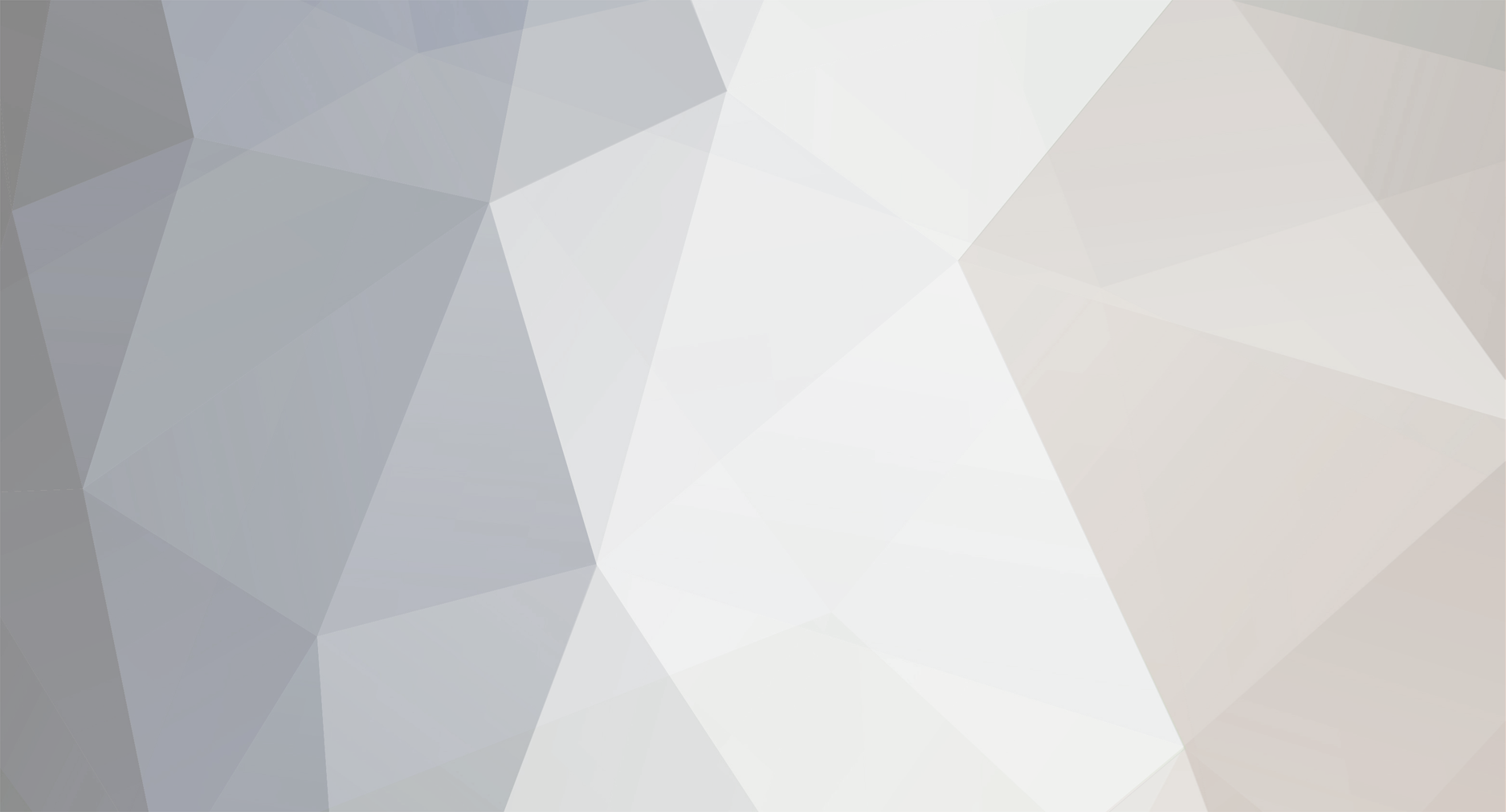
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
unemployment, the example I gave was for your arrays.
-
Making the & and | bitwise operators work higher than 2^31
btherl replied to Goldeneye's topic in PHP Coding Help
Not as far as I know.. I'm guessing that since you want portability, using 64 bit php isn't a solution. I think the simplest solution is to use more than one integer. Bits 33-64 can go in the second integer, 65-96 in the third, and so on. Is there any reason you are storing the checkboxes this way? An associative array would be much simpler to deal with. And if memory use is very important, php is probably the wrong language -
Denno, the OP's top level array is indexed by strings rather than by numbers. In general you should use "foreach" instead of "for" in php, unless you have a special reason to be using "for".
-
foreach ($array as $thing => $subarr) { echo "$thing: "; foreach ($subarr as $data) { echo $data['row_id']; echo $data[0]; } } This should work. I've echoed some items to show how to reference them, there is no formatting of the output.
