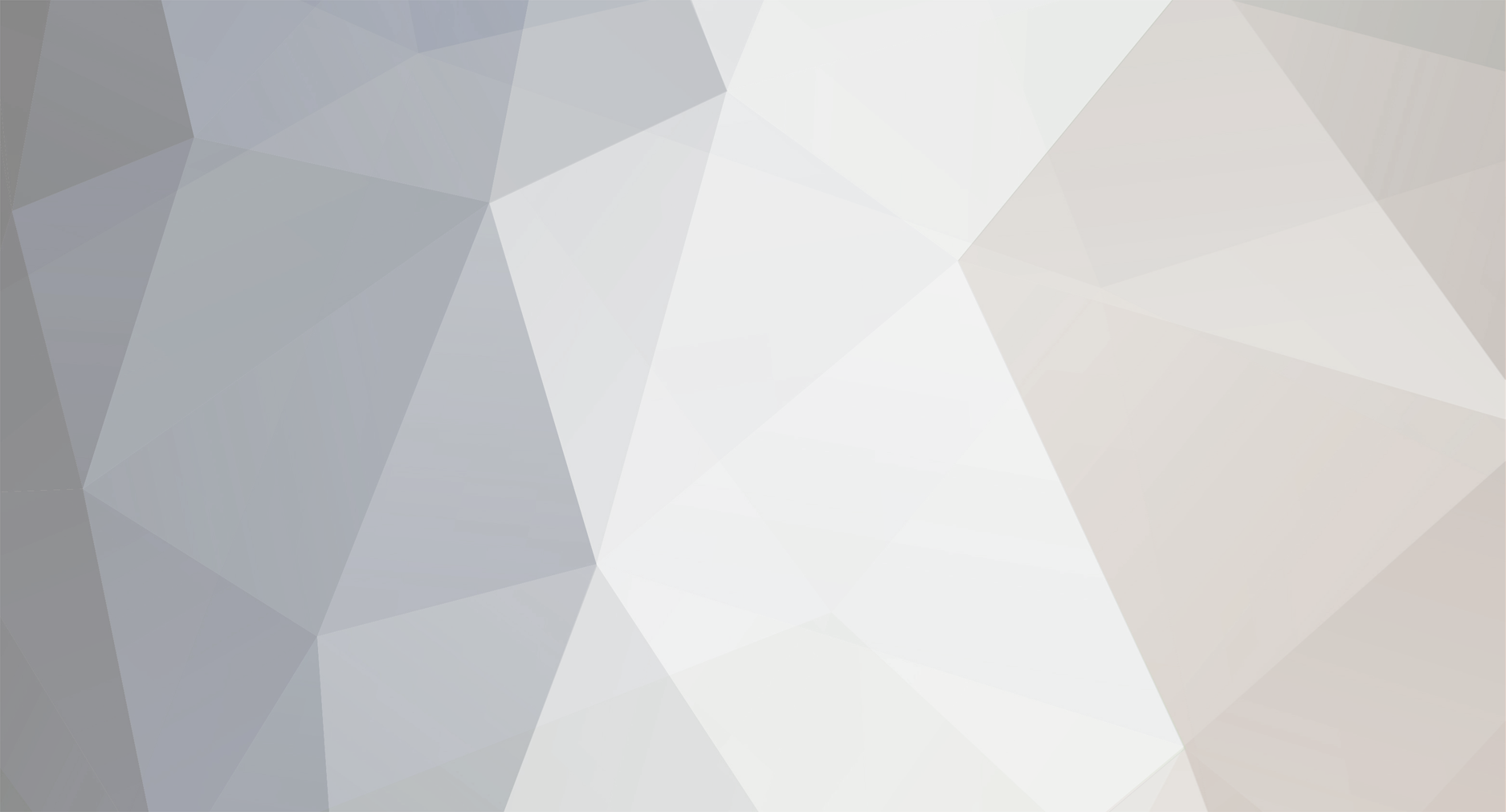
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
there is also an error where I put the comment......
