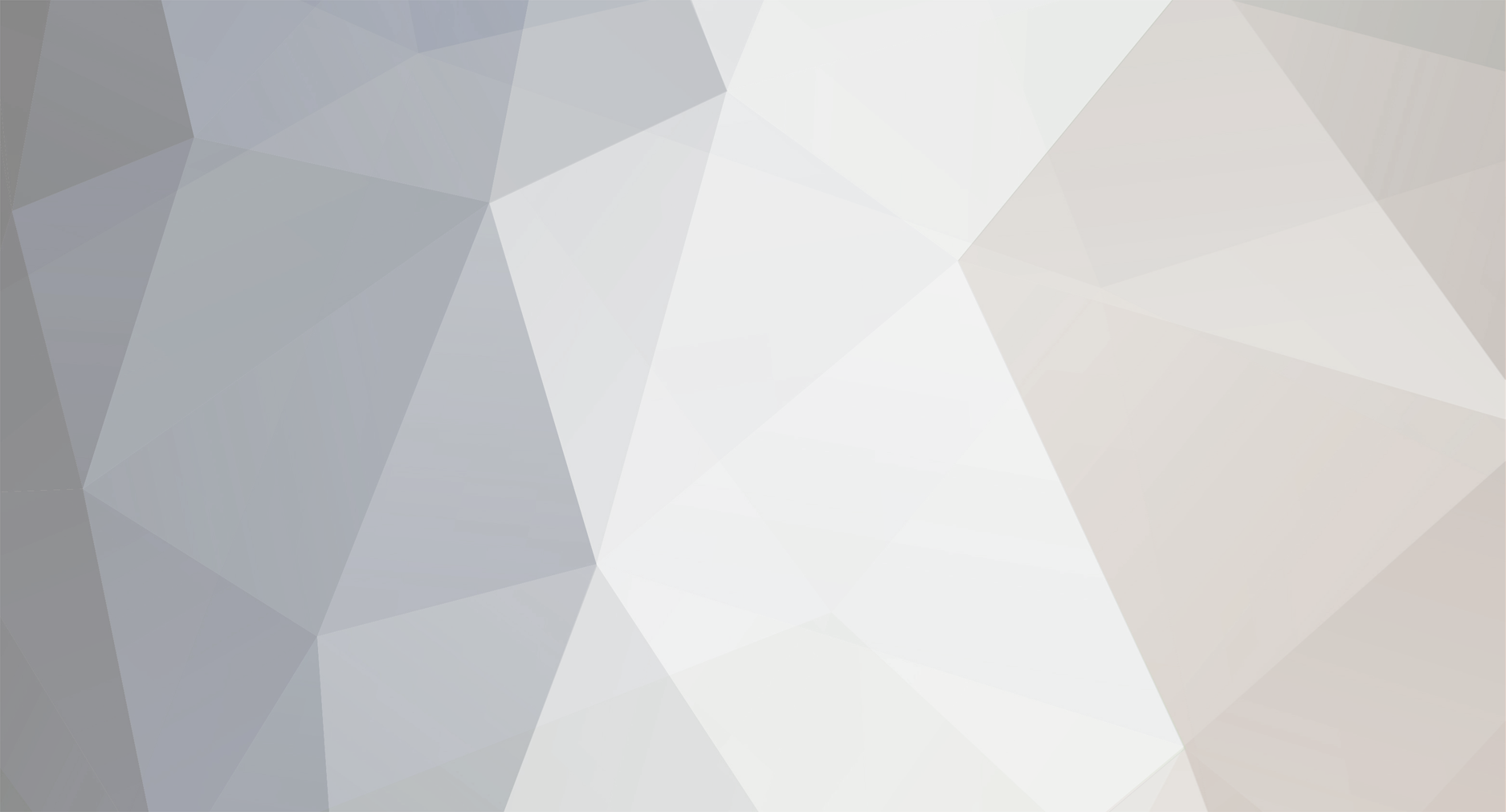
trq
Staff Alumni-
Posts
30,999 -
Joined
-
Last visited
-
Days Won
26
Everything posted by trq
-
I'm working on a rather simple jQuery plugin designed to dynamically load pages when you click on an #anchor. This is all working. The problem is, that some of the pages being loaded also contain the #anchor links, these are not working. In fact, no links within the dynamically loaded pages are working. The plugin: (function($) { $.fn.extend({ stageloader: function(options) { var attach = function() { var $page = $(this).attr('href'); $(ops.stage).fadeOut(ops.fade, function() { $(ops.stage).load( ops.location + $page.replace('#', '') + ops.extension ); $(ops.stage).fadeIn(ops.fade, function() { $(ops.stage).find('a[href^="#"]').click(attach); }); }); } var getUri = function(def) { var uri = document.location.toString(); if (uri.match('#')) { return '#' + uri.split('#')[1]; } if (def) { return def; } return ''; } var defaults = { init: '#home', stage: '#content', location: '/', extension: '.html', fade: 10 } var ops = $.extend(defaults, options); $(ops.stage).load( ops.location + getUri(ops.init).replace('#', '') + ops.extension ); $(ops.stage).fadeIn(ops.fade); $(this).find('a[href^="#"]').click(attach); return this } }); })(jQuery); The html: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en"> <head> <title></title> <meta http-equiv="content-type" content="text/html; charset=utf-8" /> <link rel="stylesheet" type="text/css" media="screen" href="assets/css/styles.css" /> </head> <body> <div id="wrapper"> <div id="inner"> <div id="header"></div> <div id="content"></div> </div> <div id="nav"> <ul id="sliding"> <li><a href="#home">Home</a></li> <li><a href="#author">About The Author</a></li> <li><a href="#books">About The Books</a></li> <li><a href="#videos">Videos</a></li> <li><a href="#downloads">Downloads</a></li> <li><a href="#games">Games</a></li> </ul> </div> <div id="push"></div> </div> <div id="footer"></div> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.1/jquery.min.js"></script> <script src="assets/js/jquery.slide.js"></script> <script