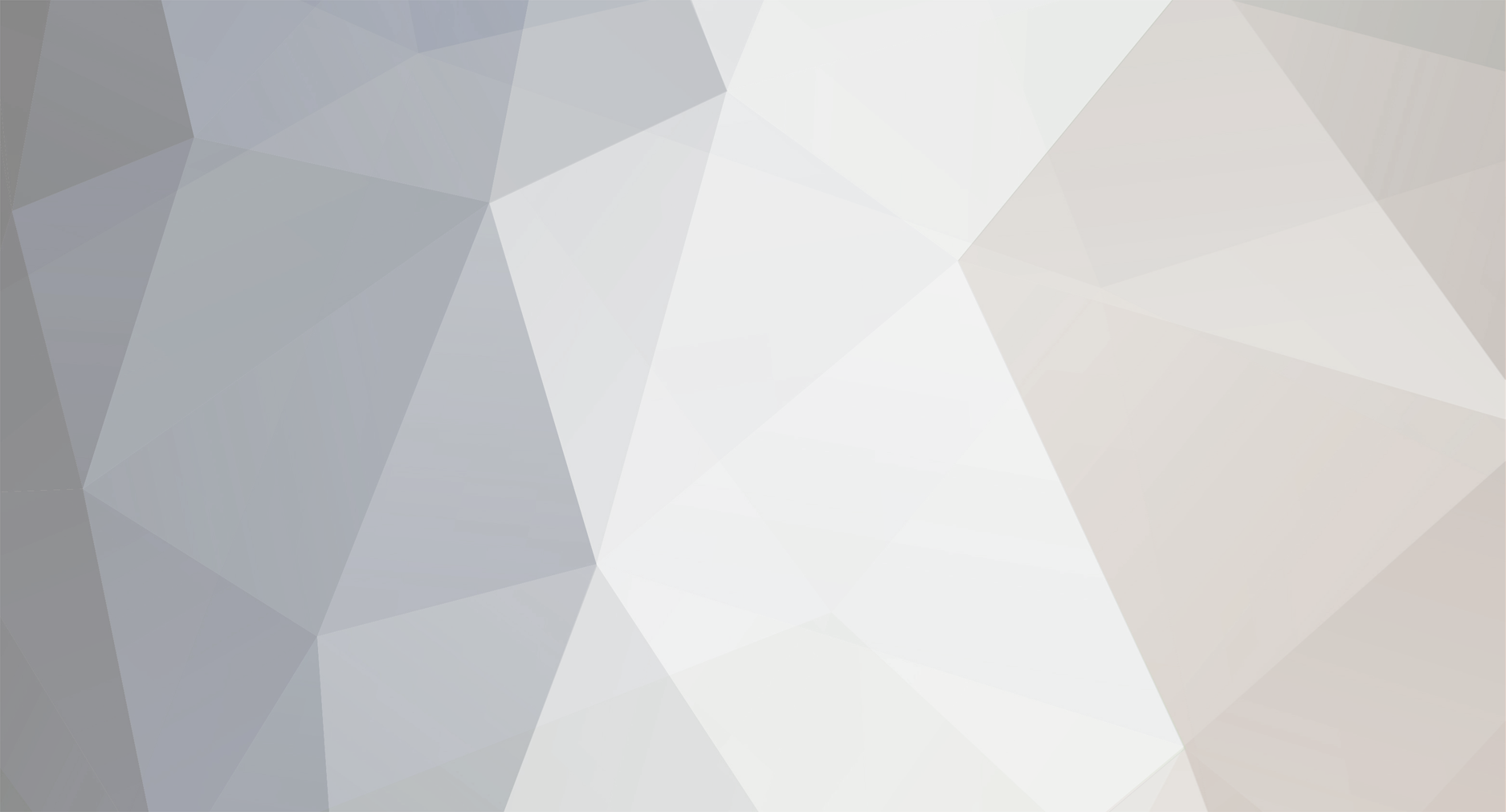
wildteen88
-
Posts
10,480 -
Joined
-
Last visited
Never
Posts posted by wildteen88
-
-
I guess line 182 is
$rating = (int)$row[rating]
You have forgotten to add ; at the end of that line. Also when using associative arrays you should ideally wrap your keys within quotes $row['rating'] rather than $row[rating].
