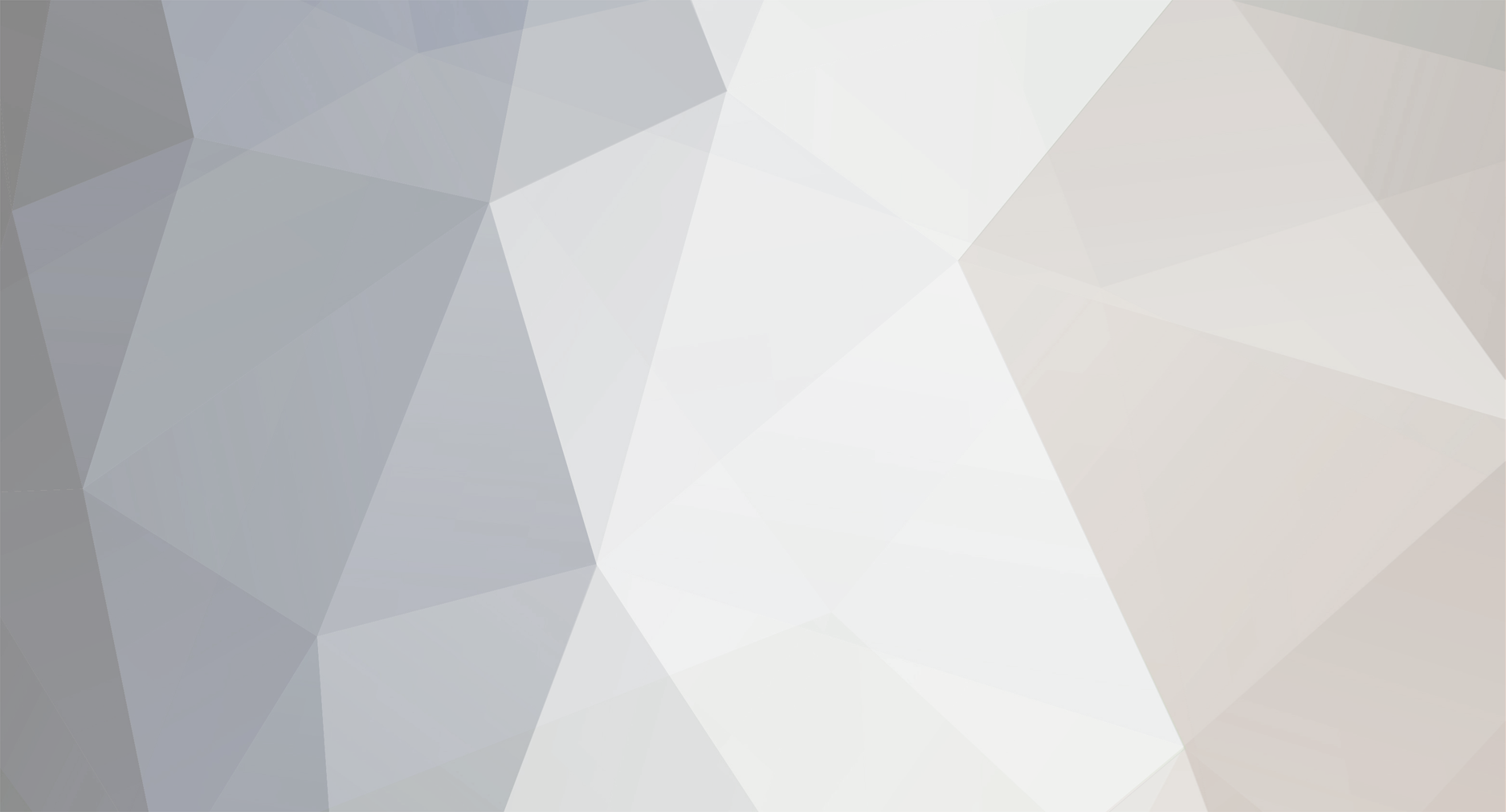
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
It is because you're using the assignment operator rather than the comparison operator (==)
<?php if ($awardType = 'Singular') { ?>
-
So $a will contain the id of a div you want to get, for example when $a is set to info only select the div with the id of info. In that case you can do this.
$a = 'info'; preg_match_all('#<div id="' . $a . '">(.*?)</div>#sim', $page_all, $div_array);
-
Any reason why you're creating files with a bash script. PHP has many functions for reading/writing to files.
-
What is col10value1 and col10value2. Not sure what you're trying to do.
