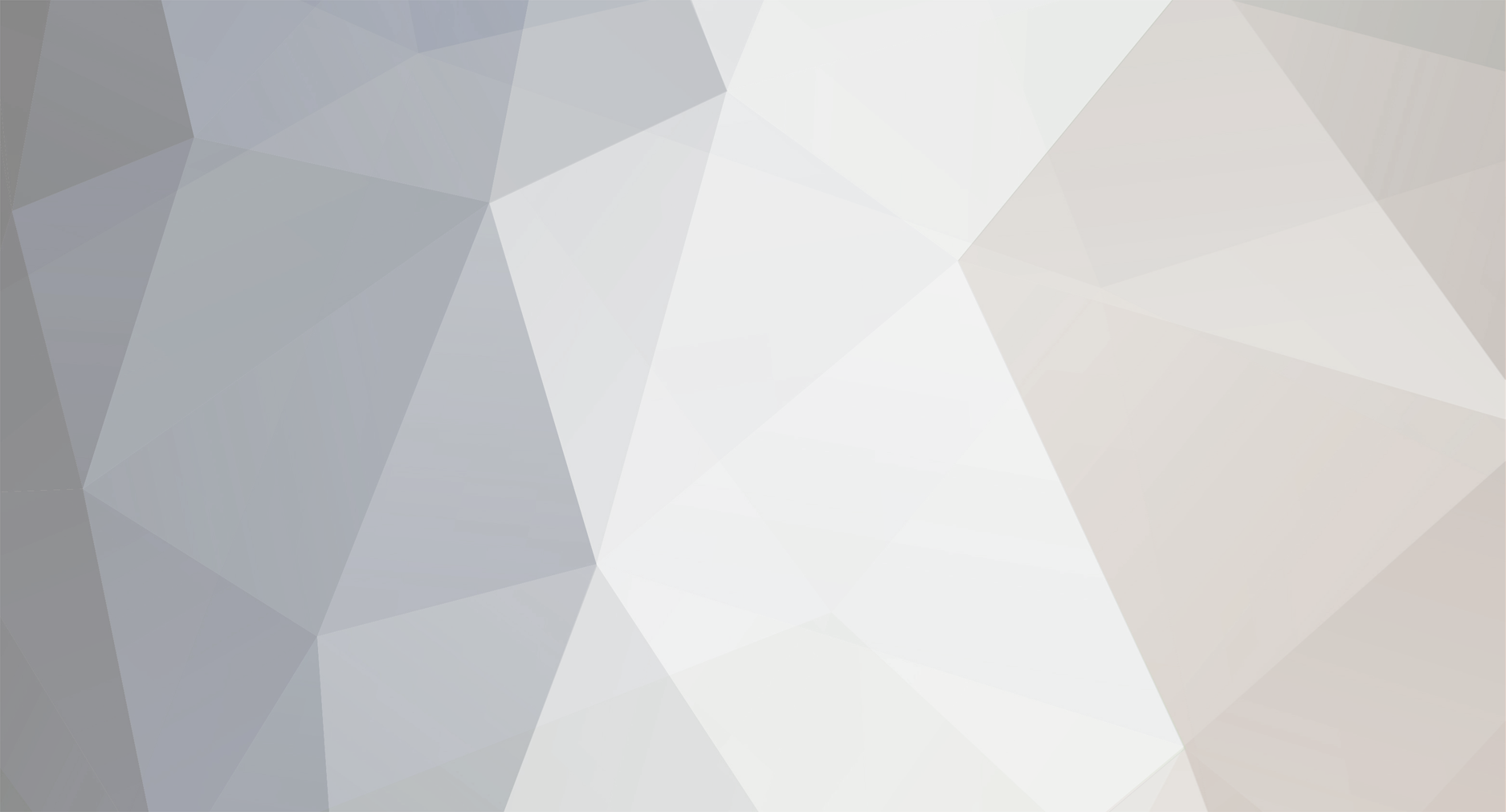
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
What do want to the results to be ordered by? The cust_id field in numerical order
SELECT cust_id, customer FROM customerinfo ORDER BY cust_id ASC
or the customers field in alphabetical order?
SELECT cust_id, customer FROM customerinfo ORDER BY customer ASC
if you don't specify an order it will just return the results in the order they where added into the database.
-
If you are submitting the form to itself then leave the action attribute empty
<form name="search" method="post" action="<?=$PHP_SELF?>">
-
What is outputted when you echo $search
echo "'$search'";
-
You just need to separate your queries
// get the cid $cname = mysql_real_escape_string($_POST['cname']); $getCID = "SELECT cid FROM course WHERE cname='$cname'"; $result = mysql_query($getCID); if($result) { $row = mysql_fetch_assoc($result); $course_id = $row['cid']; // add the student to the course_student table $addCID = 'INSERT INTO course_student (cid, sno) VALUES(' . $row['cid'] . ', ' . $YOUR_STUDENT_ID_VARIABLE . ')'; mysql_query($addCID) or die('Error:' . mysql_error()); }
Or If the student is already within your course_student table then you'll run an update query
$addCID = 'UPDATE course_student SET cid=' . $row['cid'] . ' WHERE sno=' . $YOUR_STUDENT_ID_VARIABLE;
-
You're sort of one the right track. The issue you have is variables are not parsed within single quotes. You should instead concatenate your variable into the string
<?php $files = glob( './docs/' . $row_fullname['department'] . '/*.*' ); foreach ( $files as $file ) { echo '<a href="./docs/' . $row_fullname['department'] . '/' . basename( $file ) . '"target="_blank">' . basename( $file ) . '</a><br />'; } ?>
-
If my code is outputting
SELECT * FROM products WHERE keywords LIKE '%testtest%'
Then I don't think you're using my code correctly. As my code should be generating your query as
SELECT * FROM products WHERE keywords LIKE '%test%' OR keywords LIKE '%test%'
How I tested my code
$search_exploded = explode(' ', 'test test'); $searches = array(); foreach($search_exploded as $search_each) { $searches[] = "keywords LIKE '%$search_each%'"; } $construct = "SELECT * FROM products WHERE " . implode(' OR ' , $searches); echo $construct;
-
Hi there i have an array that catches all appropriate records from my database and selects a random row which is then outputted as a hyperlink.
