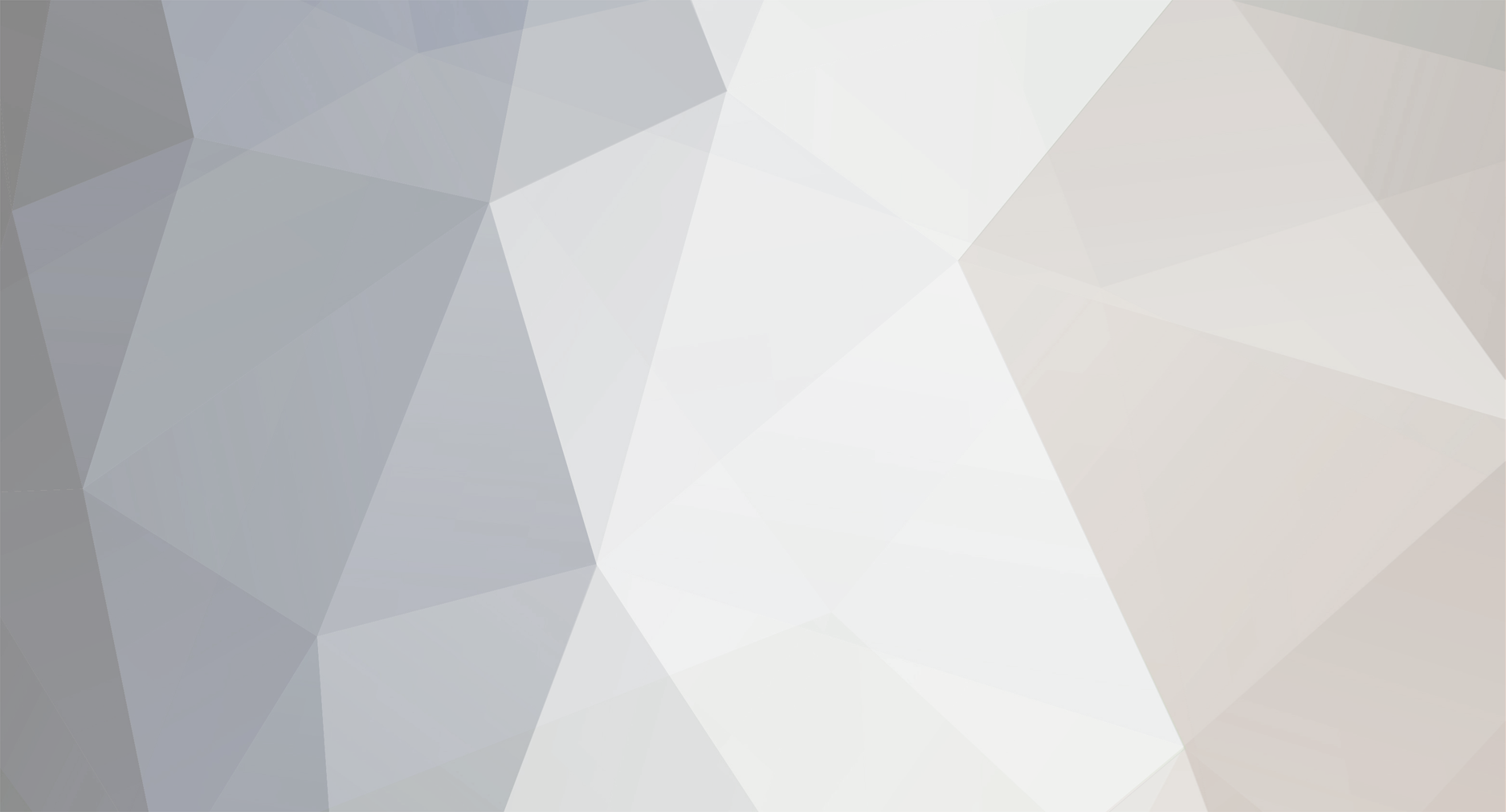
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
Because your url is now site.com/users/username for viewing a users profile. Your browser is thinking user/ is a directory. It is trying to load your links/images within this non existent directory. To prevent this start your url paths with a /
For example
<a href="/link.php">Link</a> OR <img src="/image.png" />
-
Change the rewrite rule to
RewriteRule user/([a-z0-9_-]+)/? members.php?username=$1 [NC,L] -
This topic has been moved to PHP Applications.
