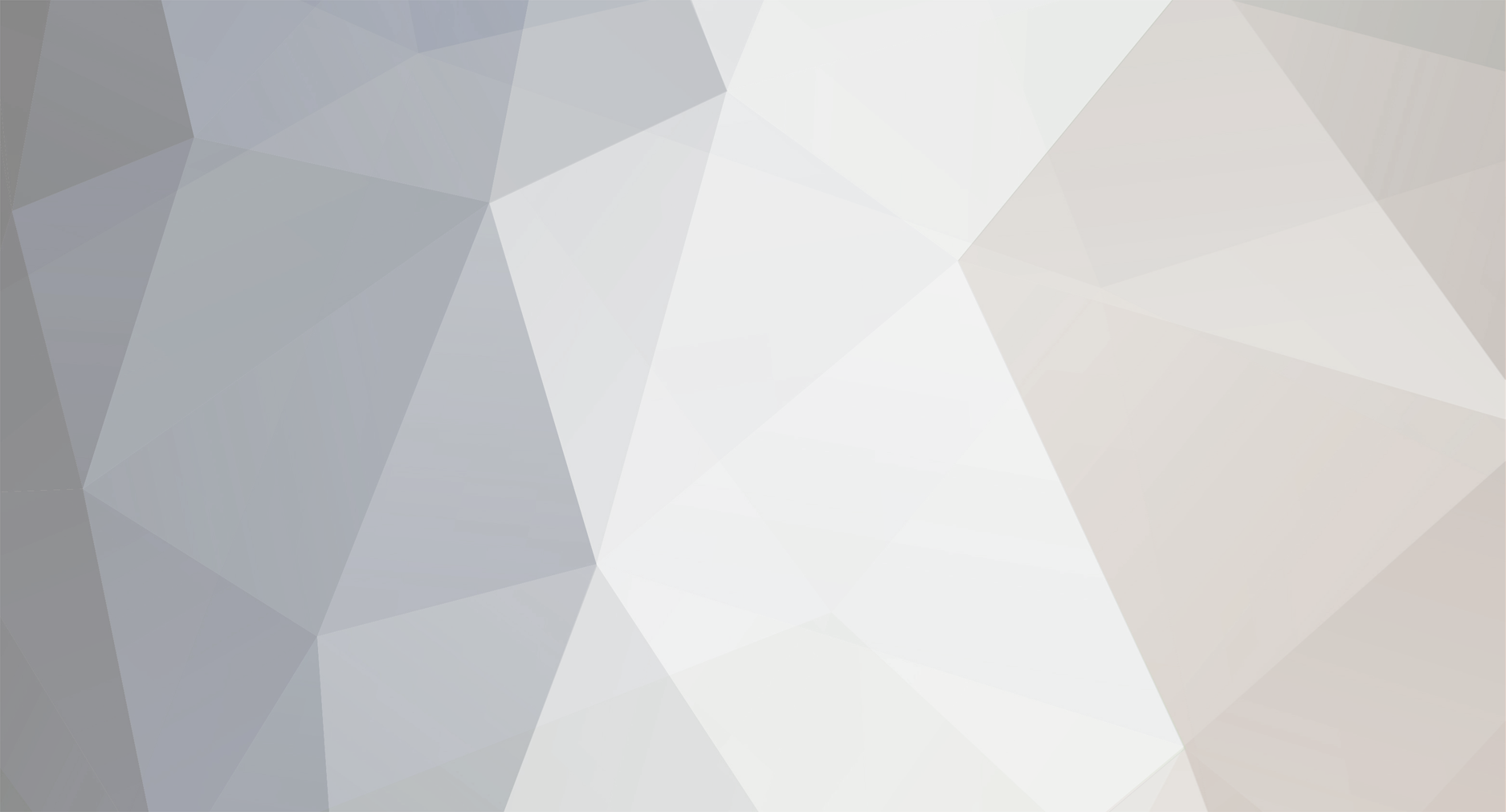
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
You need to change where you set the array as well. These lines are inconsistent: $array['EUR'] = 1.00; #<--- this one foreach ($xml->Cube->Cube->children() as $node) { $result = $node->attributes(); // returns an array $currency = $result['currency']; $value = $result['rate']; $array["'".$currency."'"] = $value; #<--- this one When you set the EUR value, you don't include quotes in the array index. When you set other currencies, you are including quotes. You should remove the quotes from all of them. So the code will be this: $array['EUR'] = 1.00; #<-- correct, quotes are needed to tell PHP that EUR is a string foreach ($xml->Cube->Cube->children() as $node) { $result = $node->attributes(); // returns an array $currency = $result['currency']; $value = $result['rate']; $array[$currency] = $value; # <-- correct, quotes are NOT needed here. PHP already knows $currency is a string.
