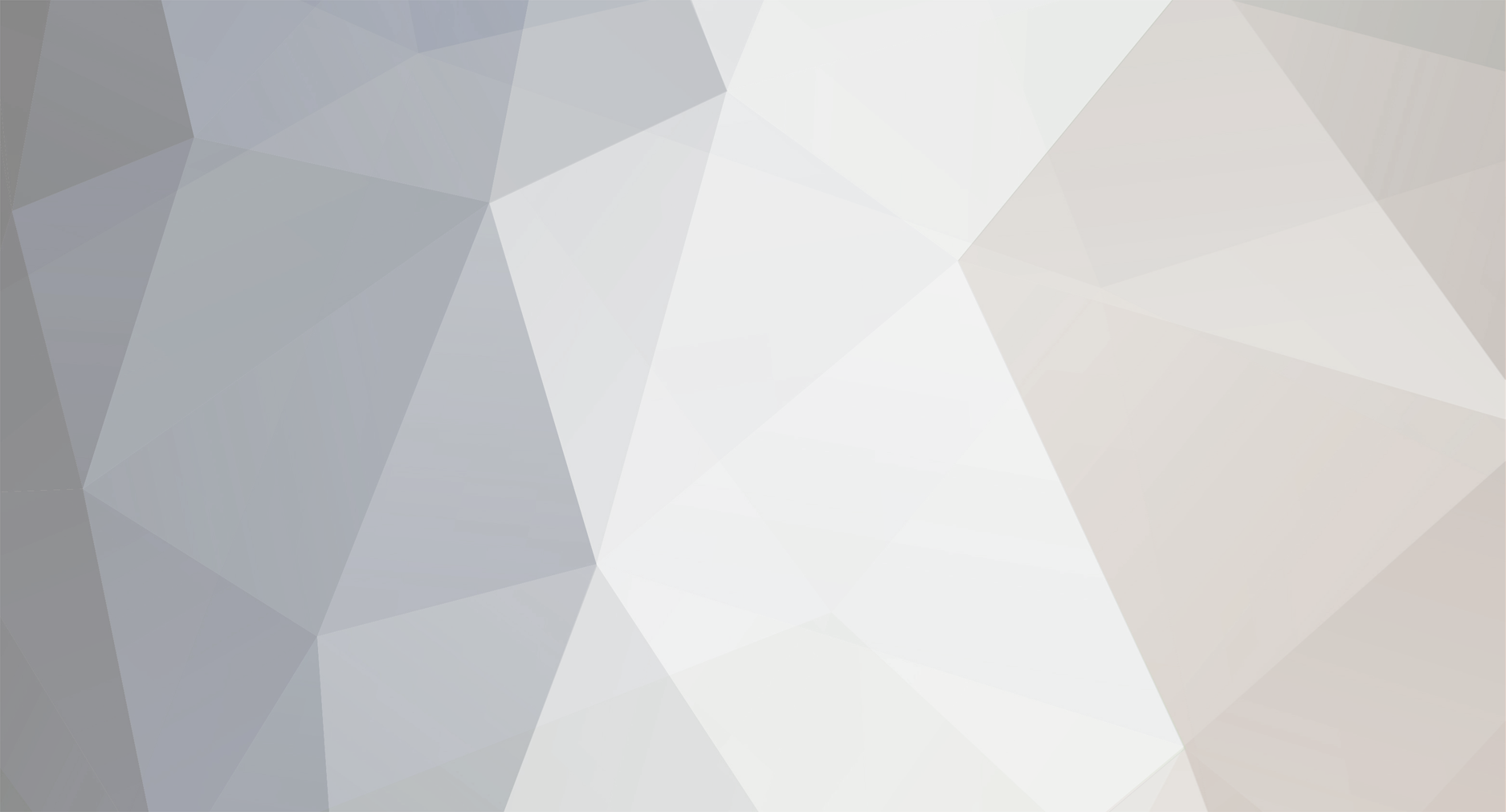
wickning1
Members-
Posts
405 -
Joined
-
Last visited
Never
Everything posted by wickning1
-
Too many database queries load my page too slow
wickning1 replied to algarve4me's topic in PHP Coding Help
You're still not using SELECT COUNT(*). Your approach is fundamentally flawed. You are making mysql return all the data in your database when all you need is one number. [code]$res = mysql_query("SELECT COUNT(*) as cnt FROM table WHERE country='Portugal' and rentaltype='holiday rental' and foto='y'"); $row=mysql_fetch_assoc($res); mysql_free_result($res); $pgl_1 = $row['cnt']; $res = mysql_query("SELECT COUNT(*) as cnt FROM table WHERE country='Portugal' and propertytype='villa' and rentaltype='holiday rental' and foto='y'"); $row=mysql_fetch_assoc($res); mysql_free_result($res); $pgl_v=$row['cnt']; $res = mysql_query("SELECT totalbedrooms as beds, COUNT(*) as cnt FROM table WHERE country='Portugal' and propertytype='villa' and rentaltype='holiday rental' and totalbedrooms BETWEEN 1 AND 6 AND foto='y' GROUP BY totalbedrooms ORDER BY totalbedrooms"); while ($row=mysql_fetch_assoc($res)) { $varname = "pgl_v_" . $row['beds']; $$varname = $row['cnt']; } echo $pgl_1 . ' ' . $pgl_v . ' ' . $pgl_v_1 . ' ' . $pgl_v_2 . ' ' . $pgl_v_3 . ' ' . $pgl_v_4 . ' ' . $pgl_v_5 . ' ' . $pgl_v_6;[/code] -
Is it firewalled?
