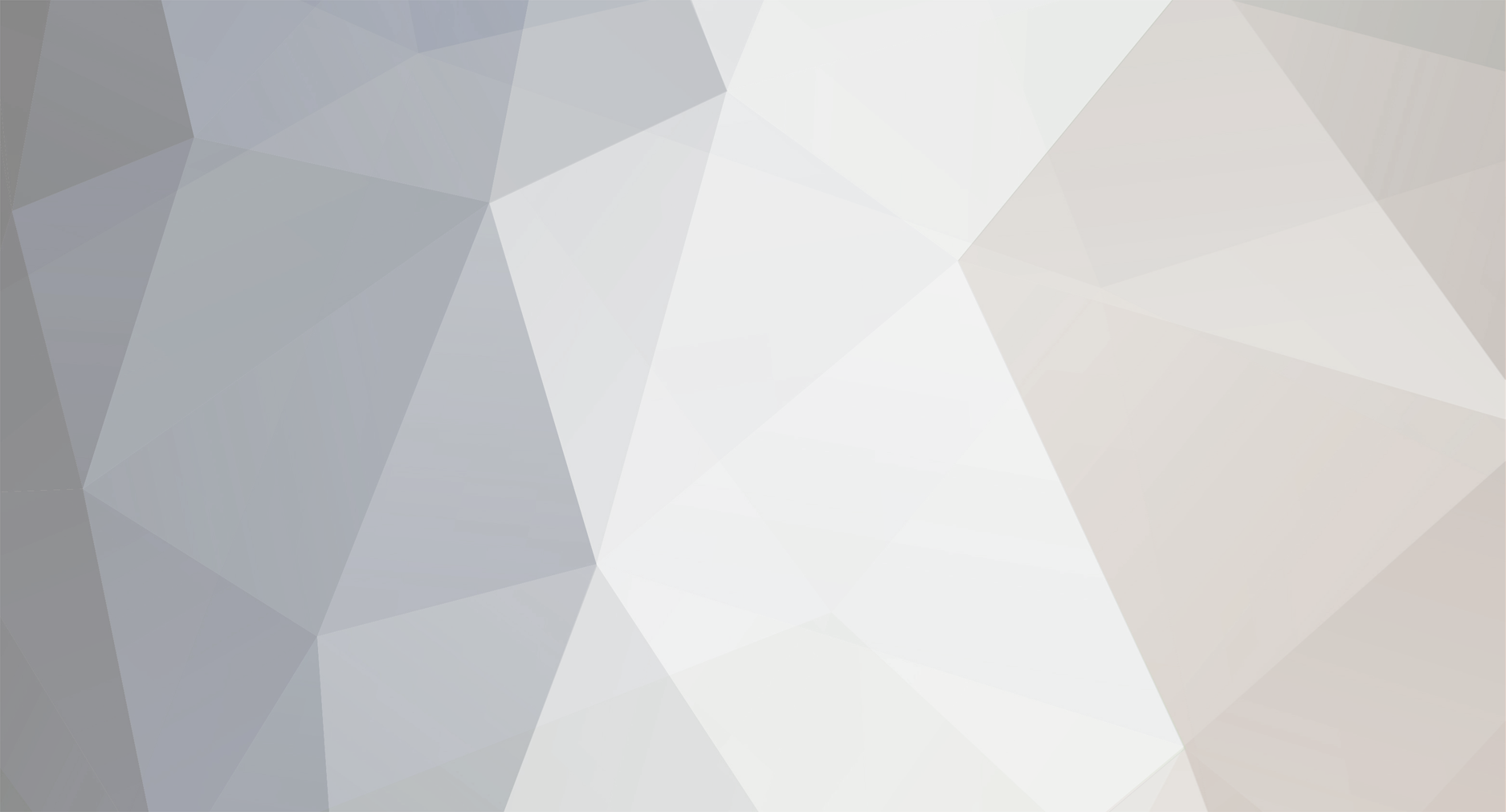
JD*
Members-
Posts
230 -
Joined
-
Last visited
Everything posted by JD*
-
You have two ways of doing it. Currently, you're doing one of them, where you re-query the database with the submitted item, which you can then use to grab all of the information and insert that into another table. Another way of doing it is to make the value of each of your options the $row[item].'-'.$row[fare], and on your processing page, you can explode the posted value into an array based on the '-' character, since it looks like each part of your journeys uses that to separate what you need to collect, except for the price. So what you'd have is: $trip = explode('-', $_POST['item']); print_r($trip); The print_r will show the following: Array ( [0] => sukkur [1] => karachi [2] => nonac [3] => 550 ) And that should let you construct your query. Additionally, if you want your database to be truly relational, you could just store the ID of the journey and then reference your journey table with that ID to get all of the values.