-
In main.php you're calling header("location:attendance.php"); after output has been sent. header() cannot be called after output has been sent to the browser. You should move this block of code in main.php
<?php if(!empty($_POST['submit'])) { $username=$_POST['username']; $pwd=$_POST['pwd']; $_SESSION['user']=$username; //$_SESSION['user']; $_SESSION['pswd']=$pwd; } header("location:attendance.php"); ?>
Into the same code block where you have session_start();.
Use this code for main.php
<?php session_start(); //include "../sabkuch/dbconnect.php"; if(isset($_POST['submit'])) { $username = $_POST['username']; $pwd = $_POST['pwd']; $_SESSION['user'] = $username; $_SESSION['pswd'] = $pwd; header("location:attendance.php"); } ?> <form name="login" method="post" action="#"> <b>ENTER YOUR DETAILS BELOW</b><br/> <hr/> USERNAME:<input name="username" type="text" value=""><br/> PASSWORD:<input name="pwd" type="password" value=""><br/> <input name="submit" type="submit" value="LOGIN"> </form>
The next issue is in attendence.php, on line 16 you're calling session_destroy();. Calling this function will destroy the current session. You should only call this function in certain circumstances, such as when logging out the current user. If you leave it as is every time you go to main.php and fill in your username/password your session will always be reset.
-
No problem. Also another tip. When comparing values you should be using the comparison operator (==), not the assignment operator (=). String should always be wrapped in quotes. Otherwise PHP will think the word news is a constant rather than a string.
if($page = news){
So that line should read
if($page == 'news'){
-
Just include news.php and don't pass a query string to.
include("inc/news.php");
$_GET['id'] in news.php will return the id passed to it from your parent script (index.php?page=news&id=123)
Before I said you can't pass a query string to an include. You can do this by using a url as the path
include "http://site.com/file.php?var=value"
However if you wish to use any variables from file.php within your parent script you wont be able to do this. This is because when you use a url as the path for an include only output from that file (text/html) will be returned. The raw php source code of that file will not be returned.
-
I still don't fully understand the logic with the . ';" '; stuff
It is because you're outputting CSS. PHP and CSS statements must be ended with a semi-colon at the end of the line. A better way to code this
<p <?php echo ' style="font-family: ' . $_POST['font'] . '; '; echo 'font-size: ' . $_POST['size'] . '; '; echo 'color: ' . $_POST['color'] . '; "'; ?> >
Could be as
<p style="font-family: <?php echo $_POST['font'] ?>; font-size: <?php echo $_POST['size'] ?>; color: <?php echo $_POST['color']?>;">
-
This topic has been moved to Installation on Linux.
-
You cannot pass a query string to a php include.
-
If you're comparing values you should be using the comparison operator == (two equal signs together). At the moment you're using the assignment operator = (one equal sign). Change $info['message']= to $info['message']==
-
Post your code here.
-
That is what my original code did! Did you even try my code example?
if(!empty($store[$i])) $values[$i] = "( '{$id[$i]}', '{$store[$i]}', '{$item[$i]}', '{$itemprice[$i]}', '{$itemnumber[$i]}', '{$couponvalue[$i]}', '{$couponsused[$i]}')"; // build the array of values for the query string
The ! symbol means NOT. The if statment means this is. If the store field for the current row IS NOT empty them add the store, item, price etc into the database.
-
I haven't got a clue your code is a complete mess.
-
No not at all. I am saying this
<a href="http://www.ebermylove.com/login.php"><input type="submit" name="submit" value=" Submit! "></a>
Is invalid html syntax.
What are you trying to do when the form is submitted?
