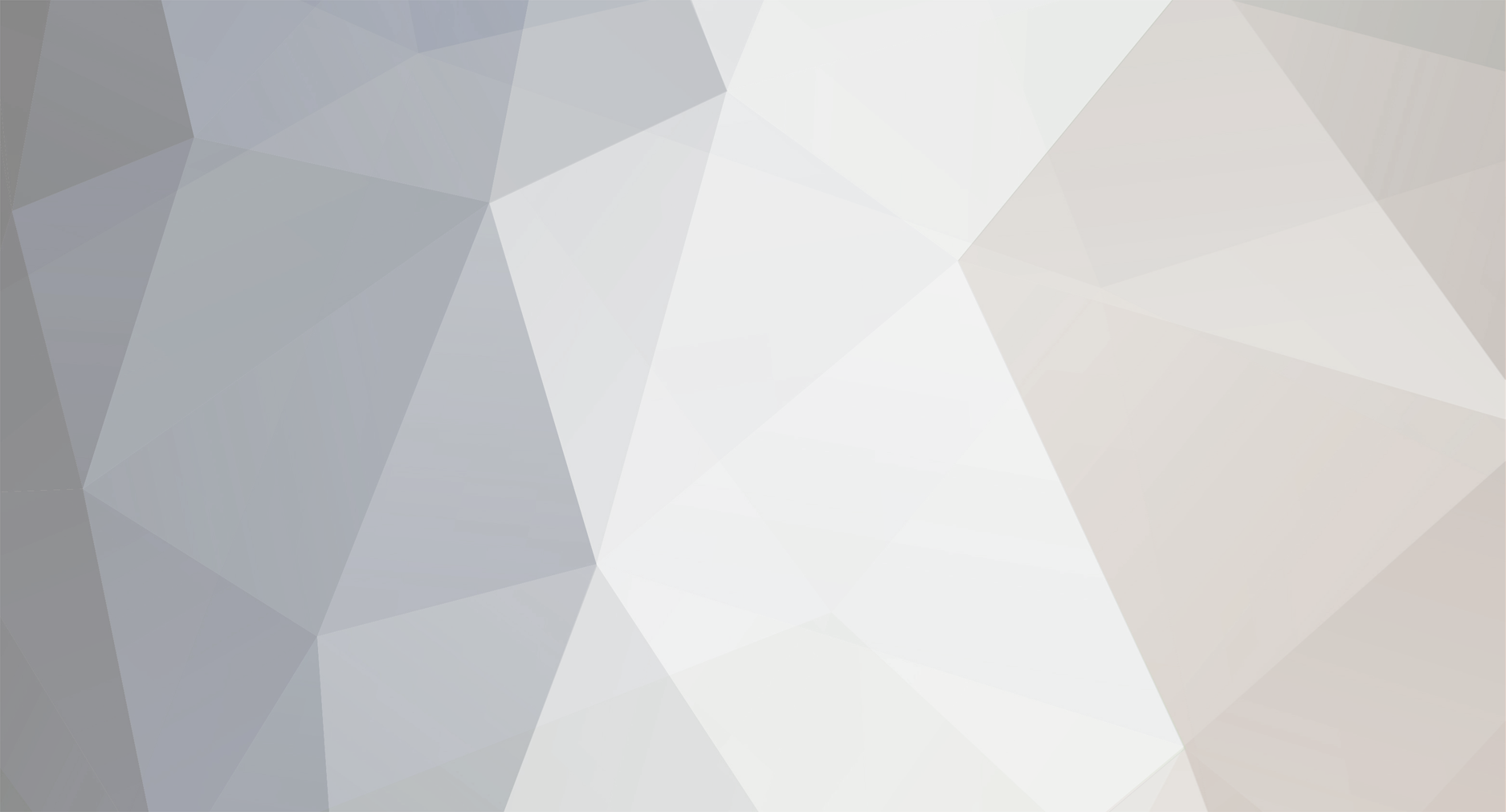
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Point taken! But honestly I just try to set good coding examples for the beginners perusing these forums. There's a lot of instances where data validation and testing will save a ton of headache. Also, you never know when you'll have to go through and fix someone else's horrible, broken code. As that's part of my current job, I'd like to save anyone else the trouble of having to do it if I can. Cheers!
