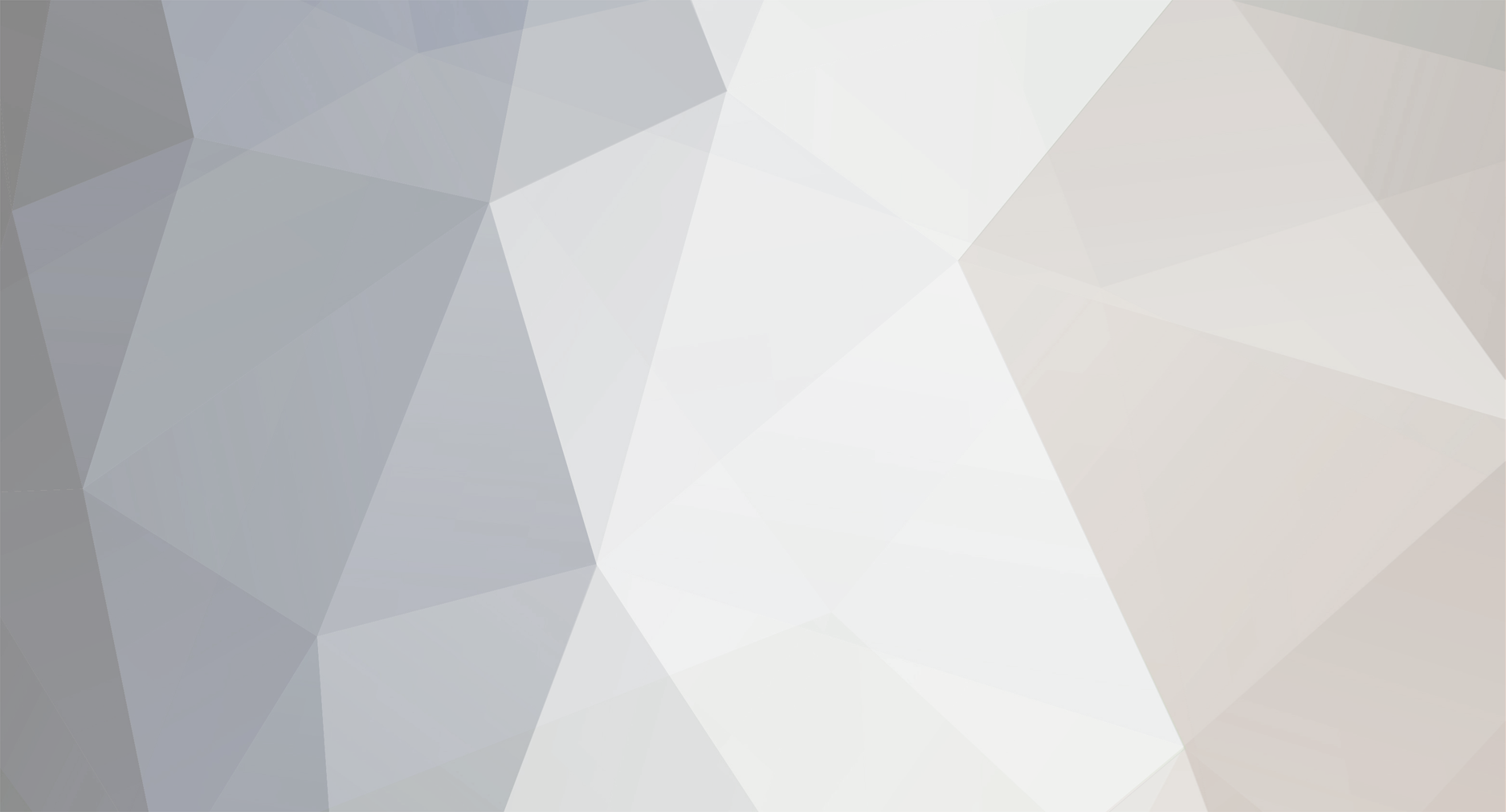
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Also, you need to check if the result of mysql_query is a valid resource or not before using it. http://www.php.net/mysql_query Look at the section [b]Return Values[/b] and not that FALSE indicates a bad query. You'll want to do something like: [code] <?php $check_admin = mysql_query("SELECT * FROM admins WHERE email = '$email'"); if($check_admin){ $admin = mysql_num_rows($check_admin); }else{ $admin = 0; } ?> [/code] Since all you're doing is a simple assignment, you can shorten it up a bit into: [code] <?php $check_admin = mysql_query("SELECT * FROM admins WHERE email = '$email'"); $admin = $check_admin ? mysql_num_rows($check_admin) : 0; ?> [/code]
-
I made a typo in my post, after calling header("Location: <some_url>"); you should make a call to exit(), otherwise your script will keep running. As I said in my original post, I'm not sure what's causing your errors specifically; it all looked fine to me as far as mysql is concerned.
