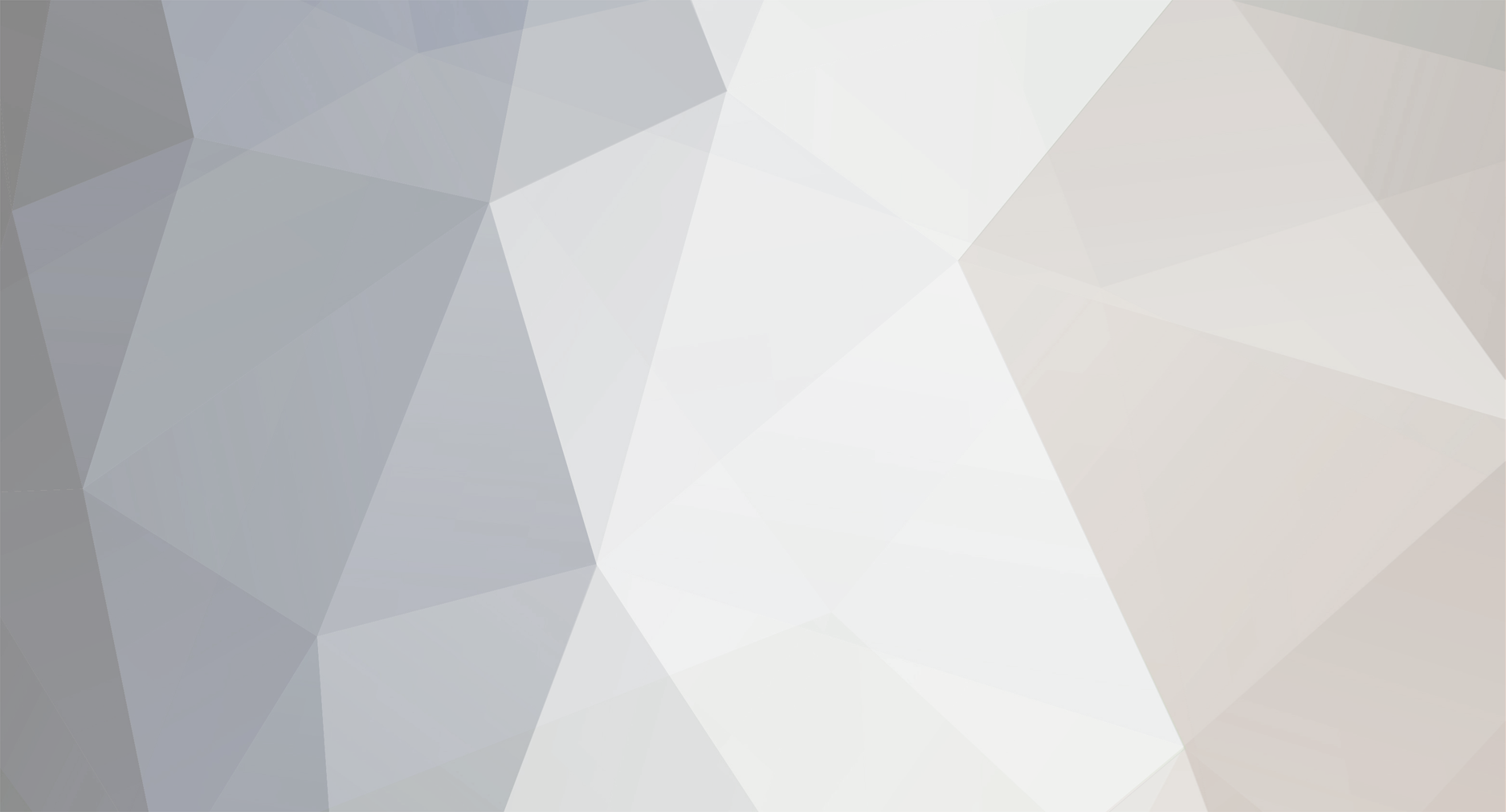
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
OK, I have modified your two functions: Before using my code make sure you backup your orignials. All code below is untested so it may work or it may not. function display_cart($cart, $change = true, $images = 1) { //Basic Flow: /* 1:loop through each iteam and pass the product number of each item to get_book_details() so that we can summerize the info of each book. 2:provide an image for each book if one exits 3:if we call function with the change parameter set to true (or not it defaults to true), show box with the quantities in them as a form with the save changes button at the end. */ // display items in shopping cart // optionally allow changes (true or false) // optionally include images (1 - yes, 0 - no) echo ' <form action="show_cart.php" method="post> <table border="1" bordercolor="#000000" width="100%" cellspacing="0"> <tr> <th colspan="' . $images+1 . '" bgcolor="#cccccc">Item</th> <th bgcolor="#cccccc">Price</th> <th bgcolor="#cccccc" align="left">Quantity</th> <th bgcolor="#cccccc" align="left">Shoesize</th> <th bgcolor="#cccccc">Total</th> </tr>'; //display each item as a table row foreach ($cart as $prdnumber => $prddetails) { extract($prddetails); $products = get_book_details($prdnumber); echo " <tr>\n"; if($images == true) { echo ' <td align="left" width="5%">'; if (file_exists("images/$prdnumber.jpg")) { $size = GetImageSize('images/'.$prdnumber.'.jpg'); if($size[0] > 0 && $size[1] > 0) { echo '<img src="images/'.$prdnumber.'.jpg" border=0 '; echo 'width = '. $size[0]/3 .' height = ' .$size[1]/3 . '>'; } } echo "</td>\n "; } echo '<td align"left">'.$products['title']."</a></td>\n "; echo '<td align="left">'.number_format($products['price'], 2)."</td>\n "; echo '<td align="left">'; // if we allow changes, quantities are in text boxes if ($change == true) echo "<input type=\"text\" name=\"{$prdnumber}[qty]\" value=\"$qty\" size=\"3\" align=\"left\">"; else echo $qty; echo "</td>\n "; // if we allow changes, quantities are in text boxes if ($change == true) { echo '<td align="left"> <select name="' . $prdnumber . "[shoesize]\"> <optgroup label=\"sizes\">\n"; $shoe_sizes = range(40, 46); $selected = null; foreach($shoe_sizes as $shoe_size) { if($_SESSION['cart'][$prdnumber]['shoesize'] == $shoe_size) { $selected = ' selected="selected"'; } echo ' <option value="' . $shoe_size . '"' . $selected . '>' . $shoe_size . "</option>\n"; } echo ' </optgroup> </select> </td> <td align="left">'.number_format($products['price']*$qty,2)."</td>\n </tr>\n "; } } // display total row echo '<tr> <th colspan="'. (2+$images) .'" bgcolor="#cccccc"> <th align="left" bgcolor="#cccccc">' . $_SESSION['items'] . '</th> <th align="left" bgcolor="#cccccc"> </th> <th align="left" bgcolor="#cccccc">' . number_format($_SESSION['total_price'], 2) . "</th> </tr>\n "; // display save change button if($change == true) { echo '<tr> <td colspan="'. (2+$images) .'"