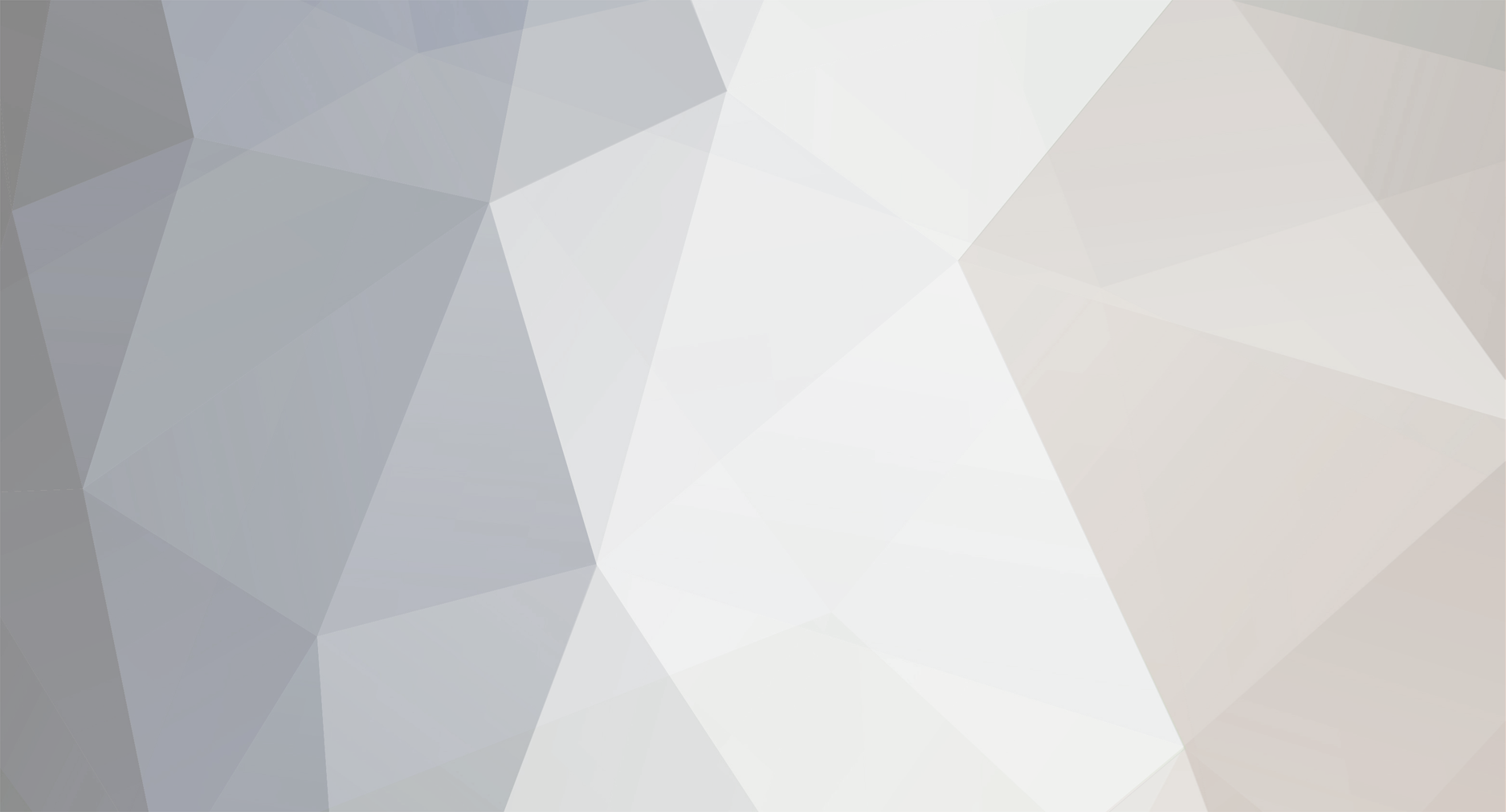
KaiSheng
Members-
Posts
79 -
Joined
-
Last visited
Everything posted by KaiSheng
-
echo doesn't output-- help, please.
KaiSheng replied to SpartanTacoDuckie's topic in PHP Coding Help
Post your whole code here. -
echo doesn't output-- help, please.
KaiSheng replied to SpartanTacoDuckie's topic in PHP Coding Help
This Query is wrong. -
Mysql Query taking too much time. Almost 10 minutes
KaiSheng replied to boomarang's topic in MySQL Help
1) Do not use SELECT * FROM wherever in a query. 2) Follow Barand coding ^ -
echo doesn't output-- help, please.
