to earn, will add a certain amount (via an UPDATE statement) and when they buy something will subtract. Unless I'm missing something?
-
Topflight, it would be best if you could convert your dates into the standard yyyy-mm-dd. If not, you could always get the db result, break it into an array, then use that to compare: $result = mysql_result("SELECT * FROM table ORDER BY date") or die(mysql_error()); for($i=0; $i < mysql_num_rows($result); $i++) { $date = explode('.', mysql_result($result, $i, "date")); $date = $date[2].'-'.$date[1].'-'.$date[0]; if(date('Y-m-d') - $date > 30) { echo 'Last flight was greater than 30 days'; } elseif(date('Y-m-d')-$date > 20) { echo 'Last flight was greater than 20 days, but less than 30'; } else { echo 'Last flight was less than 20 days ago'; } echo '<br /><br />'; } I didn't test the above code, so your mileage may vary, but that would be the general idea.
-
You can do it either in the mysql or in PHP. If you want to do it in mysql, you can make your selects with the NOW() variable. Subtract date from NOW() and if it's greater than 30 or greater than 20 and less than 30. If you do it in PHP, you can use the date() function to do something similar, just have a for loop for each row and do the check.
-
I wouldn't say I'm experienced to the point of rewriting Wordpress, but the idea was to come up with something modular that they could work on and improve over the 16 weeks. As you said, teaching them about forms (add/modify/delete posts, users, login, etc), creating functions and classes, etc. I've tried, in other web classes, to encourage grouping, but I'll have to see how I can handle that in this one, as it usually backfires (one/two people work and the others sit around). We're actually going to be getting our own server just for this class, so they will def. have access to a live system (would be pointless otherwise ).
-
Not so much a coding help question, but rather a request for some advise. I've been tapped to develop a course on PHP/MySQL for a community college that I teach at and I wanted to see what the freaks thought about it. It will be a 16 week long class, 4 hours per week. I'm thinking the whole class will cover the development of a blogging type system, as it will cover basic PHP concepts (using PHP to get and convert dates, do simple themes based on current day/time, includes, etc) and MySQL. If you could develop a course like this or take a course, what would you like to see included?
-
$data = mysql_query("SELECT * FROM users WHERE userid = '".$_SESSION['SESS_USERID']."'")) or die(mysql_error()); extra ) at the end of your query (before the "or die") part. Did you try to echo out the whole query (ie, between the $data =... and the $user =... you put a die($data) and see if it successfully executes in MySQL?
-
Reading Old Access / CSV data with line breaks?
JD* replied to scottwhittaker's topic in PHP Coding Help
You could write a PHP script to read in the csv file (fopen), then make a SQL insert for each row. Echo everything out to the page first, and check to see what the line breaks are like and see if you can use nl2br to convert them, then put it into your database (one row at a time) and see what happens. If you echo out your sql insert statement first, you can copy/paste it into a MySQL frontend tool and see if it executes -
Is there a CSS rule somewhere like this: -moz-border-radius This will cause your corners to be rounded in firefox. Alternatively, if you know the name of the class or ID of those boxes, you can make your own rule at the very bottom of your CSS file that says: -moz-border-radius: none;