-
Again you're not writing your queries correctly
$u2 = "UPDATE student SET `cname` = '$_POST[cname]' WHERE cname = $_POST[cname]";
$_POST[cname] should be wrapped in quotes, like you did in the second line of code above. Also posting raw post values into a query without sanitizing it will result in your queries being hijacked using SQL injection.
-
You should read up on variable scope. Functions have their own variable scope meaning variables defined outside of them cannot be used within them. When using variables with functions you should be passing them as arguments. eg
function myFunc($myVar) { echo $myVar; } $myVar = 'something'; myFunc($myVar); // pass $myVar to the function
-
Read my reply above. It is because your queries are not constructed correctly.
-
Use $div_array[1][0] instead maybe.
-
To have that result you only need a few lines of code
$resultSet = array(); while($row = mysql_fetch_assoc($query)) { $resultSet[] = $row; } echo "<pre>".print_r($resultSet,true)."</pre>";
-
The function preg_match_all() is returning an array of matches. print_r() will output the contents of an array. If all you want is the text within the div tags then just echo out the variable $div_array[1] rather than using print_r.
<textarea cols="50" rows="10"><?php echo $div_array[1];?></textarea>
-
String values should be wrapped in quotes within your queries. Your first query should be fine however I'd write it as
$cid = (int) $_GET['cid']; $q = "SELECT * FROM course WHERE cid = $cid";
For your second query you should write it as
$cname = mysql_real_escape_string($_GET['cname']); $q2 = "SELECT * FROM student WHERE cname = '$cname'";
Those two queries should work as expected but you could actually merge those two queries together using a JOIN.
-
Post the code that adds the user into the database here. I'll try to point you in the right direction.
-
Your dates seem to be stored in the database as a coma delimited list. You will need to use explode to convert the list back into an array in order for the correct checkboxes to be selected.
To convert the dates back into an array change
$db_year[] = $row[YEAR];
to
$db_year = explode(', ', $row['YEAR']);
-
I'm not a regex expert but I believe (.*) is greedy. Whereas ([^/\.]+) is lazy. I maybe wrong on the latter.
Whenever I use regex I prefer to use character classes eg I use /([a-z])+/ if I'm only searching for letters in a segment of the url, or /([a-z0-9])+/ if a segment of the url can contain letters and numbers etc.
-
Put your rules in reverse order.
-
Could you explain what your form is, what your script does with the data it gets from your form and why the need to call a javascript function from PHP - which I have already explained you cannot do. You can use javascript (via AJAX) to call PHP but you cannot use PHP to call javascript
-
As you have set_error_handler('my_error_handler', E_ALL); within your my_error_handling() function make sure you are calling that function in order for the error handler to work. Also in order for errors to be displayed you need to enable display_errors either within your script using ini_set or within your PHP configuration file (php.ini).
Also PHP will also log the errors within your servers error logs too. You can configure PHP to log errors to a dedicated file within the php.ini. You may be able set it with ini_se too.
-
PHP cannot call javascript functions and vise versa. By the time the javascript code is executed PHP has finished. PHP is executed on the server and the results of the PHP script is sent back to the http server The http server sends the text/html/javascript/css code to the web browser. The web browser then renders the webpages.
You could use AJAX which will allow to pass data from javascript to PHP on the fly without having to reload your page.
However if all you want to do is add a new row to a table you can just use solely javascript for this. You don't need PHP for it.
-
Have you tried writing the code yourself. It helps if you learn the basics of PHP.
-
Not really. You should start your links with a / that way your links will always load from the root of your site. Otherwise when you go to mydomain.com/pages/services your web browser will try to load your images/css files from mydomain.com/pages/services
-
You'll want to add another rule. Add this line before your first rule.
RewriteRule ^pages/(.*)$ $1.php [L]
-
When you register the user into your database you can use mail function to send an email. I'm sure you know how to use variables within strings to construct your email message.
-
still same problem
Helps if you provide more information to your problem. Tell us what the problem is and what you're script should be doing.
-
Not sure what you want to do here.
Do you want www.mydomain.com/services to be redirected to www.mydomain.com/pages/services and then have www.mydomain.com/pages/services call www.mydomain.com/services.php?
Or just have www.mydomain.com/services call www.mydomain.com/pages/services.php
Login Help
in PHP Coding Help
Posted
The problem is with your column. VARCHAR(25) is too small for an md5 hash. An md5 hash returns a 32 character random string. So you need to setup your password column to store at least 32 characters. Otherwise your code will always fail even if you do use the correct username/password combination.
Also I do not recommend you do this
You should only have one query which checks the username/password. The first and last queries are not needed at all.