-
[SOLVED] Explode string and validate - HELP ME PLEASE???
JD* replied to StefanRSA's topic in PHP Coding Help
You could set your form fields (dropdowns? textfields?) to be something like 1_0, 1_1, 1_2, which would be the room (first part) and the part of the array (second part). Then, in your validation code, you could say "for size of the array for the first string, get the submitted value key 0, key 1, key 2, etc and compare them with my array key 0, key 1, key 2, etc" -
You can build a custom function, i.e. function insert_to_db($db, $fields, $values) { //Do checking here to make sure $db, $fields and $values are valid mysql_query("INSERT INTO ".$db."(".$fields.") VALUES(".$values.")") or die(mysql_error()); echo "Values Inserted"; } Just make sure you clean all of your data, and that your values are surrounded by single quotes, etc.
-
Did you look into strotime()?
-
Content Management System, which is what it looks like you're doing. The way I usually do this is I have a database table that has the username, password (encrypted with md5 usually, although that's not 100% secure) and then a column for each function a person can perform. I can then assign a numerical value to each user for each function (0=no access, 1=normal, 2=admin, or whatever kind you'd like to use). So my main index page looks like this: include("functions.php"); if(isset($_POST['Submit'])) { //Handle forms here } elseif(isset($_GET['action'])) { verify_access($_GET['action']) $_GET['action'](); } else { display_login(); } And then in my function file, I have some of the following: function verify_access($access_type, $return_type = NULL) { $result = mysql_query("SELECT * FROM permissions WHERE ID = '".$_SESSION['id']."'") or die (mysql_error()); if($return_type == NULL) { if(mysql_result($result, 0, $access_type) == 0) { $_SESSION['error'] = "no_access"; redirect("?action=home"); } } else { return mysql_result($result, 0, $access_type); } } You can then go ahead and program each part of your admin area and give each user specific access, even using the verify_access function with a return value to determine what a user can see inside of a functions (maybe level 1 access for part, level 2 for more, etc).
-
Can you echo out normal PHP without any problem?
-
What are the admins going to be doing? Are they physically changing the code of the pages, or are they using as a CMS? If they're doing as a CMS, you can set up your security so that every "page" (I use functions) checks to see if they are logged in and if they have access to that area before allowing them in. I use this method and it works very well, it's all based on sessions.
-
I'm not sure exactly what you need....do you need to figure out the path, or do you already know it?
-
[SOLVED] How to display alternative image if database record is empty?
JD* replied to hemangi's topic in PHP Coding Help
Or a slimmer way: <?php echo (!$row_rsList['WL'] ? "images/emptyGraphic.gif" : $row_rsLiost['WL']); ?> This does a comparison of two cases. -
Look into strpos
-
Also, you probably don't want to have your whole message in your URL, especially with spaces. Do something like "Location: acute.php?msg=3" and then in your page have it check for what the msg is via a switch statement: switch($_GET['msg']) { case 3: echo "Please consult your doctor before carring out these exercises"; break; } //...etc
-
echo '<div id="main_slider_div">'; $result = mysql_query("SELECT * FROM pictures ORDER BY something") or die(mysql_error()); $counter = 0; for($i=0;$i < 3;$i++) { echo '<div id="second_loop_div">'; $i2 = $counter + 6; for($i2 = $i2; $i2 < $i2 + $counter; $i2++) { echo '<div id="individual_pic">'.mysql_result($result, $i2, "picture").'</div>'; if($i2+1 > $i2 + $counter) { $counter = $i2; } } } Not sure if that will work at all, but it should. I know the first two loops will, but for counting your photos you'll have to test it out. I'm sure someone else may have a better solution.
