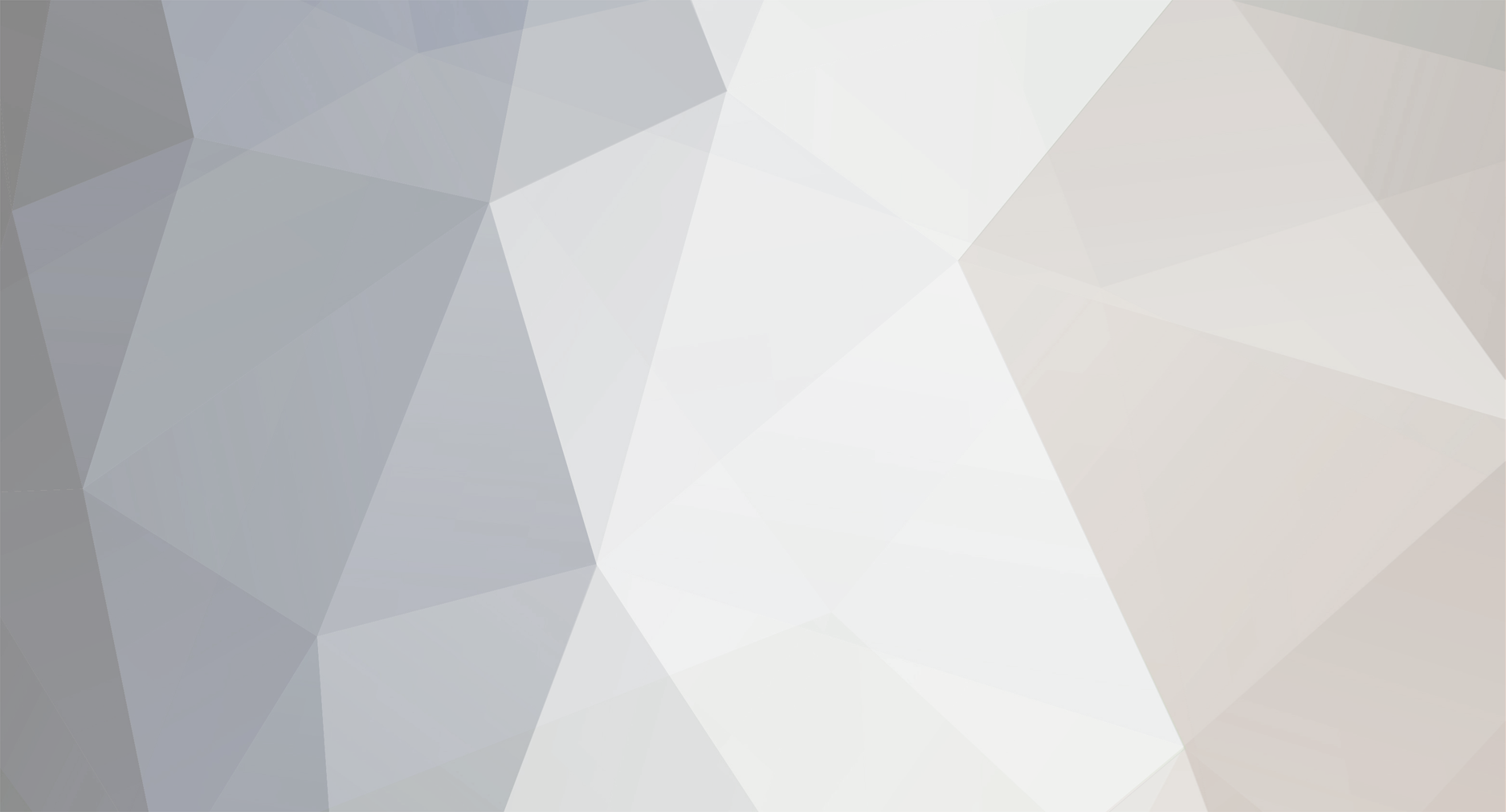
wickning1
Members-
Posts
405 -
Joined
-
Last visited
Never
Everything posted by wickning1
-
You can set up a PHP script to feed the browser a download instead of a web page. You just need to set the content-type correctly with the header() function. This script can also record the fact that it was run. See this page from the PHP documentation for more information: [a href=\"http://us3.php.net/manual/en/function.header.php\" target=\"_blank\"]http://us3.php.net/manual/en/function.header.php[/a]
-
Sounds like your web server isn't configured correctly. Apache needs to know to treat .php files differently from the rest. Check the PHP documentation for how to install correctly.
