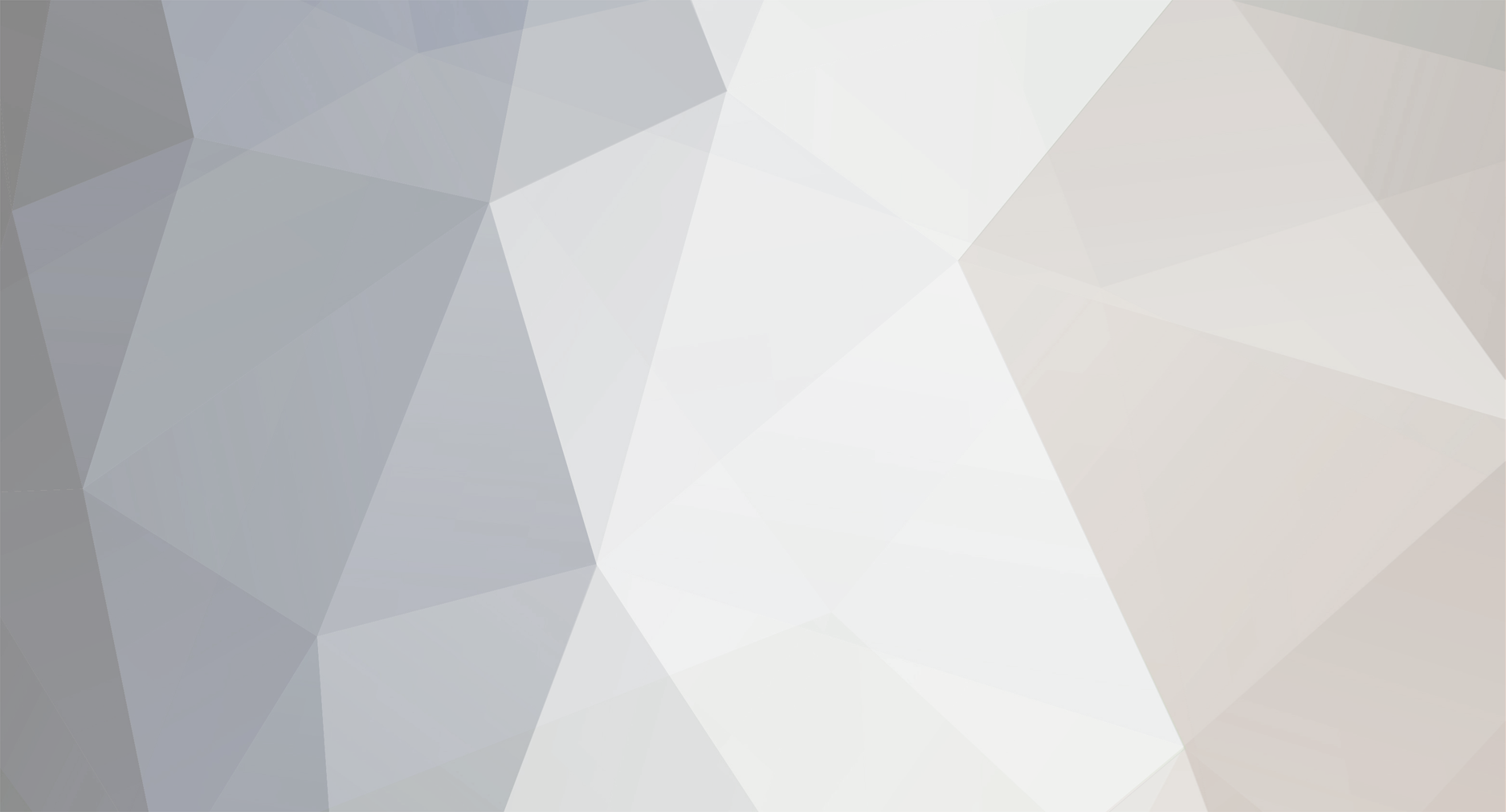
JD*
Members-
Posts
230 -
Joined
-
Last visited
Everything posted by JD*
-
[SOLVED] UpDate MySql from a form (ANOTHER PROBLEM!)
