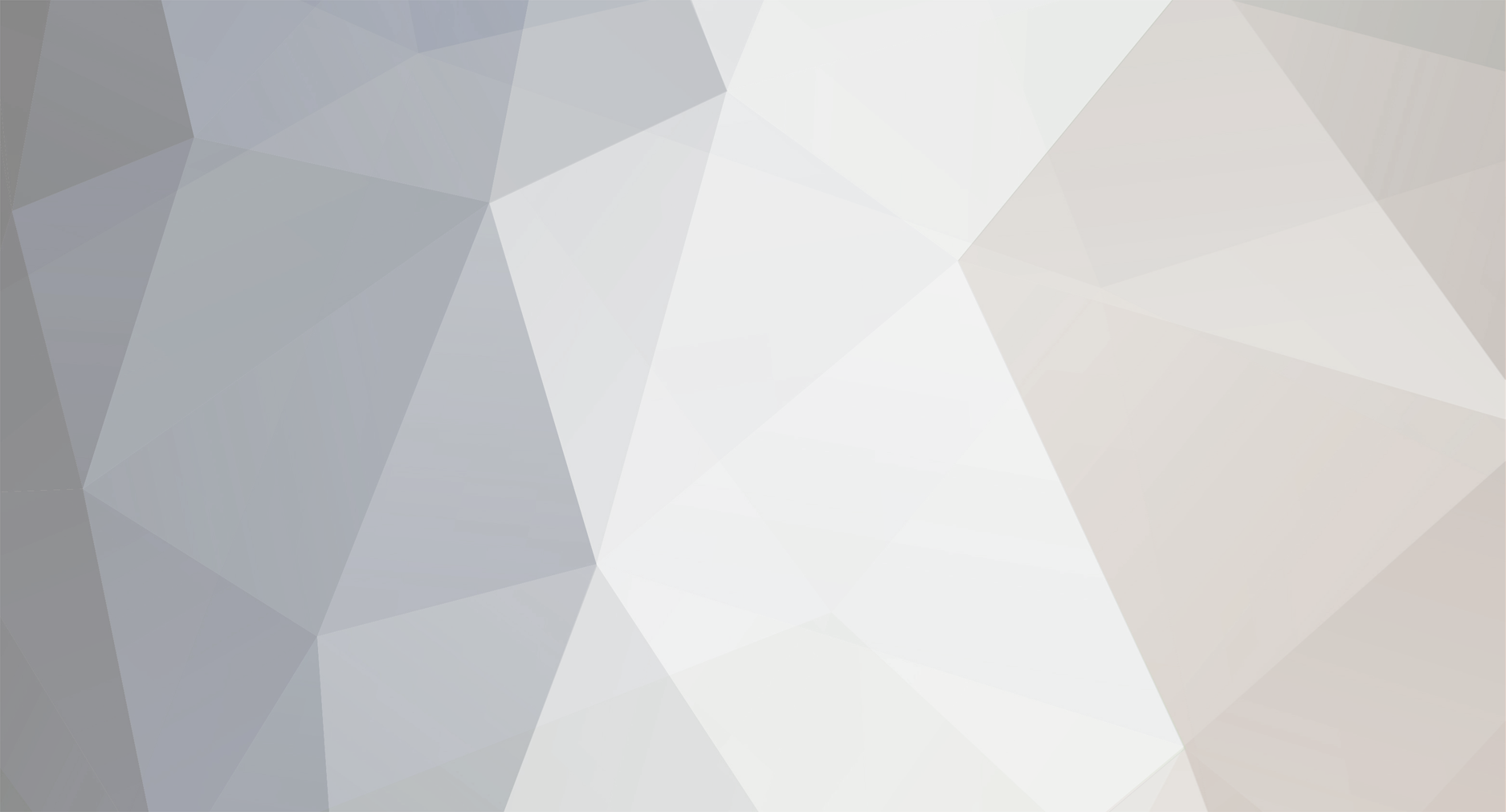
wickning1
Members-
Posts
405 -
Joined
-
Last visited
Never
Everything posted by wickning1
-
You can turn off cookies in any browser. Perhaps your version of IE has the ability to receive cookies turned off. More browsers will accept a cookie if you DO NOT give it an expiration date. That type of cookie is deleted as soon as the browser window closes. Two points: 1) Cookies are an unreliable solution for sessions in general. You need a backup plan, usually placing a session identifier in the URL for every link on your site. 2) Sending a username and a password in a cookie is not very secure. The preferred method is to send a random string of characters that can be matched up on your end with the correct username.