If all you're doing is wanting to displaying a random row. Then there is no need to get all the rows from mysql and then choose a random row with PHP. MySQL can select a random row, example query.
SELECT * FROM ships WHERE PlayerName = %s AND Class=%s AND Template='1' ORDER BY rand() LIMIT 1
-
I don't get your code, you are exploding your string ($data) on a quote. But your string doesn't contain any quotes. What are trying to do only allow four words to be in a string.
-
Change your foreach loop to
$searches = array(); foreach($search_exploded as $search_each) { $searches[] = "keywords LIKE '%$search_each%'"; } $construct = "SELECT * FROM products WHERE " . implode(' OR ' , $searches);
-
Mysql_query() can only process one query at a time. So you will have to get the cid from the course table first and then insert the cid into your course_student table.
-
if the sno field auto_increments you'd do
mysql_query($sql) or die('Error:' . mysql_error()); $sno_id = mysql_inser_id();
$student_id will now hold the id of the student just inserted into your database.
You can assign $student_id to a session so you have access to it throughout your site.
$_SESSION['student_id'] = $student_id;
In order for sessions to work properly make sure you call session_start as the first line of your PHP scripts.
-
You can get the generated id of the previously inserted row with mysql_insert_id
-
I think the issue is to do with the onClick attribute
... <a href="#" onClick="CCPEVE.showInfo{1377, 359320675}>StyphonUK</a> ...Notice you have left of the closing double quote after the } and before the >. That could be the issue.
Change
echo "<td><a href=\"#\" onClick=\"CCPEVE.showInfo{1377, " . $charId . "}>" . $charName . "</a></td>";
to
echo "<td><a href=\"#\" onClick=\"CCPEVE.showInfo{1377, " . $charId . "}\">" . $charName . "</a></td>";
-
Your code is correct. There are no problems I can see which could trigger an error. If you go to your page and right click view source what html is being generated
-
What do you mean by not outputting properly in the While loop? Is it not parsing your variables or is the HTML table not displaying properly
-
With those changes it should be working,
Entering 70 for weight and choosing Kg outputs You weight 70Kg
Entering 155 for weight and choosing lbs outputs You weight 70.30681735 Kg
Define what is not working.
-
The real reason for that error will be logged within your sites error log. That error usually occurs due to problem with the servers configuration. PHP may also trigger the error if there is a problem in your script. Bluehost should provide a facility for viewing your logs.
-
On these lines
elseif($weight != '' AND $units = "kg") ... elseif($weight != '' AND $units = "lbs")
You should be using the comparison operator (==) not the the assignment operator (=) for checking what $units is set to.
-
You'll need to use a third foreach loop to echo the key/value pairs for the $products['pens']['sex'] array
foreach ($products as $section => $items) { foreach ($items as $key => $value) { echo "$section:\t$key\t($value)<br>"; // check whether the current item is an array! if(is_array($value)) { echo "$key is sub array:<br />"; foreach($value as $subKey => $subValue) echo "$subKey:\t$subValue<br />"; } } }
-
Rather than use echo within your function you'd use return
-
I don't thing you can use the matches returned from regex to call a variable/array item. Instead you'll need to use preg_replace_callback. Example
$array[1] = 'test'; $array[23] = 'foobar'; $content = 'test-23<br /><br />test-1'; $match = preg_replace_callback('|test-([0-9]+)|', create_function('$matches', 'global $array; return $array[$matches[1]];'), $content);
-
If you want to run PHP code on the replacement you'll need to use the e pattern modifier.
$match = preg_replace('|test-([0-9])|e', "$array[\\1]", $content);
EDIT Just been testing my suggestion and I think you may need to use preg_replace_callback instead.
-
One way you can do it is with CSS. Add a new stylesheet to your webpage that is only used for printing (make sure you give it the media type of print), eg
<link rel="stylesheet" type="text/css" media="print" href="print.css" />
What will happen now is your web browser will apply the styles within print.css and override the css defined in your main stylesheet.
Or in PHP just create a new file which only outputs the bare minimum data needed for printing.
-
You will need to do two things. First change product.php so your links are in your new url format mysite.com/1/product-name-here
Second use mod_rewrite to map site.com/1/product-name-here to product.php
linking tables
in PHP Coding Help
Posted
Your query here is all wrong
That code will produce the following query
SELECT fname,sname FROM student WHERE Array = 'sno'
That query will tell MYSQL to fetch the fname and sname fields from the student table where the field Array equals sno.
What you want to do is use a JOIN, Example query
Your fixed code