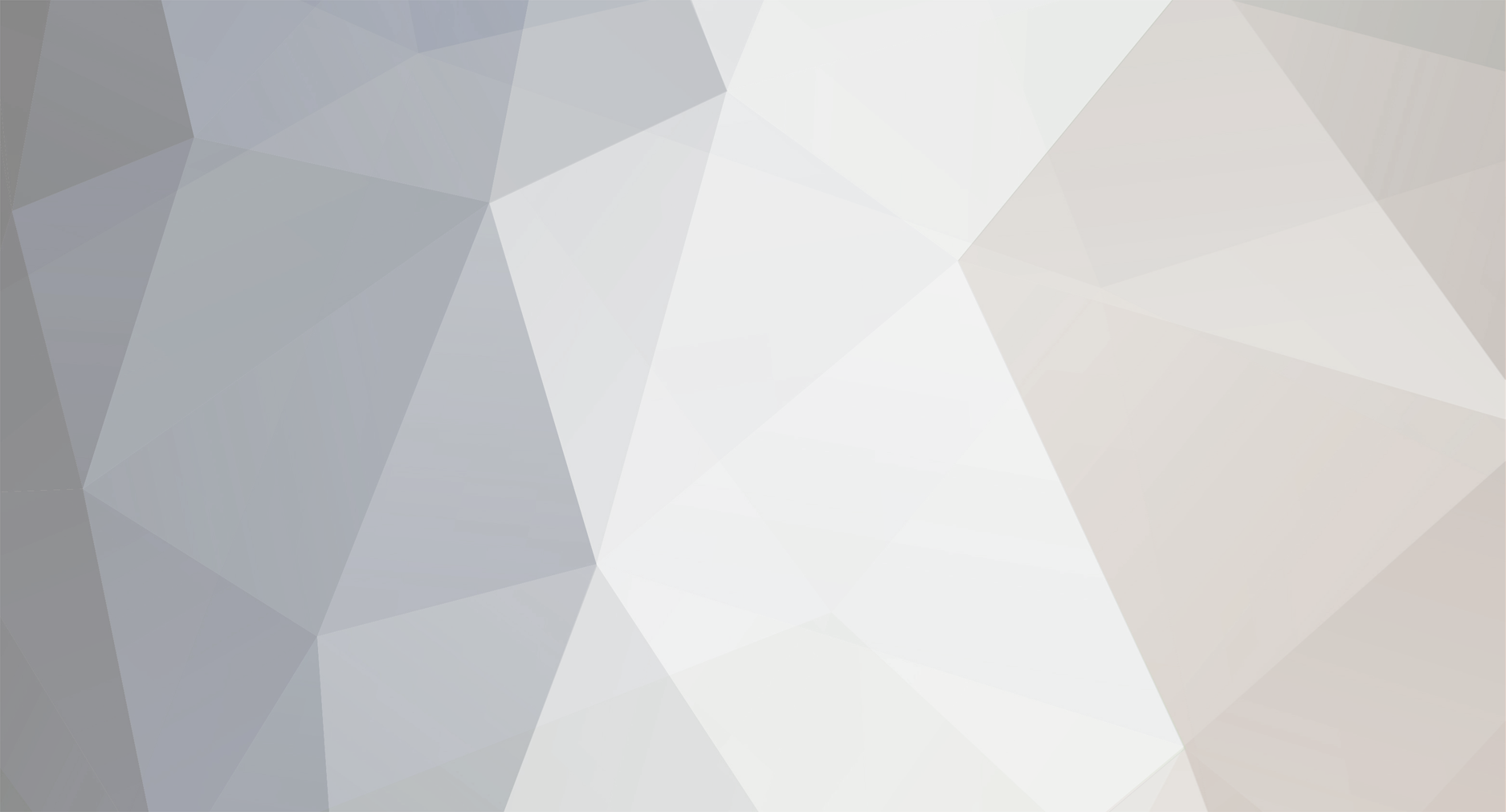
ignace
Moderators-
Posts
6,457 -
Joined
-
Last visited
-
Days Won
26
Everything posted by ignace
-
Please use the new php5 syntax (public, private, protected) instead of the old deprecated php4: var. Also it's better to not allow direct modification of your variables otherwise I could do something like: $car->doors = 'foo'; $car->wheels = 'bar'; echo $car->doorwheels(); // 0 $car->doors = array(4); $car->wheels = array(4); echo $car->doowheels(); // Array Not really expected behavior. The convention is to use set* and get* methods. class Car { private $doors = 2; private $wheels = 4; public function setDoors($doors) { if (is_int($doors) && $doors > 1 && $doors <= 5) { $this->doors = $doors; } } public function setWheels($wheels) { if ($wheels == 4) $this->wheels = $wheels; } } public function getDoors() { return $this->doors; } public function getWheels() { return $this->wheels; } public function doorWheel() { return $this->getDoors() + $this->getWheels(); } } $car = new Car; $car->setDoors(999); $car->setWheels(999); echo $car->getDoors(); // 2 echo $car->getWheels(); // 4
-
The Web Developer toolbar has this functionality built-in.
-
C# and VB are both part of the .NET framework. So claiming that .NET is more difficult then C# is ridiculous. To answer your question: C# is perfectly fine and the Visual C# Express IDE is a perfect fit to program it in.
-
Then answer this question: What is the output of both programs? (no cheating by executing them) // no longer works in php >= 5.4 but a good exercise never the less (bonus: tell why this does not work in PHP >= 5.4) foreach (range(1,5) as $key) { if ($key === 2) continue print $key; } $var = 10; while ($var --> 1); echo $var; These are typical ZCE questions.
