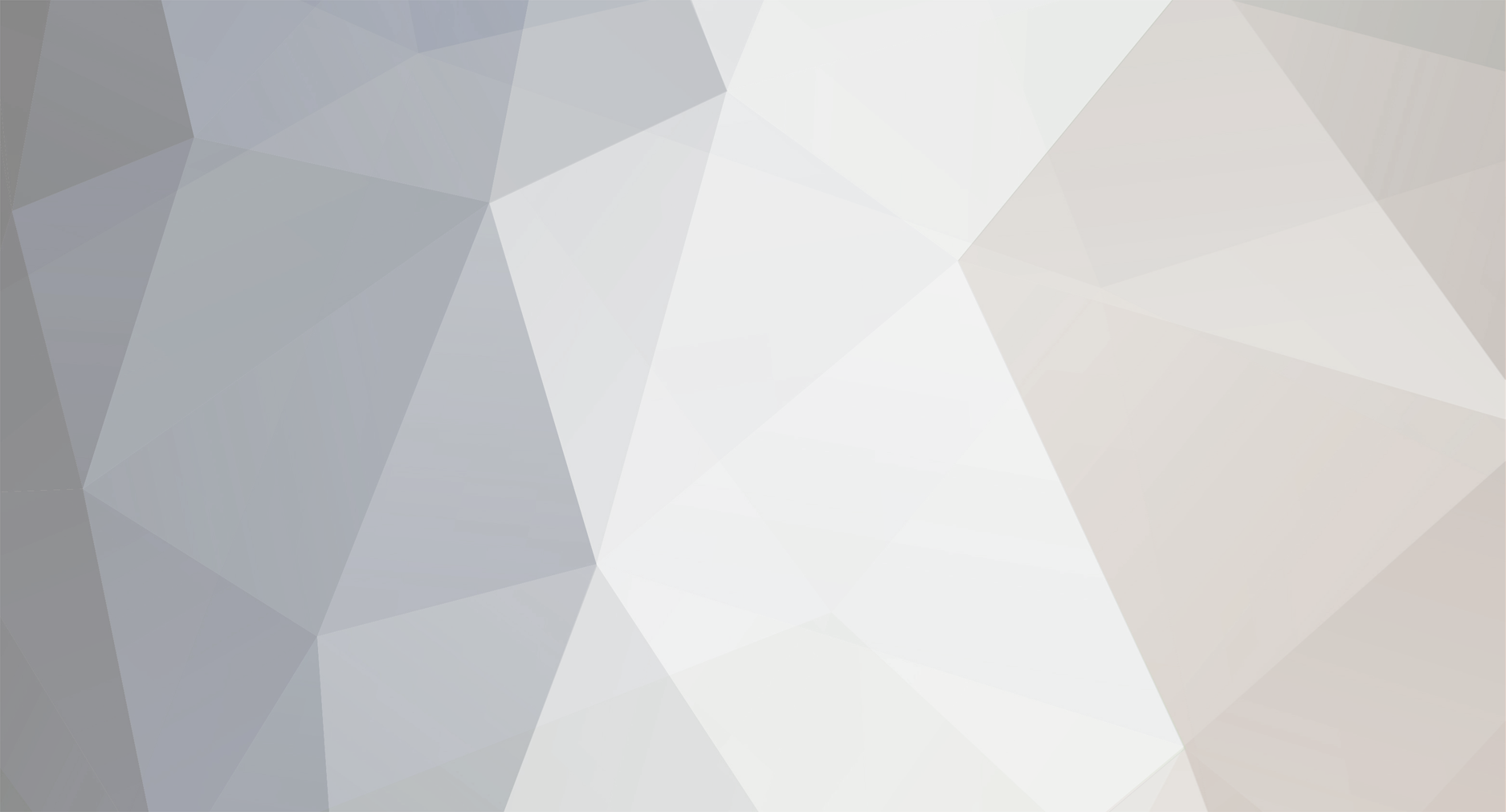
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
I think the performance impact between single and double quoted strings is probably minimal at best and probably not worth worrying about in most cases. I do agree that leaving variables outside the strings makes them easier to find though.
-
Like I said, the first 12 or so are not difficult to read. But what about when you get up to your 16th or 21st permission? Constants do not break encapsulation. It is much easier 6 months later to read: [code] if($perm & _MOD_FORUMS){ } [/code] than [code] if( $perm & 2097152 ){ } [/code] Not to mention repeatedly typing 2097152 through out your code, what if you fat finger an 8 instead of a 7? It will be very hard to catch. Use constants.
