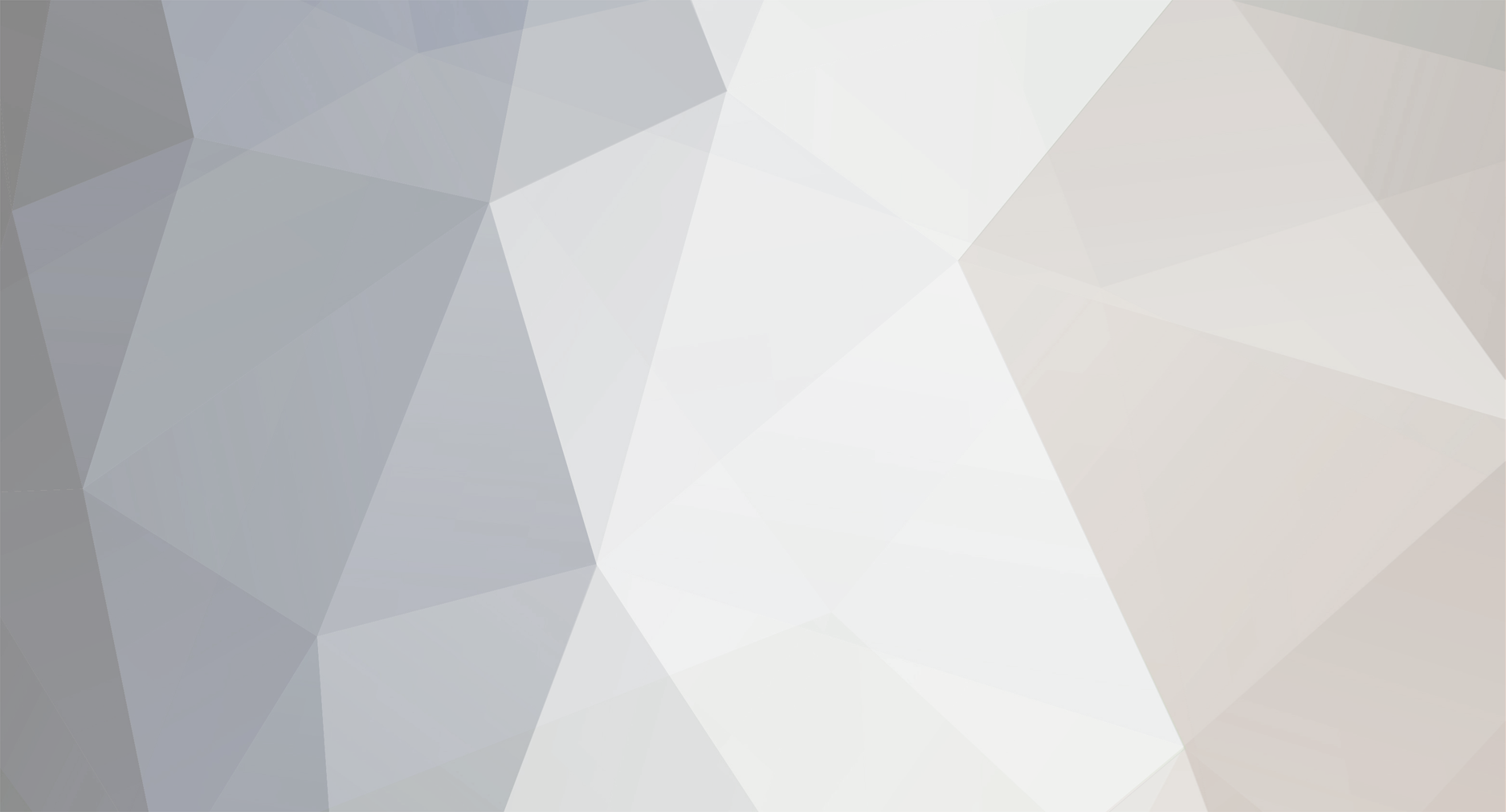
wickning1
Members-
Posts
405 -
Joined
-
Last visited
Never
Everything posted by wickning1
-
proper syntax for if else statements with queries on mutiple tables
wickning1 replied to dsaba's topic in MySQL Help
$result = mysql_query("SELECT map_name FROM maps WHERE map_name='$mname'"); $row = mysql_fetch_assoc($result); if ($row['map_name'] == $mname) { // ... do all the rest of the stuff -
It's possible, it's easy. SELECT * FROM table WHERE `field1` LIKE CONCAT('%', `field2`)
-
Your employer can easily set up the company gateway to log all web traffic. You can't erase that without access. The only way to ensure your privacy is to make a secure connection to a trusted proxy BEFORE you browse. Of course, your employer may be curious about all the secure traffic to an off-site machine, but it's somewhat rare for someone to watch things that closely.
