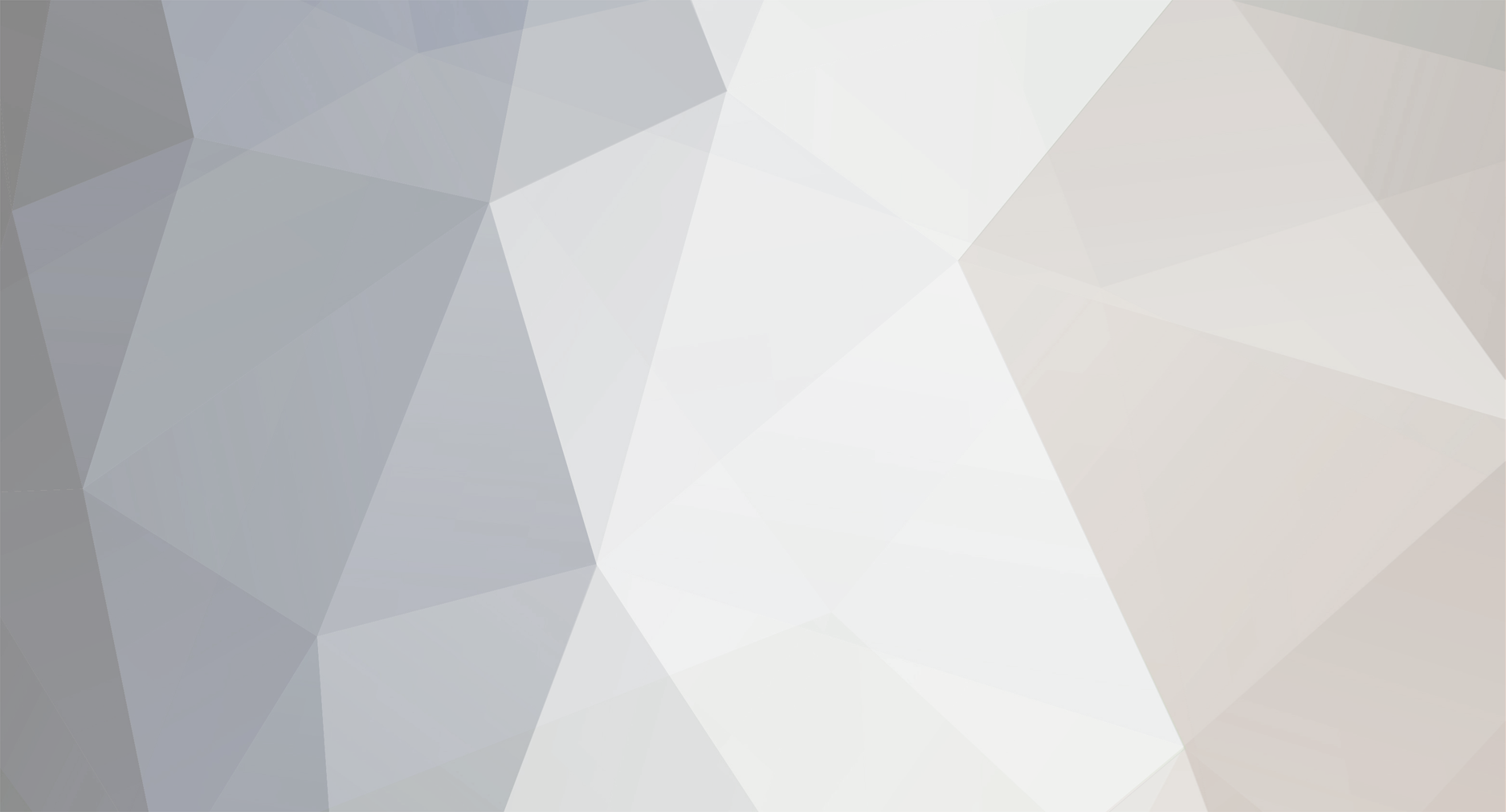
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
Add all possible checkbox values into an array
$checkbox_values = array('Stage 1', 'Stage 2', 'Stage 3', 'Stage 4');
Next get all the db values into an array
$res=mysql_query("SELECT stage FROM ACTIVE WHERE INDEX_ID=$id"); if(mysql_num_rows($res)==0) echo "There is no data in the table <br /> <br />"; else { while($row=mysql_fetch_assoc($res)) $db_values[] = $row['stage']; // add value into array }
No that we have the necessary data we can generate the checkboxes.
