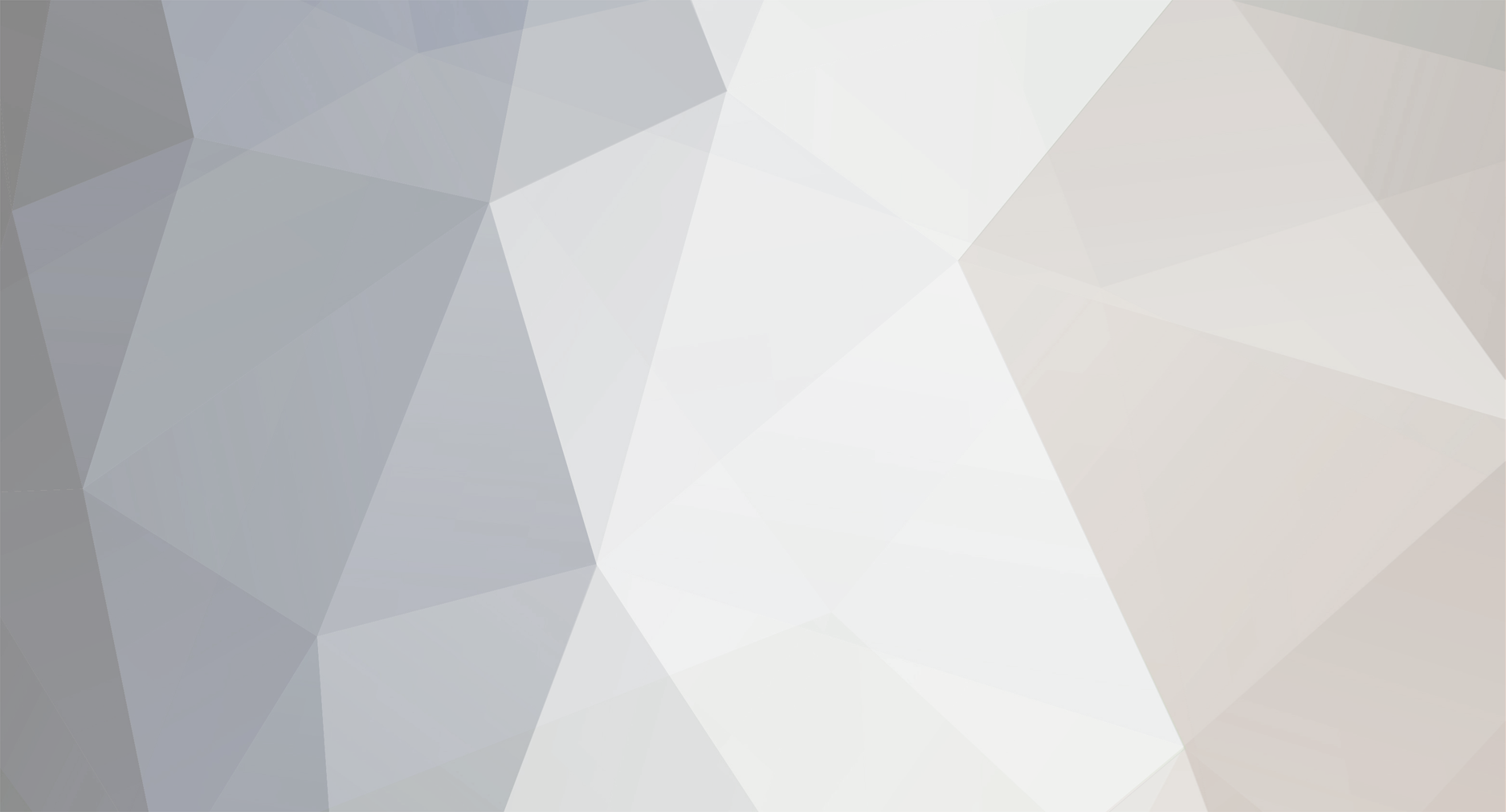
Jessica
Staff Alumni-
Posts
8,968 -
Joined
-
Last visited
-
Days Won
41
Everything posted by Jessica
-
People aren't going to write the entire code for you. You said you need help, but help with what? Is there a specific part that is not working, and how so?
