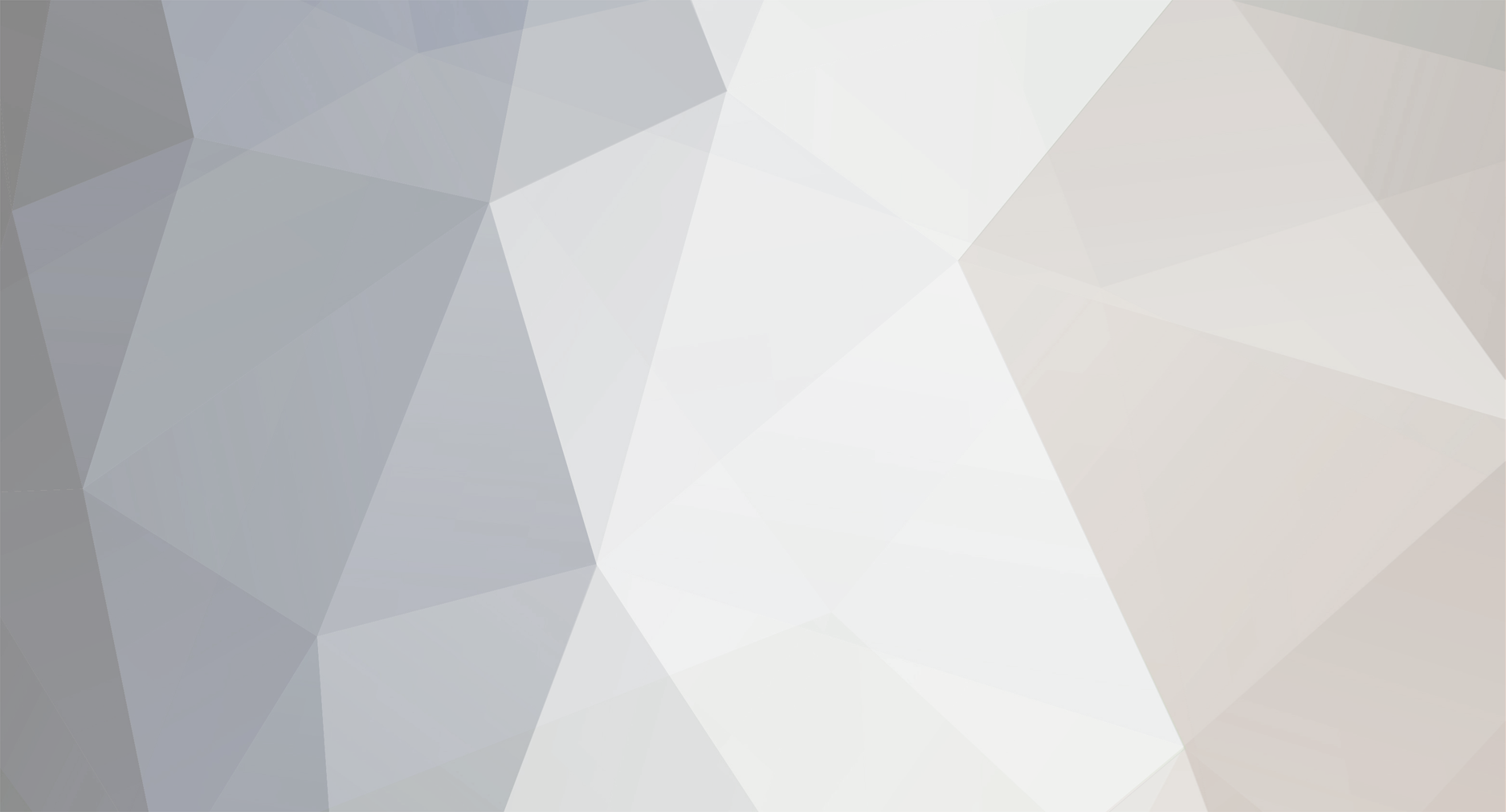
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
Testing for equality is this: if (this.value == "") Not this: if (this.value = "") Likewise, this: $_POST['your_telephone'] == 'Telephone' should replace this: $_POST['your_telephone'] = 'Telephone'
-
There's a few ways to do that. ORDER by field = 3 DESC, field That should do it. Or ORDER BY CASE WHEN field = 3 THEN 0 ELSE field END Or you could order by "field mod 3", which will map 3 to 0 and leave 1 and 2 unchanged.