-
I recommend using named constants to represent the values of your permissions and not sticking $perm ^ 2 all over the place. Also, hex is more convenient as you add more permissions. You might know the value of 2^11 or 2^12 off the top of your head, but after that you're gonna need a calculator. Not to mention when you look at the code later you're going to go, "What the Hell does $perm ^ 2197987252197 do again?"
-
I used "we" as in us, the human species, not as in you and me. I could very well argue that by not doing anything to change the norm that we have also decided to do this as well though. And I did make a somewhat clouded reference to the size of a word being platform dependent. Binary and hex are so fundamental to computing and programming, shame to any programmer that doesn't understand them. And really, they're not all that difficult.
-
We'll start from the beginning. Computers are run by electrical current; the current is either there or it isn't. At the most fundamental levels, computers are only checking for the presence or lack of electrical current. This leads to the [i]b[/i]inary dig[i]it[/i], or bit. It is called a binary digit because it can only contain two possible values, 0 or 1. We call it a bit for short because we're lazy. Now, humans like to group things, it makes our lives easier. So we arbitrarily decided that 8 bits is called a byte, and 4 bytes is a word. (On some platforms a word could be more than 4 bytes, but that's irrelevant). PHP is different than most programming languages in that it isn't strongly typed, meaning that PHP converts relatively freely from an integer to a string to a floating point number. Other languages, like C, are very strongly typed. An integer can only interact with other data types that are a type of a number. Let's say that you're programming in C and that you happen to know that an integer in C is 1 word, which is the same as 4 bytes, which is the same as 32 bits. This means that you can think of any integer in C has being a string of length 32 where every place in the string is a 0 or a 1. For example: [code] 0000 0000 0000 0000 0000 0000 0000 0000 0110 0010 0101 1111 0101 1000 0000 0001 [/code] Let's throw a little bit of math in here for fun. Ignoring negative numbers, how many different numbers can we represent with such a string? Starting small... Bit string of length 1 [code] 0 1 [/code] We can store two values in a string of length 1, notice that this is the same as 2^1 Bit string of length 2 [code] 00 01 10 11 [/code] We can store four values in a string of length 2, notice that this is the same as 2^2 If we keep going, we find that we can store 2^n possible values in a bit string of length n. A bit string of length 32 can store 2^32 possible integers, which is why you can store some really large numbers in an integer variable! (Note to the more advanced reader: Yes, I'm ignoring sign bits. Don't get hung up on the details.) Explained in other words, every unique combination of a bit string is mapped to a unique integer. It's done through a mathematical formula which isn't really important for this discussion. But, rest assured that the following is true: 0000 equals 0 0001 equals 1 0010 equals 2 0011 equals 3 ... 1110 equals 14 1111 equals 15 Now, I ask you a question. You're designing a website that has 16 permissions that are on or off. The user has permission to do this or the user doesn't. Here is one approach. You can create 16 integer variables. Set a variable to 0 to indicate the user doesn't have permission. Set a variable to 1 to indicate that they do. But what have you done, you've created 16 integers, each requiring 4 bytes of memory space. That's 64 total bytes of storage. Wouldn't it be really cool if we could store all those settings in just 4 bytes? The answer is yes and here's how you do it. You let each [b]bit[/b] of a single integer variable represent a permission. We count bit positions starting from 0 and from the right of the bit string. 0101 - bit string 3210 - bit position In position 0 (bottom) we have a value of 1 (top). In position 1 we have a value of 0. In position 2 we have a value of 1. In position 3 we have a value 0. If each position (0, 1, 2, & 3) represent a different permission, we can store 4 permissions in just 4 bits! This means that a 32 bit integer can be used to store 32 permissions that have an on or off setting. So how do we get at the individual bits? We use a mask. This would be a good point to look up a tutorial on hexadecimal, but for reference: 0000 = 0 = 0 0001 = 1 = 1 0010 = 2 = 2 0011 = 3 = 3 0100 = 4 = 4 0101 = 5 = 5 0110 = 6 = 6 0111 = 7 = 7 1000 = 8 = 8 1001 = 9 = 9 We have now run out of 0-9 digits to represent values, so we use letters! 