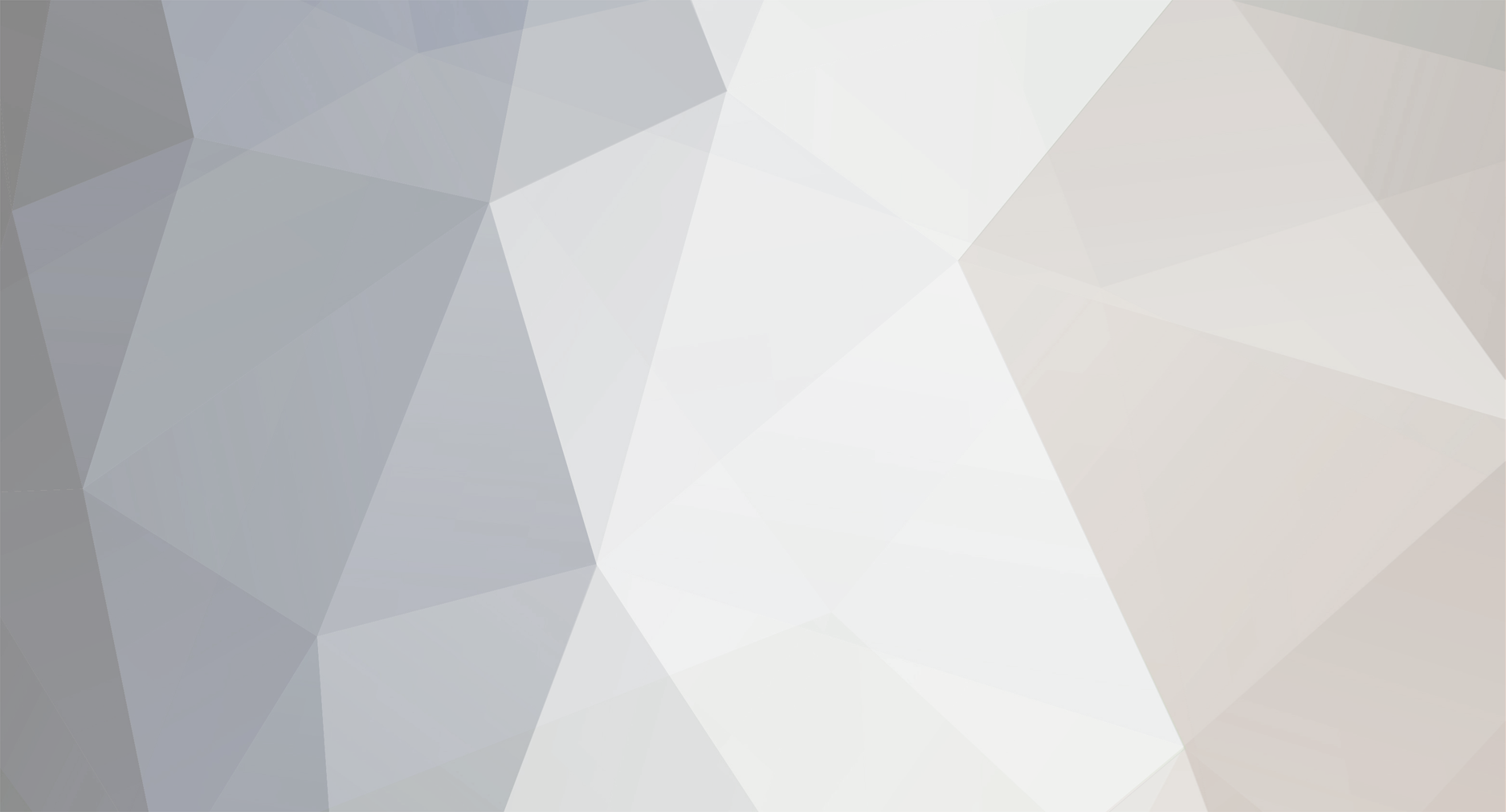
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
My Social Networking Website. In progress, looking for suggestions
ignace replied to shortysbest's topic in Website Critique
user/pass please. old one doesn't work anymore. -
Or in the Controller, since you may want at some point support OAuth (Facebook Connect, Twitter, ..). class AuthController { public function loginAction() { // normal form login // forwards to authenticateAction() for authentication } public function authenticateAction() { // authenticate callback of OAuth } } Which means your User object has to be completly authentication/database agnostic and be nothing more then a simple DTO. class User { private $id; private $username; private $password; // getters/setters }
-
Great, now my license is set to expire in August, 2014. If they keep this up my license will live beyond me!