foreach($checkbox_values as $cbox_value) { $selected = if(in_array($cbox_value, $db_values)) ? ' checked="checked"' : null; echo '<input type="radio" name="stage" value="' . $cbox_value . '"' . $selected . ' />' . $cbox_value . '<br />'; }
-
I'm reading through this book which is actually not bad but, on page 286 there's a shopping_cart.php code (which I downloaded from the web site). The code that seems relevant to me is this:
<?php
$totalPrice = 0;
foreach ( $_SESSION["cart"] as $product) {
$totalPrice += $product->getPrice(); //<<<LINE 94
?>
The error I get is
Fatal error: Call to a member function getPrice() on a non-object in C:\usr\web\shopping_cart.php on line 94
Should $product be a cast to the Product object?
Yes as long as you have initiated the object
$product = new Product;
-
Not sure really. It is reporting it has found over 9000 mp3 files. Do you have that many mp3's?
-
Your issue is to do with this line
$row = mysqli_fetch_array($result);
Remove that line and the first question should now be displayed. Each time mysqli_fetch_assoc is called the next row in the result set will be returned.
-
Change this
echo" <td nowrap width='75'> <a href= ".$image_id1."> <img src= '".$image_id1."' width='50' height='50' border='0'/>";
to
if(!empty($image_id1)) echo" <td nowrap width='75'> <a href= ".$image_id1."> <img src= '".$image_id1."' width='50' height='50' border='0'/>"; else echo " <td width='75'></td>";
You'd do the same thing for the $image_id2 variable
-
this is the line showing error
echo "<form method=post action=$_SERVER['PHP_SELF']>";
Did you read Pikachu2000's reply earlier
-
I modified an existing directory size script and came up with this
<?php function sizeFormat($size) { if($size < 1024) { return $size." bytes"; } else if($size<(1024*1024)) { $size=round($size/1024,1); return $size." KB"; } else if($size<(1024*1024*1024)) { $size=round($size/(1024*1024),1); return $size." MB"; } else { $size=round($size/(1024*1024*1024),1); return $size." GB"; } } function getFileSizeByExtension($path, $extensions=array()) { $ignore = array('.', '..', '.htaccess'); foreach($extensions as $ext) { $totalsize[$ext]['size'] = 0; // file size in bytes $totalsize[$ext]['count'] = 0; // file counter } if ($handle = opendir ($path)) { while (false !== ($file = readdir($handle))) { $nextpath = $path . '/' . $file; if (!in_array($file, $ignore)) { $nextpath_ext = pathinfo($nextpath, PATHINFO_EXTENSION); if (is_dir ($nextpath)) { $result = getFileSizeByExtension($nextpath, $extensions); foreach($result as $ext => $data) { $totalsize[$ext]['size'] += $data['size']; $totalsize[$ext]['count'] += $data['count']; } } elseif (is_file ($nextpath) && in_array($nextpath_ext, $extensions)) { $totalsize[$nextpath_ext]['size'] += filesize ($nextpath); $totalsize[$nextpath_ext]['count']++; } } } } closedir ($handle); return $totalsize; } $search_path = $_SERVER['DOCUMENT_ROOT']; $search_extensions = array('php', 'jpg', 'mp3'); $filesizes = getFileSizeByExtension($search_path, $search_extensions); foreach($filesizes as $ext => $data) echo $data['count'] . ' ' . strtoupper($ext) . ' files used ' . sizeFormat($data['size']) .' of disk space<br />'; ?>
-
Yes that should work. Just make sure you have populated the $__TEXT__ array for all your field names.
