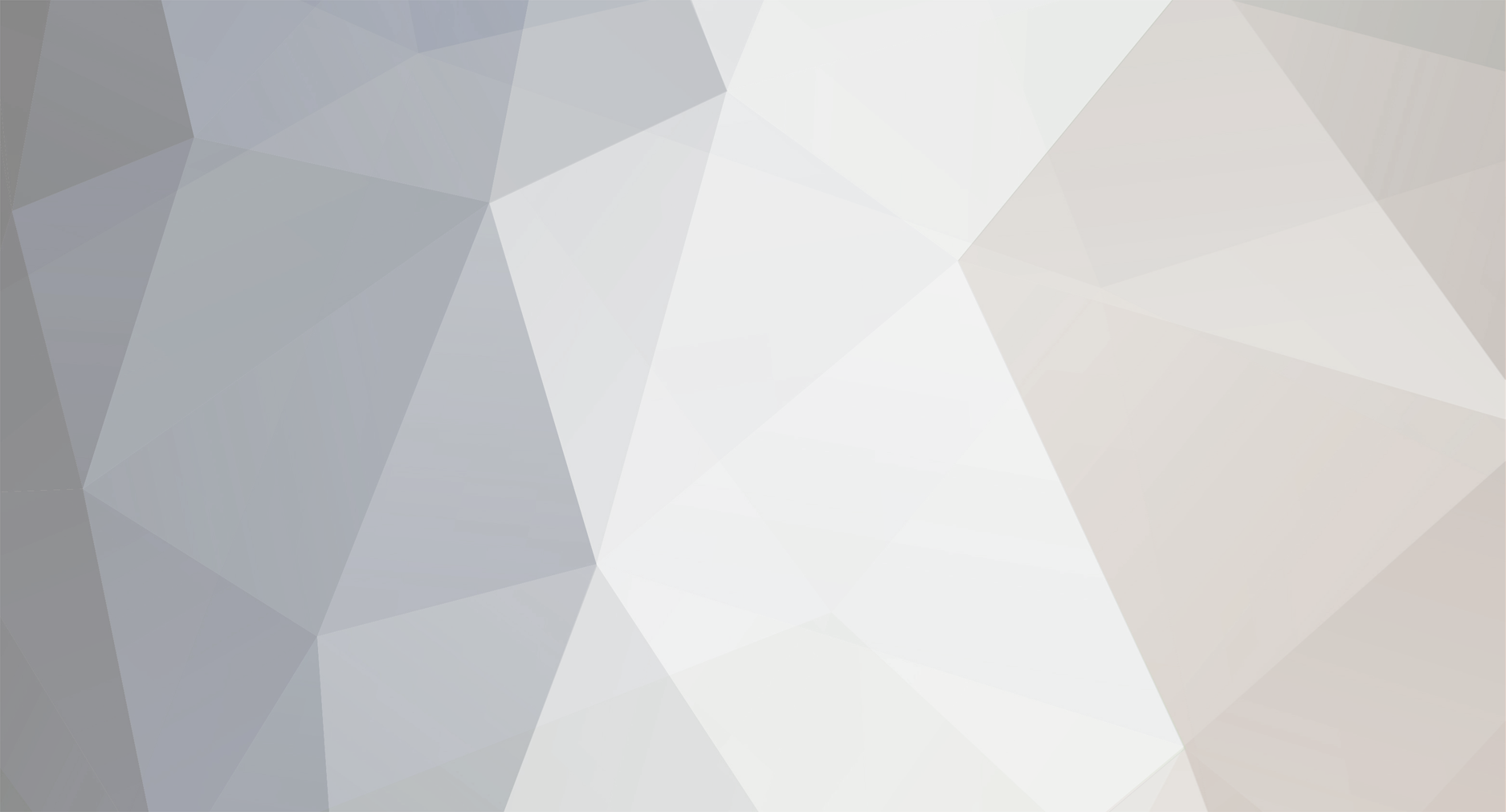
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
Your query is constructed incorrectly
$query = mysql_query("SELECT * FROM final WHERE name=$username");
$username needs to be wrapped in single quotes.
-
while($result == mysql_fetch_array($query)) {
You should be using the assignment operator (=) there.
-
Looks like the connection to mysql is failing for some reason, as $db is not passing a valid link resource. This will happen if mysql_connect does not connect to mysql properly. You should check to see if there is a connection. Change contentdb.php to
<? include("config.php"); $db = mysql_connect("$hostname", "$user", "$pass"); if(!$db) { trigger_error('Connection to mysql failed. ' . mysql_error(), E_USER_ERROR); } mysql_select_db("$database",$db); ?>
-
Do you want it to also list files within subfolders too, for example
file1
file2
directory
(indent)file1
(indent)file2
(indent)file3
(indent)... etc
(indent)sub-directory
(indent)(indent)file1
(indent)(indent)file2
(indent)(indent)file3
(indent)(indent)... etc
file4
file5
...etc
If you do then you'll need to make a recursive function.
-
$_SERVER["QUERY_STRING"]; is a PHP variable which returns the query string in the url.
-
You'll first need to find all the <code></code> blocks with preg_match_all using regex. Then use htmlentities to display the code as readable text
-
The second parameter for mysql_query should be a valid mysql link resource. Where is the variable $db being defined?
Note a mysql link resource is only returned from mysql_connect() when a connection to the mysql server has been established.
-
So you want to get the keys for each instance of asd within your array. You can use array_keys for this
$duplicate_values = array_keys($array, 'asd'); echo '<pre>' . print_r($duplicate_values, true) . '</pre>';
-
Here is an example script I was going to post last night but my internet was not working.