-
Heh, logical errors happen. I still consider my coding more careful than Joe Schmoe's and overall more reliable. If I were actually using that function I would have found the bug in a couple moments and fixed it then. But careful eye you have, Sir!
-
Well, we were both wrong. I made a silly typo. In the case of the array of arrays, it did behave the same as array_values. With other inputs they behaved differently. [code] <?php define('NL', "\n"); $Out = ''; $A = array( "hello", "world", "how", "are", "you" ); $B = array( "key1" => "i", "key2" => "am", "key3" => "fine" ); $C = array( array( "key1" => "row1_val1", "key2" => "row1_val2" ), array( "key1" => "row2_val1", "key2" => "row2_val2" ), array( "key1" => "row3_val1", "key2" => "row3_val2" ), array( "key1" => "row4_val1", "key2" => "row4_val2" ), array( "key1" => "row5_val1", "key2" => "row5_val2" ) ); $Out .= 'ArrayFromArrayByKey($A, \'Key\'):' . NL . print_r(ArrayFromArrayByKey($A, 'Key'), true) . NL; $Out .= 'array_values($A):' . NL . print_r(array_values($A), true) . NL; $Out .= 'ArrayFromArrayByKey($B, \'key2\'):' . NL . print_r(ArrayFromArrayByKey($B, 'key2'), true) . NL; $Out .= 'array_values($B):' . NL . print_r(array_values($B), true) . NL; $Out .= 'ArrayFromArrayByKey($C, \'key2\'):' . NL . print_r(ArrayFromArrayByKey($C, 'key2'), true) . NL; $Out .= 'array_values($C):' . NL . print_r(array_values($C), true) . NL; echo '<pre style="text-align: left;">' . $Out . '</pre>'; // ArrayFromArrayByKey // $arr - The original array // $key - the key we are looking for // RETURN: An array of values // For each element in $arr, look for the key $key and add it's value // to our return array function ArrayFromArrayByKey($arr, $key){ $Ret = Array(); if(is_array($arr) && count($arr)){ foreach($arr as $val){ if(is_array($val) && isset($val[$key])){ $Ret[] = $val; } } } return $Ret; } ?> [/code] gave this output [code] ArrayFromArrayByKey($A, 'Key'): Array ( ) array_values($A): Array ( [0] => hello [1] => world [2] => how [3] => are [4] => you ) ArrayFromArrayByKey($B, 'key2'): Array ( ) array_values($B): Array ( [0] => i [1] => am [2] => fine ) ArrayFromArrayByKey($C, 'key2'): Array ( [0] => Array ( [key1] => row1_val1 [key2] => row1_val2 ) [1] => Array ( [key1] => row2_val1 [key2] => row2_val2 ) [2] => Array ( [key1] => row3_val1 [key2] => row3_val2 ) [3] => Array ( [key1] => row4_val1 [key2] => row4_val2 ) [4] => Array ( [key1] => row5_val1 [key2] => row5_val2 ) ) array_values($C): Array ( [0] => Array ( [key1] => row1_val1 [key2] => row1_val2 ) [1] => Array ( [key1] => row2_val1 [key2] => row2_val2 ) [2] => Array ( [key1] => row3_val1 [key2] => row3_val2 ) [3] => Array ( [key1] => row4_val1 [key2] => row4_val2 ) [4] => Array ( [key1] => row5_val1 [key2] => row5_val2 ) ) [/code] [b]The fixed code:[/b] [code] <?php define('NL', "\n"); $Out = ''; $A = array( "hello", "world", "how", "are", "you" ); $B = array( "key1" => "i", "key2" => "am", "key3" => "fine" ); $C = array( array( "key1" => "row1_val1", "key2" => "row1_val2" ), array( "key1" => "row2_val1", "key2" => "row2_val2" ), array( "key1" => "row3_val1", "key2" => "row3_val2" ), array( "key1" => "row4_val1", "key2" => "row4_val2" ), array( "key1" => "row5_val1", "key2" => "row5_val2" ) ); $Out .= 'ArrayFromArrayByKey($A, \'Key\'):' . NL . print_r(ArrayFromArrayByKey($A, 'Key'), true) . NL; $Out .= 'array_values($A):' . NL . print_r(array_values($A), true) . NL; $Out .= 'ArrayFromArrayByKey($B, \'key2\'):' . NL . print_r(ArrayFromArrayByKey($B, 'key2'), true) . NL; $Out .= 'array_values($B):' . NL . print_r(array_values($B), true) . NL; $Out .