-
WHERE week BETWEEN 13 AND 52
-
trouble wrapping my head around using many-many tables
wickning1 replied to SpectralDesign.Net's topic in MySQL Help
I think you didn't quite understand or think through what I said. I said to store the role with name_address.. that would make it BECOME name_address_role. If you have name_address and name_address_role, you are duplicating name_address. How could you have a role at an address you're not linked to? -
preg_match_all optimizing (not working probably)
wickning1 replied to hero's topic in PHP Coding Help
Use (.*?) instead of (.*). Other than that your regex is fine. Use something like this to get your values out: [code]<?php foreach ($found as $match) { echo $match[1] . "<br>\n"; } ?>[/code] -
Dude, I knew you could pick up chicks in an XML file! Seriously though, after reading through that website it seems that the code posted there does not support extracting those tags. I haven't had to parse XML with PHP yet but I may investigate and see if I can be of more help later.
-
Too many database queries load my page too slow
wickning1 replied to algarve4me's topic in PHP Coding Help
Yeah, I was afraid it might not work perfectly. You can just do each query separately (remove the UNIONs and do 4 queries), and that should be better. -
That's the character set you want to have as the default on every MySQL table you create. Once it's set as the default for a table, you won't have to worry about it when you pull or store values.
-
This will sort based on the second word (won't catch middle names correctly). [code]SELECT * from catalog ORDER BY SUBSTR(LTRIM(fullname), LOCATE(' ',LTRIM(fullname)))[/code]
-
trouble wrapping my head around using many-many tables
wickning1 replied to SpectralDesign.Net's topic in MySQL Help
Yeah, I would probably create a roles table and then store links to it in the name_address table. It looks like address_role should definitely come out of the address table; it just doesn't belong there. -
Yes, that's exactly the problem, you're multiplying by $rows_per_page before you've set it to 30!
-
While logic on SQL handle cracking me up. Plzz help :(
wickning1 replied to Lt Llama's topic in PHP Coding Help
Try changing the query to this: [code]SELECT s1.nickNames, s1.mapName, s1.`curDate`, s1.runTime FROM skillmaps s1 INNER JOIN (SELECT mapName, MIN(runTime) as bestrunTime FROM skillmaps GROUP BY mapName) s2 ON s1.runTime=s2.bestrunTime AND s1.mapName=s2.mapName ORDER BY mapName ASC[/code] Also add "echo mysql_error();" right after "$result=mysql_query($query);" and report back with what it prints out. If you are using an old version of mysql (<4.1) then this won't work at all, since pre-4.1 didn't support subqueries. -
trouble wrapping my head around using many-many tables
wickning1 replied to SpectralDesign.Net's topic in MySQL Help
See how this works for you. I think the big thing you were forgetting was that you only want phone numbers for each name and address both. For instance, when you print out Joe and the address for Joe's apartment, you only want to print phone numbers for reaching Joe at his apartment. You'll print out his summer home and its phone numbers later. A few joins helped clean up the code, too. [code]<?php // if a client has been selected and submitted, view the //entire set of records related to that client... if ( $_POST['submit'] == "view" ) { $id_name = $_POST['client']; // grab the client names and title $name_populate = mysql_query("SELECT * FROM names WHERE name_id='$id_name'") or die('ERROR: '.mysql_error()); $row = mysql_fetch_assoc($name_populate); $f_name = $row['name_first']; $l_name = $row['name_last']; $m_name = $row['name_middle']; $title_name = $row['name_title']; ?> <div id="main"> <div class="content"> <hr class="hide" /> <h2>Client Records</h2> <table summary="client records" border="0" cellpadding="4"> <tr><td class="left"><h3> <?php echo $title_name." ".$l_name.", ".$f_name." ".$m_name ?> </h3></td></tr> <?php // grab all addresses linked to the selected client $address_id_populate = mysql_query("SELECT a.