-
I'm not sure what's causing your errors, but just a design suggestion. I notice you're returning your error string as part of the URL; this is going to make it difficult to provide information about multiple errors. Not to mention your URLs are going to get very long on pages that cause errors. You're using sessions, so why not have a $_SESSION['Errors'] array? Each time a page loads, it will check for error messages in that array, display them, and then clear the array. [code] <?php // Login.php session_start(); $HadErrors = false; if(!$email){ $_SESSION['Errors'][] = 'Missing email address.'; $HadErrors = true; } if(!$password){ $_SESSION['Errors'][] = 'Missing password.'; $HadErrors = true; } if($HadErrors){ header( 'Location: index.php' ); exit(); } // Rest of code below ?> [/code] [code] <?php // index.php session_start(); // First check for errors if(is_array($_SESSION['Errors']) && count($_SESSION['Errors'])){ $out = 'The following errors occured:<ul>'; foreach($_SESSION['Errors'] as $Error){ $out .= '<li>' . $Error . '</li>'; } $out .= '</ul>'; } $_SESSION['Errors'] = Array(); // Clear any previous errors // Rest of page follows ?> [/code]
-
1) "SELECT COUNT(*) AS Num FROM results WHERE user=$user_id GROUP BY user"; 2) In the results table, does the folder column have the same value for a user in all of that user's rows? I.E. if Steve's folder is "steve," does every row in the results table have the value "steve" where the user is also steve? Or is it possible that Steve's results are stored in multiple, different folders?
-
Two assumptions: $limit holds the number of chars to search before the space The form field with the search text is named 'search'. [code] <?php $Clean['search'] = ValidateSearch($_POST['search']); $Clean['search'] = substr($Clean['search'], 0, $limit) // Get first $limit chars $sql = "SELECT ... WHERE <field> LIKE '{$Clean['search']} %'"; ?> [/code]
-
How are you determining how many characters to search before the space?
-
This is under the assumption that your query is returning only valid market IDs and not just all of them. [code]<?php // method 1 $q = mssql_query($sql); if($q){ $Ret = Array() while($row = mssql_fetch_array($q)){ $Ret[] = $row['MarketID']; } } // BETTER yet method $q = mssql_query($sql); if($q){ $Ret = Array() while($row = mssql_fetch_array($q)){ $Ret[$row['MarketID']] = true; } } ?>[/code]
-
Are you saying you only want it to accept the first two characters of what the user entered and only return entries that have those two characters followed by a space and then followed by other text?
-
http://php.mirrors.ilisys.com.au/manual/en/function.mssql-fetch-array.php Returns: An array that corresponds to the fetched row, or FALSE if there are no more rows. Your SQL query looks something like "SELECT MarketID FROM ..."; When you run your query, $res3 holds the return set. The while loop in conjunction with mssql_fetch_array() returns [b]each individual record[/b] as an array. Each array returned in this fashion has numeric indexes that correlate to each column in the SQL query in addition to associative indexes named after the columns themselves. So... [code] <?php $q = mssql_query($sql); if($q){ while($row = mssql_fetch_array($q)){ echo $row[0] . "<br/>"; echo $row['MarketID'] . "<br/>"; // prints same as above } } ?>[/code]
-
Assume the field name in the form is [b]search[/b]. [code] <?php $Clean['search'] = ValidateSearch($_POST['search']); $sql = "SELECT * FROM <table> WHERE <field> LIKE '{$Clean['search']}%'"; ?> [/code]
-
CREATE TABLE IF NOT EXISTS Users( uname VARCHAR(16) NON NULL, ... UNIQUE(uname) ); http://dev.mysql.com/doc/refman/4.1/en/create-table.html
-
It means you need to access the data in the array with a different syntax than: echo Array[0]; echo Array[1]; etc.