= 'ArrayFromArrayByKey($C, \'key2\'):' . NL . print_r(ArrayFromArrayByKey($C, 'key2'), true) . NL; $Out .= 'array_values($C):' . NL . print_r(array_values($C), true) . NL; echo '<pre style="text-align: left;">' . $Out . '</pre>'; // ArrayFromArrayByKey // $arr - The original array // $key - the key we are looking for // RETURN: An array of values // For each element in $arr, look for the key $key and add it's value // to our return array function ArrayFromArrayByKey($arr, $key){ $Ret = Array(); if(is_array($arr) && count($arr)){ foreach($arr as $val){ if(is_array($val) && isset($val[$key])){ $Ret[] = $val[$key]; } } } return $Ret; } ?> [/code] gives: [code] ArrayFromArrayByKey($A, 'Key'): Array ( ) array_values($A): Array ( [0] => hello [1] => world [2] => how [3] => are [4] => you ) ArrayFromArrayByKey($B, 'key2'): Array ( ) array_values($B): Array ( [0] => i [1] => am [2] => fine ) ArrayFromArrayByKey($C, 'key2'): Array ( [0] => row1_val2 [1] => row2_val2 [2] => row3_val2 [3] => row4_val2 [4] => row5_val2 ) array_values($C): Array ( [0] => Array ( [key1] => row1_val1 [key2] => row1_val2 ) [1] => Array ( [key1] => row2_val1 [key2] => row2_val2 ) [2] => Array ( [key1] => row3_val1 [key2] => row3_val2 ) [3] => Array ( [key1] => row4_val1 [key2] => row4_val2 ) [4] => Array ( [key1] => row5_val1 [key2] => row5_val2 ) ) [/code]
-
how do i update multiple tables at once....
roopurt18 replied to emehrkay's topic in PHP Coding Help
When you insert into a table, if there is an auto increment field you can call mysql_insert_id() to get the id of the last value inserted. You can then use that to insert into other tables and link data together. [code] $res = mysql_query("insert some sort of data"); $id = mysql_insert_id(); if($id){ mysql_query("insert more data using $id to link across tables"); } [/code] That's just a quick snippet written from memory. You'll want to check the PHP manual for the specific usages of any functions I've used, in case I misused them. (EDIT) Alternatively you can check the MySQL documentation and see if there is a variation of the INSERT or UPDATE statements that will do this. I don't think they exist though. -
[quote author=Barand link=topic=107669.msg432237#msg432237 date=1158003452] Roopurt, Thats a very long-winded way of writing $newarray = array_values ($arr); [/quote] I don't think they do the same thing. ^_^
-
Most of the time e-mail is almost instantaenous. Sometimes it gets delayed for a bit though. I wouldn't be surprised if you logged into AOL tomorrow and had several e-mails.
-
You wouldn't need to assume anything about the array if you program carefully. A safer function, IMO: [code] <?php // ArrayFromArrayByKey // $arr - The original array // $key - the key we are looking for // RETURN: An array of values // For each element in $arr, look for the key $key and add it's value // to our return array function ArrayFromArrayByKey($arr, $key){ $Ret = Array(); if(is_array($arr) && count($arr)){ foreach($arr as $val){ if(is_array($val) && isset($val[$key])){ $Ret[] = $val; } } } return $Ret; } ?> [/code]
-
I have an array: [code] Array ( [72] => Array ( [Trade] => CAB130 [tdesc] => CABINETRY ) [73] => Array ( [Trade] => CON160 [tdesc] => CONCRETE - SLAB 1ST DRAW ) [74] => Array ( [Trade] => CON162 [tdesc] => CONCRETE - SLAB 2ND DRAW ) [75] => Array ( [Trade] => CON168 [tdesc] => CONCRETE-POST TENSION INSPECTION ) ) [/code] Is there an existing php function that given that array will return an array from a specific index? [code] // Return an array of values from $OldArr using key 'Trade' $NewArr = Func($OldArr, 'Trade'); [/code] Writing such a function is trivial, but why re-invent the wheel?