-
One artist is part of one-or-more bands. One band has one-or-more artists. +-------------------------+ | artist | +-------------------------+ | PK,AI | artist_id | INT | +-------------------------+ +-------------------------+ | band | +-------------------------+ | PK,AI | band_id | INT | +-------------------------+ +--------------------------+ | band_has_artist | +--------------------------+ | PK,FK | band_id | INT | | PK,FK | artist_id | INT | | NN | joined_at | DATE | | | left_at | DATE | +--------------------------+ One album has one-or-more songs. One song is on one album (technically). +--------------------------+ | song | +--------------------------+ | PK,AI | song_id | INT | | FK,NN | album_id | INT | | NN | length | INT | +--------------------------+ +--------------------------+ | album | +--------------------------+ | PK,AI | album_id | INT | | FK,NN | band_id | INT | +--------------------------+
- 6 replies
-
- architecture
- best practices
-
(and 1 more)
Tagged with:
-
Separting Logic From Design, Ideas For Outputting
ignace replied to sn2005's topic in Application Design
All HTML should be in an HTML file, not in a PHP file/class. If you want to use a templating system you can achieve this using a two-step view. I highly suggest you check out Twig or Smarty instead of rolling your own. -
Flaw In Php's Namespace, Cannot Import An Entire Namespace,
ignace replied to Hall of Famer's topic in Miscellaneous
use \Model\User\Member as Member; use \Model\User\Admin as Admin; use \Model\User\Banned as Banned; use \Model\User\Guest as Guest; LOL 1) The as Class part is redundant 2) Why would you create a class for each user role? 3) Assuming all of them implement a UserInterface, how would you check if you are dealing with a banned user? if ($user instanceof Banned) { Which is: 1) Not flexible and also hard-couples your code to an implementation 2) If at some point you wish to create a PrivilegedMember you will have to hunt down all type checking code and add it. 3) It's error prone, for example if PrivilegedMember extends Member type checking like this: if ($user instanceof Member) { // .. } else if ($user instanceof PrivilegedMember) { Means a privileged member is no longer so privileged. 4) It's boring. A new type means lots of code changes as does privilege changes. Instead use roles, resources, and privileges: if ($this->acl->isAllowed($this->user, 'content', 'edit')) { Banned is not a role, more a state, since anyone can be banned, afterwards you still have to know what role he has/had, and it can have special behavior like being only temporary. Something that is not easily captured in an ACL if ($user->isBanned()) { $this->redirect()->to('/you-are-banned'); } -
Calling A Method In A Class From Another Class
ignace replied to programming.name's topic in PHP Coding Help
class AnObject { private $anotherObject; public function __construct(AnotherObject $B) { $this->setAnotherObject($B); } public function setAnotherObject(AnotherObject $B) { $this->anotherObject = $B; } public function showAnotherObject() { $this->anotherObject->show(); } } class AnotherObject { public function show() { echo 'I got in!'; } } $a = new AnObject(new AnotherObject); $a->showAnotherObject(); -
Have you tried anything so far? Like read the manual page file_put_contents. There is an FILE_APPEND constant that you can pass... function object2file($dir, $object, $mode = 0) { file_put_contents(rtrim($dir, '\/') . DIRECTORY_SEPARATOR . get_class($object) . '.obj', serialize($object), $mode); } object2file('/path/to/dir', new stdClass, FILE_APPEND);
-
function object2file($dir, $object) { file_put_contents(rtrim($dir, '\/') . DIRECTORY_SEPARATOR . get_class($object) . '.obj', serialize($object)); }
-
=? is most likely a placeholder for whatever value you want to pass to the database, and make sure it's safe to do so.