*" . "FROM addresses a INNER JOIN name_address n USING (address_id)" . "WHERE n.name_id='$id_name'") or die('ERROR: '.mysql_error()); while ($row = mysql_fetch_assoc($address_populate)) { $street_address = $row['address_street']; $suite_address = $row['address_suite']; $city_address = $row['address_city']; $province_address = $row['address_province']; $postcode_address = $row['address_postcode']; $role_address = $row['address_role']; $id_address = $row['address_id']; // present the address to the client-application... echo '<tr><td class="left">' . $role_address . '<br />' . $street_address . ' ' . $suite_address . '<br />' . $city_address . ', ' . $province_address . '<br />' . $postcode_address . '<br />'; // grab the phone records linked to the selected client at // the selected address $name_phone_id_populate = mysql_query("SELECT p.*" . "FROM phones p INNER JOIN name_phone n USING (phone_id)" . "INNER JOIN address_phone a USING (phone_id)" . "WHERE n.name_id='$id_name' AND a.address_id='$id_address'") or die('ERROR: '.mysql_error()); while ($row = mysql_fetch_assoc($name_phone_id_populate)) { $id_phone = $row['phone_id']; $number_phone = $row['phone_number']; $extension_phone = $row['phone_extension']; $desc_phone = $row['phone_description']; // present each phone record to the client-application echo "<br />" . $desc_phone . " - " . $number_phone . " extension: " . $extension_phone . "<br />"; } } ?> <tr><td><br /> <form method="post" action="<?php echo $_SERVER['PHP_SELF']?>"> <input type="submit" name="submit" alt="new search" value="new search" /> </form></td></tr> </table> <?php include_once $_SERVER['DOCUMENT_ROOT'].$basedir.'includes/foot.inc'; } // end of case where client selection has been submitted ?>[/code] -
I believe foreign keys are supported if you create an InnoDB table, but MyISAM does not support them. You can read about the various differences between InnoDB and MyISAM in the mysql documentation.
-
While logic on SQL handle cracking me up. Plzz help :(
wickning1 replied to Lt Llama's topic in PHP Coding Help
See if this works for you: [code]<?php include("dbinfo.inc.php"); mysql_connect($host,$username,$password); mysql_select_db($database) or die( "Unable to select database"); $query="SELECT s1.nickNames, s1.mapName, s1.`curDate`, s1.runTime FROM skillmaps s1 INNER JOIN (SELECT mapName, MIN(runTime) as runTime FROM skillmaps GROUP BY mapName) s2 ON s1.runTime=s2.runTime AND s1.mapName=s2.mapName ORDER BY mapName ASC"; $result=mysql_query($query); mysql_close(); echo "<b><br><center><u><font face=verdana size=3 color=F4A21B>Skill@TFC4ever.de - Speed Run Records</b></u></font><br><br><br>"; ?> <title>Skill@TFC4ever.de - Speed run records</title> <head> <STYLE type="text/css"> BODY {scrollbar-3dlight-color:#2E8B57; scrollbar-arrow-color:#F4A21B; scrollbar-base-color:#4D5546; scrollbar-track-color:#33382E; scrollbar-darkshadow-color:#000000; scrollbar-face-color:#4D5546; scrollbar-highlight-color:#000000; scrollbar-shadow-color:#404040;} </STYLE> </head> <BODY BGCOLOR="#4D5546" TEXT="#FFFFFF" LINK="#ff9900" VLINK="#ff9900" ALINK="#ff9900" leftmargin=0 rightmargin=0 topmargin=0 bottommargin=0 marginheight=0 marginwidth=0> <div align="center"> <table border="1" bordercolor="#000000" cellspacing="0" cellpadding="0" width="570" style="border-collapse: collapse"> <tr> <th align="center" bgcolor="#403F2E"><font face="verdana, Arial, Helvetica, sans-serif" size="2"> Mapname </font></th> <th align="center" bgcolor="#403F2E"><font face="verdana, Arial, Helvetica, sans-serif" size="2" color="#00FF00"> Nickname </font></th> <th align="center" bgcolor="#403F2E"><font face="verdana, Arial, Helvetica, sans-serif" size="2" color="#00FFFF"> Record Date </font></th> <th align="center" bgcolor="#403F2E"><font face="verdana, Arial, Helvetica, sans-serif" size="2" color="#FF0000"> Runtime </font></th> </tr> <? while ($row = mysql_fetch_array($result)) { $recordtime = $row['runTime']; $mapname= $row['mapName']; $nickname = $row['nickNames']; $curdate = $row['curDate']; if ($recordtime > 0) { $hours = ($recordtime / 3600) % 24; if ($hours < 10) $hours = "0" . $hours; $minutes = ($recordtime / 60) % 60; if ($minutes < 10) $minutes = "0" . $minutes; $seconds = $recordtime % 60; if ($seconds < 10) $seconds = "0" . $seconds; $formatTime = $hours . ":" . $minutes . ":" . $seconds; ?