-
It would also help to see the code the user is pasting into the form.
-
I would recommend against being so lazy as to want to write: echo $c[0] as opposed to echo $c['FName'] The second line of code is going to make a lot more sense 6 months from now.
-
Also, try entering the query through phpMyAdmin; you may have spelled one of your columns incorrectly and it'll catch it.
-
Instead of showing us the code that creates the query, try echoing the query itself. It's often easier to spot errors when looking at the finished query, without all the extra php syntax.
-
You still have to check the return value of mysql_query and handle errors. If you don't want to do it every time, write a function. You'll still have to check the return value of your own function though.
-
write to txt file - but not erase last entry
roopurt18 replied to GroundHogDay's topic in PHP Coding Help
Why not just open the file for append? -
TBH, I would start with the UMS (user management system) since no one will be able to do anything without logging in. The UMS will most likely be the most simple part of the website. Working on it now will allow you to continue thinking about the rest of the DB design and still produce some working code. I would also start gathering information from your vendors as to how they'd like to use the site. Do they want static reports that are built every day? Or do they want up-to-date reports generated on the fly? Some may want both. It doesn't matter how you program the site if it doesn't work for them. (EDIT) Forgot to mention something. It might be beneficial to allow super-users to tell the site to treat them as a particular user. For example, you're the admin, you're logged in, but you need to switch to one of your regular users to test something. Rather than logging out and back in, just add a feature for admins to switch to a specific user and then switch back when they're done. It's something I wish I had done.
-
One query at a time.
-
I wonder if the OP plans on returning to this thread...
-
From http://phplens.com/lens/php-book/optimizing-debugging-php.php [quote]From the above figures, you can see that biggest speed improvements are derived not from tweaking the code, but by simple global optimizations such as ob_start(), or using radically different algorithms such as HTML caching.[/quote] Also, no worries. It's a fairly common misconception that shorter implies faster. Sometimes it is true, sometimes it isn't. Also, a determining, often variable factor, in this entire thing is the size of the data set. An algorithm that runs at O(N * N) might actually outperform one that runs at O(log(N)) for small record sets. But as the record set approaches infinity, or scales upward, the log(N) algorithm will win every time. Garaunteed.
-
I would like to introduce you to my good friend Big O: http://en.wikipedia.org/wiki/Big_O_notation#Common_orders_of_functions particularly the section: http://en.wikipedia.org/wiki/Big_O_notation#Common_orders_of_functions Here is a little something I found through google: http://www.cs.wisc.edu/~hasti/cs367-common/notes/COMPLEXITY.html Your example of a 6 million line .php script is a little absurd to say the least. Further, I use the word algorithm because every script / program is the implementation of an algorithm. Here are two algorithms: Algorithm 1 [list][*]Get 1k records [*]Loop over records and do process 1 [*]Loop over records and do process 2 [*]Loop over records and do process 3[/list] Algorithm 2 [list][*]Get 1k records [*]Loop over records [list][*]For each record, do process 1, then do process 2, then do process 3[/list] [/list] Both algorithms work with 1k records. The first loops 3 thousand times, the second loops 1 thousand times. Which algorithm is faster? FYI, most institutions offer [b]Introduction to Algorithms[/b] or something similar as their first computer science course.
-
placing something in the middle of a file.
roopurt18 replied to Ninjakreborn's topic in PHP Coding Help
[code]<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Template 1</title> </head> <body> <div id="content"> <!-- dynamically place content here !--> {CONTENT} </div> </body> </html>[/code] Are you saying you want to replace dynamic content from the DB where I've placed the {CONTENT}? [code] <?php // Build the page content into a variable $content $html = file_get_contents($file); $html = str_replace("{CONTENT}", $content, $html); echo $html; ?> [/code] -
The "way" the code is written, or the algorithm used for processing the data, is the determining factor in how quickly your page will display, not the length of the .php file.