It'll be helpful if you posted the actual error message.
-
Your query is only returning the eventid column. You need to amend the query so it returns the shutoff column aswell.
$sql = "SELECT shutoff, eventid FROM Registration WHERE eventid=".$_GET['eventid']." LIMIT 1";
-
$product->$getName() should be $product->getName()
-
-
An if/else statement is constructed like so
if( condition ) { // do something when condition is true } else { // do something when condition is false }
What are you trying to do?
-
You don't need to call mysql_select_db($database_swb, $swb); every time you run a query. I assume this is code that Dreamweaver has generated. You should really learn to hand code your script rather than relying on dreamweaver.
-
Killing the script for each instance where the user hasn't filled in the form correctly is bad application design.
You should instead add your error messages into an array when your validation for whaterver field fails, example for the Name field
if($Name == '') { $errors[] = '<P class="form">Name field left empty</p>'; }
When you have finished validating the user input you can check whether any errors have accrued. if there are errors then display them, along with the form. If there are no errors then display your success message.
if(!empty($errors)) { echo 'Sorry correct the following errors: <ul><li>' . implode('</li><li>', $errors); // display your form here } else { echo("<P class=\"passed\">Thankyou for submiting your details, you will be added to our directory shortly</p>"); }
-
Change the foreach loop to
foreach($checkbox_values as $cbox_value) { $selected = null; $cbox_label = $cbox_value; if(in_array($cbox_value, $db_values)) { $selected = ' checked="checked"'; $cbox_label = "<b>$cbox_value</b>"; } echo '<input type="radio" name="stage" value="' . $cbox_value . '"' . $selected . ' />' . $cbox_label . '<br />'; }
-
You code sets $reg to the string " AND status=0 "; if you select Unpaid from the drop down box. And it is inserting this value into your query correctly.
Could you explain what you mean by unexpected result. The problem is not so much to do with your PHP code, but your SQL query. Although placing raw user input into query is not recommended for security reasons, you should be validating/sanitizing your user input.
-
You need to check if the fields are not empty within the for loop when building the query.
if(!empty($store[$i]) && !empty($item[$i]) && !empty($itemprice[$i])) $values[$i] = "( '{$id[$i]}', '{$store[$i]}', '{$item[$i]}', '{$itemprice[$i]}', '{$itemnumber[$i]}', '{$couponvalue[$i]}', '{$couponsused[$i]}')"; // build the array of values for the query string
That if statement will check to make sure the fields store, item, and price have not been left empty. It'll only add the rows that have these three fields filled out.
-
Add an if statement which checks to see if $_GET['id'] isset. If it does then set $id to the value of $_GET['id']. Otherwise set $id to 1
The above translated into PHP code
$id = isset($_GET['id']) ? $_GET['id'] : 1;
Note the ?: syntax is called a ternary operator (an in-line if/else statement)
-
Are you using the latest version of MyUPD? I have just downloaded and installed the latest version from the sourceforge link your provided and no errors have occurred whilst installing/using the forum software.
-
This is because you need to check if these variables are set before using them
if(isset($_GET['username']) && isset($_GET['pwd'])) { // your code here for authenticating the user } else { // display login form here }
having the username/password within the url is insecure. You should be submitting your form with the post method.