src="assets/js/jquery.stageloader.js"></script> <script> $(document).ready(function() { $('body').stageloader({location: 'assets/pages/'}); }); </script> </body> </html> The author.html file: <h2>About the Author</h2> <h3>Suzanne Collins</h3> <p> Some text </p> <p> <ul> <li>Download <a href="assets/pdfs/qandq.pdf" target="_blank">the PDF</a></li> <li>Read the <a href="#answers">answers to your Burning Questions</a></li> </ul> </p> <p style="float:right"><img src="assets/imgs/sc.jpg" alt="author" /></p>
-
You can use MAX like that, your right though about the potential to break if a record is removed.
-
$style = mysql_query("SELECT borderbg, bg1, bg2 FROM ".PREFIX."cup_baum WHERE cupID=MAX(cupID)-1);
-
Generally, class get instantiated into objects. These objects have methods that operate on data within the objects themselves. If, you have a class that doesn't contain its own set of data, there isn't really any need to instantiate it into an object. That's when you would use static methods. The problem with your Cor class for instance is it contains a bunch of unrelated methods that don't really share common data. A class should describe one (and one only) 'thing'. The code within 'Core' should likely be moved out into what is known as 'client code'. This is the code that call's upon objects to do its work. Some of the methods within 'Core' however could likely be turned into there own classes.
-
For instance. Say I am using an sqlite database, but I wont to use your SomeClassThatNeedsAccessToADb in my project. I know it requires a database object that implements the 'Db' interface, so all I have to do is create an sqlite class that implements the same interface and It is guaranteed to work with your SomeClassThatNeedsAccessToADb without me ever having to edit its contents. A big point of OOP is that classes should never need to be edited to be used.
-
This forces the __construct method to accept only an object of type 'Db'. I'll assume you meant you dont understand. By forcing your code to implement interfaces you define what objects can and can't accept. This makes your code allot more reliable and in the long run, more flexible.