-
You can map domian.com/user/user to domain.com/members.php?username=user using
RewriteEngine On RewriteRule user/([a-z0-9_-]+)/? members.php?user=$1 [NC,L]
In members.php you will need to modify your existing links so they adopt your new url format. Mod_rewrite will not do this for you. For example you may output your links like this
<a href="members.php?user=<?php echo $username; ?>"><?php echo $username; ?></a>
You'll need to change the above to
<a href="/user/<?php echo $username; ?>"><?php echo $username; ?></a>
-
nl2br is your friend
-
To get random results use ORDER BY rand() and use LIMIT 3 to limit the results to 3 rows.
SELECT * FROM users order by rand() limit 3
-
This topic has been moved to Installation in Windows.
-
Is because you have invalid html
<input type="hidden" name="event_id" value=<?php echo $event_id; ?>> <input type="hidden" name="park" value=<?php echo $park; ?>> <input type="hidden" name="orderdate" value=<?php echo $orderdate; ?>> <input type="hidden" name="description" value=<?php echo $description; ?>> <input type="hidden" name="meetingPlace" value=<?php echo $meetingPlace; ?>> <input type="hidden" name="leader" value=<?php echo $leader; ?>> <input type="hidden" name="sponsor" value=<?php echo $sponsor; ?>> <input type="hidden" name="hour" value=<?php echo $hour; ?>> <input type="hidden" name="min" value=<?php echo $min; ?>> <input type="hidden" name="ampm" value=<?php echo $ampm; ?>>
HTML attribute values should be wrapped in quotes, eg value="<?php echo $meetingPlace; ?>"
-
Probably due to this
date('Y', mktime(0,0,0,0,0,$i-1))
. You do not need to have that as $i already contains the year.
-
No, what MadTechie's suggestion does is passes the value of $cell1['value'] in your url.
So if $cell1['value'] is set to 'hello' you'll see the following code
<a href='file.php?mycell=<?php echo $cell['value'];?>'>
Will produce the following url yousite.com/file.php?mycell=hello
When you echoi $_GET['mycell'] in file.php it will return 'hello'. It doesn't access the variable $cell1['value'].
-
Why are you performing three queries on the same table and only fetching one field at a time? MySQL can fetch more than one field per query.
Also if your query is only going to return one result you do not need to use a while loop.
As for the error you need to check whether your queries are returning any errors. You can do this using mysql_error
-
You want to de increment $i (--$i) not increment ($i++)
for($i = 1988; $i >= 1911; --$i)
-
This topic has been moved to Installation in Windows.
-
Change the default port xampp runs on. By default it will use port 80 same as IIS. Changing the port is explained here
-
You'll want to use a switch statement. Not define.
switch($row['userlevel']) { case 1: echo 'Külaline'; break; case 9: echo 'Administraator'; break; }
When $row['userlevel'] is 1 it'll echo Külaline.
When $row['userlevel'] is 9 it'll echo Administraator.
-
im doing it wrong can some one help me ? , thanks
{
define('userlevel'( 9="admin" , 1="guest"));
}
It helps if you tell use what you're trying to do with it.
The function define is used to create constants. Constants are like variables expect you cannot change their values once you have created them. I don't think this is the function you want to use.
I think what you're trying to do is associate a users userlevel with a title, eg when a user has a userlevel of 9 give them the title of admin. If it is 1 give them a title of guest.
-
What is your code supposed to do?
-
Sorry the code I gave you is incorrect. I didn't think it through properly. In your query you'll actually need to perform a join on both the course_student and course tables. Try this code
<?php if(isset($_GET['cid'])) { $cid = (int) $_GET['cid']; $query = "SELECT student.fname, student.sname, course.cname FROM student LEFT JOIN course_student ON (course_student.sno = student.sno) LEFT JOIN course ON (course_student.cid = course.cid) WHERE course_student.cid = $cid"; $result = mysql_query($query); if($result) { if(mysql_num_rows($result) > 0) { $courseName = mysql_result($result, 0, 2); // get the course name echo 'Student\'s Signed up to course: <b>' . $courseName . '</b><br />'; mysql_data_seek($result, 0); // reset the internal data pointer back to the first row in the result set. while($row = mysql_fetch_assoc($result)) { echo $row['fname'] . ' ' . $row['sname'] . '<br />'; } } else { echo "No results returned!"; } } else { echo "Problem with the query: <pre>$query</pre>Error: " . mysql_error(); } } ?>
-
Its due to $eh[] and $eh1[]. Get rid of the square brackets.
The following should do it.
$lchild = tree_gather($array['lchild']); $rchild = tree_gather($array['rchild']); $child = array_merge($lchild, $rchild);
-
the table might have 80 records, but you only get the first one.
