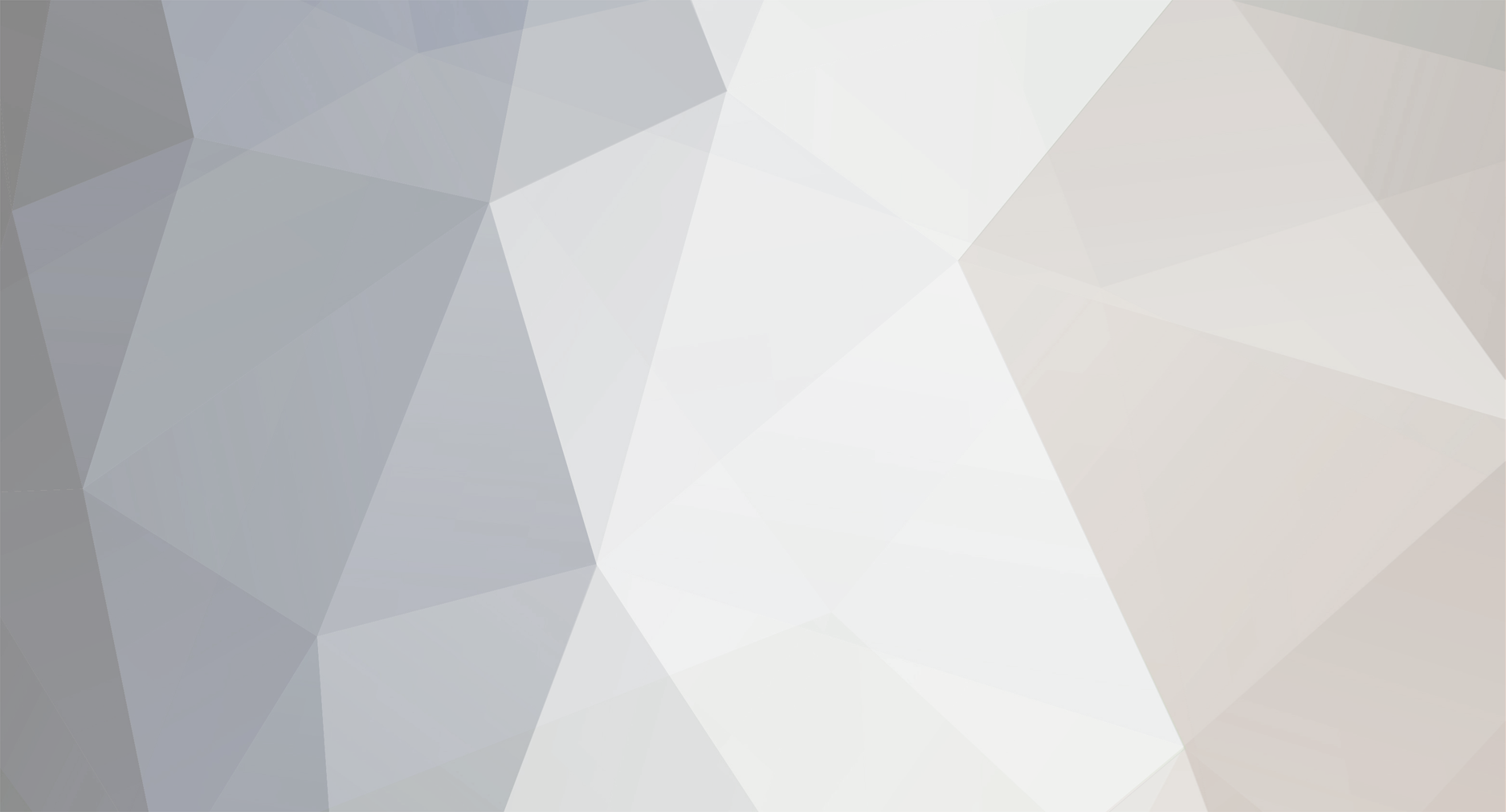
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Determine Last Access Date for All Tables in a DB
roopurt18 replied to roopurt18's topic in MySQL Help
Thanks! -
Is there a query that will return the last date all of the tables in a database were last accessed? By accessed I mean data inserted, retrieved, or other such operations. I ask because I've inherited a project that has a lot of tables that need to go. I've done what I can to eliminate some of them but I want to make sure there's no application code still running queries on them.
-
Caesar, redesigning the database isn't always an option as much as we'd sometimes like it to be. Also, if the OP was the original designer of the DB, then that's the best design they were able to come up with. Telling them to redesign it without giving any tips or pointers is pretty pointless IMO.
