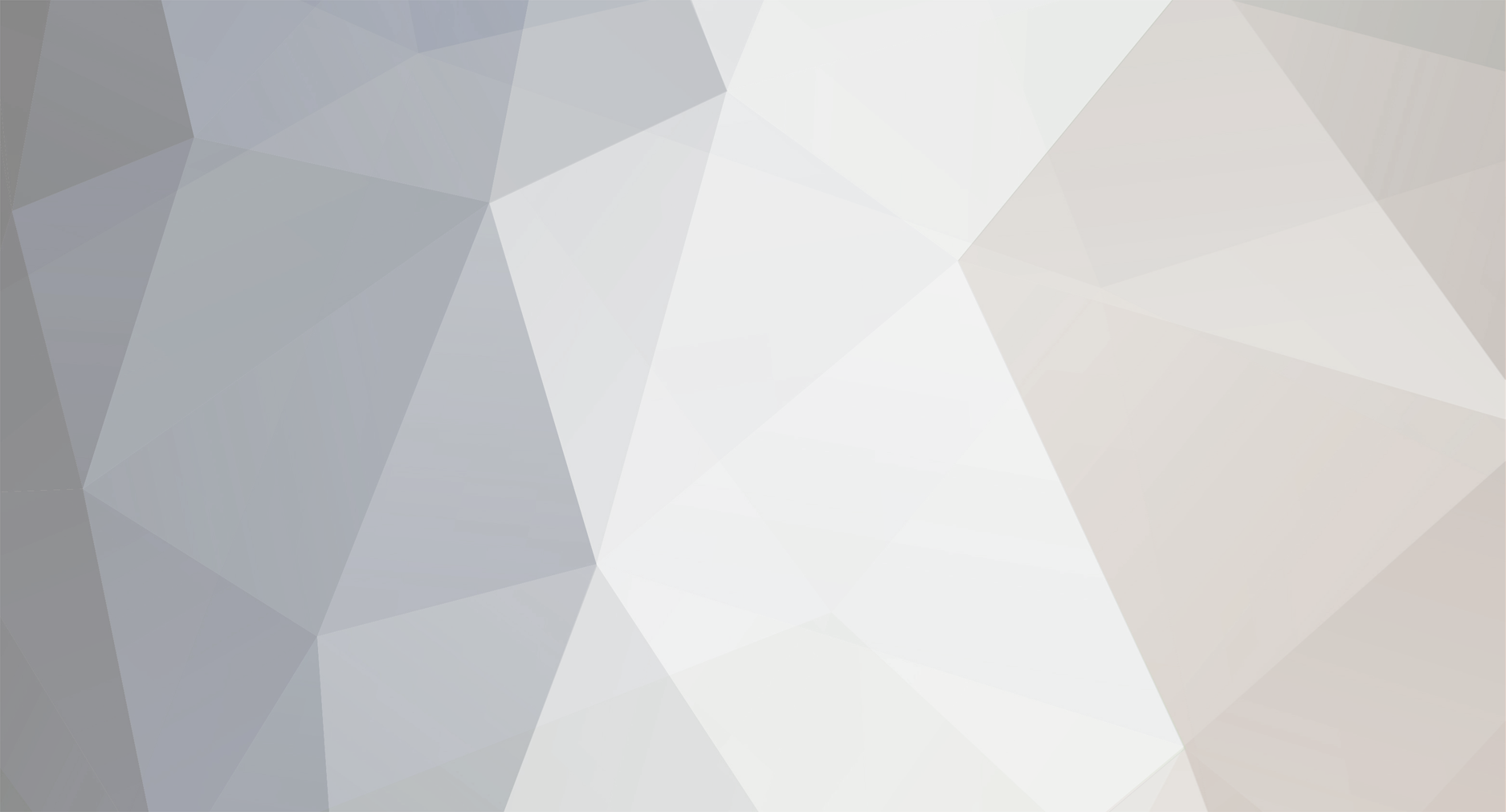
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
The <optgroup> tag is to be used within a <select> list only, eg
<select> <optgroup label="Swedish Cars"> <option value="volvo">Volvo</option> <option value="saab">Saab</option> </optgroup> <optgroup label="German Cars"> <option value="mercedes">Mercedes</option> <option value="audi">Audi</option> </optgroup> </select>
You probably want to use a div or fieldset/legend tags maybe?