JD* replied to TheStalker's topic in PHP Coding Help
Yes, and you can condense your code to read like the following: $con = mysql_connect("localhost","root",""); if (!$con) { die('Could not connect: ' . mysql_error());} mysql_select_db("randv", $con); mysql_query("UPDATE customers SET Surname = '$_POST[surname]', Forename = '$_POST[forename]', AddressLine1 = '$_POST[addressLine1]', AddressLine2 = '$_POST[addressLine2]', Postcode = '$_POST[postcode]' WHERE customerID ='$_POST[customerID]'", $con) or die('Error: '.mysql_error()); $custId = mysql_insert_id(); mysql_query("UPDATE accounts SET Surname = '$_POST[surname]' WHERE customerID ='$_POST[customerID]'", $con) or die('Error: Could not insert account when inserting customer: ' . mysql_error()); ?> -
Don't forget to mark your post as solved if it's fixed.
-
If the text is being inserted into the database with line breaks, then when it comes back out just do: nl2br(return_query_string_here) That will take the new line character and turn it into a <br /> on the resulting page.
-
$_POST only works when you post the infomation, i.e. you submit the form. So unless I misread, you're clicking on a hyperlink to get a description, but not submitting the form and that's where the problem is.
-
You need a script to process the form first. Try this: <? if(isset($_POST['button']) && $_POST['button'] == "Go") { header("Location:/test/search.php?search_by=".$_POST['search']; } else { ?> <form action="" method="post"> <select name="search" id="search"> <option value="">No.</option> <option value="">---</option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> </select> <input type="submit" name="button" id="tools_button" value="Go" /> </form> <? } ?>
-
Why not just use strstr? $video = strstr($post_url, "?v=");
-
[SOLVED] UpDate MySql from a form (ANOTHER PROBLEM!)
JD* replied to TheStalker's topic in PHP Coding Help
To be safe, I usually structure mine as WHERE Surname = '".$_POST['customerID']."' that way if I have to do any extra checking (yeah, sometimes I do it inline because I'm crazy), my statement is all ready to go with the proper quotes. -
You can also just get the name of the current page with this: <?php $this_page = basename($_SERVER['REQUEST_URI']); if (strpos($this_page, "?") !== false) $this_page = reset(explode("?", $this_page)); ?> Just clean up the string a bit more and you'll always know where you are.
-
try to echo out your $sql string and see if the statement looks correct. If your $user variable is empty, you'll have to put in some error checking to make sure it can't be that way, and then find out why it is empty.
-
[SOLVED] UpDate MySql from a form (ANOTHER PROBLEM!)
JD* replied to TheStalker's topic in PHP Coding Help
Also, sometimes it's easier to get rid of the if statement and just do mysql_query($sql, $con) or die("Error: ".mysql_error()); -
Using foreach to print out arrays to form a search query
JD* replied to harold's topic in PHP Coding Help
Or you can use concatenation. The reason your variables are being overwritten is because every loop you're tell it to set the variable to the current value, not add on. change your strings to be: $search_geography.= "AND geography = '$geography' "; That .= means "take the current $search_geography variable and add this (everything after the .=) to the end of it" -
Try instead: if ($row['reg'] == '1'){
-
Oh, duh, I see what's going on....you're putting the values in to the URL, so you should be using $_GET instead of $_REQUEST. Try that and see if it works.
-
You should look up on exploding strings into arrays: http://us3.php.net/manual/en/function.explode.php You can use that to do: $array = explode("/", $_SERVER['REQUEST_URI']); then access the $array[1] or $array[2] or where ever your "section" part happens.
-
Try to echo out your mysql statement by doing the following: //mysql_query("INSERT INTO object (module_name, object_name, xpos, ypos) VALUES ('$g_model', '$g_objName', '10', '10')"); echo("INSERT INTO object (module_name, object_name, xpos, ypos) VALUES ('$g_model', '$g_objName', '10', '10')"); and just see what shows up. If you don't see anything in the $g_model and $g_objName parts, then the value isn't found from the javascript. In that case, try to echo your $_REQUEST['g_mode'] and see what happens. If that still doesn't work, then you have to look into the javascript. If it does work, let me know and we'll look further into it
-
Still here, just went away for a bit... So for the user request, I would have the page have a print.css file that can show/hide elements you do/don't want to show up on the print. This way, when a person chooses "File->Print" it just does what it needs to do. As for the downloading of the page, if you want it to go out to a PDF file, you can look into a class like http://sourceforge.net/projects/pdf-php, which you can use to output content to a PDF file.