-
MySQL query and foreach loop not acting as wanted
wickning1 replied to TRobs's topic in PHP Coding Help
mysql_fetch_array() only returns one row at a time. You have to use something like while ($leader = mysql_fetch_array($member_info_query)) { // do your output } to get all the rows. -
Is this a web page or a command line script? You can't do that with a web page. On the command line you may be able to use fseek() and fwrite() on STDOUT. I've never tried it though. Make sure it's automatically flushing output. You can also try clearing the screen with a system command just before a write.
-
You can do this on the MySQL end with GROUP BY. Post your current query and I will modify it for you.
-
<input type=text name='headline' size=63 tabindex=13 value='<?php echo htmlspecialchars($Headline); ?>'> That doesn't solve your problem?
-
Dynamic function to select mysql data based on date
wickning1 replied to tdnxxx444's topic in PHP Coding Help
if () { } elseif () { } elseif() { } .... :) Aren't those all basically date ranges? Have the user give you a start and an end, and figure out good defaults when they leave one or both empty. -
PHP error, when you concatenate the string later you end up with: SELECT price, title, id FROM tm_inventory WHERE category='hand' ORDER BY id DESCLIMIT x,y DESCLIMIT is bad mmkay?
-
Try echoing mysql_error() after the query. Does mysqld have permission to read the file in question? Is the file on the database server (instead of the web server, if they're different)? Does the file use unix-style line feeds (\n)?
-
I've never needed any more than the online documentation, it's pretty extensive. I think the best way to internalize joins and subqueries is to practice a lot. You might try finding an old used textbook that has sets of problems to solve. Many of them will be Oracle based but it doesn't really matter all that much. Or you could hang around here and try to help people. :)
-
You are still doing a join. If you have two tables in the FROM part, you are doing a join. The comma is just another way to write INNER JOIN. Be sure you know what you are joining on and that you set up the WHERE conditions appropriately. To answer your other question, there will be no error when you have duplicate return names, and you can still access them by the numeric index if you use mysql_fetch_array(), but mysql_fetch_assoc() will not work properly. Setting an alias, like SemiApoc showed you, is the almost always best way to deal with it.
-
In PHP a variable is only valid inside the function where it is created. Using global variables is a bad practice. Instead, return the name from your function: [code]function confirmUser($username, $password){ global $conn; /* Add slashes if necessary (for query) */ if(!get_magic_quotes_gpc()) { $username = addslashes($username); } $sql = "SELECT name FROM users WHERE username = '$username'"; $result = mysql_query($sql) or die (mysql_error()); $r = mysql_fetch_array($result); $rname = $r['name']; return $rname; }[/code] Then when you call the function you call it like this: [code]$rname = confirmUser($username, $password);[/code]
-
MySQL can do all of that. [code]<?php echo 'Top 5 Pages:<br>'; $query = mysql_query("SELECT page, SUM(hits) as hitcount FROM stats__pv WHERE date BETWEEN LAST_DAY(CURDATE())-INTERVAL 1 MONTH AND LAST_DAY(CURDATE()) GROUP BY page ORDER BY hitcount DESC LIMIT 5"); while($r=mysql_fetch_array($query)) { echo $r['page'] . ' - ' . $r['hitcount'] . ' hits<br>'; } ?>[/code]
-
Is magic_quotes on? If so, the addslashes() will mess up your query. Use get_magic_quotes_gpc() to find out if they are turned on.
-
I stand corrected - good to know, thanks.
-
For future reference: [a href=\"http://dev.mysql.com/doc/refman/5.0/en/reserved-words.html\" target=\"_blank\"]http://dev.mysql.com/doc/refman/5.0/en/reserved-words.html[/a]
-
Nope, even with an index, I'm 90% certain that that subquery you wrote will table scan. Ideally it shouldn't, but I know from experience, in 5.0, it still does. The parsing engine can't be absolutely sure that the subquery will be the same for every row, so it assumes that it won't be. In general, avoid putting subqueries in the WHERE clause. Put them in the FROM clause instead, where the statement will only be evaluated once.
-
You mean "SELECT COUNT(*) as total FROM pages"? Yes that will be very fast.
-
Using backticks all the time is almost always unnecessary and very cumbersome. Just avoid reserved words.
-
Are you sure the query is malfunctioning? The one I posted with MySQL date calculations looks fine to me no matter how many times I look at it. I guess the next step is to develop some test data, tell me what you expect to get from the query, and what you're actually getting.