-
extracting arrays from multidimensional arrays based on values
roopurt18 replied to silphium's topic in PHP Coding Help
Why not do something like: [code] $cols = Array('foo', 'bar', 'qwerty', 'wysiwyg'); foreach($cols as $col){ $tmp_col = addslashes($col); $sql = "SELECT * FROM table WHERE col='{$tmp_col}'"; $q = mysql_query($sql); while($record = mysql_fetch_assoc($q)){ $Results[$col] = ProcessRow($Results[$col], $record); } } [/code] From my experience, it's best to offload as much logic into SQL as possible. If it provides the functionality, why not take advantage of it? -
I'm using PHP and MySQL dynamically build pages of purchase orders. Each page contains a footer of two signature fields which must display at the bottom of each page. Each "page" of the PO is a [b]div[/b] with width 7.25in and height 9.25in. The footer has the following style set in the .css file: [code] .PurchaseOrder .POFooter{ width: 7.25in; position: absolute; left: center; } [/code] In addition, each footer is contained in a [b]div[/b] with the following: [code] <div class="POFooter" style="top: {FOOTER_TOP}in;"> [/code] where [B]{FOOTER_TOP}[/B] is replaced by: [code] <?php $footer_top = ($page - 1) * 9.25 + 9; if($sig){ // If a signature jpeg exists $footer_top -= .5; } $page = str_replace('{FOOTER_TOP}', $footer_top, $page); ?> [/code] Each page of the PO displays correctly in the browser, meaning the footer of every page sits directly on top of the header of the next page. However, when I print preview in IE (or print) the footer starts climbing higher and higher up each page. [img]http://www.ibswebview.com/test/po_imgs/footer_climb.JPG[/img]
-
By placing: $page = isset($_GET['page']) ? $_GET['page'] : '1'; at the top of the page you garauntee that $page is set, so you can remove the opening if statement. I.E., [b]if($page)[/b] is not necessary [b]unless[/b] you specifically want nothing to be shown when the url contains [b]page=0[/b].
-
Unless I totally misunderstood you, I'm not sure you even looked at what I wrote.
-
I fail to see why you have <? and ?> all over the place. [code] <?php // What happens if the url contains page=0? // 0 could be a valid page number, but won't pass the test (I think) // I recommend testing: if(isset($_GET['page'])){ if($_GET['page']){ // In your query you refer to $page, but nowhere did you give it a value // change $page in your query to $_GET['page'] $sql = "SELECT * "; $sql .= "FROM pages "; //$sql .= "WHERE page_id=".$page." "; $sql .= "WHERE page_id={$_GET['page']}"; // $page changed to use $_GET $result = mysql_query($sql) or die (mysql_error()); if(mysql_num_rows($result) == 1) { $row = mysql_fetch_array($result); $content = $row['content']; $content // This should probably read: echo $content; } else { $sql = "SELECT * "; $sql .= "FROM pages "; $sql .= "WHERE page_id=1"; $result = mysql_query($sql) or die (mysql_error()); if(mysql_num_rows($result) == 1) { $row = mysql_fetch_array($result); $content_empty = $row['content']; $content_empty // should probably say: echo $content_empty; } } } ?> [/code] If you have questions about the following line of code: $page = isset($_GET['page']) ? $_GET['page'] : 1; See [b]The Ternary Operator[/b] at [url=http://www.php.net/operators.comparison]http://www.php.net/operators.comparison[/url] [code] <?php // Here is a shorter block of code that should do what you want // Set the page, using a default if $_GET['page'] is not set $page = isset($_GET['page']) ? $_GET['page'] : 1; $sql = "SELECT * FROM pages WHERE page_id={$page} LIMIT 1"; $q = mysql_query($sql); if(mysql_num_rows($q) == 1){ while($row = mysql_fetch_array($result)){ $content = $row['content']; } }else{ $content = "You're trying to find random pages in my website!"; } echo $content; ?> [/code]
-
The OP appears to want a general function that loops but has a different body depending on from where it's called. I'm guessing their intended use is: [code] // get DB items $items = mysql_query($sql); // Loop over items and do something $results = Loop($items); // Loop over the results and do something else entirely $new_results = Loop($results); [/code] To the OP, if this is what you want, sorry you can't do that. You'll just have to write a new function for each type of looping functionality you want to provide.