></td> <td align="center"> <input type="hidden" name="save" value="true"> <input type="image" src="images/save-changes.gif" border="0" alt="Save Changes"> </td> <td> </td> </tr>'; } echo "\n </table>\n</form>"; } // if a user has come to show_cart.php page by clicking an Add to Cart button add the item tot he cart, //if user did not have a cart, creat one, an empty one if(isset($_GET['new']) && is_numeric($_GET['new'])) { $prd_id = $_GET['new']; //second, after we know that cart is set-up we can add the item to it if(!isset($_SESSION['cart'])) { $_SESSION['cart'] = array(); $_SESSION['items'] = 0; $_SESSION['total_price'] = 0.00; } //check whether the item is already in the cart, if it is increment the quanity of that item by one. if(isset($_SESSION['cart'][$prd_id])) { $_SESSION['cart'][$prd_id]['qty']++; } else { //if not, you add the new item to the cart $_SESSION['cart'][$prd_id] = 1; } //work out the total price and number of items in the cart. for this we use function //calculate_price() and calculate_iem() $_SESSION['total_price'] = calculate_price($_SESSION['cart']); $_SESSION['items'] = calculate_items($_SESSION['cart']); } //user has come to show cart via save changes button. in this case user has come via a //form submission. if the hidden variable on the form is set we know that the user //has come from the save chnages button. this also means that the user has edited the //quanity values in the cart and we need to update them. if(isset($_POST['save'])) { //for each prdnumber in the cart you check the post variable with that name foreach ($_SESSION['cart'] as $prdnumber => $product_details) { extract($product_details); //if any of the variables are set to zero, then remove all ietms from the cart if($_POST[$prdnumber] == '0') { unset($_SESSION['cart'][$prdnumber]); } else { //otherswise update the cart to match the form fields. $_SESSION['cart'][$prdnumber]['qty'] = $_POST[$prdnumber]['qty']; $_SESSION['cart'][$prdnumber]['shoesize'] = $_POST[$prdnumber]['shoesize']; } } //after that we again use the calculate_price() and calculate_items() to work //out the new values of the total_price and item sessions variables. $_SESSION['total_price'] = calculate_price($_SESSION['cart']); $_SESSION['items'] = calculate_items($_SESSION['cart']); } do_html_header('Your shopping cart'); //no matter which page the user has come from display the content of the cart //if cart has content call display_cart() if($_SESSION['cart'] && array_count_values($_SESSION['cart'])) { display_cart($_SESSION['cart']); } else { echo '<p>There are no items in your cart</p><hr />'; }
-
The way you are handling your sessions when saving products to the cart is not adaptable enough to easily integrate the shoe size into the session. The cart session handling is going to have to be modified or prehaps redone. You are going to have to have a session like the following $_SESSION['cart']['PRODUCT_ID_NUMBER_HERE']['qty'] $_SESSION['cart']['PRODUCT_ID_NUMBER_HERE']['shoesize'] Currently it is just: $_SESSION['cart']['PRODUCT_ID_NUMBER_HERE] which just holds the quantity for the ordered item. In order to save the shoesize to the session you are going to have to change $_SESSION['cart']['PRODUCT_ID_NUMBER_HERE] from holding just the quantity to holding both the shoesize and the quantity. In order to do that $_SESSION['cart']['PRODUCT_ID_NUMBER_HERE] is going to have to store an array. In doing that it'll break your session handling. Due to how your script process the $_SESSION['cart']['PRODUCT_ID_NUMBER_HERE] variable. From looking at your script I think I understand how it works. I'll provide you will the modifications in abit.
