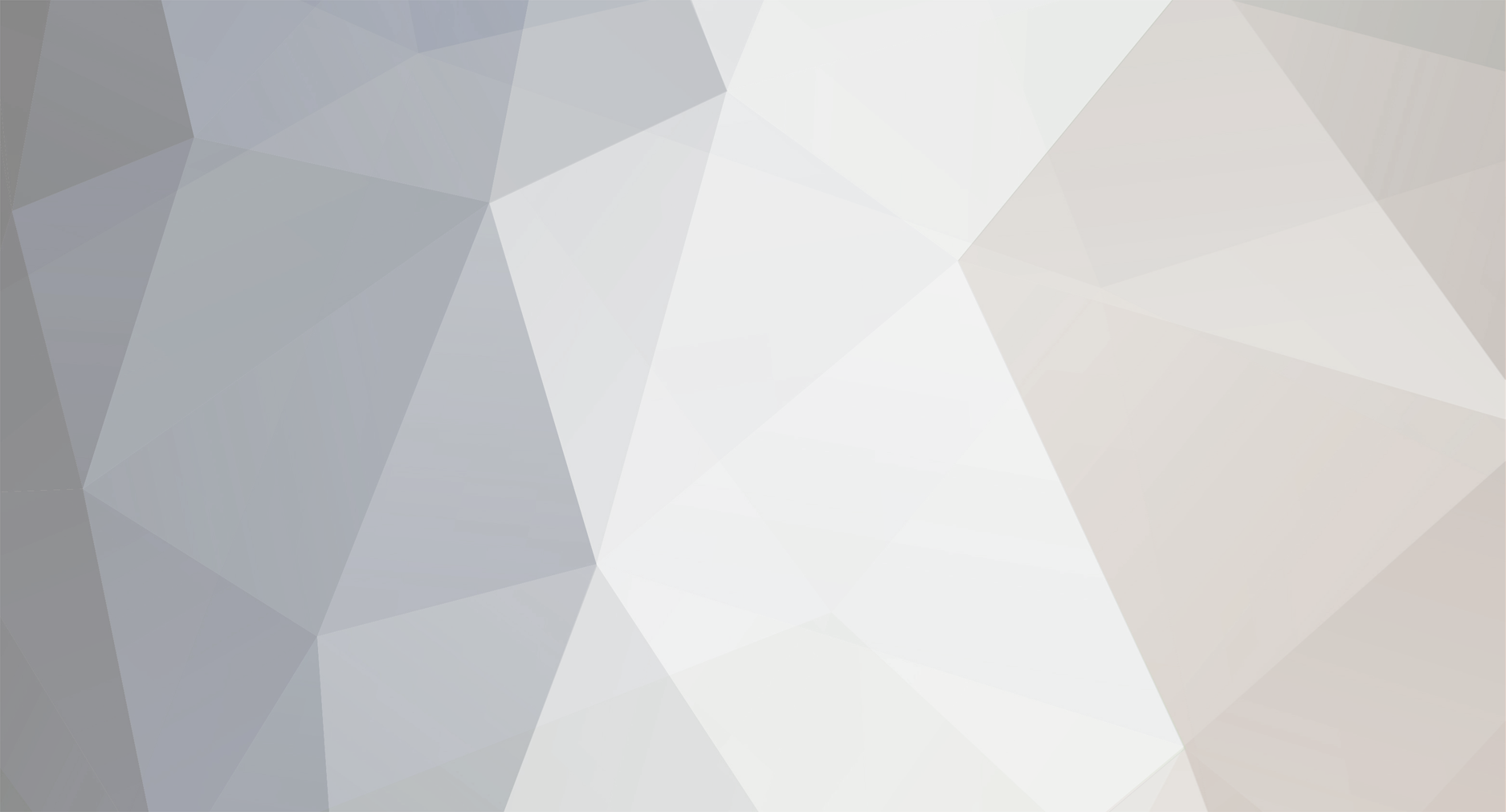
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
gizmola is right, there's too many changes to the original script. Traditional debugging techniques like what he is suggesting will work for you.
-
Can you post the original script too? If it stopped working after you modified it, then the fastest way to fix it is to find out what got modified.
-
What does your current system look like?
-
Try this: $result = mysql_query("SELECT city,abbr_state,zip FROM `zip_codes` WHERE `abbr_state` = '" . mysql_real_escape_string ( $_GET['abbr_state'] ) . "'"); I'm a postgres user, so no guarantees it'll work.. Edit: Fixed misplaced ")"
-
I was also a bit confused as to why this wasn't working so I looked into it further.. it turns out the problem is in this line: while ($k = 0); I'll leave it to you to work out what is wrong with it and why it makes the script act that way
-
I would add some debugging statements. Each time you add to a bin, print out "Adding block of size {$arr[$i]} to bin $j\n". And also print out each time you consider a bin and a block. Printing out the values of $i, $j and $k at each step woudl be good too.
-
Hmm.. well I don't see what could be going wrong. You can remove ob_flush(), that's not doing anything, unless something is secretly switching on output buffering before your script runs. Have you tried readfile() or fpassthru() instead?
-
Where do you reset $k back to 0? On a side note, you should remove the "&" in front of $arr and $max at the top of your code, unless you really do need to alter the value of those variables outside the function.