-
Print specified text, if the SQL table's column's value is 0.
Jessica replied to username01's topic in PHP Coding Help
It didn't work because = is assignment. == is comparison. -
You'd need to use an if statement. Are all of the names in your database already? If you put the names into an array and store the selected one as a variable, it's a simple foreach to loop through them, and an if to check if it's selected.
-
You need to ask a question. What do you need help with, and what is the relevant part of your code?
-
Yay! Don't forget to mark as solved!
-
Has anyone here used the ADOdb Active Record implementation? http://phplens.com/lens/adodb/docs-active-record.htm I'm trying to use the ClassHasMany option to automatically load children. But it's not working. I have ClassBelongsTo on another class and it works fine, but this one is not working. I think the problem might lie with the primary key - foreign key relationships. My parent table: CREATE TABLE IF NOT EXISTS `grids` ( `grid_id` int(10) unsigned NOT NULL AUTO_INCREMENT, `x` int(11) NOT NULL, `y` int(11) NOT NULL, `location_id` int(11) NOT NULL, `city_id` int(10) unsigned DEFAULT NULL, `nation_id` int(10) unsigned DEFAULT NULL, `continent_id` int(10) unsigned DEFAULT NULL, PRIMARY KEY (`grid_id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=3 ; -- -- Dumping data for table `grids` -- INSERT INTO `grids` (`grid_id`, `x`, `y`, `location_id`, `city_id`, `nation_id`, `continent_id`) VALUES (1, 10, 10, 1, 1, 1, 1), (2, 10, 9, 1, 1, 1, 1); The child table CREATE TABLE IF NOT EXISTS `beasts` ( `beast_id` int(10) unsigned NOT NULL AUTO_INCREMENT, `beast_type_id` int(10) unsigned NOT NULL, `max_hp` int(10) unsigned NOT NULL, `current_hp` int(10) unsigned NOT NULL, `grid_id` int(10) unsigned NOT NULL, PRIMARY KEY (`beast_id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=5 ; -- -- Dumping data for table `beasts` -- INSERT INTO `beasts` (`beast_id`, `beast_type_id`, `max_hp`, `current_hp`, `grid_id`) VALUES (1, 1, 10, 10, 1), (2, 2, 5, 5, 1), (3, 1, 10, 10, 2), (4, 2, 5, 5, 2); The Class definitions <?php Class Grid Extends ADOdb_Active_Record{ } //This line is commented out because it didn't seem to help the problem, but I have tried it with it uncommented. I have also tried with only this one and not the Class one. //ADODB_Active_Record::TableKeyHasMany('Grids', 'grid_id', 'Beasts', 'grid_id') ADODB_Active_Record::ClassHasMany('Grid', 'Beast','grid_id'); Class Beast Extends ADOdb_Active_Record{ function __toString(){ return $this->Beast_Type->beast_type; } } //This one actually works. If I load a beast, the beast type does show up. ADODB_Active_Record::ClassBelongsTo('Beast','Beast_Type','beast_type_id'); ?> The php code I run <?php $grid = new Grid(); $grid->load('grid_id=?', 1); var_dump($grid); var_dump($grid->Beast); $beast = new Beast(); I've tried $grid->Beast, ->Beasts, ->beast, and ->beasts. Nothing is in any of them. $grid does have the right data: object(Grid)[40] public '_dbat' => int 0 public '_table' => string 'Grids' (length=5) public '_tableat' => string 'Grids' (length=5) public '_where' => string 'grid_id=?' (length=9) public '_saved' => boolean true public '_lasterr' => boolean false public '_original' => array 0 => string '1' (length=1) 1 => string '10' (length=2) 2 => string '10' (length=2) 3 => string '1' (length=1) 4 => string '1' (length=1) 5 => string '1' (length=1) 6 => string '1' (length=1) public 'foreignName' => string 'grid' (length=4) public 'grid_id' => string '1' (length=1) public 'x' => string '10' (length=2) public 'y' => string '10' (length=2) public 'location_id' => string '1' (length=1) public 'city_id' => string '1' (length=1) public 'nation_id' => string '1' (length=1) public 'continent_id' => string '1' (length=1) array empty Help? Thanks!