<?php session_start(); $pages = array('page1', 'page2', 'page3', 'page4'); // add pages into this array $offers = array('offer1', 'offer2'); // add offers into this array ?> <a href="<?php echo basename(__FILE__) ?>?reset">Reset</a> | <a href="<?php echo basename(__FILE__) ?>">Next</a> <hr /> <?php if(isset($_GET['reset'])) { $_SESSION['shownOffers'] = array(); $_SESSION['shownPages'] = array(); $_SESSION['currentPage'] = null; } function getPage($pages) { if(isset($_SESSION['currentPage']) && $_SESSION['currentPage'] == end($pages)) { $_SESSION['shownPages'] = array(); $_SESSION['currentPage'] = null; } foreach($pages as $key => $page) { if(!in_array($page, $_SESSION['shownPages'])) { $_SESSION['shownPages'][] = $page; $_SESSION['currentPage'] = $page; return $page; } } } function getOffer($offers) { if(isset($_SESSION['currentOffer']) && $_SESSION['currentOffer'] == end($offers)) { $_SESSION['shownOffers'] = array(); $_SESSION['currentOffer'] = null; } foreach($offers as $key => $offer) { if(!in_array($offer, $_SESSION['shownOffers'])) { $_SESSION['shownOffers'][] = $offer; $_SESSION['currentOffer'] = $offer; return $offer; } } } $currentPage = getPage($pages); $currentOffer = getOffer($offers); ?> <h1><?php echo $currentPage; ?></h1> <h2><?php echo $currentOffer; ?></h2> <?php echo '<pre>' . print_r($_SESSION, true) . '</pre>'; ?>
-
Check the contents of the $db_year array. Add the following after the while loop:
echo "<pre>".print_r( $db_year, true)."</pre>";
Post the output here.
I have tested the code by setting $db_year with hard coded values, eg add this after your while loop.
$db_year = array('2001', '2006', '2012');
And the script does select the correct checkboxes. Maybe the $db_year is not being populated correctly?
Also one problem you'll find when you submit your form is only the last checkbox value will be submitted. This is due to how the checkboxs are named
echo '<input type="checkbox" name="year"....
Adding [] after the name will allow for all selected checkbox values to be submitted. If you do not add the square brackets at the end of the name only the last selected checkbox value will be submitted.
-
Change
$num = count($array); $random = rand(0, $num); $file = $array[$random]; include "modules/did-you-know/$file";
to
$file = $array[ array_rand($array) ]; $chosen_file = "modules/did-you-know/$file"; if(is_file($chosen_file)) include $chosen_file; else echo "$chosen_file does not exits";
-
You could use sessions instead. Rather than writing $getcurrent to your lprotation and offerrotation text files.
Also you could store all your rotation pages and offeres within a database. That way you don't need to keep defining your pages/offers each time they change. You can then completely automate the rotation with a loop or to.
-
This line
$result[] = array_unique($count_begin);
Will make $result a multidimensional array, as array_unqiue returns an array of unique values from the $count_begin array . Remove the [] after $result.
-
I assume you're outputting the array with print_r().
$result[$i] prints
Array ( [0] => 2011-05-01_right [1] => 2011-05-02_left [3] => 2011-05-02_right )
To get that output are you passing $result[$i] to print_r() or just $result?
-
The second page with is called from the form does write to the browser, so it does set the cookies. I just gave a revised php section at the top of that page.
It will set the cookie. But you wont be able to retrieve the cookie immediately after you call setcookie(). That is what I trying to say. Try it yourself
<?php setcookie('test', 'blah...', time()+60); echo "\$_COOKIE['test'] = " . $_COOKIE['test']; ?>
When first run that code it will just display the text
$_COOKIE['test'] =However when you reload the page it'll show
$_COOKIE['test'] = blah.. -
From the example she gave in post #1 it's the text between the <h1> tags she's looking for.
Which is what my code does. It grabs the text between the <h1> and </h1> for each heading it finds.
For example, if $homepage is set to
<h1>one</h1> text blah <h1>test123</h1> boob
Then the outut of my script will be
Array ( [0] => one [1] => test123 )
-
Oh, hangon. I see what it is doing now. Sorry about that.
It is because dir() is returning . and .. into your array. These periods have special meanings in file paths. You need to filter the results that dir() returns. Change the while loop to
while($file=$myDirectory->read()) { if($file != '.' && $file != '..') { $array[$i]=$file; $i++; } }
-
It is is because $result[$i] returns an array, Explode requires a string as the second parameter not an array.
-
It helps if you stop censoring your error messages. What file path does it error out on?
-
When setting cookies, the value of the cookies will not become available until you refresh the page. So you will not be able to immediately retrieve the cookie once you have set it. However you will when you reload page.
-
You need to add modules/did-you-know to your include path
$file = $array[$random]; include "modules/did-you-know/$file";
-
Your query is failing because the username you're searching for doesn't exist. What is $_SERVER['MM_Username']? Should that not be $_SESSION['MM_Username']?
-
$done should be an array. in_array() expects an array to passed to it as the second parameter.
-
You could go the regex approach which is alot more cleaner
$homepage = file_get_contents("./".$id.".php"); preg_match_all('/<h1>(.*?)<\/h1>/i', $homepage, $matches); echo "<pre>".print_r($matches[1], true)."</pre>";
$matches[1] will return an array of h1 headings it finds (without the <h1> and </h1> tags).
How to get one dimensional array and not multidimensional array
in PHP Coding Help
Posted
These line are causing your array to be multidimensional
You may want to use array_merge here. Rather than assign the result of tree_gather() to your $child array. As you have it at the mement you will always get a multidimensional array.