-
The mysql_query documentation says the query should not end with a semicolon. I've not tried submitting multiple queries in that way, but would that return all of the results in a single result set?
-
Retrieving data from listbox and inserting into DB
roopurt18 replied to gopichand's topic in PHP Coding Help
Here you are specifying which columns you are inserting values for, 8 of them. INSERT INTO tutorials (uid, name, dname, cname, date, description, Keywords, SLO) Here is your first set of values, 8 of them ('22','akkf','kajfkafka','kasjfk','1157560060','kdfakf','fjakfaj','9'), Here is your second set of values, 1 value ('8'), If you tell MySQL you are inserting into 8 columns, you have to insert 8 values for every row. -
Store the results of each query in an array: $arr1, $arr2, $arr3, etc. Each array is basically a set, so we take the UNION of any two minus their INTERSECTION. $Result = array_merge( $arr1, array_diff($arr2, $arr1) ); $Result = array_merge( $Result, array_diff($arr3, $Result) ); Perhaps there is a better way, but that should do the trick.
-
I set my editor to replace tab keystrokes with two spaces. The simple rule I use is to press tab on a new line after any opening curly brace and to delete two spaces when I type the closing curly brace. Always use curly braces, even when they're optional. And never let a line go past 80 characters. Use lots of comments, avoiding the block comment (/* */) syntax so you can comment out blocks later if need be. [code] // Define consts define('CONST1', 'VAL01'); define('CONST2', 'VAL02'); // This class does blah blah yadda yadda // Use for asdf qwerty class MyClass extends MyBase{ function MyClass(){ // comment $var = $val; if(isset($something)){ // comment // comment $hello = $world; } $this->ReallyLongVarName = SomeOtherClass::ReallyLongFuncName( $val01, $val02, $val03 ); $this->arr = Array( $ReallyLongArrayValue01, AnotherBetterClass::WithAnotherLongFunc(), ); return; } } [/code] $val02 and $val03 in the function call within SomeOtherClass should be directly below $val01, my browser isn't placing it correctly when I view my post though. Last note, there is no right or wrong way. But there are best and worst ways. The best ways are so that you can come back to the same code written a few months ago, or not even your code, and still figure out what it does.
-
Are you saying that you want to display text, however once the text reaches a certain length to stop outputting that text and include elipses instead? For example, the text "Hello, World! Today I went shopping." would be displayed as "Hello, World! Today ..."
-
multiple condidtions in an if statement
roopurt18 replied to CircularStopSign's topic in PHP Coding Help
Most languages that share the c-style syntax use: || for OR && for AND ! for NOT -
multiple condidtions in an if statement
roopurt18 replied to CircularStopSign's topic in PHP Coding Help
if( $one || $two || $three ){ echo "hi2u"; } -
They don't show up until you refresh the page because the browser is displaying the old, cached version of the image. You can force refresh the image by adding a ?mktime() to the end of the image filename. echo '<img src="path/path2/file.ext?' . mktime() . '" alt=""/>'; It's not the most recommended method however, since it will always refresh the image and not just when it's new.
-
^ Agreed. What he's really trying to say is doing that with PHP will not turn out to be so simple.
-
Not sure but DELETE table or DROP Table how to use it O_O?
roopurt18 replied to Demonic's topic in PHP Coding Help
If you deleted your entire forum with PHPMyAdmin it's because you don't have a fundamental understanding of MySQL. Changing from the PHPMyAdmin interface to entering SQL queries directly isn't going to change anything as far as your understanding goes. My recommendation is to have a local development site on your desktop that mirrors your production site. This way you can break it all you want on your local machine without worrying about trashing your data on the web. XAMPP is a useful tool for setting up such a local site.