> <tr> <td align="left" bgcolor="#4C5844"><font face="verdana, Arial, Helvetica, sans-serif" size="2"><? echo $mapname; ?></font></td> <td align="center" bgcolor="#4c4d43"><font face="verdana, Arial, Helvetica, sans-serif" size="2"><? echo $nickname; ?></font></td> <td align="center" bgcolor="#4C5844"><font face="verdana, Arial, Helvetica, sans-serif" size="2"><? echo $curdate; ?></font></td> <td align="center" bgcolor="#4c4d43"><font face="verdana, Arial, Helvetica, sans-serif" size="2"><? echo $formatTime; ?></font></td> </tr> <? } } echo "</table>"; ?>[/code] -
Too many database queries load my page too slow
wickning1 replied to algarve4me's topic in PHP Coding Help
"Also, where do you have your mysql_close();? You could try to close and open db after each query maybe." Bad advice! This will slow things down considerably. As for the code, this might work for you: [code]$sql = "(SELECT COUNT(*) as cnt FROM table WHERE country='Portugal' and rentaltype='holiday rental' and foto='y')" . "UNION" . "(SELECT COUNT(*) as cnt FROM table WHERE country='Portugal' and propertytype='villa' and rentaltype='holiday rental' and foto='y')" . "UNION" . "(SELECT COUNT(*) as cnt FROM table WHERE country='Portugal' and propertytype='villa' and rentaltype='holiday rental' and totalbedrooms='1' and foto='y')" . "UNION" . "(SELECT COUNT(*) as cnt FROM table WHERE country='Portugal' and propertytype='villa' and rentaltype='holiday rental' and totalbedrooms='2' and foto='y')"; $result = mysql_query($sql); $r = mysql_fetch_assoc($result); $n_pgl_1=$r['cnt']; $r = mysql_fetch_assoc($result); $n_pgl_v=$r['cnt']; $r = mysql_fetch_assoc($result); $n_pgl_v_1=$r['cnt']; $r = mysql_fetch_assoc($result); $n_pgl_v_2=$r['cnt'];[/code] -
Use uasort() instead of usort().
-
"function counting();" just lose the semicolon: function counting()
-
If it's a really long process, you probably don't want to make your user(s) wait. You should be able to send them to an intermediate page while they wait, one with an animated gif or something, and set it to redirect to a "completed" page after a period of time.
-
As far as I know, it's not possible, at least on the PHP end. Apache doesn't call the script until it's gotten the whole message. There may be an HTML/Javascript solution, but I don't know of one.
-
Yep
-
trouble wrapping my head around using many-many tables
wickning1 replied to SpectralDesign.Net's topic in MySQL Help
Wow. I don't have time to parse all that at the moment but I'll post later if someone doesn't beat me to it. -
You can store it in a cookie or put "?code=$code" after all of your links. Sessions are a way of storing lots of persistent information using a cookie or a variable on every link. PHP can automate the process somewhat. You can look into that as well.
-
I see your point. I'd still change the query a little bit for clarity, and removing the LIMIT 50, as that will limit you to only the first two users (and the second one will have an incomplete total). [code]SELECT tips.username, tips.round SUM(tips.tip='draw' AND fixtures.result='draw' OR tips.tip=fixtures.result) as points FROM fixtures JOIN tips on (fixtures.round=tips.round AND fixtures.game=tips.game) GROUP BY tips.username, tips.round[/code] And then I'd do the code like this. Switch the 1 and -1 in mysort() to reverse the sort. [code]<?php $userPoints = array(); while ($row = mysql_fetch_assoc($result)) { $userPoints[$row['username']][$row['round']] = $row['points']; $userPoints[$row['username']]['total'] += $row['points']; } function mysort ($a, $b) { return ($a['total'] == $b['total'] ? 0 : ($a['total'] > $b['total'] ? -1 : 1)); } usort ($userPoints, "mysort"); foreach($userPoints as $username => $rounds) { echo "<tr>"; echo "<td>".$username."</td>"; for($i = 1; $i < 27; $i++) { echo "<td>".($rounds[$i] ? $rounds[$i] : "-")."</td>"; } echo "<td><strong>".$rounds['total']."</strong></td>"; echo "</tr>"; } ?>[/code]