-
This block of code: <select name=\"$shoesize\"> <option >--</option> <option name=41 value = \"41\">41</option> <option name=42 value = \"42\">42</option> <option name=43 value = \"43\">43</option> <option name=44 value = \"44\">44</option> <option name=45 value = \"45\">45</option> <option name=46 value = \"46\">46</option> </select></td>"; should be: <select name=\"shoesize\"> <option >--</option> <option value=\"41\">41</option> <option value=\"42\">42</option> <option value=\"43\">43</option> <option value=\"44\">44</option> <option value=\"45\">45</option> <option value=\"46\">46</option> </select></td>"; You should define the name for the pull down menu within the select tag and not for the individual options. You'd then use $_POST['shoesize'] to get the selected size the customer selected from the list. Also I noticed you are using the old superglobal variables $HTTP_*_VARS. These variables are now depreciated and have been replaced with the new shorter versions, eg: $HTTP_POST_VARS should be $_POST
-
help needed with changing td background with css and php
wildteen88 replied to spindriftmed's topic in PHP Coding Help
bankground-image should be formatted like so: background-image: url(path/to/image); /* NOT - background-image: "path/to/image"; */ -
What do you mean by date cookie? I am not sure what you are asking.
-
What function to use to get the first day of the month ?
wildteen88 replied to jd2007's topic in PHP Coding Help
date and strtotime, eg: // date for the 1st of december 2007 $date = '01-12-2007'; // convert date into a timstamp $date_timestamp = strtotime($date); // get the day $day = date('l', $date_timestamp); echo $day; -
To install phpBB you just run the install/index.php script by going to http://localhost/phpBB/install/index.php you then run through the on screen instructions. phpBB provides a few video tutorial of installing/setting up phpbb here
-
Oh please... will you stop posting code suggestions. Its not do with the code. Its to do with the SQL query being used. All is explained in Barand's post above.
-
0 just mean no margin for the left and right. Note: margin: 1em 0; means apply a margin of 1em to the top and bottom and zero for the left and right. When you specify a zero for a value you don't have to provide a unit. You only set a unit for a size when you set a value greater than zero.
-
When PHP shows a notice: undefined index. It means the that that the index (the index is defined within the [] brackets) you have defined does not exist. What you should do is check if it exists first before using the index. To check whether it is exists refer to thorpe's code. Always check to see if _GET, _POST and _COOKIE variables exists first before using them.
-
What version of PHP5 do you have? The allow_url_include setting was added in version 5.2.x If you have a version lower than 5.2.x then you'll want to enabled allow_url_fopen instead.