-
I just did something very similar. Here's what I wrote. It's not well tested but should be working. [code]<?php $tags = array('b', 'i', 'h\d'); function preserveHTML($text, $tags) { global $tags; foreach ($tags as $tag) { $text = preg_replace('/<(\/)?(' . $tag . '(\s.*?)?)>/i', '[$1$2]', $text); } return $text; } function restoreHTML($text, $tags) { global $tags; foreach ($tags as $tag) { $text = preg_replace('/\[(\/)?(' . $tag . '(\s.*?)?)\]/i', '<$1$2>', $text); } return $text; } ?>[/code]
-
No, auto increment is great, fabulous, fundamental, can't live without it. Just don't fiddle with the id once it's been assigned. Don't try to fix gaps or make the numbers "pretty".
-
proper syntax for if else statements with queries on mutiple tables
wickning1 replied to dsaba's topic in MySQL Help
Yeah, you'll never be able to insert a map with that script. You need to actually check the database for $mname for that first if() statement. What you're doing now is just checking that the user sent a map, which will always be true. What you want to do is find out if what the user sent *matches* something in the database. Then you know whether you should UPDATE OR INSERT. You're constantly trying to UPDATE a map that doesn't exist. As far as reference material, you can't beat mysql.com and php.net. The only other references you'll ever need are tutorials, which are a dime a dozen. There's quite a few here at PHPFreaks, just look around. -
Too many database queries load my page too slow
wickning1 replied to algarve4me's topic in PHP Coding Help
Look, when you use COUNT(*), MySQL sends one little integer back to you over the internet. It doesn't even have to do a disk read to get that number because all the information it needs is sitting in memory in an index. When you select all the rows, MySQL has to retrieve all the thousands of rows of data from the hard disk, send you that data over the internet, and when they all finally arrive, you use PHP to count how many. By all that is holy, there is no way in hell that selecting all the rows is faster. In any universe, even the evil one where Spock has a beard. The only time you should EVER use mysql_num_rows is if you need all the data AND a count. You just need a count, so don't use it. Btw, if you ask a mysql developer about this, he will most likely laugh at you. Hard. So be prepared. :) -
proper syntax for if else statements with queries on mutiple tables
wickning1 replied to dsaba's topic in MySQL Help
You can't just throw two queries together the way you are doing, you're going to have to call mysql_query() twice. You're not even concatenating them both into $query correctly, that's just bad PHP. [code]if($mname) { $query = "UPDATE `maps` SET `map_author`='$mauthor', `map_description`='$mdescription', `mapmode_chaos`='$chaos', `mapmode_sts`='$sts', `mapmode_predator`='$predator', `map_template`='$mtemplate', `map_brushset`='$mbrushset', `map_dateadded`='$date', `map_email`='$email', `map_ip`='$userip', `map_submitter`='$sname' WHERE `map_name`='$mname'"; $query2 = "UPDATE `comments` SET `comment_text`='$mcomments', `comment_dateadded`='$datetime', `comment_name`='$sname', `comment_ip`='$userip' WHERE `map_name`='$mname'"; } else { $query = "INSERT INTO `maps` ( `map_name`, `map_author`, `map_description`, `mapmode_chaos`, `mapmode_sts`, `mapmode_predator`, `map_template`, `map_brushset`, `map_dateadded`, `map_email`, `map_ip`, `map_submitter` ) VALUES ( '$mname','$mauthor','$mdescription','$chaos','$sts','$predator','$mtemplate', '$mbrushset','$date','$email','$userip','$sname' )"; $query2 = "INSERT INTO `comments` ( `map_name`, `comment_dateadded`, `comment_name`, `comment_text`, `comment_ip` ) VALUES ( '$mname', '$datetime', '$sname', '$mcomments', '$userip' )"; } //data is now in the database $results = mysql_query( $query ); $results2 = mysql_query( $query2 ); //success statement based on results if( $results1 && $results2 ) { echo( "You have succesfully submitted a map into the database! With your help XboxStuff.org is becoming the largest Farcry Map database known to man!"); } else { die( "There was a problem with the file you uploaded or the data you submitted. Try resubmitting again, if problem persists post an error ticket here" . mysql_error() ); }[/code] -
I'm confused, are you getting those errors when you're the only one on your site? Or do you already have a sizable audience? Are you renting a shared server? Your host may be limiting your account to try to ensure quality of service for everyone on that machine, or it could just be misconfigured. In general, MySQL has a lot of performance parameters to help keep your machine from crashing, but if you tune those parameters, it is only limited by the hardware capabilities. Compared with other database solutions, MySQL is one of the best performers around, so you're not going to do much better with the same hardware. If you're looking at very high traffic, you may have to look into multi-server load balancing. Some webhosts will manage that kind of thing for you (for a price). Very few sites ever really push a modern server machine to its limits unless they are writing bad queries. The first thing you should do when you are experiencing performance problems is start tracking down slow queries and find ways to speed them up.
