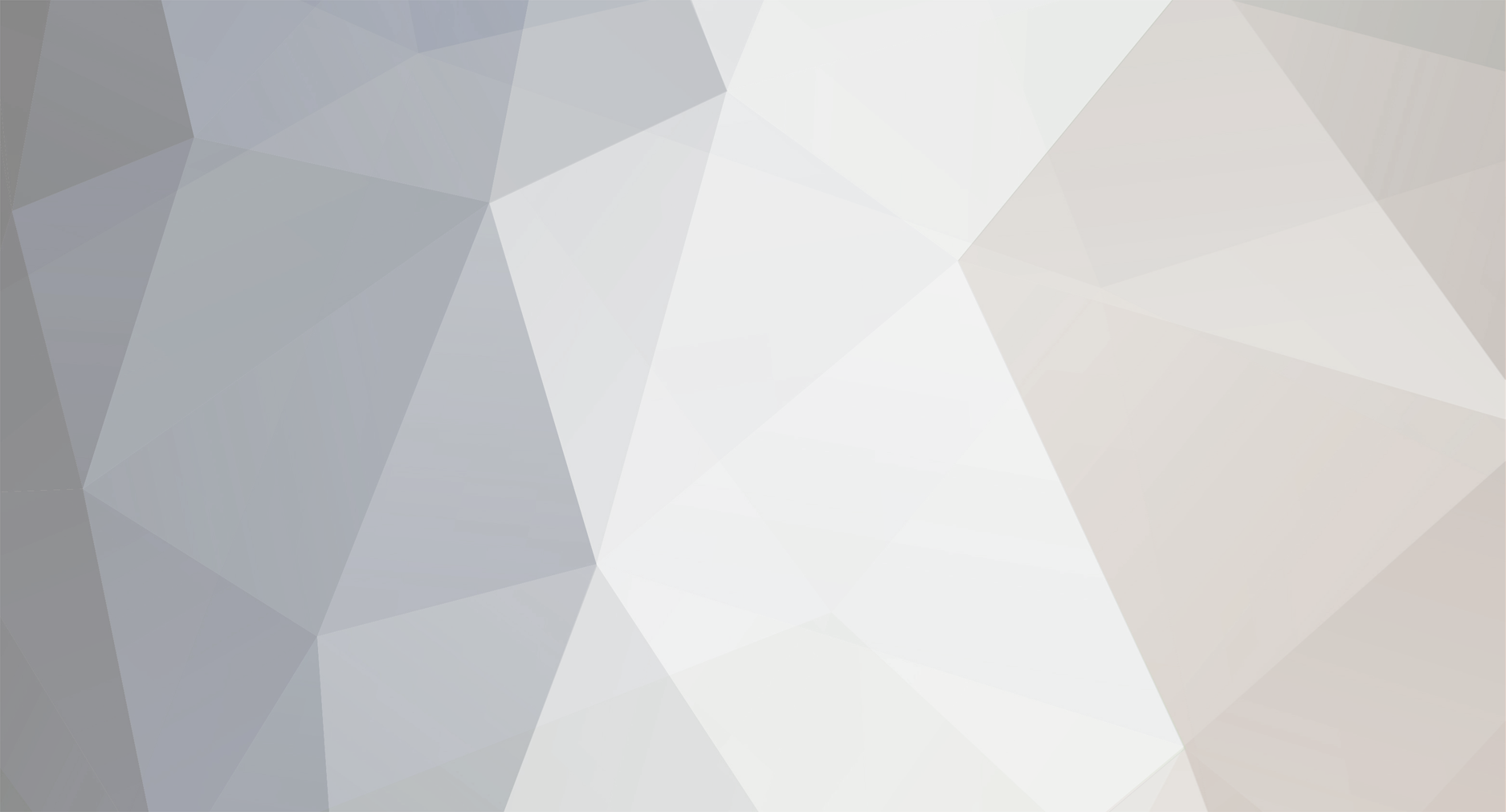
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
Did you not try my code from your previous post
http://www.phpfreaks.com/forums/index.php?topic=333081.msg1568706#msg1568706
-
You're using the wrong variable here
$f1 = "From:" . mysql_result($messrow,$i,"sender");
You want to use the $messageres variable (this is the result resource)
-
To store the serialized array you'll want to use the TEXT data type.
To get array indexes (keys) you can use array_keys.
-
Not quite understanding your question by seems youre wanting to do store the actual array within your database? If you do then you need to serialize it first. Then when you get it out of the database you'll need to unserialize it to convert it back to an array. The data type you'll want to use would be TEXT.
-
Characters such as ' or " will cause problems within your sql queries. You need to escape these characters. To prevent this you should pass all string values to mysql_real_escape_string before using them within your queries. Example
$name = mysql_real_escape_string($_POST['name']);
-
Use number_format rather than substr maybe?
-
Remove this line.
$petinfo = mysql_fetch_array($petcrap);
And change $petinfo to $petcrap on this line
while ($row = mysql_fetch_array($petinfo)){
-
You only need to escape the single quotes if you are stating the string with a single quote. You don't need to escape the double quotes too!