-
This is the kind of indenting I mean: { print 'Could not open xml file' ; $url_contents = file_get_contents($url); if ($url_contents === false) { die("file_get_contents($url) failed"); } if ($url_contents === '') { die("file_get_contents($url) returned no data"); } $retval = file_put_contents($filename, $url_contents); if ($retval === false) { die("file_put_contents($filename) failed"); } $xmlReader->open($filename); while ($xmlReader->read()) { switch ($xmlReader->name) {
-
The solution is to indent your code consistently. Every time you use a "{", increase the indent. Every time you use a "}", decrease the indent. Then it will be obvious where one is missing.
-
Well that is odd indeed. Can you try printing the values like this: echo "Postcheck: [" . urlencode($postcheck) . "]<br>"; echo "Post: [" . urlencode($post) . "]<br>"; If that shows them to be identical, try this: echo "<pre>"; echo "Postcheck: "; var_dump($postcheck); echo "Post: "; var_dump($post); One of those should show up why they look the same but are unequal according to php.
-
How to match particular (switched) records in array
btherl replied to AndyPSV's topic in PHP Coding Help
So you want to match entries where c0 and c1 are switched? It can be done in SQL, but it's a bit tricky. It's a bit tricky in php as well. In SQL you could do something like this: SELECT (CASE WHEN c0 > c1 THEN c0 ELSE c1 END) AS c0 , (CASE WHEN c0 > c1 THEN c1 ELSE c0 END) AS c1 , COUNT(*) AS cnt FROM table GROUP BY c0, c1 What this does is it switches c0 and c1 if c0 > c1, but leaves them unswitched if c0 == c1 or c0 < c1. The end result will have all the c0 and c1 in the same order. Then "GROUP BY" can aggregate the results without worrying about switching. -
Try $array[$currency] instead. The following are equivalent: $var = 'EUR'; print $array[$var]; print $array['EUR']; In other words, you don't need to put the quotes in when using a variable to index an array.
-
All that script does is set headers. Where is the code to send the file? And what do you mean by "it's downloading the shell"?
-
I don't think that code example is what you want. This looks more like what is meant: http://en.wikipedia.org/wiki/Forward-confirmed_reverse_DNS It has to be done at the DNS configuration level, it's nothing to do with php (unfortunately). I'm not convinced it's related to the problem you're having with Google though. If I google "tickerfind.com" I find your site, not the duplicate.
-
When you submit that form you will get every hidden element submitted, and you won't be able to tell which was selected. I would do some basic form tutorials first. There's a number of ways you could do it - a drop-down list, a radio button, or submit buttons which identify which genre was selected.
-
The errors it gives you automatically often aren't enough. Change this code: file_put_contents($filename, file_get_contents($url)); to this: $url_contents = file_get_contents($url); if ($url_contents === false) { die("file_get_contents($url) failed"); } if ($url_contents === '') { die("file_get_contents($url) returned no data"); } $retval = file_put_contents($filename, $url_contents); if ($retval === false) { die("file_put_contents($filename) failed"); } That's a good start. That will catch a number of possible failures you could get while reading the data and writing it to the file.
-
Yes, you need to add something to your code to identify errors.
