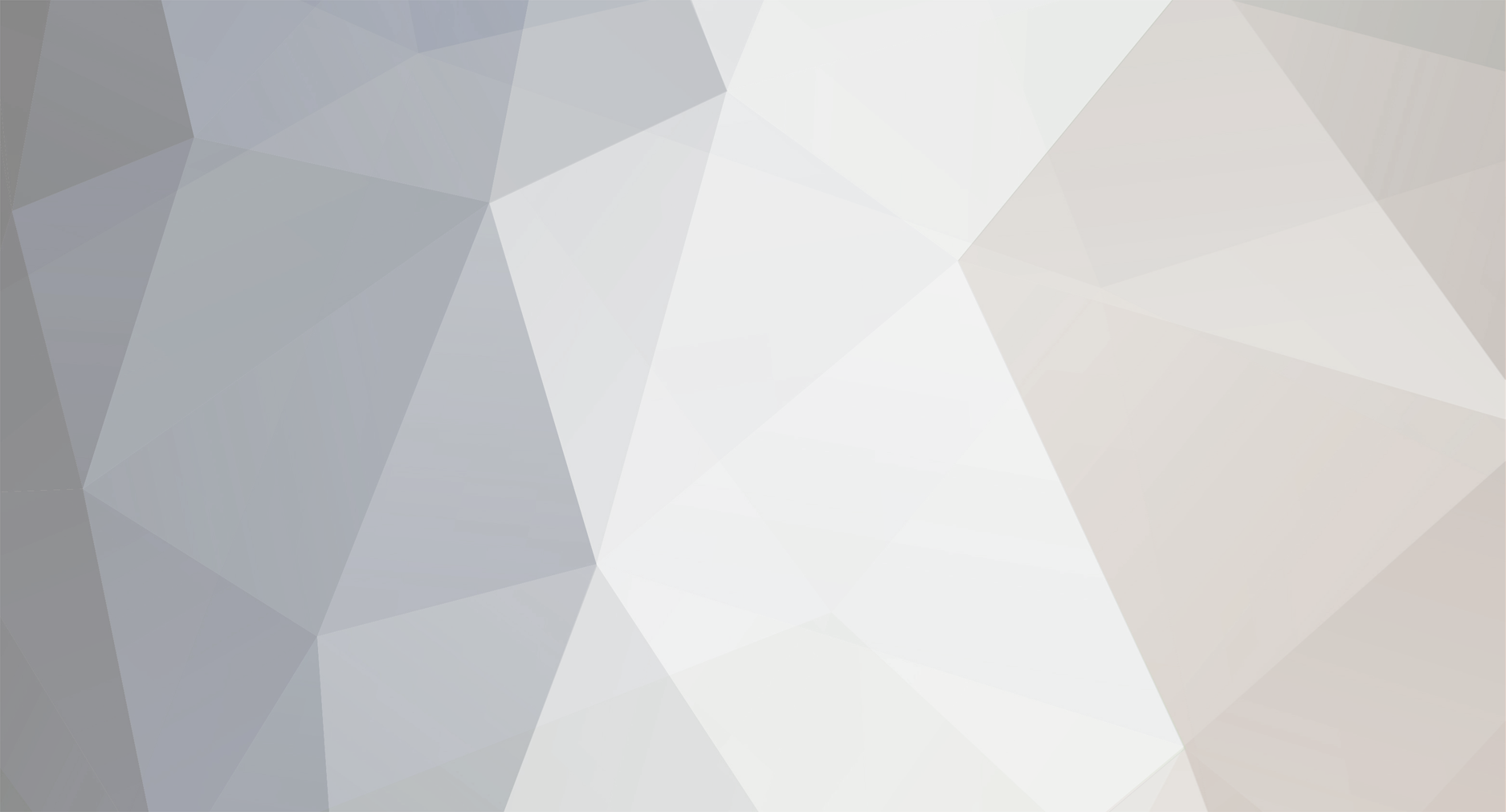
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
It really depends on where the data is going. If it's going to a database, follow that database's rules for sanitizing (eg mysql_real_escape_string() or prepared statements). If it's going to an HTML page, use html entities. If it has a restricted range or character set (such as an integer, or an email address) then regex is appropriate for checking and/or enforcing that range.
-
Practicing with IF statements to answer my own question - question
btherl replied to boblan66's topic in PHP Coding Help
You should be using name in the html, not id. id is a unique identifier for a single HTML tag. Name can be shared between multiple tags. Then you can address the value using the name in php. But there's another issue - once the radio button is selected, are you going to wait until the form is submitted before displaying the additional input tag? If you want it to appear right way you will need to use javascript. It can only be done in php if you don't mind requiring the form to be submitted first. -
The biggest improvement you could make to that code would be to document it I have no idea what those 1s and 3s and 4s are doing. A number that appears in code without any explanation is often called a "magic number", because it appears to work by magic, without helping the reader understand how it works. Full marks for indenting though - your code structure is very easy to read, and I can see the flow of control immediately.
