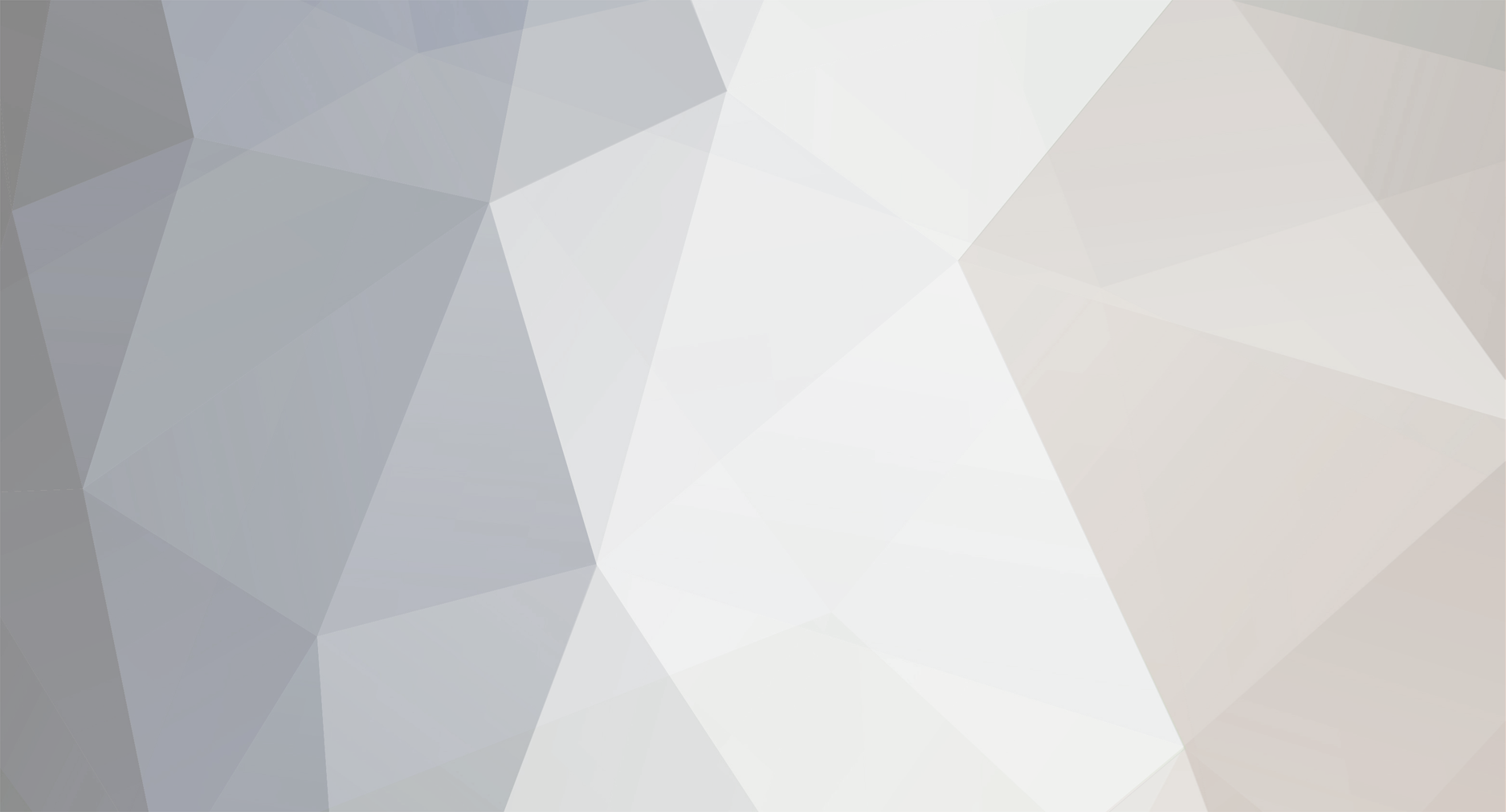
wickning1
Members-
Posts
405 -
Joined
-
Last visited
Never
Everything posted by wickning1
-
[code]<?php $db = mysql_connect('yourserver', 'yourusername', 'yourpassword'); mysql_select_db('championships', $db); $result = mysql_query("INSERT INTO signups (id, username, status) VALUES (3,'adrian','pending')"); if ($result) echo "woohoo it worked!"; else echo "sorry buster"; ?>[/code]
-
Show us an echo of the query after it's finished, just before you give it to mysql_query()
-
Bad approach, use "SELECT * FROM guestbook ORDER BY id DESC LIMIT $start, 15"
-
RegEx limiting result lines by 21 characters
wickning1 replied to MarkBad311's topic in PHP Coding Help
Talk about anti-climactic. I had my regex cookbook out and everything. -
You're performing 530 communications.. that's a lot of overhead. Try bundling it up into one query. You just need to do some string manipulation and use the "INSERT INTO table(col1, col2) VALUES ('data1','data2'),('data3','data4')" syntax.