1010 = 10 = A 1011 = 11 = B 1100 = 12 = C 1101 = 13 = D 1110 = 14 = E 1111 = 15 = F What we have here is a mapping of 4 bits to a single value in the range of 0 through 9, A through F. You start a hexadecimal number with 0x and then end it with the digits (0-9, A-F). For example: The bit string: 0110 0010 0101 1111 0101 1000 0000 0001 is equivalent to the hex number: 0x625F5801 Look at that and absorb it until it makes sense; it's nothing more than a shorter way to write a bit string! You might be familiar with the [b]logical[/b] operators in PHP that compare values. These are && and ||. Well guess what, there are binary operators that do very similar tasks. & - From two values, make a bitwise comparison and return a composite result based on AND. For example: 0111 & 1010 = 0010 - Notice that the result only contains a 1 where [b]both[/b] bit strings also contained a 1. | - From two values, make a bitwise comparison and return a composite result based on OR. For example: 0101 | 1010 = 1111 - Notice that the result contains a 1 where [b]either[/b] bit string contained a 1. There is also a negation operator: ~ From a single value, flip all of the bits. For example: ~0101 = 1010 - Notice how all the bits changed to the opposite value of what they were. Now we can start packing multiple values into a single set of 32 bits (or an integer). [code]<?php define('MOD_FORUMS', 0x00000001); define('DEL_THREAD', 0x00000002); ... define('BOOT_USER', 0x80000000); var $perms = 0x00000000; // We init our current permissions to NONE // Let's turn on DEL_THREAD $perms = $perms | DEL_THREAD; // $perms now contains 0x00000002 // Let's turn on MOD_FORUMS $perms = $perms | MOD_FORUMS; // $perms now contains 0x00000003 (the right most set of four bits is 0011, or 3 in hex) // Let's turn on our last permission $perms = $perms | BOOT_USER; // perms now contains 0x80000003 // Now lets check for a permission if($perms & MOD_THREAD){ // Check if the MOD_THREAD bit is turned on! echo 'user can mod a thread'; } // Now let's turn a permission off // here we use the negation operator, we flip all of the bits in the permission constant and // AND it with the current set of permissions // Do it on paper and see why this turns off only one permission out of all of them :D $perms = $perms & ~MOD_THREAD; ?>[/code] Wow! That's great for on or off type permissions. But now you might be thinking what if a permission has more than one value? You can still pack those types of settings using bitwise operators. I'll leave that as an exercise for you to research or discover on your own though. (EDIT) Because you turn permissions on with |, silly me.
-
AFAIK you can't redirect with a delay in PHP; you'd need to use javascript or (not sure about this) a meta tag.
-
Try placing simple echo statements after each of your sql queries and then tell us when and where it blows up.
-
If that is the only thing in home.php, you need to enclose it between <?php and ?> Look at the top of index.php. With the URL you gave, there is no $_GET param, so it includes home.php. home.php is just opened and dumped in place. If you want to put php into an included file, you still need to start your php with <?php and end it with ?>
-
What is the name of the file you posted the code for? What is inside home.php?
-
The function is undefined because it doesn't exist. You could very well use this line:[code]$cat_id = $_GET['categoryid'];[/code] I included the function to emphasize that you are using a value that comes from your user [b]and you should always validate such values.[/b] You and only you, as the developer of that site, know what a valid category ID is. It could be as simple as:[code]$cat_id = is_numeric($_GET['categoryid']) ? $_GET['categoryid'] : 0;[/code] But you need to put something, because imagine if the user some how found out a little about your database, like the existence of your [b]plateau_pros[/b] table. What happens if they give you this URL: [code]http://www.nameofsite.com/category.php?categoryid=0; DROP TABLE IF EXISTS plateau_pros;[/code] Your sql statement will turn into: SELECT p.* FROM member_cat c, plateau_pros p WHERE c.categoryid=0; [b]DROP TABLE IF EXISTS plateau_pros;[/b]AND c.memberid=p.memberid Luckily, mysql_query only runs one query at a time, AFAIK, but why take chances? [b]Always[/b] validate your data!
-
Assuming: [code]http://www.nameofsite.com/category.php?categoryid=1[/code] category.php would need something like: [code] <?php $cat_id = ValidateCatID($_GET['categoryid']); // Double check for a valid value // Grab data from plateau_pros, where the member has the specified category id $sql = "SELECT p.* FROM member_cat c, plateau_pros p WHERE c.categoryid={$cat_id} " . "AND c.memberid=p.memberid"; $q = mysql_query($sql); if($q){ while($row = mysql_fetch_assoc($q)){ echo '<pre style="text-align: left;">' . print_r($row, true) . '</pre>'; } } ?> [/code] See what that gives you.
-
The functionality of creating folders on the client machine is usually available through the Save dialog box anyways. Creating a link to download a file is as simple as creating the [code]<a href></a>[/code] that I gave you above. If there's multiple items you want to offer for download, you have to create a bunch of those links, one for each item. The thing that differs is how you build the list. If you have 100 files you want to offer for download, you certainly don't want to type that out 100 times. And you certainly want it to stay up to date with which archives are actually valid and available for download. We can help you do that, but not without you being more specific about where these files are stored, how your website "knows" about them, etc.