-
Just because one object may rely upon the functionality of another does not mean it should be an extension of that other object. For example, an Authentication class may need to use a database class in order to lookup username/password combinations within a database, does it extend the database classes functionality though? No, it simply uses it. As for your global situation and your database object. Any class that requires the ability to query the database should be passed the database object either through it __construct, or some other method. In order to make this as flexable as possible, your database object should also implement some 'interface' so that your objects that are going to use it understand exactly what your database object provides. A very simple example (not tested and no error handling in place). <?php interface Db { public function connect($host, $user, $pass, $db); public function query($sql); } class Mysql implements Db { private $conn; public function connect($host, $user, $pass, $db) { $conn = mysql_connect($host, $user, $pass); mysql_select_db($db); $this->conn = $conn; } public function query($sql) { return mysql_query($sql); } } class SomeClassThatNeedsAccessToADb { private $db; public function __construct(Db $db) { $this->db = $db; } public function getAllUsers() { $this->db->connect(); return $this->db->query(" SELECT * FROM Users "); } } $obj = new SomeClassThatNeedsAccessToADb(new MySql('foo','foo','foo','foo')); $result = $obj->getAllUsers(); Doing things this way it is easy to change databases because your objects rely on a defined interface. eg; SomeClassThatNeedsAccessToADb relies on being passed an object that implements the Db interface. Because of this, it is always safe to use the connect() and query() methods this interface provides. You could easily build a database class now that connects to an sqlite database, as long as it implements the same Db interface your application won't break. This is by no means a perfect example, but it hopefully gets accross the idea.
-
The error relates to the fact that you are storing data within a variable: $this->Global[$Data['Name']] which has not been declared within the class definition. Having said that. This entire design is shot. Classes should one entity within your system. Creating a class called 'Core' and jamming misc functionality within it is NOT oop. Then, there is the issue of globals. Global data breaks your objects encapsulation because they are now relying on outside data suddenly turning up. If you need to get data into your objects, force it to be provided through a proper interface.
-
With hat many globals kicking around you may as well drop the entire idea of using classes anyways.
-
I could use a design sanity check, new to OOP...
trq replied to linus72982's topic in Application Design
Classes should represent one and only one entity. The idea of jamming login / logout, session handling, error handling & validating input all in one class wreaks of poor design. -
Have you had a look in the Apache configurations? (httpd.conf or apache.conf) There is a section in there which denies access from ip ranges which fall outside what are valid lan ranges.
-
Can we see all of the code in this class plus any code where you are calling it?
-
I'm not sure there is any good way of doing this. Patches generally need to be applied to unmodified source. That's why any fixes should always be sent upstream, so they can be maintained buy the developers there. As for modifications, as soon as you start down that path, its up to you to maintain your own work. If you want to be able to apply upstream patches along with your own work then you should likely keep your own work in patches too and apply them both (upstream and yours) to an unmodified source.
-
Read the examples in the manual. Its not rocket science.
-
Put simply, your client (PHP) does not have enough memory to execute the query. To put it frankly, I'm not surprised.
-
echo "<a href='http://{$row['ownip']}'>{$row['fullname']}</a>";
-
I wouldn't say the script is outdated so much as very poorly written. A few things. Firstly, mysql's PASSWORD function should never be used as means of hashing passwords. There is no guarantee they won't change there algorithms between versions (this is highlighted within the mysql manual). Secondly, there is no need at all for the while loop. You should only have one record. Other than that, there probably not too much wrong with it. I prefer to use sessions rather than cookies to maintain a users session data but whatever....
-
You need to make sure all special chars are escaped before you can safely insert them into a database. Take a look at mysql_real_escape_string.
-
Describe 'not working'. We are not mind readers.
-
function strtoarr($option){ if($option == 'abc'){ $string = 'qwertyuiopasdfghjklzxcvbnmQWERTYUIOPASDFGHJKLZXCVBNM ,.'; }else{ $string = $option; } return str_split($string); }
-
The form doesn't show up because the very first line checks to see if the script has been passed an id. if (isset($_GET['id'])) {
-
I think you mean mediator, and I'm not sure I see where / how it relates to a login system. Generally, applications are made of several different patterns. There is no one pattern that would be recommended for a login system.
-
You need to pass an id to this script via the url so that your query has something to look up.
-
The best advice I could give to learn OOP is to use one of the already existing OOP frameworks. Even if you end up rollong your own (as I did) a well designed framework will teach you allot. Zend was where I started but it has a pretty steep learning curve. There's plenty of others around though.
-
Are you passing the id param along with the request for this page?