-
As for your question about forms, my recomendation is to design a form class that handles all basic handling required by every form. Build in the ability to add any type of fields to the form, some basic validation and a way of declaring which fields follow which validation rules, etc. After you create this class, let's call it CForm, you create another class for your current application. [code] class CMyAppForm extends CForm{ } [/code] Now in CMyAppForm, you include all of your application specific fields in here. For example, let's say you have a page for registration, another for editing information, maybe one for account transfering, etc. Each of these pages is likely to have a first name field. On every page where you use this field, do you want to have to re-type all of the code to lay that field out, validate it, etc.? Or do you just want to do this: [code] $form = &new CMyAppForm(); $html .= $form->GetField_FirstName(); [/code] and then when it needs to be validated: [code] $form = &new CMyAppForm(); $valid = $valid && $form->ValidateField_FirstName(); [/code] With this approach, every page in your application that has a first name field in a form will behave and validate the same. If you need to change its behavior, it's in one location. Also, it eliminates confusion from your users about how come that field behaves differently on 3 out of 5 different pages? Lastly, the reason you extend your CForm with CMyAppForm is later when you create a new application, you can create a new class CMyOtherAppForm that extends CForm. This way, you can have all of your application forms using the same logic, which makes it easy to fix if there's a glaring problem with security or something else. Also, your applications can still have independence from each other; who says a First Name field in application A behaves like the one in application B? Hope that helped.
-
I typically separate the process of retrieving data from a database and the process of formatting and displaying it. A simple example is if I'm writing a forums application. I might have forums.php which handles all of the forum logic such as how everything is displayed, who sees what, etc. But I do not put any SQL into that file. I separate it into another file, maybe forumsDAO.php, and this file contains functions to retrieve any necessary data from the database. It's one more file to maintain and keep track of, but let's say my site has been finished for 6 months and now I want to go back and redesign the database but only the database. Now the only file I have to go in and edit is forumsDAO.php and as long as it continues to provide the same functions (or interface) to the DB, forums.php will continue to work without any changes. This isn't exactly how I set things up, but it's a simple example to get the point across. Long story short, I don't like to have MySQL queries sitting in data objects (php classes) or in any code that strictly handles page layout.
-
For this project, are they looking at functionality or design? Building a simple shopping cart app probably is too easy. But building an entire site with adminstrators, users, proper security settings, feedback mechanisms, data import mechanisms, etc. is no easy task. As part of your design, you might want to allow the site to be easily customized in appearance and functionality. If your design supports this kind of functionality, you'll surely want to provide a couple examples of how you used it in developing the site.
-
It depends on how the system is intended to be used. In regards to the OP, I imagine that admins will want access to user data. Separating that data into another database only serves to make accessing that data slightly more difficult. Most of the time, a separate database will likely not help much. In the case of my current project at work, we have multiple clients using the same system. So we've assigned a separate database to each client.
-
Discussion - Do you separate your JS, HTML, and PHP?
roopurt18 replied to roopurt18's topic in Application Design
I don't see any reason why you'd want to include control structures within a template, so I agree with redbullmarky on that point. I think having to introduce extra syntax into HTML just so portions of it can be repeatable [b]is[/b] a bad idea. Inventing a new language is rarely a good idea. Separating most, if not all, of the major HTML from the data in a site makes it easier to design. You can easily see the page structure just from the HTML, even if you're not the author or it was written 6 months ago. I think it has the benefit of being more easily maintained. Additionally, if the site you are writing is a general purpose site for, let's say restaraunts, it will improve your marketability. It opens the door for multiple, completely different looking sites to run off the same set of source code. -
I do not recommend this game. I played it quite heavily and there are just too many timesinks and treadmills required to progress your character. On the bright side I'm gonna make a bundle on ebay.
-
Discussion - Do you separate your JS, HTML, and PHP?
roopurt18 replied to roopurt18's topic in Application Design
redbullmarky, what do you mean by accelerators? -
I personally do not like to use includes. I find that they make the code harder to read and follow, encourage the use of global variables, and in general are a PITA. For multipage forms, I prefer to use a hidden page parameter and use this value to choose which page to display.
-
I've been thinking about designing a modular site as well and here are my thoughts on permissions. I've not yet put it into practice and the coding would be tricky, but I think it would offer the most robust solution. Each module installed on the site should export privileges relating to that module. For instance, a forums module should provide privileges to edit, moderate, delete, move, etc. posts. A gallery module should allow for the deletion of images. Each module would be required to provide a privileges.php with a function export_privileges(). Every account to the site should have a user type associated with it and the user types [b]should not[/b] be hard coded (with the possible exception being the administrator)! They should exist in a table. The admin should be able to log in and dynamically create new user types. As each type is created, the site should get a list of the exported privileges from each module and allow the admin to configure those privileges for the new user type. With this method, when the site is accessing a module for a user, it can pass in the user's privileges for that module and the module can behave accordingly.