If it is only showing one record then you need to check your query as well as the code that processes the query. However the snippet of code you posted should be displaying the results from your query fine. However there is no need for a do/while loop just a plain while loop will be fine.
while ($row_info = mysql_fetch_assoc($info)) { echo' <tr> <td>' .$row_info['uname']. '</td> <td>' .$row_info['realname']. '</td> <td>' .$row_info['memloc']. '</td> <td>' .$row_info['regdate']. '</td> </tr>'; }
-
Your code does not work as expected because your logic is flawed from the start. Such as why the need for this:
$rand = rand(1,3); if ($rand == 1) { $rand = rand(1,3); if($rand == 1) {
That makes no logical sense at all. The likelyhood of rand(1,3) returning the number 1 twice on every page load is very unlikely. So when you first go to your page it may return the number 1 twice and show your form for claiming the prize. But when you submit your form it'll reload your page and this time rand(1,3) will return a different number other than one and so your code will never run. I suggest that you remove those two if's.
You can select a random row using MySQL. Simply add the following code ORDER BY rand() LIMIT 1 to the end of your query.
$sql = "SELECT * FROM randomevents WHERE rarity = '1'"; $result = mysqli_query($cxn, $sql); while ($row = mysqli_fetch_assoc($result)) { $event[] = $row['phrase']; } //This will pick a random event and show it $renum = rand(0,count($event)-1); $display = $event[$renum];
So with that addition your code then just becomes
$sql = "SELECT phrase FROM randomevents WHERE rarity = '1' ORDER BY rand() LIMIT 1"; $result = mysqli_query($cxn, $sql); $row = mysqli_fetch_assoc($result) $display = $row['phrase'];
This code
if (isset($_POST['claimitem'])) { $sql = "SELECT * FROM useritems WHERE itemid='".$itemid."' AND userid='".$_SESSION['userid']."'"; $result = mysqli_query($cxn, $sql) or die(mysqli_error($cxn)); $num = mysqli_num_rows($result); if ($num == 0) { $sql = "INSERT INTO useritems (userid, itemid, name, image, quantity) VALUES ('".$_SESSION['userid']."', '".$itemid."', '".$itemname."', '".$itemimage."', '1')"; mysqli_query($cxn, $sql) or die("Query died: insert for itemgain"); } else { $sql = "UPDATE useritems SET quantity = quantity+1 WHERE itemid='".$itemid."' AND userid='".$_SESSION['userid']."'"; mysqli_query($cxn, $sql) or die("Query died: update for itemgain"); $eventdisplay = "<table cellspacing=\"0\" class=\"events\" align=\"center\"> <tr> <td width=\"350px\"><center><b><h1>Random Event</h1></b></center></td> </tr> <tr> <td><img src=\"http://www.elvonica.com/".$image."\" style=\"position:relative;float:left;\"> <p><center>".$display."</center></p> </td> </table><br>"; }
Should be at the top of your file
Also in order for the code to to either update/insert the random item in your database you need to pass the $itemid from your form. Eg
$eventdisplay = "<table cellspacing=\"0\" class=\"events\" align=\"center\"> <tr> <td width=\"350px\"><center><b><h1>Random Event</h1></b></center></td> </tr> <tr> <td><img src=\"http://www.elvonica.com/".$image."\" style=\"position:relative;float:left;\"> <p><center>".$display."</center></p> <p> <form action=\"".$_SERVER['SCRIPT_NAME']."\" method=\"post\"> <input type=\"hidden\" name=\"itemid\" value=\"$itemid\" /> <input type=\"submit\" name=\"claimitem\" value=\"Claim Egg!\" /> </form> </p> </td> </table><br>";
Now you'll get the itemid from your form using $_POST['itemid'].
-
The code you are posting should be working fine provided you using the same username/password you added into your admin table. When you added a row to your Admin table in phpmyadmin what did you type for the username and password?
-
This topic has been moved to Third Party PHP Scripts.
-
You are overwriting the variables you defined for your first query ($sql, $result and $row) when you go to run your second query:
$sql = "SELECT * FROM items WHERE itemid='".$itemid."'"; //selecting the name and image of the item that is displaying $result = mysqli_query($cxn, $sql) or die(mysqli_error($cxn)); $row = mysqli_fetch_assoc($result);
However performing a second query from the results of your first query is not recommended. This is an inefficient way of getting data from your database as it takes up server resources. What you can do is use an alternative query (called a join) which will grab the necessary data from both your useritems table and items table using just one query.
preg_match multiple finds
in PHP Coding Help
Posted
An easier method is to use glob