-
Your rewrite rule is incorret, it should be
RewriteRule cat/(\w+)$ urls.php?cat=$1
Mod rewrite will not rewrite your existing links within your files. You'll need to change say
<a hef="urls.php?cat=whatever">whatever</a>
to
<a hef="/cat/whatever">whatever</a>
Yourself.
If you went to http://localhost/urls/urls.php?cat=mouse it will not redirect the user to http://localhost/urls/cat/mouse
In fact, even http://localhost/urls/urls.php/cat/and/mouse/are/having/a/fight/over/bacon does not give an error, instead, it is still displaying the content of http://localhost/urls/urls.phpThat is because that is infact a valid url
-
Have a look at youtubes developer api
-
I don't think you can make a submit button a link
<a href="http://www.ebermylove.com/login.php"><input type="submit" name="submit" value=" Submit! "></a>
What are you trying to do submit the form to login.php and form.php?
PS when posting code wrap it within
or
tags
-
The text that comes after the question make in the url is referred to as the query string. It is up to you what you set in the query string in order for your php script to function. For example you're making a news script. You may code this script so it requires an id variable to be passed in the query string to grab a news entry within a database that is associated with that id.
-
PHP can send emails with the mail function.
-
IIS is preventing PHP from writing files to C:\inetpub\wwwroot\. I think you need to configure IIS to allow PHP to write files to www directory.
To fix this error
It is not safe to rely on the system's timezone settings. You are *required* to use the date.timezone setting or the date_default_timezone_set() function. In case you used any of those methods and you are still getting this warning, you most likely misspelled the timezone identifier. We selected 'Europe/Paris' for '1.0/no DST' instead in C:\inetpub\wwwroot\10.php on line 3Set date.timezone to Europe/Paris or whatever your timezone is within the php.ini
date.timezone = Europe/Paris
IIS may need to be restated in order for the changes to take affect.
-
Strange whey you're getting that error when on line 18 it is the the <p> tag. Have you modified the script in any way?
-
I would then like to sort those arrays by things like branch name, costs and profit.
Wouldn't it be easier to do this within your query eg
SELECT brand, costs, profit FROM table ORDER BY brand ASC
That will return all the branches in alphabetical order.
-
Change the if statement to just
if(!empty($store[$i])) $values[$i] = "( '{$id[$i]}', '{$store[$i]}', '{$item[$i]}', '{$itemprice[$i]}', '{$itemnumber[$i]}', '{$couponvalue[$i]}', '{$couponsused[$i]}')"; // build the array of values for the query string
That will make just the store field require. The others will be optional.
As your table stands you have 7 fields in your table (user_id, store, item, itemprice, itemnumber, couponvalue, couponsused). Each of those fields will require a value of some sort (be it an empty value or what is entered in your form). If you only want the store name inserting into one row, then on the next row you add in the item, itemprice, itemnumber, couponvalue, couponsused values then that is bad database design.
A better way would be to enter all your stores within your stores table, then just have the store id of the store that the product belongs to within your item table.
-
I assume you mean on your webpage. You can use an ordered list
$result = mysql_query($query); echo "<ol>"; while(list($username, $dl_sumtotal) = mysql_fetch_row($result)) { echo "<li>$username: $dl_sumtotal</li>"; } echo "</ol>";
-
You should format your code so it becomes easier to read. The issue is your're not ending your echo statements with a ;
if (strtotime($row['shutoff']) <= time()) { echo 'No longer accepting registrations.'; } else { echo "<INPUT TYPE='submit' name='submit' VALUE='Register'>"; }
-
Yes a form can have multiple submit buttons.
<?php if(isset($_POST['bob_submit'])) { echo "send message to bob"; } elseif(isset($_POST['richard_submit'])) { echo "send message to richard"; } ?> <form action="" method="post"> <input type="submit" name="bob_submit" value="Send to Bob" /> Or <input type="submit" name="richard_submit" value="Send to Richard" /> </form>
Going to next page problems!!!
in PHP Coding Help
Posted
You do not wrap an anchor tag around a submit button to send the user to login.php. Where the form submits the data to is controlled by the action attribute