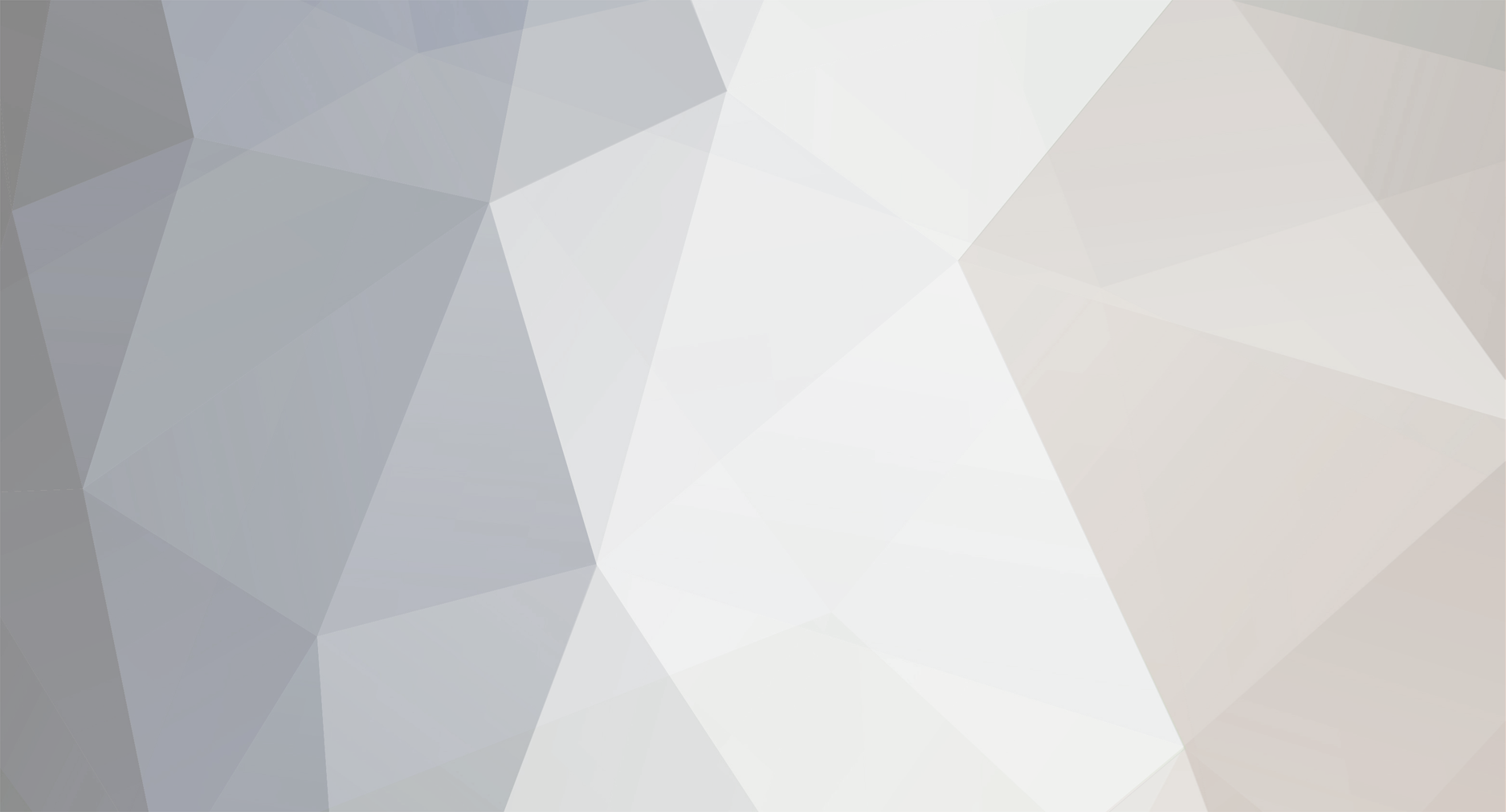
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
<?php function forumTopics() { // // When asking the database for data, it's more efficient to ask for // only the columns you plan to use. We are only interested in FORUM_TOPIC // and TID. The next line of code will build a string that looks like: // FORUM_TOPIC, TID // So that our select will be: SELECT FORUM_TOPIC, TID FROM ... // rather than: SELECT * FROM ... // // With the SELECT * lingo, consider what would happen if you added an extra // column to your table that had an average of 2MB of data in it. // Every time you called this function and ran your query, you'd be asking // the database for an *extra* 2MB of data *per* topic! If you had only // 10 topics, you'd be pushing an extra 20MB of data between your app and // the database engine when less than 200KB is probably needed! $cols = implode( ', ', array( FORUM_TOPIC, TID ) ); $sql = " SELECT {$cols} FROM forum_topic WHERE forum_topic.user_id='{$this->userId}' ORDER BY datetime DESC LIMIT 5 "; $result=$this->db->query($sql); $FTopics = array(); // Initialize your return value -- not necessary but good practice! if( $result ) { // Error checking! Only proceed if the $result is good while ($row = $result->fetch()){ // Your fetch here is calling mysql_fetch_assoc() under the hood, thus $row is already an array print_r( $row );// REMOVE THIS LINE LATER $FTopics[] = $row; } } return $FTopics; }?> I've added changes and comments. Let me know if anything is unclear. This is based off koob's code above.
-
cUrl alternative - so can use Google Maps Geocoding
roopurt18 replied to johnsmith153's topic in PHP Coding Help
I don't see what this has to do with cURL. Sounds to me like you either need a valid API key as suggested by premiso or set up a proxy of some sort. -
We use PhpED from NuSphere where I work. It's a lot faster than any of the other IDEs I've tried and it has the best support for debugging. It still has issues I don't like though. I wish Microsoft would make a PHP IDE; it'd be top-notch.