-
/** * Takes an URL and returns a JSON object, throws an Exception if anything goes wrong. * * @param string $url A WOT URL * @param bool $isUrl true = http, false = file for testing * @return object The JSON response * @throws Exception When something goes wrong */ function readWotResponse($url, $isUrl = true) { if ($isUrl && !ini_get('allow_url_fopen')) { throw new Exception('allow_url_fopen = Off'); } $content = file_get_contents($url); if ($isUrl) { $status_code = substr($http_response_header[0], 9, 3); if ($status_code != 200) { throw new Exception('Got status code ' . $status_code . ' expected 200'); } } $json = json_decode($content); if (!$json) { throw new Exception('Response contained an invalid JSON response'); } if ($json->status != 'ok') { throw new Exception($json->status_code); } return $json; } /** * Inserts $data in $table * * @param mysqli $dbconn * @param string $table * @param array $data */ function sqlInsert(mysqli $dbconn, $table, $data) { //.. } $url = '..'; try { $json = readWotResponse($url); foreach ($json->data->items as $item) { sqlInsert($dbconn, 'items', (array) $item); } } catch (Exception $e) { echo $e->getMessage(); }
-
while ($list = mysql_fetch_assoc($result)) { $id = $list['id']; $username = $list['username']; $name = $list['name']; $email = $list['email']; $level = $list['level']; $table_content .= "<tr><td><input name='usercheckbox[]' type='checkbox' value='".$id."' title='Select/Deselect User'></td> <td>".$username."</td> <td>".$name."</td> <td>".$email."</td> <td>".$level."</td> <td><a href='view.php?id=".$id."'><img src='images/view.png' title='View details' class='menuentry'><a href='edit.php?id=".$id."'><img src='images/edit.png' title='Edit' class='menuentry'><a href='delete.php?id=".$id."'><img src='images/remove.png' title='Delete' class='menuentry'></tr>"; } You can reduce this further so that you have one part that retrieves the rows and puts them into an array and then afterwards in your html: <?php foreach ($rows as $row): ?> <tr> <td><?php echo $row['username'] ?></td> <td><?php echo $row['name'] ?></td> <td><?php echo $row['email'] ?></td> <td><?php echo $row['level'] ?></td> <td> <a href="/view.php?id=<?php echo $row['id'] ?>"> <img src="/images/view.png" title="View details" class="menuentry"> </a><!-- you forgot these --> <a href="/edit.php?id=<?php echo $row['id'] ?>"> <img src="/images/edit.png" title="Edit" class="menuentry"> </a><!-- you forgot these --> <a href="/delete.php?id=<?php echo $row['id'] ?>"> <img src="/images/delete.png" title="Delete" class="menuentry"> </a><!-- you forgot these --> </td><!-- you forgot these --> </tr> <?php endforeach ?> Furthermore introducing functions/classes would help you a lot: function getAllUsers(mysqli $dbconn, $count = null, $page = 1) { $sql = ' SELECT user_id, user_name, user_email FROM users '; if ($count !== null) { $sql .= sqlGenerateLimit($count, $page); } $result = mysqli_query($dbconn, $sql); return dbResultToArray($result); } function sqlGenerateLimit($count = null, $page = 1) { $limit = "\nLIMIT %d, %d"; $offset = ($page - 1) * $count; return sprintf($limit, $offset, $count); } function dbResultToArray($result, $mode = MYSQLI_ASSOC) { $rows = array(); if ($result instanceof mysqli_result) { if (mysqli_num_rows($result)) { while ($row = mysqli_fetch_array($result, $mode)) { $rows[] = $row; } } } return $rows; } Though you would need to add validation before using these.
-
Post your current code if you need coding help
-
I see you already solved it, though I should point out that you need to put an exit after the header. I would also expand a bit to make use of the extra parameters header provides. class Response { public function redirect($to, $code = 302) { header('Location: ' . $to, true, $code); exit; // or //$this->setHeader('Location', $to); //$this->setStatus($code); //$this->sendHeaders(); //exit; } }
-
I think all of this can be solved in a more mature way, no? Barand uses table aliasing as does 99% of the other programmers because you just can't do a self-join without them nor can you for any complex queries like many-to-many. He writes kick ass queries with his eyes closed. Do you really think that by using insults you are changing the world? Make everyone somehow 'believe' in your method? All you are getting is a ban!
-
That's why they nicknamed me 'The Rebel'
-
You should use a foreach
-
Call to undefined method 'World' on object 'Hello'. Greetings traveller! There are new topics available on the forum board.
-
It's in JSON format. json_decode
-
Go to your employer, tell him he called you names and see what he does. If he does nothing substantial, leave. There are better work environments. If he does, chances are it doesn't stop and he will still harass you but in different ways like bumping your shoulder, and generally not giving you any respect at all. At that point you should consider talking with the other employees and maybe they don't like him either and you can go ALL to your employer and demand he fires him. The important bit here is that you don't let him get to you, or your work will suffer, and you will be fired. I have been in this situation, where I was the new kid on the block among older employees who had their way of programming, which was insecure and hard to maintain, a hacker's paradise: every vulnerability was possible. Telling the employer about this and you are bound to have a few enemies. But me and a colleague didn't give up and after 2 years, now we have a professional setup: Scrum, OOP, QA, .. and I got a 45% raise
-
There are other fallen brothers, not just CSSFreakie, also Mchl and probably more