-
Ok.. so it is sending the correct length in the header, and it is sending the correct number of bytes.. Can you try adding this line, just before the exit: readfile_chunked($path); sleep(1); exit; I want to see if giving a bit of extra time before the script finishes will make a difference. It shouldn't, but it's worth a try. BTW have you posted the entire script or just part of it?
-
If your numbers always look like that, clustered around the lower numbers, you might try applying a function like log or sqrt before normalizing the range to 0-100%.
-
$bytes_sent = readfile_chunked($path); print "We send $bytes_sent bytes"; There may be an issue here with seeing that message, so you may have to disable the "echo $buffer;" line and all the header() calls temporarily. Another thing to check - is the content-length header being set correctly? Try printing out $headsize['Content-Length'], it should be the exact length of the file.
-
In some old versions of php, you could access session variables directly as $amano. This caused more problems than it solved, so the standard now is that you must use $_SESSION['amano'] instead.
-
What byte count does your function return?
-
Try this: $_SESSION['amano'] = $_POST['amano']; Make sure you only run that code if $_POST['amano'] is supposed to be stored into the session, otherwise it'll get overwritten. Then you can access $_SESSION['amano'] on subsequent calls to the script, the same way as you would have used $_POST['amano']
-
I doubt session_regenerate_id() is necessary. And if you do use it, you'll definitely need to understand why. I've been using php for 5 years now and even I don't know what it does, and that's because sessions work fine for me without it
-
Building Chat Database ouput but not parsing properly. Need help.
btherl replied to ScoreKeeper's topic in PHP Coding Help
I copied and pasted your Smiley() function from the second section of code in your first post. That version works. Make sure you are using this version: function Smiley($texttoreplace) { $smilies=array( '' => "<img src='images/smile.gif'>", ':angry' =>"<img src='images/angry.gif'>", ''=> "<img src='images/shocked.gif'>", 'fuck'=>"$#$%", 'Fuck'=>"&$#@" ); $texttoreplace=str_replace(array_keys($smilies), array_values($smilies), $texttoreplace); return $texttoreplace; } -
If you want to check if a variable is really false, and not anything else, you should do this: if ($var === false) If you want to check if a variable is false or null or 0 or empty string, use this: if ($var == false) If you want to set a variable to false: $var = false; So to set and do a strict check: $var = false; if ($var === false) { print "False is true."; }
-
Does a straightforward test of sessions work? This script will let you do a basic test: <?php session_start(); print "Stored: " . $_SESSION['val'] . "<br>"; $_SESSION['val'] = mt_rand(); print "New value: " . $_SESSION['val'] . "<br>"; ?> I'm a bit suspicious of the tricky session stuff you are doing, such as calling session_regenerate_id(), and checking if $_SESSION is set before calling session_start() (this isn't necessary). You might want to start simple and then add those things step by step.
-
Building Chat Database ouput but not parsing properly. Need help.
btherl replied to ScoreKeeper's topic in PHP Coding Help
The problem is here: $message=Smiley($message);//should make smiley here but not working....hmmmm print "<font color='red'><b>$getmsg3[name]:</b></font> $getmsg3[message]<br>"; Your Smiley() function works, but you are applying it to $message, then you are displaying $getmsg3['message'] instead. Try: $message=Smiley($getmsg3['message']);//should make smiley here but not working....hmmmm print "<font color='red'><b>$getmsg3[name]:</b></font> $message<br>"; -
I can't make much sense of that query without knowing where each column is coming from. The best way to show it is to give each table an alias, and include the alias with each column. For example: SELECT * FROM sportsdb_wins sw WHERE st.winner = win.teamid AND ... FROM sportsdb_teams st This is especially important if you query the same table multiple times in one query (which I'm not sure if you're doing, maybe you should post the entire query as well as the section you're working on).
-
Indenting is just as important in SQL as it is in PHP: (SELECT COUNT(*) FROM sportsdb_wins WHERE winner = teamid AND (winortie = 1 or winortie = 2) AND (SELECT teamid FROM sportsdb_teams WHERE teamid = loser AND teamdiv = teamdiv)) AS divwins, (SELECT COUNT(*) FROM sportsdb_wins WHERE loser = teamid AND (winortie = 4 or winortie = 5) AND (SELECT teamid FROM sportsdb_teams WHERE teamid = winner AND teamdiv = teamdiv)) AS divlosses, "AND teamdiv = teamdiv". Which teamdiv? From the inner query or the outer query? If both are from the inner query then all this condition does is exclude null values from the result, which I'm guessing isn't what you intended. If one teamdiv is from the outer query, the table needs to be specified, preferable for both. You can alias the tables to make the names shorter. I don't see any other obvious problems, but maybe if you deal with this teamdiv condition it'll start giving you the right result.
-
Try using "\r\n" instead of "\n"
-
Have you read the manual for both? It appears that they act the same for printable characters, but that call to filter_var() will also do this: "and characters with ASCII value less than 32, optionally strip or encode other special characters." The "optionally" part is controlled by additional flags.
-
$currency is either an array or an object, most likely an object. The solution is not to add quotes around it, but to cast it to a string like this: $array[(string)$currency] = $value; That's assuming you get the correct value out when you cast it. The reason you didn't get this error before would be that adding quotes to $currency automatically converted it to a string. Then check that you put the right value into $array. You can use var_dump() or print_r() on it.
-
The problem is that $matches[0] is empty. Try printing out each $description value that you process, and see if they match the expression you are using in preg_match()