-
If you're sure it's an error in your SQL statement, why not just post the statement?
-
Make a link to it in your html: [code] <a href="http://www.yoursite.com/file/path/filename.ext">Download this file</a> [/code] I know that's not the answer you're looking for, but your question was rather vague. Why don't you be a little more specific about how you're deciding what files to offer for download or some source code. It's like joining an automobile enthusiasts forum and saying, "How do I build a car?" Well, lots of ways.
-
Are you familiar with joins on MySQL tables?
-
So what you're saying is that by purchasing a product they gain access to a menu?
-
effigy, that will miss any embedded <? ... ?>
-
$regexp = '/^(^<\?(php)?(.*|\s*)/?>)$/'; I think that says, "Starting from the beginning, match what is not [b]<?[/b], followed by an optional [b]php[/b], followed by an character or whitespace, followed by [b]?>[/b], until the end of the string."
-
Operator -> on a function that returns an object
roopurt18 replied to CoolMints's topic in PHP Coding Help
I've not tried it, but would PHP allow: (FuncReturnsObj())->ObjFunc(); -
So why are you listing folder in every row for Steve if it's always the same? folder sounds like a column more suited for the users table IMO, otherwise you're just wasting space.
-
http://www.phpfreaks.com/forums/index.php/topic,96280.0.html
-
Can you post the current code and current set of errors?
-
That if statement is all kinds of wrong for what you want. Let's start with the syntax of if: if([b]expr[/b]){ [i]stmts[/i] } If [b]expr[/b] is true, then do [i]stmts[/i]. Now, what you want to do is check if $partno is equal to any of multiple possibilities. Let's say it in english, if $partno is equal to XXX OR $partno is equal to YYY OR $partno is equal to ZZZ, then do something. if($partno == 'XXX' || $partno == 'YYY' || $partno == 'ZZZ'){ [i]something[/i] } There are a couple difference between that and what you wrote. The first is the usage of [b]==[/b] instead of =. Also, you have to check $partno against each possible value separately. Here is what happens with the original [b]$partno = 'B-100' || 'B-200'[/b]. Remember, if is trying to evaluate an expression as true or false. We will also need to look at PHP's operator precedence, http://aspn.activestate.com/ASPN/docs/PHP/language.operators.precedence.html From the manual, we can see that [b]||[/b] has a higher precedence than [b]=[/b]. So here's what happens: [list] [*]'B-100' || 'B-200' evaluates first. A string is non-null so evalutes to true. true OR true gives true. [*]$partno = true evaluates next. $partno is [b]reassigned[/b] to the value true. [*]$partno is evaluated next. $partno now has the value true, and the expression passes the true / false test. [/list] I just wanted to clear up the way in which PHP evaluates expressions for you because without knowing that you'll run into a lot of trouble later. Let's think about the process of a shopping cart from the user's perspective: 1) User clicks a shopping link 2) User might filter their shopping categories, repeatable step 3) User adds items to their cart, repeatable step 4) User submits order 5) User prints or saves order confirmation Now let's think about the process from your side of things: 1) Each time the user filters the products or views a shopping cart page, you're going to have to run some sort of database query to get the proper products. 2) For each product, that query is going to return information such as if it's in stock, the unit price, the product name, the model number, the product description, etc. 3) You will then have to format all of these products into an HTML form so the user can actually shop. 4) When the user submits the order, all of their selections will be available in $_POST 5) You look at the $_POST data and create new orders in your site's database as necessary. Basically, what I'm trying to understand is why do you need to branch on the confirmation page based off of what was selected? Most, if not all, of the necessary information should already be contained within $_POST when the buyer submits the order.
-
Another error: [code] <?php $admin = mysql_num_rows($check_admin); if($admin['email'] == $email){ ?> [/code] mysql_num_rows returns an integer but you're using it as an array. Also, I'm not sure you why'd type: [code] if($admin['email'] == $email){ [/code] in the first place since the query is already checking if it exists. Basically what you've done is: 1) "Hey, MySQL, can you find all rows where email is equal to $email" 2) "Thanks MySQL, now can you give me the number of rows that were returned." 3) "Now I want to know if the row returned has the same email as what's in $email" - [b]but you already did that in step 1![/b] At the point of that if statement, $admin will contain the number of rows returned, either 0 or greater than 0. If you want to know if the user is an admin, just do [code] if($admin){ // greater than zero evaluates as true // do admin stuff } [/code]