-
EDIT: Oh, I could of just used array_diff_key instead
$array[0] = "asd"; $array[1] = "ter"; $array[2] = "asd"; $array[3] = "asd"; $array[4] = "xfh"; $unique_values = array_unique($array); $duplicate_values = array_diff_key($array, $unique_values); echo '<pre>' . print_r($duplicate_values, true) . '</pre>';
Original post
I came up with
$array[0] = "asd"; $array[1] = "ter"; $array[2] = "asd"; $array[3] = "asd"; $array[4] = "xfh"; $unique_values = array_unique($array); $duplicate_values = array(); foreach($array as $key => $value) { if(!array_key_exists($key, $unique_values)) $duplicate_values[] = $key; } echo '<pre>' . print_r($duplicate_values, true) . '</pre>';
-
Have you tried PaulRyan or Pikachu2000's suggestions?
-
My code selects the rows that correspond to the numbers within your $_POST['benifits'] array. So if you select the checkboxes that have the value of 1, 3 and 6. It'll return the records that have a b_id value of 1, 3 and 6 from your database.
-
__FILE__ returns an absolute filesystem path (eg /home/website/public/filename.php). That should never be used as the form action!
-
Does the numbers 1, 3, 9, 6 correspond to a value in your b_id field? If they do then you can do this.
I assume $_POST['benifits'] is an array of the ids.
$IDs = implode(',', $_POST['benifits']); $query = "SELECT * FROM benefits WHERE b_id IN ($IDs)"; $result = mysql_query($query); if(mysql_num_rows($result)) { while($row = mysql_fetch_assoc($result)) { // do what you want with the results. } }
That will return all records with the b_id value of 1, 3, 9 and 6
-
Wrap your associative arrays within curly braces {}, eg
<td width=\"87\">{$rows['ref']}</td>
Or an alternative solution would be to use concatenation operator, eg
<td width=\"87\">". $rows['ref'] . "</td>
-
i thought mysql_real_escape_string() would backslash all the quotation marks, just a work around... sorry.
It escapes harmful characters to prevent SQL injection attacks within an SQL query. There is no point in using that function if the data is not going to a database.
-
Then in this php file you would need to fetch the selected options from the combobox which will be in the following variable
$_POST['form1']['frmhowhear'];
The form name is not sent in the $_POST array.
The correct variable will be $_POST['frmhowhear'] to grab the selected value.
-
-
You're on the right path. Change these lines
$title=$parse[0]; $header=$parse[1]; $key=????
to
$title = array_shift($parse); $header = array_shift($parse); // $parse will now only contain the keys. // grab a random key $key = $parse[ array_rand($parse) ];
array_shift removes the first item from the array. So when it is first called it'll remove the title from the $parse array and assign it to the $title variable, the same applies for the header. You're now left with an array of keys. To grab a random key you can use array_rand.
-
You can use file to read each line into an array. Then use array_unique to grab all unique values. Lastly implode the array back into a string to be written back to the file.
-
Where in script1 are you setting the $rtn variable
-
Apache is reading your .htaccess file so there isn't any issue with the configuration. What are trying to test which is not working for you?
-
Post your actual code here. The source of the error is due to whatever code you have before those two lines. They are perfectly fine.
-
mysql_real_escape_string($_POST[''])
might do the trick.
Why would the OP need to use that function if they are not using a database!
@darrelf If you echo $comments variable just before you send the email does it show the message in full?
$comments = $_POST['comments']; // preview comments echo $comments;
-
What is $Path set to? Why don't you just echo it out to see what it is set to. The path it shows should be where your images are located. Do you know where the images should be?
houston I've got a problem ( hum what's new here )
in PHP Coding Help
Posted
Your not setting any values for the last four drop down menus. You're only setting the Label. The Label is never treated as the value.