-
I'm all for having guidelines, but I personally dislike Smarty quite a bit. I find it very confusing and cumbersome. Your use of sprintf() strikes me as distasteful too. I built myself an abstraction layer to do that, that looks much nicer. I think elegant and readable code is just a matter of experience. Little by little you learn the basic issues and deal with them. Eventually you're prepared to set up some personal guidelines, or build a basic framework to work in (that's what I recommend). Everybody will have different solutions, even teams who've worked together for a long time and developed a system that works wonderfully for them turn out code that is complete nonsense to another team. You do definitely need to have a way to set apart HTML, client code (javascript), and server code (php). You also need security standards for protecting against injections, session theft, and controlling user permissions. Beyond that, it's hard to say which way is the best. I have a lot of ideas, I'm working on releasing them as a package, but even then, the code is only as elegant as the programmer.
-
add items to a cart - POST, GET or GET + special code?
wickning1 replied to mmosel's topic in PHP Coding Help
Yeah, that's a pretty typical strategy for avoiding data reloads in general. Even if their browser pops up and warns them they're about to reload POST data, half your users will click OK anyway. Plus the message is annoying, best you keep them from getting it. -
I'm such a rebel. I store all my dates as BIGINT(14) - "20060306184623". You can still do date math on them in SQL because it's a recognized format, though sometimes you have to be smart about it or you lose your index. Then I have my own custom formatting function because I dislike PHP's syntax. As long as you're consistent throughout your application, whatever strategy you choose shouldn't be a problem, in terms of performance or anything else. Converting dates can be complex, but in terms of processing time it's pretty trivial.
-
Magic quotes are evil incarnate. I hate them with all my mind body and soul. They've confused so many young programmers. I'm lucky I started with Perl/DBI. That handles it the right way. Magic quotes are off by default in PHP5 and that's a very good thing.
-
Assuming you are running MySQL 4.1 or greater, here's a query to update everyone's avg_diff (untested): [code]UPDATE users u LEFT JOIN ( SELECT ID, AVG(diff) as avgdiff FROM scores GROUP BY ID ) s ON u.ID=s.ID SET u.avg_diff=s.avgdiff[/code]
-
[!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]Hmm.. I'm guesing that'd be a table with a access level, an id, and an foreign key back to the original table?[/quote] Correct. Another table is the way to go. Comma separated columns are bad. Bad!
-
Tell us more about your database. It sounds like your design may need work. How many tables do you have? What do they represent? How are you storing the score from a single round? How are you storing user information?
-
creating, connecting and intering data to the database
wickning1 replied to greeneel's topic in MySQL Help
Also if you are just starting out, you may want to try a precompiled package of binaries like XAMPP. -
There's nothing wrong from a MySQL standpoint, it has to be some kind of networking error. My best guess is that the server is not listening to the default port (3306 I believe), or there is a firewall somewhere in between.
-
Load-balanced servers will definitely make a difference. PHP sessions are stored on disk, so different web servers will not know about each other's sessions. I don't think there are significant performance differences either way, as long as your data is stored efficiently in both cases. You'll have to make more tcp/ip connections to get the data from mysql, but mysql has data access/caching optimizations that will help neutralize that cost.
-
Too many database queries load my page too slow
wickning1 replied to algarve4me's topic in PHP Coding Help
Yeah, my mistake. That's what I get for posting untested code :) mysql_free_result() isn't necessary, just good practice. It frees up memory so PHP can reuse it. -
Let mysql do that. SELECT * FROM eelist WHERE HmDept='001' ORDER BY LstName
-
PHP or MySQL stripping first record from each page
wickning1 replied to Prank's topic in PHP Coding Help
It's amazing how often people make this mistake. mysql_fetch_array() iterates through a resource, every time you call it, you advance one record, so you don't want to call it until you're ready for a row. -
creating, connecting and intering data to the database
wickning1 replied to greeneel's topic in MySQL Help
PHP uses an extension called "mysql" to communicate with MySQL. This extension must be specifically activated when you compile PHP. My guess is that you did not do this. Read the installation instructions on php.net carefully and try compiling again. -
Encrypting both ways without a library
wickning1 replied to Nolongerused3921's topic in PHP Coding Help
If you're using a MySQL database, you can use MySQL's encryption utilities, that's pretty well cross-platform and easy. Just SELECT AES_ENCRYPT('mystring', 'mykey'); If you do it in PHP, you need the mcrypt extension.. pretty tough to get around that, but you can abstract it in your application so that it's easier to port. -
Comma separated data is bad database design. Just so you know. :)
-
We aren't responding because none of us are sure what kind of help you need. What is the specific problem you are having? Why are you counting 3 different tables? What does each table represent? Why aren't you using SELECT COUNT(*)?
-
PHP arrays start at 0. 0 evaluates to FALSE. do this: [code]list($pos) = array_keys(array_keys($userPoints), $valid_user); if ($pos == "0" || $pos) $pos++;[/code]
-
You need to join on a subquery that uses MAX(date) and GROUP BY. I will attempt to take a closer look tomorrow.
-
I used Dreamweaver for a while for coding, but never did anything with the database through it. I'd stick with coding PHP in it and just use phpMyAdmin to manage your MySQL server.