-
Because your check is inside of the diamond check...change it to this: <?php $sql="SELECT * FROM player WHERE id='$id'"; $result=mysql_query($sql); while($r=mysql_fetch_array($result)) { $Status=$r["Status"]; $geneticist=$r["geneticist"]; if ($Status=="mod" || $Status=="Diamond") { if($geneticist=='no') { echo "<td class=tabletd align='center'> <span class='page'> <a href='academy_genetics.php'>Take this exam! </a></span></td>"; } else if($geneticist=='yes') { echo "<td class=tabletd align='center'><span class='page'> <font size='2px'>You've already passed this exam!</font></span></td>"; } } else { echo "<td class=tabletd align='center'><span class='page'> You have to be a Diamond in order to take this exam.</span></td>"; } } ?>
-
What you need to look into is a payment gateway. It's not a limitation of PHP, it's a service that can actually interface and do the charging, much like having a swiping machine at a store allows the card to be charged. It's in your best interested to just study up on them and pick the one that best suits you. Once you find one, they should have instructions on how to forward the appropriate information to them for charging.
-
[SOLVED] How to link pages depending on which page you arrived from?
JD* replied to Nuccah's topic in PHP Coding Help
I'm sure there is a better way with a regular expression or some such, but you could split it again with an explode based on the ".", then get the [0] place of it -
[SOLVED] How to link pages depending on which page you arrived from?
JD* replied to Nuccah's topic in PHP Coding Help
As per what I posted, on the video page you can query the referring page (where they came from) to get the last URL. You can then do a split based on the _, since that seems to be common, by doing an explode, which will turn your url into an array. Then just get the last piece of the array, which will have your eng/sp/etc, which you can then use to say "forward me to next_whatever.php" $url = $_SERVER['HTTP_REFERER']; $url = explode("_", $url); That goes at the top of your page. Then, you can write to your link echo '<a href="next_'.$url[1].'.php">Click here to skip/continue</a>'; And that will take them to the next page with the proper follow. Just make sure "next" is your page name, and you may have to check on which part of the array you need to get if there is more than one underscore (_) in your url. -
What happens when you run it?
-
[SOLVED] How to link pages depending on which page you arrived from?
JD* replied to Nuccah's topic in PHP Coding Help
You should be able to use $_SERVER['HTTP_REFERER'] to get the page that they came from, parse out the last part of the url with a strstr and then store that in a variable for when you go to do the redirection. Of course, that hinges on the fact that they will hit your index page first, then the video and not go straight to it. -
How to redirect if the $_GET['ENTERACTIONHERE']; dosent exist
JD* replied to codrgi's topic in PHP Coding Help
I like to use case statements to call my functions. switch($_GET['action']) { case "email": //do email action here break; default: // redirect to home break; } -
So I think it would be something like this: $tourney = explode('/', url here, however you're getting it) echo $tourney[array_size($tourney)-1]; That should give you the last value which is your number. Unfortunately, I can't test right now, but hopefully it gets you moving in the right direction.
-
If you want to make it a part of the picture, look into the GD Library. It has some good functionality to open a picture, create new parts of the image based on text and then write it back into one flat picture.
-
Try $array = array('1', '2-1', '2-2-1', '2-2', '2', '3-1', '3'); sort($array); print_r($array); I think that will work for you.
-
[SOLVED] Can you put html links within a php function?
JD* replied to ironman32's topic in PHP Coding Help
Just to round it out: function lfc() { echo '<a href="http://www.lfc.tv">LFC</a>'; } I like the single quotes because if I have to put something into HTML to check it, I don't have to remove the \" from everything. -
Do you have a defined area you want to get (ie always the last part, always after the 3rd slash, etc)? If so, just break out into an array based on the '/' and use your different array functions to get that part. If not, post back on here and let us know. As for the second part, you can do string matching with strpos
-
[SOLVED] Getting a checkbox array to process to page.
JD* replied to beevbo's topic in PHP Coding Help
It looks like there is a space in your call to the $_POST variable. Make sure $_POST[ 'service_required'] is $_POST['service_required']