-
Not possible to use MAX() the way you want without a subquery. Fenway's is easy but causes a potentially very slow table scan. Also, I'm assuming you would rather group by day, not by second? Grouping the pages updated in the same second doesn't seem useful. Here's two solutions for grouping on the most recent day: [code]SELECT COUNT(*) as total, DATE(date) as justdate FROM pages GROUP BY justdate ORDER BY justdate DESC LIMIT 1[/code] That one will be just as slow as fenway's. This one will run a lot faster though: [code]SELECT COUNT(*) as total FROM pages a INNER JOIN (SELECT DATE(MAX(date)) as justdate FROM pages) b ON a.date BETWEEN CAST(b.justdate as DATETIME) AND CAST(b.justdate + INTERVAL 1 DAY as DATETIME)[/code]
-
Yes, if the phonePic value depends completely on what modelNum is, you should have another table model_pic (modelNum, phonePic). Likewise for tarrif. It's still a design decision, you don't have to fully normalize.
-
Everything I see tells me the query is good. However, the test data you just gave me is not good data. The row that you insert into attendance has two bad references. If Bob is attending "Event" then it should be INSERT INTO `attendance` VALUES (10, 9, 11, 'yes'); Because Bob's id column is set to 9, and "Event" has its id column set to 11. The attendance table needs to link 9 in the player table to 11 in the calendar table. A table is not an array. You cannot use 1 and 2.
-
edit_db.php: [code]<?php mysql_connect($DBhost,$DBuser,$DBpass) or die("Unable to connect to database"); mysql_select_db("$DBName") or die("Unable to select database $DBName"); $sql = "SELECT row_number FROM weather WHERE today=CURDATE()"; //now spit out the table and rows for the table $rs = mysql_query($sql,$dbc); $noerrors = TRUE; while ($row = mysql_fetch_array($rs)) { $i = $row['row_number']; $usql = 'UPDATE weather ' . 'SET event="' . $_POST['event'.$i] . '",' . 'hrs="' . $_POST['hrs'.$i] . '",' . 'closed="' . $_POST['closed'.$i] . '",' . 'notes="' . $_POST['notes'.$i] . '" ' . 'WHERE row_number="' . $i . '"; if(!mysql_query($usql)) { $noerrors = FALSE; $msg = mysql_errno().": ".mysql_error()."<BR>"; } } if ($noerrors) { echo "<p>Thank You, your entry has been submitted!</p>" . "<meta http-equiv=Refresh content=1;url='http://www.mysite.com'>"; } else { echo $msg; } ?>[/code] edit.php: [code]<?php //do your normal mysql setup and connection calls $dbc = mysql_pconnect('xxx','xxx','xxx'); mysql_select_db('weather',$dbc); ?> <img src="/images/1360am.jpg" width="180" height="82"> <strong> <img src="/images/realcountry.jpg" width="180" height="82"> <img src="/images/mix.JPG" width="180" height="82"><br> <br> RP Broadcasting Weather Related Announcements for <?php echo date("F j, Y"); ?> </strong><br> <?php //now get stuff from a table $sql = "SELECT row_number, event, hrs, closed, notes FROM weather WHERE today=CURDATE()"; //now spit out the table and rows for the table $rs = mysql_query($sql,$dbc); ?> <form method="POST" action="edit_db.php"> <table width='100%' border='1'> <tr> <td><div align="center">Event or School</div></td> <td><div align="center">2 Hours Late?</div></td> <td><div align="center">Closed?</div></td> <td><div align="center">Notes - when rescheduled or when open</div></td> </tr> <tr> <?php while ($row = mysql_fetch_assoc($rs)) { $event = '"event' . $row['row_number'] . '"'; $hrs = '"hrs' . $row['row_number'] . '"'; $closed = '"closed' . $row['row_number'] . '"'; $notes = '"notes' . $row['row_number'] . '"'; ?> <td><div align="center"><font color="#000000"> <input name=<?php echo $event ?> type="text" id=<?php echo $event ?> size="50" maxlength="200" value="<?php echo $row['event']; ?>" /> </font></div></td> <td><div align="center"> <input name=<?php echo $hrs ?> type="text" id=<?php echo $hrs ?> size="11" maxlength="15" value="<?php echo $row['hrs']; ?>" /> </div></td> <td><div align="center"> <input name=<?php echo $closed ?> type="text" id=<?php echo $closed ?> size="7" maxlength="7" value="<?php echo $row['closed']; ?>" /> </div></td> <td><div align="center"><font color="#000000"> <input name=<?php echo $notes ?> type="text" id=<?php echo $notes ?> size="50" maxlength="200" value="<?php echo $row['notes']; ?>" /> </font></div></td> </tr> <?php } if (!mysql_num_rows($rs)) { echo "<tr><td colspan=4>There are no weather related announcements today.</td></tr>"; } echo "</TABLE>"; ?> <input name="submit" type="submit" value="Submit" /> <input type="reset" name="Reset" value="Reset" /> </form>[/code]
-
phpMyAdmin has an export tab that you can access from the database's main structure page. If the target server has phpMyAdmin installed, you can use its import tab to load the data. If that is not a possibility, you can load the data through the command line, reply here and I'll try to instruct you.