-
Glad you're passing along the wisdom joe. :)
-
Your join conditions should always be true. [code]SELECT p.id, p.name, p.surname, p.email, c.start AS beginning, c.end AS finish, c.name AS title, c.event AS description, c.id AS eventid FROM `calendar` c, `attendance` a, `players` p WHERE (a.event = c.id AND a.player = p.id AND a.present = "yes") AND ( c.start BETWEEN "1141060879" AND "1141665679" OR c.added BETWEEN "1140974479" AND "1141060879" ) ORDER BY p.id,c.start ASC LIMIT 0 , 30[/code]
-
Why make MySQL evaluate a condition that doesn't have semantic meaning? I always do it something like this: [code]$client = $_POST['client']; if ($client != 'All') $whereclause .= 'col_clientName=' . $client; // add more conditions if ($whereclause) $whereclause = "WHERE " . $whereclause; mysql_select_db($mysqldatabase, $mysqlconnection); $testQuery = "SELECT * FROM tbl_test1 $whereclause"; $testResult = mysql_query($testQuery) or die('Error, query failed');[/code]
-
How was the database generated? Did you have to do it by hand or was it generated by an IPB configuration script? It looks to me like the column `task_id` in table `ibf_task_manager` was not set to AUTO_INCREMENT, but I am working off of very limited information here.
-
Try this: [code]SELECT DISTINCT id, desc FROM options WHERE id = '$thisOptionID' COLLATE latin1_general_cs[/code] This will break the indexing, but if it works for you, you can set the `id` column to the latin1_general_cs collation permanently with ALTER TABLE. This would allow you to create a case sensitive index, and you could go back to using your original query.
-
When you do it in the join, you are losing user_id information because you are grouping on illustrator_id. I am trying to discern what you want from this query. I'm guessing that you want to know how many people have selected the user 1699 as one of their favorite illustrators. Is that correct? [code]SELECT a.* , b.avg, b.total_votes, c.clicks, d.* , e.total_favorites FROM illustrators_table a LEFT JOIN users d ON a.user_id = d.user_id LEFT JOIN ( SELECT user_id, AVG(score) AS avg, COUNT(*) AS total_votes FROM score_table WHERE user_id="1699" GROUP BY user_id ) b ON a.user_id = b.user_id LEFT JOIN ( SELECT user_id, COUNT( * ) AS clicks FROM external_url WHERE str_date > curdate( ) - INTERVAL 14 DAY AND user_id="1699" GROUP BY user_id ) c ON a.user_id = c.user_id LEFT JOIN ( SELECT illustrator_id, COUNT(*) AS total_favorites FROM favorites WHERE illustrator_id="1699" GROUP BY illustrator_id ) e ON a.user_id = e.illustrator_id WHERE visible = "t" && s_verified = "t" && a.user_id="1699"[/code]
-
That won't work, but this will: [code]$sql = "SELECT * FROM AAForm WHERE Honor_Level='$honor' AND Favourite_map_1 IN ('$map1', '$map2', '$map3') AND Favourite_map_2 IN ('$map1', '$map2', '$map3') AND Favourite_map_3 IN ('$map1', '$map2', '$map3') ORDER BY Honor_Level ASC";[/code]
-
/<p>((A |An |The )?)<b>Theodore Roosevelt<\/b>([^\.]*Jr\.)?[^\.]*(\.|\?|!)/i
-
CASE is a reserved word. Try this: [code]SELECT * FROM customer cu LEFT JOIN `case` c ON cu.custID = c.custID[/code]
-
Most web hosts offer to set up an installation of osCommerce, an open source web package for running an online store. It's a very popular solution for small time operations. Even some bigtime operations start with osCommerce and hire programmers to modify it. As far as credit card processing, authorize.net is one of the most popular services. You send them the data over HTTP post and they process the payment and send you the results. There are several modules for osCommerce that can automatically communicate with authorize.net. Of course, there are many other payment processors, look around and try to find the best deal.