-
Because PHP does not pass on variables page to page. You can save the posted data into a session. So when you submit the first form to your second form save the posted data into a session, eg
$_SESSION['step1_data'] = $_POST;
After you have saved the posted data. Display your next form. On your final page you can work with the data you have collected. Here is an example
step1.php
<form action="step2.php" method="post"> <p> Loan Type: <select name="loanType"> <option>Loan Type 1</option> <option>Loan Type 2</option> <option>Loan Type 3</option> </select> </p> <p> Property Type: <select name="peopertyType"> <option>Property Type 1</option> <option>Property Type 2</option> <option>Property Type 3</option> </select> </p> <p> Property State: <select name="peopertyState"> <option>Property State 1</option> <option>Property State 2</option> <option>Property State 3</option> </select> </p> <p><input type="submit" name="step1_submit" value="Continue →" /></p> </form>
step2.php
<?php session_start(); if(isset($_POST['step1_submit'])) { $_SESSION['step1_data'] = $_POST; /* add all data from step1 to session variable */ ?> <form action="step3.php" method="post"> <p> Credit Score: <select name="creditScore"> <option>500 - 600</option> <option>600 - 700</option> <option>800 - 900</option> </select> </p> <p> Phone Number: <input type="text" name="phoneNumber" /> </p> <p> Email Address: <input type="text" name="emailAddress" /> </p> <p><input type="submit" name="step2_submit" value="Continue →" /></p> </form> <?php } else { ?> Please fill in <a href="step1.php">Step 1</a> <?php }; ?>
step3.php
<?php session_start(); if(isset($_POST['step2_submit']) && isset($_SESSION['step1_data'])) { echo "Your data: <h1>Step 1</h1>"; foreach($_SESSION['step1_data'] as $field => $value) { if($field != 'step1_submit') echo "<p><b>$field</b> = $value</p>"; } echo "<h1>Step 2</h1>"; foreach($_POST as $field => $value) { if($field != 'step2_submit') echo "<p><b>$field</b> = $value</p>"; } } else { echo 'Fill in <a href="step1.php">Step 1</a>'; } ?>
-
Where are you setting the variables $email, $firstname, $park, $orderdate, $hour, $min $ampm, $description and $leader from?
-
In main.php you're submitting the form to attendence.php
<form name="form1" action="attendance.php" method="get">
If you don't want the user to go to attentdence.php then set the forms submit action to main.php. Now above your form include attendence.php. Also I'd recommend you to change your forms submit method to post. Passing usernames/passwords over the url is insecure. You should also be encrypting your passwords within the database too.
-
Okay I have fixed the code.
Change this line
@$rows[$j] .= ' <td class="member">'.$data."</td>";
to
@$rows[$j][] = ' <td class="member">'.$data."</td>";
Now change the foreach loop to
foreach($rows as $row) { echo " <tr>" . implode("\n", $row); // if the current row doesn't have enough columns // then output the required number of blank cells to complete the row if($j = (count($row) < $num_cols)) { for($i = 0; $i < $j; $i++) echo '<td class="member"></td>'; } echo " </tr>"; }
The @ symbol is used for error suppression.
-
Okay, I see the problem. My original code which you're basing your code off of is not smart enough to output the desired amount of <td></td> to complete the table structure. That is why your table borders are not matching up. I'll see if I can fix my original code.
-
You cannot place php code within a .html file. That is why it is not working. Rename index.html to index.php and the PHP code will be parsed.
-
What do mean by "checked". You need to post more information to your problem along with your code.
-
Post your actual code here. Your cannot expect the the codes you're trying will work straight away for you. They will need to be adapted to work with your data.
-
Where are you calling $product->getPrice()? Is it in the same file as when you initiated the product object (when you created the product). Or in a different page?
If its in a different page then variables you define in one page will not be passed on to the next. Variables die when the script finishes.
-
Start a new thread. This thread is 3 years old.
-
It's in a .php file and I'm using include
Where are you using the include?
All PHP code must be within a .php file. PHP code will not work within any other file type (unless the server is configured to do so).
How to turn this off ?
in Apache HTTP Server
Posted
Are you getting a directory listing of all the images in that folder? This is called FancyIndexing. You should be able to disable it by adding the following line into a .htaccess file within your photos directory
Or you can simply add a blank index.html file inside that directory.
Which ever option you take you'll get a 403 forbidden error or a blank screen when going to mysite.com/photos/