-
This has been addressed several times. The most recent I recall is here: http://www.phpfreaks.com/forums/index.php/topic,304652.0.html It's not pixel perfect. It never will be. Read that thread and then post some code if you can't get a solution! Good luck.
-
It's looking like this isn't something I can easily fix with CSS. I can't find any examples of a table looking the way I want without that 1 pixel. (IE6 - for work). I'm wondering about instead of using a CSS border on the TH, use a background image which consists of one pixel in the color I want, and repeating it from top right down? I need to play with this, but if anyone has any other ideas please let me know.
-
That would add a TON more html to the end result, which is something we need to avoid - but I like the idea and appreciate the quick suggestion!!
-
See attached image for example of problem. I want to have a 1px border on my th and my td. I want the border to be a different color on the th. The problem is the RIGHT and LEFT borders seem to take one pixel from the TOP and BOTTOM. Is there ANY way to do what I want without that obnoxious one pixel of the wrong color? [attachment deleted by admin]
-
I'm trying to write a simple game. I vaguely understand the ACL stuff for user logins, but here is my confusion. When the controller first loads, I want it to get the user object based on the ID in the session. Then do all the logic (and be able to access the user object). Then before it's displayed, save the user object to record any changes. I feel like I'm missing something - HOW do I do this?
-
need an example using php_rand on a multidimensional array please
Jessica replied to silverglade's topic in PHP Coding Help
Did it work? -
Having a litle problem with a membership script here
Jessica replied to sanmagician's topic in PHP Coding Help
It's pretty obvious... Did you look at the line it's talking about? Just looking at the code you can see a big error. } else { ?> -
| | v
-
You'd need to use mod_rewrite, otherwise your server is going to look for a folder called departname and an index file inside of it.
-
Understanding where to put logic in CakePHP for my game?
Jessica replied to Jessica's topic in Frameworks
I decided to make a table called "Map Spots" and every hour will generate new items on the map, and it will be communal. So I added a relationship with Maps and Map Spots var $hasMany = array( 'MapSpot' => array( 'className' => 'MapSpot', 'foreignKey' => 'map_id', 'dependent' => false, 'conditions' => '', 'fields' => '', 'order' => '', 'limit' => '', 'offset' => '', 'exclusive' => '', 'finderQuery' => '', 'counterQuery' => '' ) ); And added recursive to my user so when the user is found, their map and all map spots are as well. <?php class User extends AppModel { var $name = 'User'; var $recursive = 2; -
Understanding where to put logic in CakePHP for my game?
Jessica replied to Jessica's topic in Frameworks
Zanus, what I need to do is run some php code (a loop to generate random stuff basically) and ADD it to the object before it goes into the session. And only if it's not already there. -
ETA: Title said login, I meant logic. Not tackling login yet Hey guys. For fun I decided to try to make a web game using CakePHP. For now I'm completely ignoring the user login and just trying to build it as if one user is logged in. So the user has a Map they can see (it will be a 5x5 grid, with them in the center). When they load their map page, I want it to generate some random things onto the map (so placing them in random spots in the 5x5 grid). Then save that into the session so that the whole time they are logged in, those items are in those spots. Right now this is my maps_controller.php <?php class MapsController extends AppController { var $name = 'Maps'; function index() { $user = $this->Session->read('User'); $map = $this->Map->findById($user['User']['map_id']); $this->set('map', $map); } } ?> The logic I need to add is that after retrieving the Map (which is just an ID and name right now), to check if I have already generated the items. If not, do so, then save it into the session. THEN I need to set the whole thing into the view using $this->set(). Can someone help point me in the right direction? I am not sure where I'd put this logic...in the afterFind() maybe?
-
Where did you put it? Show your code every time. So go back and read what Wolphie and Mac said.
-
use strtoupper or lower to compare the two strings. a more effective way would be to use user ids.
-
The tubes are a wild and crazy place.
-
You keep ignoring several very good suggestions to get help. Here's another: add print_r($_POST); before your query.
-
You know, "it doesn't work" is usually the quickest way to get answers on how to fix it. /sarcasm. Why?
-
We'd need to see some of your code. One way to do it would be to before you print out the next row, check if the difference between that and the last is over one hour. If it is, do a while() to create $0 rows for each hour missing.