-
-
How does your script load login.tpl? If you are using include/require then the PHP code within login.tpl will be executed. If you're using fopen/fread or file_get_contents() then the PHP code will not be executed and it'll returned as text.
-
-
The extensions php_mysql.dll and php_mysqli.dll require an external library called libmysql.dll in order to load properly. This file should be in C:/PHP can you confirm that file is present? If that file does not exist then I guess you used the PHP installer
If you did use the installer go to php.net and download the zipped binaries version of PHP 5.2.17 and extract the contents of this zip to C:/PHP overwriting any existing files. The installer never installs all the required files for extensions to load properly. The zip file contains all required external libraries for most of the extensions.
After unzipping restart the Apache service.
-
Sounds like you need to remove the @user within $row2['message'];
}else{ $msg = str_replace("@$mto", '', $row2['message']); echo "<font color='red'>@$mto</font> $msg"; }
-
First in your second page where you display the data to be edited you are only showing one field to be edited, which is the Property_Rating.
<td align="center"> </td> <td align="center"><strong>Property_Name</strong></td> <td align="center"><strong>roperty_Rating</strong></td> <td align="center"><strong>Property_Address</strong></td> </tr> <tr> <td> </td> <td align="center"><input name="Property_Rating" type="text" id="Property_Rating" value="<? echo $rows['Property_Rating']; ?>"></td> <-- the only editable field you have </tr>
You do not have a field for Property_Name or Property_Address. However in the page you update the data in the database you are trying to update the Propety_Name field! You should add in the missing form fields.
In your update page the variables $Property_Name and $id are undefined. Before updating your database you should first get the data from your form. That data is stored within the $_POST superglobal. Example
$id = $_POST['id']; $Property_Name = $_POST['Property_Name']; $Property_Address = $_POST['Property_Address'];
-
Not sure what you mean but change
function fetch_user_info_by_id() { global $user_info;
to
function fetch_user_info_by_id($id = null) { global $user_info; if(is_null($id)) $id = $user_info['uid'];
Next change
WHERE `users`.`id` = '${user_info['uid']}'";
to
WHERE `users`.`id` = '$id'";
-
Have a look at Multi Column Results within the Code Repository
-
You will want to use mysql_affected_rows rather than mysql_num_rows() when checking to see how many rows was updated by your query
-
This topic has been moved to Third Party PHP Scripts.
-
In order to run PHP code on your computer you need to install a http server (IIS or Apache) which is configured with PHP. Simply just loading test.php directly into your web browser will not work.
If you have installed a Server that is configured with PHP then make sure you are saving the php files in your servers root folder and going to http://localhost/test.php to run it.
-
If your table is not displaying correctly it is probably due to your script outputting invalid HTML.
-
I all have a system path set up in Windows to C:\PHP
In order for the new system path to take affect you need to restart your computer.
-
$percentage should be $percent
-
You need encode the array you're sending to your AJAX script as json. Use json_encode.
-
Your using preg_match incorrectly here
o in the "case" section I added thiscase preg_match("/{SUBGAME[1-9]?}/",$html_file):
preg_match will return 1 if it found a match and 0 if it didn't.
The third parameter for preg_match will return any array of matches. Syntax for preg_match: preg_match(pattern, subject, $matches)
NOTE: preg_match will return one match from the pattern. If you have multiple {subgameX}'s you need to use preg_match_all.