-
Try this instead: mysql_query("INSERT INTO object (module_name, object_name, xpos, ypos) VALUES ('".$g_model."', '".$g_objName."', '10', '10')");
-
Does it need to do all three automatically, or upon user request? Also, how does it need to be saved (csv, text, etc)?
-
Ah, I see what you're saying. I think he's looking for the former, though, like on this board where you can only see your own profile (and probably edit it), but you're correct, $_GET would be better used to only see one profile at a time for others.
-
Sessions are a bit more secure, DarkWater, because you can change a $_GET variable in the URL and there is nothing to check against so that the user can only see their profile. Having a session can (somewhat) ensure that they can only see their own information.
-
It's a call to a class. You can read up on them here http://us3.php.net/zend-engine-2.php
-
[SOLVED] linking a file (pdf) to an article
JD* replied to anthony-needs-you's topic in PHP Coding Help
Try doing a base64_encode when putting it into the database. $fp = fopen($_FILES['userfile']['tmp_name'], 'r'); $content = fread($fp, filesize($_FILES['userfile']['tmp_name'])); $content = base64_encode($content); ...insert into db with $content... and then on the download page, do the same thing but with a base64_decode. Not sure if that's the problem, but I know I had a lot of problems with PDFs read into my database before I did this. -
The code I posted simply sets the $_SESSION variable to the user's ID without putting their name or password into a $_SESSION. Once you're on the profile page, you can do a check to see if the page ID equals the $_SESSION['id'] and if so, place editing links on the page. I'll step you through my code, though, so you can see how it differs from yours: if(check_required2("user_name, password")) { $_SESSION['error'] = "required_missing"; unset($_POST); redirect("http://admin.mysite.com"); exit(); } This is a custom function that checks to see if required fields have been filled out. If they haven't, it sets an error and returns them to the main login page. You can put in your own error checking for this part. $result = mysql_query("SELECT * FROM permissions WHERE userName = '".addslashes($_POST['user_name'])."'") or die(mysql_error()); O.k., we have a name and password, so lets just try to select * from the permissions database (says what a user can and can't access in my case) just by their name alone. if(mysql_num_rows($result) != 1) { $_SESSION['error'] = "nosuchuser"; unset($_POST); display_login(); exit(); } We only expect one user to be returned. If that isn't the case (either no users, or more than one), we put another error message up and redirect them back to the login page. Not perfect, but it works in the cases necessary, and the error message contains instructions to contact the webmaster if they think there's been a mistake. if(md5($_POST['password']) == mysql_result($result, 0, "userPassword")) O.k., so if the user exists, now we take their posted password, apply the md5 encryption to it, and then compare it to what's in the database. Again, not a perfect solution (as the original password gets submitted in plain text) but it helps against SQL injection attacks to have two separate checks instead of one generic that can be escaped. { $_SESSION['id'] = mysql_result($result, 0, "ID"); $_SESSION['logged_in'] = 1; unset($_POST); redirect("?action=home"); exit(); So we've been assured that their name and password is correct, now we set two sessions. One is that they're logged in (in case they come back to the login page later, this is checked to auto log them in) and we also set their ID, so we can check what permissions they have later without comparing names and passwords again. In my case, I have it redirect them with a custom function and also remove any current $_POST content, and also exit out of the script, just as an added precaution ( the redirect is javascript, so if they have it disabled and were able to get this far, I don't want it to error out); } else { unset($_POST); $_SESSION['error'] = "badcombo"; display_login(); } This was in case the password they submitted does not match against the user account. Again, I remove the $_POST information, server them an error and redirect them back to the login page.
-
[SOLVED] linking a file (pdf) to an article
JD* replied to anthony-needs-you's topic in PHP Coding Help
The only error I can see so far is your first if(isset($_GET))...should that be if(!isset($_GET)) or if(empty($_GET))? -
Getting data from mysql database for a specific ID number
JD* replied to Shaba's topic in PHP Coding Help
It's an array assigner. It's saying that for each item in $data (which is an sql result), split the result as columnvalue belongs to (=>) columname. This way, you can directly access $data like this: echo $data['usrname'] and it will return the user's name, etc.