-
I'm not sure if this will have the desired effect or if SUM is a valid MySQL function, but: [code] $sql = "SELECT DISTINCT uname, SUM(points) AS total GROUP BY uname ORDER BY total DESC LIMIT 10" [/code]
-
There are several considerations when providing posting capabilities to a website. You want to give users the ability to format their posts using basic HTML, but you want to prevent them from embedding harmful scripts. The best solution in my opinion is to use a combination of htmlentities and regular expressions. You allow your users to enter special codes in square brackets in their posts which can be saved [b]as is[/b] into the database. Then when the data is displayed you use regexps to pull out the codes and replace them with HTML equivalents, such as exchanging [b][b][/b] for [b]<b>[/b]. This way your forums will only display user-entered "html" that you've allowed and coded for. If you want to display images in your forums, determining if the image is too large to display within your page width and needs to be resized is another issue entirely.
-
I don't see how regular expressions are going to help you solve that problem.
-
There are a few ways you could go about handling this situation depending on the nature of the form. My understanding is that there are limitations depending on the database fields and how they're defined, but usually requiring that some combination of data be unique within your table will solve this problem.
-
if (strpos($music, "2diealbum1")) { $song = "2diealbum/songs/2diealbum/2diealbum1.wma"; $songdisplay = "2die4 - Paranoid Entity"; } If it's garaunteed that the data you need is [b]always[/b] enclosed in double quotes and that it [b]always[/b] appears in that order, you can follow this algorithm: 1) Open file 2) Using a regular expression, fill an array with all data enclosed in double quotes only 3) With the array a) Starting at index 0, every third element comes from the line containing [b]strpos[/b] b) Starting at index 1, every third element comes from the line containing [b]$song =[/b] c) Starting at index 2, every third element comes from the line containing [b]$songdisplay =[/b] All it takes is for one body of the if / else if construct to assign variables out of order to ruin that approach though.
-
If you don't want to learn PHP yourself, then a freelancer might be your best bet. Using and tweaking scripts from the net that weren't meant to be used together can have unexpected consequences, such as your site not being quite secure.
-
You can use a combination of regular expressions or built in PHP functions to achieve this. htmlentities and strip_tags are the built in functions that could get you started. I find using htmlentities but allowing common bulletin board codes to be a simple way of giving formatting options and still preventing the insertion of unwanted scripts.
-
a mad quistion on stripslashes addslashes trim all together
roopurt18 replied to redarrow's topic in PHP Coding Help
I reread your last post, if you're asking is there a generic function that you can write that will validate any and all data, yes you can. It will be long, ugly, and messy. I'd recommend several smaller sections and possibly taking advantage of regexps for that. -
a mad quistion on stripslashes addslashes trim all together
roopurt18 replied to redarrow's topic in PHP Coding Help
[code] <?php // MakeDBSafe // $val - the value to make safe for insertion into the database // RETURN: $val modified to be safe for insertion into a database function MakeDBSafe($val){ if(!is_numeric($val)){ $val = "'" . trim(addslashes($val)) . "'"; } return $val; } ?> [/code] -
Is your PHP configured to display errors? If it's not and you have a script error somewhere chances are no output may even be making it to the browser. When you view the page in the browser, look at the HTML source the browser received and see where it ended. That may help.
-
Assuming that each row of your users table has an id field and that each row of your items table has an id field, I'd create a table: [b]ratings[/b] id int auto_increment non null primary key UserID int non null ItemID int non null Rating tinyint UNIQUE(UserID, ItemID) I'm not sure that tinyint would be appropriate, but if you're storing values 1 - 5 you certainly don't need a regular size int. The UNIQUE constraint is important IMO because it will prevent your application from inserting duplicate rows. Thus if you try to insert for a user and the query fails, you'll know to try and update instead, assuming you want people to edit their previous ratings.
-
Without clarification as to why it wasn't working, you may run into a similar problem down the road. So here it is: if([i]expr[/i]){ [i]stmts[/i] } [b]stmts[/b] will execute if [b]expr[/b] is true, easy enough. if([i]expr_1[/i] || [i]expr_2[/i]){ [i]stmts[/i] } [b]stmts[/b] will execute if [b]expr_1[/b] OR [b]expr_2[/b] is true. Still, easy enough. In your case above, [b]expr_1[/b] was: $aname == 'height' and [b]expr_2[/b] was: 'width' So [b]expr_1[/b] will only be true when $aname is the string 'height'. However, [b]expr_2[/b] will always be true because a string is a non-zero value. Hope that helps you avoid it again!
-
Discussion - Do you separate your JS, HTML, and PHP?
roopurt18 replied to roopurt18's topic in Application Design
I tend to use a single format.html file to control the layout of every page. As an example, if I had to go in and change the doctype, I'd only need to change a single file.