-
The API is the set of function you use to interact with your database. Are you using mysql_connect(), mysql_query(), etc? Are you using PDO? The API you're using determines the answer to your other question.
-
NetBeans and Eclipse are Ok. But they suffer from the same sluggishness as all Java apps.
-
It's Ok everyone. I solved it. <?php $x10="co\165\x6e\164"; $x11="\151\x73\137\x62\157o\x6c"; $x12="\x69\x73_\x6e\165\x6d\x65ri\143"; $x0b = array('Jan' => 288,'Feb' => 52,'Mar' => 94,'Apr' => 167,'May' => 98,'Jun' => 312,'Jul' => 42,'Aug' => 923,'Sep' => 222,'Oct' => 929,'Nov' => 99,'Dec' => 55);echo x0b( $x0b );function x0b( array $x0c ) { global $x10,$x11,$x12; $x0d = false;$x0e = false;if( $x10( $x0c ) === 0 ) return false;foreach( $x0c as $x0f ) {if( $x12( $x0f ) ) {if( $x0d === false || $x0f < $x0d ) {$x0d = $x0f;}if( $x0e === false || $x0f > $x0e ) {$x0e = $x0f;}}}if( $x11( $x0d ) || $x11( $x0e ) ) return false;return $x0e - $x0d;}?>
-
I'm not talking about the indexes being the same. I'm talking about the data in the array representing the same thing. Your array is: array( 0 => 'topic', 1 => 'id' ); When you reference it later in the calling code, you have to use the indexes 0 and 1. The identifier 0 doesn't tell me anything about the data being pointed at. Neither does the identifier 1. Your code will be more clear if your arrays were created as: array( 'topic' => 'My Pets', 'id' => 1 ); Then when you reference the elements of the array you have to use 'topic' and 'id' and the code becomes self-documenting. Numeric indexes are appropriate when all of the elements of the array are homogeneous. For example: $employees = array ( 0 => 'Betty', 1 => 'Fred', 2 => 'Suzy' ); Each element of the array is an employee so we don't need the indexes to tell us anything about the data they index to. Let me know if that's not clear. Also, out of curiosity, what underlying API are you using to interact with your database?