-
That's too much logic to be automatic. How is it supposed to discern between an abbreviation and the end of a sentence? Regexes are powerful but they can't read English. You could add in some things to ignore, like "Jr.", but your regex would have to keep growing as you add more. example: /<p>((A |An |The )?)<b>Theodore Roosevelt<\/b>([^\.]|Jr\.)*(\.|\?|!)/i
-
Generally you set up a cron job, but that's on linux. On WinXP it'd be the scheduler or something. I always write my cron jobs in Perl, I don't know anything about PHP on the command line.
-
You're not allowed to name a column with a number. It must start with an alphabet character. Why call it "1"? Why not 'voted' or something like that? Also your database structure sounds ill-thought out. You have a table for every movie? So every time you add a movie, you add a table to the database? That's not good design. Why not 3 tables: movies (id, title, description) users (id, ipaddress) votes (userid, movieid)
-
You'd have to store the salt in MySQL, which would compromise it.
-
It's impossible to say for certain without looking over the code. Could they just be users making errors?
-
$row=mysql_fetch_array($query); Delete that line from above your while loop.
-
Ask your host about the security situation. If they've given you a database on their mysql server and an account that can access it, you should be able to ask them to limit access so that only your web server has permission to send queries.
-
They are reserved words, they need to be backticked (`columnname`). That will not cause random instability though. I'm leaning towards hardware failure on this one, but you can try a fresh MySQL installation.
-
To encode something: UPDATE table SET passwordcolumn = ENCODE("thePassword", "yourSalt") WHERE id=1; To decode it: SELECT DECODE(passwordcolumn, "yourSalt") FROM table WHERE id=1; You must choose a salt, that is your secret key for decoding the string. If there was no secret key, and all you had to do to decode it was say DECODE(passwordcolumn), then anybody could do it, and there'd be no point encrypting it. Clear?
-
I don't think you can avoid the loop on that one. If you're using mysqli you can use prepared statements to cache the query with MySQL and get a speed boost. You can even build it into that database class you're using. That's what I did with mine. You could also use mysqli_multi_query() to send them all at once, again mysqli only. I cleaned up the PHP a little bit so I could actually read what was going on: [code]<?php $sql = 'SELECT profile_id, profile_name, profile_description FROM '.PROFILE_FIELDS_TABLE; $fields = $db->db_query($sql); foreach ($fields as $field) { $field['value'] = $_POST[$field['profile_id']]; $sql2 = 'UPDATE '.USER_PROFILE_FIELDS_TABLE. ' SET profile_value = "'.$field['value'].'" WHERE user_id = "'.$auth->userdata['user_id'].'" AND profile_id = "'.$field['profile_id'].'"'; $db->db_action($sql2); } ?>[/code]
-
$db = "framedata"; MySQL will know where to look for the actual file.
-
Heh whoops, keys is a reserved word, use mykeys.
-
Your MySQL server should only be accepting connections from a very small number of known IPs (or domain names). Additionally, you can firewall the MySQL port and only access the database from the local machine or inside your own network. If you set it up correctly, you could put your username and password on the evening news and not be worried. Just watch out for other kinds of attacks that could compromise your web server machine. Insertion attacks, buffer overflows, trojans, etc.
-
I suspected that you'd done something like that, that's why I recommended the dump & restore. Theoretically you should be able to copy the files, but there are several known issues when you do it between different versions, especially between 4 and 5. The bigger the version jump, the more likely to have problems.