-
-
-
Search google for PHP pagination
-
What you want to do is change this
echo "<td><input type = 'text' name = 'udata[fname][{$list['id']}]' value = '{$list['fname']}' />\r\n"; echo "<td><input type = 'text' name = 'udata[lname][{$list['id']}]' value = '{$list['lname']}' />\r\n"; echo "<td><input type = 'text' name = 'udata[dob][{$list['id']}]' value = '{$list['dob']}' />\r\n"; echo "<td><input type = 'text' name = 'udata[email][{$list['id']}]' value = '{$list['email']}' />\r\n";
to
echo "<td><input type = 'text' name = 'udata[{$list['id']}][fname]' value = '{$list['fname']}' />\r\n"; echo "<td><input type = 'text' name = 'udata[{$list['id']}][lname]' value = '{$list['lname']}' />\r\n"; echo "<td><input type = 'text' name = 'udata[{$list['id']}][dob]' value = '{$list['dob']}' />\r\n"; echo "<td><input type = 'text' name = 'udata[{$list['id']}][email]' value = '{$list['email']}' />\r\n";
Next change
// UPDATE: if we have name(s) to change... if($_POST['udata']) { /* // for each name to change... foreach ($_POST['udata'] as $input => $v) { echo "$input = $v<br />"; $sql = "UPDATE test SET "; foreach ($v as $id => $value) { echo "$id = $value<br />"; } $sql .= "WHERE ..."; } echo "<hr />$sql<hr />"; print_r($_POST['udata']); */ for ($i = 0; $i < count($_POST['udata']['fname']); $i++) { $fname = $_POST['udata']['fname'][$i]; echo "$i = $fname<br />"; } /* foreach($_POST['cname'] as $cid => $cname) { // little bit of cleaning... $id = mysql_real_escape_string($cid); $fname = mysql_real_escape_string($cname); $lname = mysql_real_escape_string($cname); $dob = mysql_real_escape_string($cname); $email = mysql_real_escape_string($cname); // update name in the table $sql = "UPDATE test SET fname = '$fname', lname='$lname', dob='$dob', email='$email' WHERE id = '$id'"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); } // end foreach */ } // end if
to
// UPDATE: if we have name(s) to change... if($_POST['udata']) { // update data foreach ($_POST['udata'] as $record_id => $fields) { $sql = "UPDATE test SET "; $field_data = array(); foreach($fields as $name => $value) { $value = mysql_real_escape_string($value); $field_data[] = "$field = \"'$value'\""; } $sql .= implode(', ', $field_data); $sql .= "WHERE id=$record_id"; echo "<hr />$sql<hr />"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); } } // end if
-
To output a chunk of html you can do either of the following
Using single quotes (make sure you escape any ' within your string using \')
echo '<div class="textColor"> <h1>title</h1> <p> Hello phpfreaks</p> <h2> some title</h2> <p> another paragraph</p> </div>';
Or double quotes (make sure you escape any " within your string using \")
echo "<div class=\"textColor\"> <h1>title</h1> <p> Hello phpfreaks</p> <h2> some title</h2> <p> another paragraph</p> </div>";
Or using heredoc syntax
echo <<<HTML <div class="textColor"> <h1>title</h1> <p> Hello phpfreaks</p> <h2> some title</h2> <p> another paragraph</p> </div> HTML;
Or you can go in/out of PHP
<?php // your code here ?> <div class="textColor"> <h1>title</h1> <p> Hello phpfreaks</p> <h2> some title</h2> <p> another paragraph</p> </div> <?php // some more code here ?>
-
Its not doing anything because you're not calling your commPortal() function.
-
This topic has been moved to Apache HTTP Server.
-
You cannot place JavaScript code within PHP code. $_POST['password'] will get the text the user typed in the password field when the form has been submitted.
-
Then you should be setting up only two tables, users and diaries. When the user registers you add them in to the users table. When they add a diary entry you insert it into the diaries table. Creating separate tables for each user is bad database design.
-
Add \n after $AS and before the "
-
You most likely need to wrap $name,$Level,$CT,$Kills,$Deaths,$killdeaths,$AS within double quotes. You cannot pass multiple parameters to fwrite
fwrite($open, "$name,$Level,$CT,$Kills,$Deaths,$killdeaths,$AS");
fwrite can only take three arguments, quoted from the manual.
int fwrite ( resource $handle , string $string [, int $length ] )
-
What are you trying to do? Can you post a more descriptive explanation.
Also when posting code wrap it within
tags.
-
Any reason why you're creating a new table for each user? Why not just have one tabled called users which stores all users personal details.
-
Are you wanting to add all the keywords returned from your query into the $_SESSION['scope'] array? If so all you need to do is
while($row = mysql_fetch_assoc($get)); { $_SESSION['scope'][] = $row['keyword']; }
-
Sounds to me you need to create a dynamic BBCode Parser which replaces [pedigree id=20] with data from your database? If you look at how BBCode parsers are made you maybe able to come up with a solution.
-
Make sure you are using mysql_data_seek() before your second dropdown menu. Do not place it inside the while loop.
explode? array? list? need to break values from msql text box for select list.
in PHP Coding Help
Posted
If all $allwordsgenral contains a comma delimited list of words then change
to
NOTE: When posting code please wrap it within
or
) tags.