-
Every time you take an action which might fail, like these: file_put_contents($filename, file_get_contents($url)); $xmlReader->open($filename); you need to check if it failed. For example: $retval = file_put_contents($filename, file_get_contents($url)); if ($retval === false) { die("file_put_contents to $filename from $url failed"); } The manual explains what return values you should check for for each function, eg http://php.net/manual/en/function.file-put-contents.php
-
Looks like a trailing space will cause them to compare differently, but not a leading space: <? $a = '1.89'; $b = ' 1.89'; if ($a != $b) print "[$a] != [$b]\n"; $b = '1.89 '; if ($a != $b) print "[$a] != [$b]\n"; ?> Print out urlencode($post) is also a good way of finding any hidden characters.
-
Can you also tell us what you expected to see and what you actually saw. I'm not clear on whether the "if" succeeded when you thought it wouldn't, or it failed when you thought it would succeed.
-
Please add some code around this line: $videos[$i]['img'] = str_replace(">"," width=40% height=40% >", $matches[0]); So it looks like this: print "Original string: {$matches[0]}<br>"; $videos[$i]['img'] = str_replace(">"," width=40% height=40% >", $matches[0]); print "Replacement: {$videos[$i]['img']}<br>"; then run the script and copy and paste the output
-
What is the contents of $matches[0] ? And what is the contents of $videos[$i]['img'] after doing the substitution?
-
I would find your code easier to read if you indented like this: if (isset($_POST['Submit'])) { $month = $_POST['month']; $date = $_POST['date']; $message = $_POST['message']; $title = $_POST['title']; for($i=0;$i<$count;$i++){ $monthday = $month[$i]."<br>".$date[$i]; $sql1="UPDATE $tbl_name SET monthday='$monthday', month='$month[$i]', date='$date[$i]', message='" . mysql_real_escape_string($message[$i]) . "', title='" . mysql_real_escape_string($title[$i]) . "' WHERE id='$id[$i]'"; $result1 = mysql_query($sql1); } header("location:update2.php"); } I also moved the assignment from $_POST outside the loop. As for finding your bug, litebearer is giving you the right advice. The first step in diagnosing a "why doesn't it update the database?" is to display the query, and see if it looks right. The code he posted: echo $sql1; exit(); is equivalent to this, suggested by JD: die($sql1);
-
How about three tabs? How long does each request take? Eg if each request takes 0.5 seconds on average, you'll need three tabs to make 300 requests in a minute. You can do the requests in parallel in php but that's much trickier than just running multiple copies of the script.
-
Can you try this: $query_bf=mysql_query (" SELECT * FROM bf WHERE location IN ('England', 'Japan', 'Colombia', 'USA', 'Russia', 'Italy', 'Turkey') AND owner = '$username' ") or die("Query failure: " . mysql_error()); $num_rows = mysql_num_rows($query_bf); die("We got $num_rows results"); If it says "We got 0 results", then your query is not matching anything. If it says 1 or more, then your fetching code should work.
-
Are you able to look in the HTML source of a page that links to the at&t url and see what it is doing? You need to whatever it is doing. Usually people don't use an "&" in the path component of a url, but if you wanted to do it I think you would encode it using urlencode(). htmlentities() is for displaying things in HTML, not for urls.
-
You could use a foreach loop like this: foreach ($weight_category as $category_name => $category_weight) { if ($weight < $category_weight) { # Match } } I'm not sure if you want "<" or "<=" for the comparison. As long as you have listed the maximum weights, and you have all the weights in ascending order, then this algorithm will work.
-
Are you sure that is the code causing the warning? Yes, you should be using echo.
-
Yes you can do that. We have one app that does it that way, and another app that has the db connection in a global variable (not inside any class). Both of those approaches work.
-
In functions.php you have code which looks up the names of the products. You can use similar code in mail.php. Ideally you should have a function which takes as input the array of product ids, and returns as output an array containing product names, ids and quantities. Then you can use that same function in mail.php and also in showCart()
-
Also, the formatting of your code could use some improvement. Consistent indenting would make the logic clearer, and seperating out the validation for each form field onto a different line, or even a different if condition altogether, would make the code easier to read and make bugs easier to find.