-
Same for me, also Firefox 1.5. The warning you're getting about the result is because the query is erroring out. Echo mysql_error() right after mysql_query() to see what the problem is.
-
I don't really know what the Zend framework is either, but I'm an excellent PHP programmer. :) I do have a faint idea though. Zend is the base engine upon which PHP is built, it's sort of a sub-project, like the kernel of an OS. It provides a lot of common functions and a general framework for the people who write the PHP engine. You don't really need to worry about it when writing PHP scripts.
-
Is ID a primary key? Auto-increment? Why are you querying both ID and Name? Isn't ID enough? You say you have an index on the table, which columns are indexed? Are they indexed separately or as part of a multi-index?
-
Put the <tr> before the loop, the </tr> after the loop, and <td></td> around each element inside the loop.
-
Too many database queries load my page too slow
wickning1 replied to algarve4me's topic in PHP Coding Help
[!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]It seems that to use COUNT is quicker than to use mysql_num_rows (although there is still some debate out there on some of the forums)[/quote] Debate?? From who? The insane code-monkey squad? SELECT COUNT(*) will always be faster than returning the whole dataset if you're after a count. Always. No exceptions. Do not pass Go, do not collect $200, do not feed the trolls. -
If you don't want the page to reload, you have to write it in Javascript, or use Javascript to pull off an AJAX maneuver. Not to put too sharp a point on it, but there's another forum for that. :)
-
There's nothing wrong with ANY of the queries in this thread save the first ones you posted. I loaded your test data and get expected results with my query, your query, and without the "c.start stuff". Somehow when you "remove the c.start stuff" you are doing something very wrong and confusing yourself. I don't know what else to tell you. The query is correct.
-
Use javascript to pop up a new window that loads a PHP script when the select box is changed.
-
[code]echo "<td><a href=\"http://www.xxx.com/cart.php?action=add&item=".$row[product_id]."\" target=\"_blank\" onMouseOver=\"window.status='Add To Cart!'; return true;\" onMouseOut=\"window.status=''; return true;\" onClick=\"window.open(this.href, 0, ' directories=no, location=no, menubar=no, status=no, titlebar=no, toolbar=no, scrollbars=yes, width=700, height=400, top=5, left=5, resizable=yes'); return false\">Add To Cart!</a></td>\n";[/code]
-
What column type is date_date? Is it a string type or a date type?
-
RegEx limiting result lines by 21 characters
wickning1 replied to MarkBad311's topic in PHP Coding Help
Heh, sorry, I was just kidding. :) I used the Perl Cookbook and Programming Perl when I was learning regular expressions, but these days I have no need for such things. -
[a href=\"http://www.phpfreaks.com/tutorial_cat/25/Page-Number--Pagination.php\" target=\"_blank\"]http://www.phpfreaks.com/tutorial_cat/25/P...-Pagination.php[/a]
-
InnoDB appears to go faster because it uses insert buffering. Basically it updates the index after it sends a reply saying the insert was successful. Updating the index is what makes inserts take as long as they do. Under InnoDB, the database continues to work after you think you're done. Choosing properly between MyISAM and InnoDB is somewhat involved. I suggest you stick with MyISAM until you know the difference. Now, back to the matter at hand, you SHOULD be preprocessing your input values and concatenating them all into the same string. Only ONE query is required, not 530. Your insert query should look something like this: [code]INSERT INTO table (columnname) VALUES ('row1'), ('row2'), ('row3'), (...)[/code]
-
Too many database queries load my page too slow
wickning1 replied to algarve4me's topic in PHP Coding Help
Why post here if you're not even going to read the replies? USE SELECT COUNT(*) FOR PETE'S SAKE! -
Your structure is fine. Zawadi's code is a little weird. Here's how I would write it: [code]$albums= mysql_query("select * from albums ORDER BY albums_ID"); while ($album = mysql_fetch_assoc($albums)) { echo 'Album: ' . $album['album_name']; $tracks = mysql_query("select t.* FROM tracks t INNER JOIN albums_tracks a ON t.track_ID=a.track_ID WHERE a.albums_ID='$album[albums_ID]'"); while ($track = mysql_fetch_assoc($tracks)) { echo ' ' . $track['track_name']; } }[/code]
-
You don't have an ending double quote. [code]$query_food = "SELECT s.* FROM stock s INNER JOIN stock_item i ON s.modelNum = i.modelNum WHERE i.prodName = '$_POST[interest]'"; $result = mysql_query($query_food,$connect) or die ("query_food: ".mysql_error($connect));[/code]