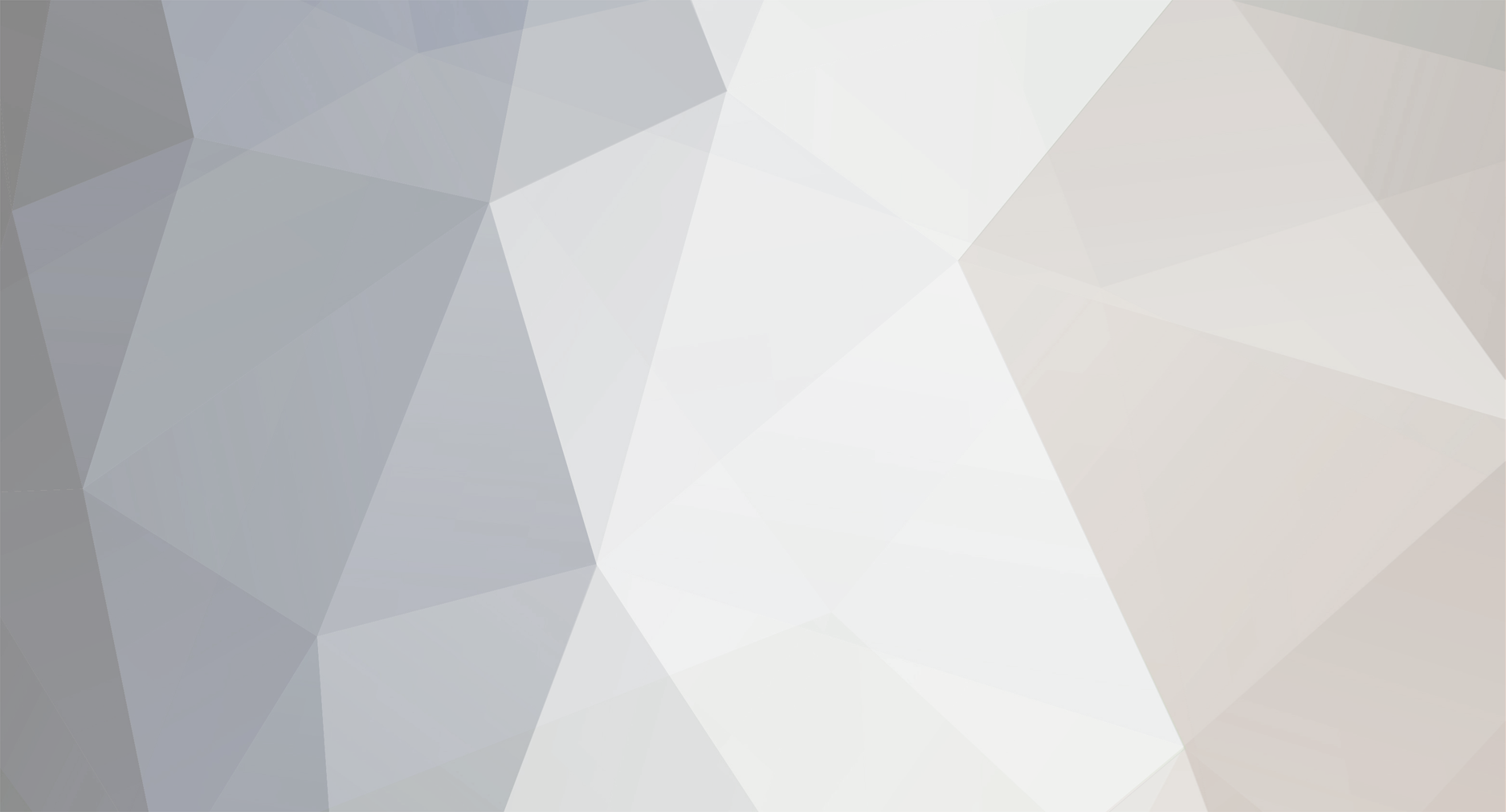
JD*
Members-
Posts
230 -
Joined
-
Last visited
Everything posted by JD*
-
Hey everyone, I'm trying to write a piece of code that will allow me to recursively look through a directory and list contents. I've tried a bunch of pre-made scripts but nothing is working correctly so far and I'm spent trying to figure it out. What we have is a list of students that a teacher can click on an individual student, and then see different documents that have been uploaded from previous years. On the file system, they're stored in a path like: /srv/www/website.com/files/student_number/2008-2009/language_arts/entry1.pdf /srv/www/website.com/files/student_number/2008-2009/language_arts/entry2.pdf What I'm looking to have is each directory from the year on down as an <h3> which I can then use some jquery to either make an accordion or some other effect, so the teachers can drill down. I was able to get the year to work, but everything below, not so much, as the looping wasn't being very flexible. Also, when putting all of this into an array, it would list entry2 first, then entry1. I want to sort the array, but since it's all contained in one giant array, sorting just screws it all up. Thanks for any help!