-
How about this: if ($check2 != 0) { echo('Anti Spam has detected multiple comments posted.'); } else { // now we insert it into the database $insert = "INSERT INTO homecomments (username, comment) VALUES ('$username', '$comment')"; $add_member = mysql_query($insert); } I'm not sure if that's the exact code you want to skip, but the general idea should work.
-
Then I think your problem is with mod_rewrite, not with php. Can you show the code you are using with mod_rewrite?
-
Ok, add the following code after the query, but before the "if" conditions following the query: print "The query returned " . mysql_num_rows($list) . " rows<br>"; And show us what the script output is.
-
I think you have no alternative there except to modify your code so it will skip the remainder if there's an error. Eg you can create a variable $error, set it to true when you encounter an error, and have subsequent code only execute if $error is still false. Or you can use exceptions and a try/catch block to break out of the code.
-
Can you please modify your code to indicate how many rows are returned from the sql query.
-
Is the title you're looking for in the database, with the single quote included?
-
In novice code, lack of authentication is also an issue. Eg accepting an object identifier from the user where these object identifiers are shared between all users, and not validating the owner of the object before using it in the script. Extreme novice code may even allow users to log themselves in just by passing the username or user id in the url In intermediate code, not authenticating object identifiers is still a huge issue in my experience.
-
What is the "4/n" that you are initializing $count with? I think that is the problem. Try this script: $count = 4/n; var_dump($count); $count++; var_dump($count); Note that the value of $count hasn't changed.
-
Try adding calls to memory_get_usage() throughout the script, and calculate the difference in usage before and after anything you think might be using memory. That can help you find where it's being used.
-
An alternative for part 1 is: SELECT venue, count(*) FROM <table> GROUP BY venue That will give you counts for all venues. For part 2, I would do it in PHP. Any method I can think of to do it in SQL is ugly. Here's one approach that pops into my head: $max_consecutive_venues = array(); $last_venue = null; while ($row = mysql_fetch_row($result)) { $venue = $row['venue']; if ($venue != $last_venue) { if ($last_venue !== null) { $max_consecutive_venues[$venue] = max($max_consecutive_venues[$venue], $current_count); } $last_venue = $venue; # Start counting next venue $current_count = 1; # And reset count } else { # This is the same venue as the previous one. Increase the count. $current_count += 1; } } # And record the final venue in the list, which won't get recorded by the while loop. if ($last_venue !== null) { $max_consecutive_venues[$venue] = max($max_consecutive_venues[$venue], $current_count); } This will (assuming it works, it's untested) count all venues and how many times they appear consecutively. It's assuming the data it got from mysql is ordered by meetingid.
-
It's difficult to advise you with that much information. Is moving that one file and changing one require_once() line the ONLY things you did, after the last time you tested that that script was working? Otherwise, if you give us the locations of each file involved and the lines being used to include each file then we might be able to see what's going wrong.
-
memory_get_usage() might help you narrow down where it's getting used.
-
Storing it in a text file may be faster than storing it in the db, depending on the data and how you query it. A more heavy duty solution is memcache, but that would be more suitable for higher levels of traffic. There's nothing wrong with the concept of storing the data in a text file.
-
Ok cool Congratulations on getting it working!
-
If you want to search post_id for the same string then you can do this: $query = "select * from phpbb_posts " . " where post_text like \"%$trimmed%\" " . " or post_id like \"%$trimmed%\" " . " order by post_text"; // EDIT HERE and specify your table and field names for the SQL query Or if post_id is a number and you want to search for the exact number only: $query = "select * from phpbb_posts " . " where post_text like \"%$trimmed%\" " . ((int)$trimmed > 0 ? (" or post_id = \"" . (int)$trimmed . "\" ") . "") . " order by post_text"; // EDIT HERE and specify your table and field names for the SQL query I've added something a bit complicated in the second version because if you are checking against an integer column, you need to make sure the value you're checking is also an integer. So that code only checks against post_id if $trimmed is an integer.
-
Well there's a number of things that need changing. The basic problem is you have the same code repeated with the same names, and a web browser can't understand that, so it just uses the last one. So you also need to change the names. The structure would be something like this: <form name="myForm" id="form1" method="post"> <?php while ($something = $something) { ?> <input name="myText$something" type="text" id="myText$something"> <?php } ?> </form> <script type="text/javascript"> window.onload = hide_box(); function hide_box() { <?php while ($something = $something) { ?> document.getElementById('myText$something').style.visibility = 'hidden'; <?php } ?> } </script> The important things are: 1. The loops are only on the parts that need repeating. The parts outside the loop must not be repeated. 2. Each input element has a different name, so that each javscript instruction to hide the element can act just on that input element.
-
Oh, it's totally unrelated to integer division then Just a coincidence.
-
I think what you need there is to generate the content of the hide_box() function with the loop. The window.onload and function hide_box() lines will need to be outside the loop. The form also needs to be outside the loop, with only the input elements defined inside the loop. If you're not clear on what I'm going on about, feel free to ask for clarification