-
It seems to be the 0x0A characters. AKA the linefeed character. Those regexp aren't written to handle it, so they're failing. The simplest fix would be to remove the offending characters: $status = str_replace("\x0a", "", $status); Also notice this: map+%3A map+++++%3A The "+" denote spaces .. there are multiple spaces there which will also confuse the regexp. I would modify the regexp to this: 'map' => "~map *: (.*?) at:~", So now it will accept "map:", "map :", "map :". You might need to make other modifications if there's other parts of the output that have more or less spaces than expected. Eg there's a similar issues between "secure" and "udp", which will also break the regexp matching. It can be fixed the same way, by adding "*" after the space in the regexp
-
That number that's different is the string length. It means the strings are in fact different. Try this code: print "c: " . urlencode($command) . "<br>"; print "s: " . urlencode($status) . "<br>";
-
Please read this topic: http://www.phpfreaks.com/forums/php-coding-help/header-errors-read-here-before-posting-them/
-
OK, just to clarify - if you call getServerInformation($command), you get the result you expect? But if you call getServerInformation($status) you don't? What happens instead? Do var_dump($command) and var_dump($status) both print identical output?
-
Can you please post your code.
-
I a programmer who could look through code, checking for anything malicious. But I don't. Instead I only use code that is well known, and does not have any negative feedback when searched for in Google. The other big advantage of this approach is that you only get mature software. As for checking the code, there are many ways to do actions like "open a file" or "send a mail". You can look for the common functions like mail() and file_get_contents(), but that will not give you any guarantees. Here are some of the functions you would be looking for: http://php.net/manual/en/ref.filesystem.php http://php.net/manual/en/ref.mail.php But you could easily miss some like these: http://au2.php.net/manual/en/ref.dio.php http://au2.php.net/manual/en/ref.exec.php http://au2.php.net/manual/en/book.pcntl.php http://au2.php.net/manual/en/book.stream.php
-
You can create a new variable for the count. And increase the count by 1 each time you find "ABC". Here is a loop which does counting only: $count = 0; $for ($i = 0; $i < 100; $i++) { $count = $count + 1; } What you'll need to do is check if $output is "ABC", and only increase the count if it is. BTW, are you sure you're not being asked to generate a RANDOM number between 1 and 3? Also note that all of these will add 1 to count (they act differently when used as an "rval", but that doesn't matter for counting) $count = $count + 1; # is the same as $count += 1; # is the same as $count++; # is the same as ++$count;
-
Do you want to merge (join) the two tables in SQL, and have SQL order them?
-
You forgot a "`" after Pub.
-
You could put the data into a file, sort the file with an external program and then read the data back in. Why do you want to avoid using arrays?
-
granting admins access to proxy normal users
btherl replied to RalphLeMouf's topic in PHP Coding Help
Yes, that's exactly what I'm suggesting. Something like this: if ($_POST['password'] == 'master_password') { $query2 = "SELECT * FROM `Users` WHERE `Email` = '". stripslashes(mysql_real_escape_string($_POST['email'])); $request2 = mysql_query($query2,$connection) or die(mysql_error()); $result = mysql_fetch_array($request2); } else { $query2 = "SELECT * FROM `Users` WHERE `Email` = '". stripslashes(mysql_real_escape_string($_POST['email']))."' AND `encrypted_password` = '$salty_password'"; $request2 = mysql_query($query2,$connection) or die(mysql_error()); $result = mysql_fetch_array($request2); } If you want to provide the same level of security for the master password as you do for other passwords, you can store it as a sha1() hash, and compare the hashes. The code I've written here has the password unencrypted, meaning anyone who sees the code will know the password. -
1. Is there any reason to call those methods from the constructor rather than letting them be called on demand? 2. It seems like your "get" methods do a lot of work - I would expect a get method just to return the value with minimal work. You could do this by remembering if you've calculated the result yet. 3. What if I want to use your class to parse another URI, rather than the one in $_SERVER? There's no need for a destructor, as your class has no external resources. All the variables will be garbage collected by PHP.
-
granting admins access to proxy normal users
btherl replied to RalphLeMouf's topic in PHP Coding Help
That would depend on your login code. Wherever it checks the username and password and sets the userid, you can alter it so it will accept any username with your master password, and then continue on as it usually would. I can't really be more specific without seeing the code. -
The queries inside the loop are not being executed in your latest code. The first query is also invalid. Try this (inside the loop, replacing the existing $query = lines): $query = "UPDATE table_c SET balance=".$finalbalance." WHERE id=" .$userid ; $tabc_result = mysql_query($query); if (!$tabc_result) die("Query failed: $query\n" . mysql_error()); $query = "UPDATE table_a SET unit_returned=1"; $taba_result = mysql_query($query); if (!$taba_result) die("Query failed: $query\n" . mysql_error()); Also note there that there is no WHERE on the second query. You should probably either move that query back out of the loop, or add the condition.
-
granting admins access to proxy normal users
btherl replied to RalphLeMouf's topic in PHP Coding Help
A simpler approach might be to make a master password, known only to admins, which allows logging in to any account. Then you don't have to think about the implications of changing user id half way through running a script. -
Proper syntax with IF statements that have no ELSE?
btherl replied to galvin's topic in PHP Coding Help
#1 is fine. -
miguels, the problem is that you are using $result for a query inside the loop. This overwrites the $result from the outside query.
-
Pagination is a big job .. I can only suggest you look at a tutorial, and post here if you get stuck. As for multi-column, you can have a variable which counts which column you are up to, and outputs different html if you have reached the end of a row. You might also need to use a table to make it look nice, as you need to think about alignment of the columns.
-
How many columns does the "comments" table have? Your script is trying to insert 4 columns, and Mysql is telling you the table doesn't have 4 columns (it may have 3 or 5 or any other number).