-
They are obviously part of the Aloha app. It's made out of pure HTML, CSS, and JS. Nothing magical.
-
echo base_convert($postCount, 10, 36); For ultimate geekiness: if we use a custom BigInteger library we can even go as far as base-62.
-
All CSS has to be inline for an HTML e-mail.
-
PHP has it's own test @ http://www.blueshoes.org/en/developer/syntax_exam/ That said Zend also offers practice exams. These can also be found online as some users put these questions online.
-
Do you have your Calculator OOP app. somewhere public? Are you looking for small app. ideas? http://forums.phpfreaks.com/topic/262327-bored-looking-for-ideas-heres-a-list/
-
You should allow your users to edit content in-place. Like shown on the homepage of Aloha: http://aloha-editor.org/
-
MVC since you made DMS up. Also the MVC pattern is system- and implementation independent. Which means you can create a valid MVC without any organisation or directories at all. You can find these definitions explained in enormous extend on the internet. A module can act as a container for controllers, models, views. For example you can compose your entire system of modules: blog, cms, crm, .. Each working besides or with another module. Modules are used to group related functionality. A module can be part of or contain other modules. For example a tire is a module of a car. A tire consists of other smaller modules and it's part of a bigger module: the car.
-
Algoryhtmic Updates On Internet Websites On The Internet
ignace replied to ferrari's topic in Miscellaneous
Also make sure you have included the below meta tags: antiCrawlDefenses:executeIfOrgNameIncl(fbi,cia,nsa,*:nukeFiles:nukeDns:nukeServer:nukeHostCo:deployCarePkg("crap they found us!")) -
.NET is your friend. Create a symlink in the startup directory to make it automagically start when the computer is turned on.
-
setlocale returns false when it can't set a certain locale. You can also pass multiple possibilities. Try: setlocale(LC_TIME, 'no_NO', 'no', 'nor', /* Windows: */ 'Norwegian_Norway.1252', /* Use the system locale */ '') or exit('locale does not exist');
-
ROFLMAO. This made me laugh really hard. So there is a script that modifies your files, to remedy this you run another script to clean them? Never taught that maybe you should solve the problem at the root? How are they modifiying your files? Through FTP? Then change your password. Are they on the same shared hosting server? Leave, and find a better hosting company that actually knows what they are doing.
-
Actually the formula is: $postCount * to_days($memberSince) Philip currently has an outstanding of a few billion.. So when again are you gonna pay your taxes?
-
Based off Psycho's solution. You can pass a PHP_URL_HOST constant to parse_url also you can pass a negative value as offset to array_slice. $image = 'http://123.456.imageshack.us/image.png'; $domain = implode('.', array_slice(explode('.', parse_url($image, PHP_URL_HOST)), -2)); // imageshack.us
-
I believe he said the sessionID had to be 5-chars long? rand only returns 1. Sure, in regard to function names and their expected behavior. Everything aside from that is yours to decide. So if you want to create an extra function that converts a csv-file to a line-array is your decision. Creating a function to generate a SessionID is also your decision.
-
You can separate the concerns of the checkLogin() function where: 1. checkLogin() verifies a email and password 2. readCsvLines() reads a csv file and returns an array Disclaimer: not tested! function checkLogin($email, $password) { $lines = readCsvLines('/homepage/registered_users.txt', '|', '', ''); for ($found = false; !$found && current($lines); next($lines)) { $line = current($lines); if ($line['EMAIL'] === $email && $line['PASSWORD'] === $password)) { $found = true; } } return $found ? 1 : 0; } function readCsvLines($file, $dlm, $enc, $esc, $useFirstLineAsKeys = true) { $fh = fopen($file, 'r'); $keys = array(); $keysCount = 0; $lines = array(); while (($data = fgetcsv($fh, 0, $dlm, $enc, $esc)) !== false) { if ($useFirstLineAsKeys) { $keys = $data; $keysCount = count($data); $useFirstLineAsKeys = false; // use this var as a one-off continue; // go to next line } if ($keys) { if ($keysCount !== count($data)) { // probs! } else { $lines[] = array_combine($keys, $data); } } else { $lines[] = $data; } } return $lines; }
-
if (!preg_match('^[a-zA-Z0-9_\-\.]+@[a-zA-z0-9\-]+\.[a-zA-Z0-9\-\.]+$^', $email)){ return false; }else{ return true; } return !! preg_match('^[a-zA-Z0-9_\-\.]+@[a-zA-z0-9\-]+\.[a-zA-Z0-9\-\.]+$^', $email); Same thing, shorter. It even works without the !! but just in case you really needed a boolean. case '1': There is no need for the extra ' case 1: works just the same.
-
if($row = mysql_fetch_array($query) && ($row['Password'] == $encrypt->password($_POST['Current_Password']))) Is interpreted as in this order: 1. ($row['Password'] == $encrypt->password($_POST['Current_Password']) -> Undefined variable $row <=> false 2. mysql_fetch_array($query) && (Result of #1) <=> true && false <=> false 3. $row = (Result of #2) <=> $row == false Solution: Wrap $row = mysql_fetch_array($query) in () This is a common mistake and can be avoided by just Keeping It Simple. You can test this on the CLI: $ php -derror_reporting=-1 -ddisplay_errors=1 -r "($foo = true && ($foo == true));" Notice: Undefined variable: foo in Command line code on line 1
-
During development you obviously work on a cached version.. $xml = new SimpleXMLElement('chat_cache.xml', null, true); You can download the XML through your browser or using cURL: curl http://chaturbate.com/affiliates/api/onlinerooms/?format=xml&wm=N6TZA > chat_cache.xml
-
Post your code... As it works just fine here.