-
Does the solution have to be better than an exhaustive search? With so few variables an exhaustive search is practical and the simplest way.
-
You can calculate 5% of something in a number of ways: $five_percent = $original * 0.05; $five_percent = $original * 5 / 100; # 5% is 5 out of 100 $five_percent = $original / 20; # 5/100 = 1/20, removing the common factor of 5 The second equation is probably the best one for you as you can just plug in 10, 15, 20, etc in place of 5.
-
There's a few approaches to deal with performance issues once you have a lot of data. You can partition the table, which means storing the data in several different tables according to a rule, such as "If (post id mod 4) == 0, store in table 1. If 1, store in table 2. If 2, store in table 3. If 3, store in table 4". Then you can look up any post in a table that's 1/4 the size of the original table. You can also cache data in memory, eg using memcache. Memcache has the advantage that you can have any number of servers load balancing and all accessing the same cached data. Some combination of caching and partitioning should be enough. The trick is caching the right things and partitioning on the right values.
-
A better setup would be a table with username (or userid) as one column, and post id as the other column. When a user likes a post, you add a row to that table. If they unlike it, you delete the row. Then to find who likes a post, you join the post table with the post_like table.
-
I definitely wouldn't recommend what's in thorpe's last post there That's not how switch was intended to be used. But apparently it does work. If you do use it, please document it to save the sanity of other people who see your code.
-
Switches are like a limited, inflexible version of if/then/else. They only work with one variable, and you have to list the exact values that variable can take. Let's say you use $_POST['mode'] to tell your script what mode to run in. Then you can do like this: switch($_POST['mode']) { case 'register': # Do register stuff break; case 'login': # Do login stuff break; default: # Handle unrecognized case } The advantage of a switch is that it enforces a good coding practice - it can reduce complexity of your code by forcing you to deal with a single variable with clearly defined values. You can't cheat with a switch because php won't allow it.
-
You could create a file reading class. The class remembers if it's reading a csv file or an excel file, and has a "next line" method. You keep calling the next line method until there's no more lines left. So instead of abstracting out the line processing magic, you've abstracted out the file reading magic. Edit: change "file processing" to "line processing"
-
You could write a function which takes an A value and the "4 different choice" as input and returns a B value. That function can have a series of if/then/else to decide what to return. As for how to implement the calculation of B from A, the most appropriate implementation depends on the rules for calculating it. If B is the same for a range of A values, you should use if/then/else. If B changes continuously with a change in A (ie there's a different B for every A), then you should do it with a calculation instead.
-
I don't think it's a problem for it to be visible in the source, pikachu. kakes, don't you have course notes saying how to change font colour? It can be done with an HTML font tag, or with CSS.
-
I think what you need is a basic tutorial for php. The first person you should be asking is your teacher or tutor. If you can't ask them for some reason, you can look for "php tutorial" in google and you will find several tutorials. You might need to look at a few before you find one that matches your learning style. I can't really help you here until you've gotten the basics.
-
Newbie taken over site - registration php broken?
btherl replied to Bifford's topic in PHP Coding Help
Can you post confirm.php as well? That is the script that is displaying the error message. The important part is the condition it checks before it gives you that message. -
For both of those, you will need to renumber your array. To set the answerid as the index I would recommend you create a new array rather than renumbering, so you don't accidentally clobber something if you have low answerids that match the old array indexes.
-
Can you post your code so far?