-
Which PHP Editor do you think is the best? [v2]
roopurt18 replied to Daniel0's topic in Miscellaneous
Can we add PhpED from NuSphere? It's a very nice IDE. http://www.nusphere.com/products/index.htm -
$FTopics[] = array($row[FORUM_TOPIC], $row[TID]); echo '<a href="../forum/view_topic.php?id='. $record[1] .'">' . $record[0] . '</a><br />'; I consider it best practice to reserve numeric indexes for identical items. In your case, $record[1] is a topic id and $record[0] is a topic title; these are not identical items. You code will become more clear now (and more importantly 6 months from now) if you use an associative array. In fact, you can let MySQL and PHP do a little of this work for you! <?php function forumTopics() { $cols = implode( ', ', array( FORUM_TOPIC, TID ) ); $sql = " SELECT {$cols} FROM forum_topic WHERE forum_topic.user_id='{$this->userId}' ORDER BY datetime DESC LIMIT 5 "; $result=$this->db->query($sql); $FTopics = array(); //initialize as an array while ($row = $result->fetch_assoc()){ // I'm assuming a fetch_assoc() method exists, and I'd even prefer to use fetch_object() $FTopics[] = $row; } return $FTopics; } ?>
-
I agree with this sentiment. If your function is called ForumTopics(), then it is only expected to return the forum topics. The function itself has no idea on how they will be used. Today they are used in an HTML page. Tomorrow they might be used in XML, Json, or a PDF report. Keep the data and the visual representation separate for as long as possible and then your code will be much more reusable.
-
I went to your link. I typed something in the box. Then I clicked on the 'view source' thing in IE. This is what I saw: Your name is: asdf <br/><a href=welcome.html>Home</a></iframe></noscript></object></layer></span></div></table></body></html><!-- adsok --> <script language='javascript' src='https://a12.alphagodaddy.com/hosting_ads/gd01.js'></script> That is not valid HTML code and likely the cause of your problems.
-
Which PHP Editor do you think is the best? [v2]
roopurt18 replied to Daniel0's topic in Miscellaneous
Not related to editors, but you folks shouldn't be using FTP to transfer your livelihood around. Use SSH. -
Well they need to provide you with a means of writing a file to their server. They could create a file upload script. They could use soap. They could allow you to use scp. They could allow you to use ftp. There's lots of choices.
-
Not without altering the current position of the array.
-
return causes a function to end, optionally returning one value to the calling code. That one value returned can be any PHP data type, including but not limited to: + ints + strings + floats + objects + arrays + arrays of objects + arrays of arrays of arrays of objects etc. Basically, if you want to return a list or collection of items, then you must build them up as an array inside the function and then return the entire array.
-
That isn't even valid PHP code.
-
Two things. 1) If you want most recent, you should be orderying by: datetime DESC 2) You're issuing a return statement in your while loop. Return causes the function to immediately return to the caller. Thus your function is issuing the query, fetching the first result, and returning.
-
If DavidAM's solution copied "duplicate" records over, then the records were not duplicates in the truest of senses. In other words, values in the other columns were different.
-
[SOLVED] Prevent script fail if mysql table does not exit?
roopurt18 replied to ghostcoder's topic in MySQL Help
It depends on how / what you're using to interact with the database. If, for example, you were using PDO and had it configured to throw exceptions, then you could wrap your code in a try...catch block. -
Explain it to us then, in clear English please.
-
My troll comment was directed towards emopoops. Gayner, I'll repeat, strtotime() returns a timestamp.
-
What are you talking about? time() returns the Unix timestamp. How you can possibly say "don't use the unix timestamp" followed by "use a function that returns the unix timestamp?" Or are you just being a troll?
-
You need to read the documentation on strtotime(). strtotime() takes a string and converts it into a timestamp. Thus strtotime( '-5 days' ) returns the timestamp for exactly 5 days ago. strtotime( '-5 minutes -20 seconds' ) returns the timestamp for exactly 5 minutes and 20 seconds ago. I was using these values to test the validity and correctness of the function I wrote.
-
Some of the contributions in this thread are /facepalm
-
So what you're saying is you're using your production server to find errors, which essentially means you're using your production server as your development server. If you want to develop and test locally, then you need to install apache, php, and mysql locally. There are a great many tutorials on doing this so I won't provide any instructions. XAMPP is a pre-built installation you can use.