-
Did you try to echo out the sql statement? Do that and see what is being filled in for your session value, and then copy/paste that into your MySQL interface and see if you get results. Right now, from your statement, I see that you may want to try and escape your "" and that you aren't ending the last quote correctly: mysql_query("SELECT * FROM users where userid="$_SESSION['SESS_USERID']) to mysql_query("SELECT * FROM users WHERE userid = '".$_SESSION['SESS_USERID']."'")
-
Check out http://www.thewebhelp.com/php/php_contact_form_with_image_validation/
-
I gave up and moved everything into the file system. I just got tired of bashing my head against the wall and people were angrily calling me. Thanks for all of the help/suggestions. Now I just have to deal with my boss and not storing the files in the db
-
simshaun - thanks for the reply. I know storing files directly in the db is not the recommended way, but, unfortunately, it's how I've been instructed to do it. As for the data, yes, it all checks out. I am currently using a LARGEBLOB type for storage, because I have some very non-tech people who will be uploading files and in most cases they do not know how to optimize (or they don't pay attention to their training, manuals, etc). Sorry for the confusion about the UNCOMPRESS, but I am using it after putting the files into the DB. There are 2 strange parts left to this: 1) PDF files come out with no problem. It's only other types (xls, doc, ppt) that are coming across corrupt 2) It works on my testing server without a hitch I just removed the database on my live server and recreated it from my test server and copied the code from my test server that does the uploading and downloading of the files, and still, only PDF files are being recognized. For a word document, the file does come down with the correct headers, it's just garbled. When I upload them, I do a base64_encode first, then the MYSQL Compress, and on the way out I do an UNCOMPRESS, then a base64_decode...is my order for those functions wrong? Or should I be using something other than the base64 (I had used it in the past to ensure binary safe data)? Some of the searching I did online showed people uploading to the db with addslashes, but then on downloading not using stripslashes. I tried both with and without the stripslashes and got the same results each time.
-
Hey all, This problem just came up in my website and I'm having a hard time figuring out what's wrong. I have a intranet portal running (custom built) and I'm storing all different types of documents in a mysql database. Previously, I was able to read the contents of the file into the DB and then pull it out and the file would open just fine. Now, for some reason, everything except PDF files come across as gibberish. I've gone ahead and tried to upload files that I know are working, I've switched from base64_encode to addslashes, tried to do a mysql COMPRESS and UNCOMPRESS when uploading a new file....nothing. I'm just not sure why all of a sudden this stopped functioning properly (haven't done any upgrades to the server, db or code) Anyone have any ideas I can try? For reference, the code for inserting and downloading follows: insert: case "Add File": $fp = fopen($_FILES['userfile']['tmp_name'], 'r'); $content = fread($fp, filesize($_FILES['userfile']['tmp_name'])); $content = base64_encode($content); mysql_query("INSERT INTO intranet.documents(id, type, contents, content, name, category, updated) VALUES ('', '".$_FILES['userfile']['type']."', '".$content."', COMPRESS('".$content."'), '".addslashes(str_replace(" ", "_", strtolower($_FILES['userfile']['name'])))."','".$_POST['category']."', '".date("Y-m-d H:i:s")."')") or die(mysql_error()); fclose($fp); redirect("?action=".$_GET['action']); break; download: $_SESSION['content'] = NULL; $result = mysql_query("SELECT name, type, contents FROM intranet.documents WHERE ID = '".$_GET['id']."'") or die(mysql_error()); $_SESSION['content'] = base64_decode(mysql_result($result, 0, "contents")); header("Content-Disposition: attachment; filename=".mysql_result($result, 0, "name").""); header("Content-type: ".mysql_result($result, 0, "type"));
-
You could have some javascript on each td that would reference the mouse over, pass the name of the td the mouse is currently over and update the background color of the tr.
-
Sorry, I didn't see in your original code that you were echoing your link with single quotes. Change this: echo'<a href="http://parobek.euweb.cz/transfer.php?t='$balance'">Transfers</a>'; to this: echo'<a href="http://parobek.euweb.cz/transfer.php?t='.$balance.'">Transfers</a>';
-
You have an extra ' at the end of your balance variable
-
This line: echo'<a href="http://parobek.euweb.cz/transfer.php?t='$balance'">Transfers</a>'; should be echo'<a href="http://parobek.euweb.cz/transfer.php?t=$balance">Transfers</a>'; you don't need quotes around url variables
-
The problem is that in HTML you would set it to CHECKED, not checked="checked" Here's some cleaned up code: <?php echo '<input type="radio" name="show_email" value="1"'.($session->userinfo["show_email"] == true ? ' checked ' : '').' /> Yes <input type="radio" name="show_email" value="1"'.($session->userinfo["show_email"] == false? ' checked ' : '').' /> No <br />'; ?>
-
How is it password protected? Using regular auth or through a custom PHP page? Either way, if it's something to be scheduled, you'll need to involve either Task Scheduler (windows) or cron (*nix/apple) to run a script/page that will do the password change
-
If you pass it through the url, you need to do this: $balance = $_GET['t']'; echo $balance
-
instead of breaking up the date, just try to do this: $date_formatted = date('F j, Y', strtotime($date)); See if that works for you (has for me in the past)
-
for($i=0;$i < 4; $i++) { $activ_code.= rand(1000,9999).($i < 4 ? "-" : '"); } That will loop through four times, generate a random number (*make sure you look up how to really make this more random*) and then add a "-" between the number so long as we're not over 4 loops
-
$result = mysql_query("SELECT account_number FROM database") or die(mysql_error()); $balance = mysql_result($result, 0, "account_numer"); echo $balance
-
Also, this may sound stupid, but did you try using single quotes for your session defines instead of double?