-
Insert to database when sent to specific email address
btherl replied to Eiolon's topic in PHP Coding Help
Autoresponders don't just do responses (well, some do but not all). If you're using unix, procmail can send the email to an external program which can do whatever you want, including storing data into mysql. -
Insert to database when sent to specific email address
btherl replied to Eiolon's topic in PHP Coding Help
"Autoresponder" might be a good keyword to look for. -
Using a generated row number in another query
btherl replied to MargateSteve's topic in PHP Coding Help
That's a reasonable hypothesis.. If $current_team_rank = 0, then it would only display ranks 1 and 2. Have you tried displaying the value of $current_team_rank? If it does turn out to be 0, try displaying every value being compared like this: $current_team = 1; print "About to find current team...<br>"; foreach ($rankings_arr as $rank => $row_table) { print "Comparing {$row_table['Tid']} with $current_team<br>"; if ($row_table['Tid '] == $current_team) { $current_team_rank = $rank; print "Match!<br>"; break; } } print "Finished finding current team, got $current_team_rank<br>"; The output of that should be enough to tell you why it's getting a rank of 0. Ohh... I was looking at the post preview (of this post) and noticed you have a stray space: $row_table['Tid']; $row_table['Tid ']; See the difference there? It showed up when I copy and pasted your code and put it in the forum php tags. -
Using a generated row number in another query
btherl replied to MargateSteve's topic in PHP Coding Help
In your SQL you do this to get the value that can be fetched as $row_table['Tm']: t.team_name as Tm So if you want team_id to be available, you can add t.team_id as Tid to your query, and then you can use $row_table['Tid']. Anything that you haven't listed explicitly after the "SELECT" will not be available at all, as the database never sent it back to php. -
That's good enough. I always keep seperation between code and display, so I would store the errors in a variable and then display all of them at once, along with all the other output. But it's basically the same idea as what you are doing.
-
Using a generated row number in another query
btherl replied to MargateSteve's topic in PHP Coding Help
Nope that all makes sense. You can break it down into two actions, "Find the current team's rank" and "Find rankings near current team". $current_team = "Mernda Mice"; foreach ($rankings_arr as $rank => $row_table) { if ($row_table['Tm'] == $current_team) { $current_team_rank = $rank; break; } } When you use foreach like that, $rank is the index and $row_table is the value. Once you have the rank for the current team, you can do a loop something like this: $start_rank = max(1, $current_team_rank - 2); $end_rank = min(count($rankings_arr), $current_team_rank + 2); for ($i = $start_rank; $i <= $end_rank; $i++) { # In here use either $rankings_arr[$i] directly, or set $row_table = $rankings_arr[$i] and then use $row_table } -
mjdamato, why would validation fail for the redirect address? If email can be sent to me@mydomain.com, then the MX for mydomain.com must be accepting mail addressed to "me".
-
Yep, exactly. Then your main code looks a bit like this: if (! validate_username($username)) { # Validation error, do something here } if (! validate_email($email)) { # Validation error, do something here } which is much easier to read than if (empty($email) || !preg_match('|[a-zA-Z0-9]+unreadable*regexp^goes?here|', $email)) { # Validation error } Then down below you have function validate_email($email) { if (empty($email)) return false; if (!preg_match('|messy regexp for email validation, found from google|', $email)) return false; # Email looks valid return true; }
-
Using a generated row number in another query
btherl replied to MargateSteve's topic in PHP Coding Help
It's the second. You could do it like this (assuming your sql result is ordered by rank ascending, which I think it is): $rankings_arr = array(); $i = 1; while ($row_table = mysql_fetch_assoc($table)){ $rankings_arr[$i] = $row_table; $i++; } foreach ($rankings_arr as $row_table) { # $row_table['GP'], etc etc can be used in this loop. } for ($i = 2; $i <= 4; $i++) { $row_table = $rankings_arr[$i]; # $row_table['GP'] etc can be used here, but only rankings 2 to 4 will be processed. }