KaiSheng replied to SpartanTacoDuckie's topic in PHP Coding Help
For the first part of code on the top, it should be: //get the form data $myusername = ($_POST['username']); $mypassword = ($_POST['password']); //check if all fields are filled in if ( ($myusername == "") || (mypassword == "") ) ) { echo "Please fill in all fields"; exit; } --------------- for the second part of code it should be : //check if user exists if (mysqli_num_rows($result) >0) { $row = mysqli_fetch_array($result); $_SESSION['username'] = $row['username']; $_SESSION['password'] = $row['password']; echo "You have been logged in successfully. Please click <a href=account.php>here</a> to continue."; } -
1) I am aware that form can only be post by method=post , not method=get, not sure if yours will work that way. 2) You called for Name and Surname to be echoed, since there is a while loop to fetch array results from db, i think you should do this $Name = $row['Name']; $SurName = $row['SurName']; then followed by echo '$Name' and echo '$SurName' , step by step instead of combining everything together. before you echo it out. 3) $query = "SELECT * FROM mytable WHERE Gender = ' " . $gender. " ' "; change to $query = "SELECT * FROM mytable WHERE Gender = '".$gender."' "; 4) I see an email echoing out from your while loop, while there is no email from the form.
-
how to become a guru ?
-
Try changing this $Addr = $_POST['addr']; to $Addr = $_POST["addr"]; and where does your $nameid come from?
-
Using specific roles codes by allowing access to certain roles is easier too. thats wha't i am doing for my case.
-
Use this and edit yourself. ---- <?php if (isset($_SESSION['user_id'])) { if ($_SESSION['role'] == 'ban') { echo '<meta http-equiv="refresh" content="0; url=ban.php " />'; die(); } elseif ($_SESSION['role'] == 'member'){ ?> <nav> <a href="home.php">Home | </a> <a href ="accountPanel.php">Account Panel | </a> <a href="logout.php">Logout</a><br /> </nav> </header> <?php $link = mysqli_connect($HOST, $USERNAME, $PASSWORD, $DB); //match the username and password entered with database record $query = ("SELECT id,role,username FROM `users` WHERE id='".$_SESSION['user_id']."'"); $result = mysqli_query($link, $query) or die(mysqli_error($link)); $row = mysqli_fetch_array($result); $_SESSION['user_id']= $row['id']; $_SESSION['username'] = $row['username']; $_SESSION['role'] = $row['role']; echo '<p><i>Welcome, '. $_SESSION['username'];?> <br> <?php echo 'You are logged in as ' . '(' . $_SESSION['role'] . ')' ?> <br> <?php } else{ ?> <nav> <a href="home.php">Home | </a> <a href ="adminPanel.php">Admin Panel | </a> <a href="logout.php">Logout</a><br /> </nav> </header> <?php }} else { ?> <nav> <a href="home.php">Home</a> <a href ="register.php">Register</a> <a href="login.php">Login</a><br /> </nav> </header> <?php } ?> -- note, the last part ^ is the condition for user it is not login.
-
Dropdown menu will not output pm only am values to table
KaiSheng replied to edfou's topic in PHP Coding Help
i see that your variables are not properly validated. Lol. -
simple, just do this. Username:<input type="text" name="username" size="17" value="<?php echo $row['username']; ?>" readonly="readonly" > be sure to do this before that code. <?php //match the username and password entered with database record $query = ("SELECT id,role,username FROM `users` WHERE id='".$_SESSION['user_id']."'"); $result = mysqli_query($link, $query) or die(mysqli_error($link)); $row = mysqli_fetch_array($result); $_SESSION['user_id']= $row['id']; $_SESSION['username'] = $row['username']; ?>
-
i got it. i did this. $query = "SELECT id,email from users ORDER BY RAND() LIMIT 1"; Thanks for whoever taking their time to read /Thread solved.
-
rand(0,100) will not work in my case. I am trying to get a string not integer. Problem : I want to get 1 username of all username in my database. (randomed ones) Anyone know of this? mind helping me? --- code -- $query = "SELECT id,email from users"; $result = mysqli_query($link, $query) or die('error query'); while ($row = mysqli_fetch_array($result)) { $id = $row['id']; $username = $row['username']; echo '$username'; This gives all username, but i only want 1 randomed one. which part of code do i add on?
-
okay got it. thanks!
-
I'm an IT student from singapore. Nice to meet you all. I have been taking up projects all mainly on web-based applications. If i need any help, hope you guys will be able to help.