-
[SOLVED] Present document outside of web path for download
JD* replied to JD*'s topic in PHP Coding Help
Thought so, but it's been one of those days where I'm second guessing everything. Thanks! -
Hello all, Trying to see if this is easier than I think it is. I have a small web portal for teachers to upload documents for their current students to be archived over the course of their years. In order to semi-protect the documents, I'm storing them outside of the normal web path. What happens is that when a teacher logs in, it gets their current students, which she can then click on and see what documents have been uploaded for that student. What I'm a little confused about is how to let them click on the link and then get that document. I plan on having a function that will check their access one more time, to make sure they're allowed to see that student's document, and then finding it in the proper folder and acting like a normal PDF download (all docs are PDFs), but I'm not sure if I should use a complicated fopen, fread etc or if it's just as simple as changing the header to point to the file where it resides on the server. Any ideas?
-
You can also do the following: $result = mysql_query("SELECT * FROM category WHERE cat_name = '$curr_cat'") or die(mysql_error()); for($i=0;$i < mysql_num_rows($result);$i++) { echo mysql_result($result, $i, "area").($i + 1 < mysql_num_rows($result) ? ", " : ""); } If I did it right, it should give you each area followed by a comma until it reaches the last one, where it will not.
-
You need to start your session at the very top of the page, before you do anything else (ie create your form).
-
You can use the mysql "LIKE" search... $result = mysql_query("SELECT * FROM table WHERE name LIKE '%name_variable_here%'") What this does is finds a similarity in strings. You can do it based on first name, last name, full name...whatever you want/need. Then, just do a: if(mysql_num_rows($result) > 1) { //create splash page here with all results, linked by ID } else { //code here will get the result from the database, or redirect to the page that does it. }
-
On your validation page, have this: if($_POST['avcoach'] == "yes" && empty($_POST['coachrep'])) { die("Your error message here"); }
-
[SOLVED] Explode string and validate - HELP ME PLEASE???
JD* replied to StefanRSA's topic in PHP Coding Help
If you're grabbing your rows from a database, you don't need to put them into an array...you can just reference them from your query. But lets clean up your code so it's a bit easier to read, and then I'll need a little explanation on some parts: <? while($row = mysql_fetch_array( $result )) { // Print out the contents of each row into a table ////////////////////////////////////// $reg_name = $row['est_name']; $inputid=$row['update_id']; $fullrow = $row['accom_type']; $fieldid = '$inputid-units'; $maxunits = $row['max']; echo '<tr> <td>'.$row['accom_type'].' - '.$row['max'].' units - '.$row['pax'].' beds per unit</td> <td><input id="'.$inputid-units.'" class="TextField" name="'.fields[$inputid][units].'" type="text" size="3" value="0" maxlength="3"></td> <td><input id="'.$inputid-adults.'" class="TextField" name="'.fields[$inputid][adults].'" type="text" size="3" value="0" maxlength="3"></td> <td><input id="'.$inputid-children.'" class="TextField" name="'.fields[$inputid][children].'" type="text" size="3" value="0" maxlength="3"></td> <td><input id="'.$inputid-infants.'" class="TextField" name="'.fields[$inputid][infants].'" type="text" size="3" value="0" maxlength="3"></td> </tr> <input type="hidden" id="'.$inputid-accom_type.'" name="'.fields[$inputid][accom_type].'" value="'.$fullrow.'"> <input type="hidden" id="'.$inputid-pp_pr.'" name="'.fields[$inputid][pp_pr].'" value="'.$row['pp_pr'].'">'; ///////////////////////////////////////// } ?> I'm not sure about these parts: $reg_name = $row['est_name']; $inputid=$row['update_id']; $fullrow = $row['accom_type']; $fieldid = '$inputid-units'; $maxunits = $row['max']; They don't seem to be referenced in any of your code from above. Also, when getting the ID and name for your fields, why are you doing fields[$inputid][units], etc, instead of just doing $row['units']? I'm not sure how others feel, but I stopped declaring variables for SQL returns a long time ago and just reference them directly in the code, so $ID = mysql_result($result, $i, "ID"); echo $ID for me just became echo mysql_result($result, $i, "ID"); -
Whoops, I meant table...early mornings and no caffine!