-
What you'll want to do is store your countries in an array
$countries = array('Afganistan', 'Albania', 'Algeria', 'American Samoa', 'Andorra', 'Angola', etc..);
Then use a loop to output each country into an <option></option>. Within this loop you'll have an if statement which selects the chosen country.
<select name="country"> <?php $selectedCountry = isset($_POST['country']) ? $_POST['country'] : $row['country']; // get the chosen country foreach($countries as $country): $selected = ($selectedCountry == $country) ? ' selected="selected"' : null; // if the current country equals the chosen country then select it. ?> <option value="<?php echo $country; ?>"<?php echo $selected; ?>><?php echo $country; ?></option> <?php endforeach; ?> </select>
-
Make sure you have typed your query exactly as Zanus posted. With that error it suggestes you havn't typed the query in correctly you have forgotten to type a space before the LIMIT keyword
-
No this will not affect PHP, only http requests to the private folder, eg if you went to site.com/private/ or site.com/private/file.php it'll return a 403 forbidden error.
-
You're right in thinking preg_match is what you need. You can use the following regex to match anything within { and }
~{[a-z0-9_\- ]+}~
Example code
$field = 'my Game'; $text = "{subgame1} {subgame} {{$field}} {subgame12}"; echo $text; preg_match_all('~{[a-z0-9_ -]+}~i', $text, $matches); echo '<pre>' . print_r($matches, true) . '</pre>';
You can then use a foreach loop to run your code for each match
foreach($matches[0] as $gameMatch) { // run code here }
-
Try
<?php class Game { public $draw = array(10,25,36,3,12,13); public $numbers = array(); public $age; public $count; public $draws = array(); function __construct($sub1, $sub2) { $this->name = $sub1; $this->age = $sub2; $this->numbers = range(1, 49); return $this->name;age; } function checkAge() { if ($this->age <18) { return "Sorry $this->name, you are too young to gamble"; } else { return "Hi $this->name welcome to Lucky Lotto Dip, how many draws would you like?"; } } function genDrawLines($lines) { // loop for as many draws required for($i = 0; $i < $lines; $i++) { $this->draws[$i] = array(); $nKeys = array_rand($this->numbers, 6); // pick random numbers foreach($nKeys as $key) $this->draws[$i][] .= $this->numbers[$key]; // add numbers to draw } } function display($sub3) { // generate the numbers for each draw $this->genDrawLines($sub3); echo "<pre>"; echo "*********************"; echo "<br />"; echo "* Lucky Lotto *"; echo "<br />"; echo "*********************"; // loop through the draws foreach($this->draws as $key => $drawNumbers) { $matches = 0; echo "<br />"; echo "* "; for ($j =0; $j <6; $j++) { if ($drawNumbers[$j] < 10) echo " " . $drawNumbers[$j] . " "; else echo $drawNumbers[$j] . " "; // increment matches when the number within the current draw exists within the draw array if (in_array($drawNumbers[$j], $this->draw)) $matches++; } echo "* "; echo "<br />"; echo "*********************"; echo "<br />"; echo "\t\t$matches matches"; // display matchers echo "<br />"; echo "*********************"; } } } $object2 = new Game("Chris", 36); echo $object2->checkAge(); echo $object2->display(4); ?>
-
What version are you using on localhost? If its before 5.0.3 MySQL didn't actually support bit datatypes, instead BIT(1) datatype was an alias of TINYINT(1). This is why on your localhost it is displaying 1 or 0. However on your godaddy server you must be using a newer version of MySQL which has support for Bits. I found this out by reading this artical.
Maybe change the data type for the volunteer, staff, admin and active columns to TINTINT(1) rather than BIT(1).
-
Yes it is possible. Have a go yourself what you're trying to do is pretty basic.
HELP with 9 lines of code
in PHP Coding Help
Posted
Is this your code for logging in a user?
If it is that is not the correct way to go about logging in users.
You should instead be performing a query like
You should then check to see if the query returned a match (mysql_num_rows). If it returned a match log the user in. If no match was returned the user entered invalid login credentials.