-
According to what you said, "When a teacher chose class, list of student name will appear in a table." This is ajax. Wrong section. Please refer to here. http://forums.phpfreaks.com/forum/38-ajax-help/
-
I do not know how to include the date they played. Because it's an update query, so the field must not be empty. For example, using INSERT query, the field in database must be empty before this will work. using UPDATE query, the field in database must not be empty. I have no idea how this is gonna work using date timestamp, although I know this is an easier way.
-
Guru is right, absolutely. Lol.
-
<form action="something.php" method="post"> <input type="radio" name="preferred_color" value="Red" /> Red<br /> <input type="radio" name="preferred_color" value="Blue" /> Blue<br /> <input type="radio" name="preferred_color" value="Green" /> Green<br /> <input type="submit" value="Submit"> </form> example ^ u can edit on your own. ;o
-
Hi all again!! I will describe the game first. According to my game, the person who plays the game will click on a "play" button, and status of the user will change from available to played. So, according to my query, i used "UPDATE users SET status ='played'" I want to include something more. anyone know how to include the query for the status to auto change (from played to available) every 12:00am (GMT+8 ) ? Greatly appreciated for all comments.
-
Well, hi again. According to my previous thread, it works perfectly. url will show ...gameInfo.php?id=1 or gameInfo.php?id=2 according to the person who login, they will received the respective links that is matched to the user id in database. Okay, so here's the problem, when i am user id 2, it shows as gameInfo.php?id=2 However, when i change the url to gameInfo.php?id=1, i can see the information of the user of userid 1. Is there any way to go about this? Help thanks <3 !
-
Thanks!! problem fixed! on the home page code was $query = ("SELECT * FROM `users` "); i changed to $query = ("SELECT id,role FROM `users` WHERE id='".$_SESSION['user_id']."'"); !! Thanks Ch0cu3rfor the hint!!!!! THANKS. HAHAHAHHAA. SO HAPPY NOW. SPENT 2 DAYS ON THIS SHIT ):
-
This is my login code. -- <?php session_start(); if (!isset($_SESSION['user_id'])) { if (isset($_POST['username'])) { //retrieve form data $username = $_POST['username']; $password = $_POST['password']; //connect to database $HOST = 'localhost'; $USERNAME = 'root'; $PASSWORD = ''; $DB = 'db'; $link = mysqli_connect($HOST, $USERNAME, $PASSWORD, $DB); //match the username and password entered with database record $query = ("SELECT * FROM `users` WHERE `username`='$username' AND `password`='".sha1($password)."'"); $result = mysqli_query($link, $query) or die(mysqli_error($link)); //if record is found, store id and username into session if (mysqli_num_rows($result) >0) { $row = mysqli_fetch_array($result); $_SESSION['user_id'] = $row['id']; $_SESSION['role'] = $row['role']; $msg = '<p><i>Hello, ' . $row['username'] . '!<br />'; $msg .= 'You are logged in.<br /><a href="home.php">Home</a></p>'; } else { //record not found $msg = '<p class="error">Sorry, you must enter a valid username and password to log in.<a href="login.php"> Back</a></p>'; } } } else { $msg = 'You are already logged in.<br /><a href="home.php">Home</a></p>'; } ?>
-
To make it more specific, i will post my code here. home page) $query = ("SELECT * FROM `users` "); $result = mysqli_query($link, $query) or die(mysqli_error($link)); $row = mysqli_fetch_array($result); $_SESSION['user_id']= $row['id']; $_SESSION['role'] = $row['role']; $status= $row['status']; echo '<p><i>Welcome!<br />';?> <br> <?php echo 'You are logged in as ' . '(' . $_SESSION['role'] . ')' ?> <br> <?php echo 'Wanna play a game and win prizes?'?> Click <tr> <td><a href="gameInfo.php?id=<?php echo $_SESSION['user_id']; ?>">Here</a></td> --- gameInfo page) $id = $_GET['id']; $sql = "SELECT * FROM users WHERE id=" . $id; $result = mysqli_query($link, $sql) or die(mysqli_error($link)); $row = mysqli_fetch_array($result); } ?> <tr> <td>Click <a href="game.php" onclick="return confirm('Do you want to test your luck?')">Play Now!</a></td> --- The problem is that the session id is always the 1st user id even i am using different session id to login to the website. --- please, anyone help me please? I believe is that I have wrong variables on going. However I do not know which is the wrong one as there is no error appeared on the screen.