-
EDIT: Ohh, beatin to it Change this line: <frameset rows="25<?php if ($fetch->bar == "1"){ ?>,*<? } ?>" cols="*" framespacing="0" frameborder="NO" border="0"> to: <frameset rows="25<?php if ($fetch->bar == "1"){ echo ',*'; } ?>" cols="*" framespacing="0" frameborder="NO" border="0">
-
For preventing SQL Injections search that term in google. You'll get many results which explain what SQL Injection is and how to protect against it. Have a read of the following three part article for writing secure PHP code: Part 1, Part 2 and Part 3
-
By default PHP disables url based include paths. To use url based include paths you'll have to enable the allow_url_include setting, by changing the default Off value to On. Note make sure you restart your server (Apache, IIS etc) for the changes you made to the php.ini to come into affect
-
Where is Online or Offline defined? I am not using WAMP so I don't know what you're referring to, however I do have used wamp before. I just prefer manuall install of Apache, PHP and MySQL myself. For now I guess Offline should do.
-
looks like you are replacing the smilie code ( eg: :P) when you save the submitted text into the text file, or however you store it. What you should do is process the smilie code when you go to display the data stored on your page instead. That way when you go to edit the text later on it'll still be in its rawest form (no fomatting, basic text).
-
try defining the regex within your filter array variable instead: <?php $filter = array( '/onmouseout/i', '/onmouseover/i', '/onclick/i', '/ondblclick/i', '/onkeydown/i', '/onkeypress/i', '/onkeyup/i', '/onmousemove/i', '/onmouseup/i', '/onmousedown/i'); $replace = ""; $string = "I like using onmOUseDown"; echo preg_replace($filter, $replace, $string); ?> When you do this: "/$filter/i" You are passing the following to the preg_replace: "/Array/i" $filter is an array, but you surround $filter within quotes and pad '/' and '/i' around the variable. Now you cannot join arrays to strings and so PHP cannot do the replacements you are asking it. If you define the regex within the filter array. PHP will process the array and do the replacements. As PHP will recognise that you have passed an array and so will loop through the array passing each item from the array into preg_replace. EDIT Hold on... I've gone mad . Why not just do the following instead: <?php $filters = array( 'onmouseout', 'onmouseover', 'onclick', 'ondblclick', 'onkeydown', 'onkeypress', 'onkeyup', 'onmousemove', 'onmouseup', 'onmousedown' ); $replace = null; $new_string = null; $string = 'I like using onmOUseDown onKeyup'; // loop through the filters. foreach($filters as $filter) { $string = preg_replace("/$filter/i", $replace, $string); } echo $string; ?>
-
There will be no risks if you are installing for testing/development purposes when creating your PHP scripts. Unless you configure your router (if you have one) ans/or firewall to allow connections via the port Apache runs on (80 is the default).
-
[SOLVED] SESSION Variable Changed For No Reason
wildteen88 replied to GDollar_Post's topic in Apache HTTP Server
Make sure you don't have register_globals turned on. Register_globals always you to use $id aswell as $_SESSION['id'] for accessing the session variable, or any other server set variables with an 'id' key. To turn register_globals off it is best to add the following at the top of your scripts: ini_set('register_globals', 'Off'); Or if you host always you change PHP settings within an .htaccess file you can add the following to an .htaccess file php_flag register_globals Off Note: It is best place an .htaccess file in you sites root folder, that way register_globals will be off through your entire website. Note: Register_globals can cause exploits within your code, as you have just discovered. This is reason why PHP now has register_globals disabled by default and is being phased out. -
winMysqladmin is part of the mysql install, it should get deleted when mysql is uninstalled. When uninstalling MySQL it is best to make sure mysql is not running, if you uninstall mysql whilst it is running it may not uninstall correctly, such as some files, processes or services may be left. If there are any files left, just delete them. Deleting left over files should not affect your computer.
-
php.ini file being ignored
wildteen88 replied to holdris's topic in PHP Installation and Configuration
You have added this line to the httpd.conf: PHPIniDir "./" the ./ means from the current working directory (where the httpd.conf file is being read). Change phpinidir to: PHPIniDir "C:/php" Move php.ini to C:/php. Save httpd.conf and restart Apache. DO not move files around the filesystem. Moving files around can cause more problems. -
trim only clears whitespace at the begining and end of the string. it does not clear whitespace between characters
-
yet ANOTHER Syntax Error . . . unexpected $end
wildteen88 replied to Styles2304's topic in PHP Coding Help
That code seems to be fine. I get no errors. Prehaps the error is coming from another file, prehaps one of the includes -
Whats not working? Need to post more information, like what's your script supposed to do, what's is it doing now. Post any errors you are getting etc.
-
Should work fine no matter what OS you use. try a simple test on linux and windows with this: <?php session_start(); if(isset($_GET['n']) && $_GET['n'] == '1') { echo '<pre>' . print_r($_SESSION, true) . '</pre>'; } else { $_SESSION['x']['y']['z'] = "aaa"; echo '<a href="?n=1">Next</a>'; } ?>