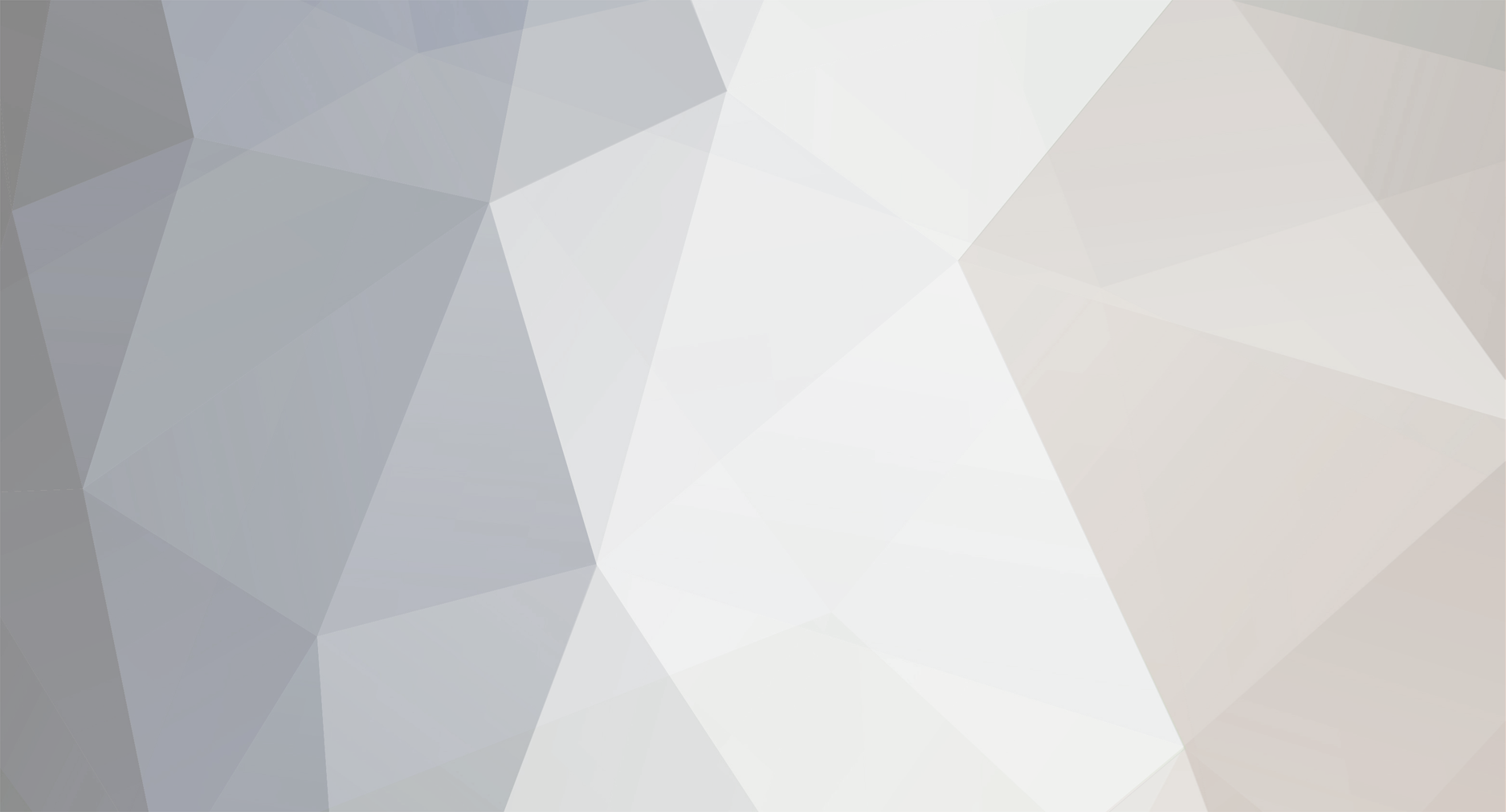
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Wich the best way to control the logic of a web application?
roopurt18 replied to lopes_andre's topic in Application Design
Sounds like a quiz question with possible answers: a) while loop b) do ... while loop c) functions d) if statement -
liNodes.onclick = setSubCatActive(this); I believe that line is in error because in that context this refers to the onload function. I didn't mean my comment on "bad JavaScript" to come off how it did. What I meant when I said, "bad JavaScript" was using code like: window.onload = function() { ... } That is very bad practice and there's no way around it. Similarly, liNodes.onclick = setSubCatActive() is also bad JavaScript practice. What's bad about it? Really what's bad is setting event handlers in that manner. Consider what happens if you write a site and set all of your JavaScript event handlers that way. You later go and install a third party script that uses the same convention. What will happen depends on which order the JavaScript runs in, but for the sake of argument let's say your JavaScript runs first and the third party runs second. What happens is your event handlers all get set. Then the third party code runs and replaces your event handlers with its own. This is why we have the more evolved (and complicated) event model in current browsers: Multiple scripts need to listen to the same events on the same objects and you can not accomplish that if you set events in this manner. My recommendation: Write "raw" JavaScript until you're comfortable with the concepts and ideas, but then learn and use a library because it's loads easier.
-
Is your JavaScript in the head section at the top of the page? If yes, then the browser is running it right when it encounters it and before the markup has been loaded! Your JavaScript should really run after the page has finished loading. This might work: window.onload = function() { var liNodes; liNodes = document.getElementsByTagName("li"); for(i = 0; i < liNodes.length; i++){ if(liNodes[i].className == "subCat"){ liNodes[i].onclick = setSubCatActive; } } } function setSubCatActive(){ this.setAttribute("id","selected") } While that may work, it's very bad JavaScript. I highly recommend using a JavaScript library. I use Dojo quite frequently at work; assuming you were using Dojo, you might accomplish the same thing like so: dojo.addOnLoad( function() { // Keep track of dojo.connects() window.appDojoConnects = []; // Add an event handler to the window onunload event to disconnect all event handlers window.appDojoConnects.push( dojo.connect( window, "unload", function( e ) { // Disconnect all event handlers while( window.appDojoConnects.length > 0 ) { dojo.disconnect( window.appDojoConnects.pop() ); } } ) ); // Add your li event handler dojo.query( 'li.subCat' ).forEach( function( n, idx, all ) { window.appDojoConnects.push( dojo.connect( n, "click", "setSubCatActive" ) ); } ) } ); /** * @param Event object */ function setSubCatActive( e ) { dojo.attr( e.target, "id", "selected" ); }
-
Hello again. I think you meant columns where you said rows, so you might be crazy after all, sorry. MySQL doesn't necessarily work like a programming language. From programming experience, our intuition would tell us d_score is defined first, then c_score, then j_score, so we should be able to use them as identifiers. But that's not the case. There are two ways you can probably work around this. The first is with sub-queries. SELECT a.*, a.`d_score` + a.`c_score` + a.`j_score` as `score` FROM ( SELECT ... `nat_d` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) AS `d_score`, `nat_c` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) AS `c_score`, `nat_j` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) AS `j_score` ... FROM ... REST_OF_YOUR_QUERY ) AS `a` ORDER BY ... What happens here is the sub-query is run first. The results (or records) of the sub-query are then SELECTed from by the outer query just as if they were an actual table in the database (and we gave that "table" a temporary name of `a`). Essentially we are performing the calculations and temporarily saving them so that we can select them again AND use them to perform more calculations. The other way involves MySQL variables like the other queries I helped you with. You'll need to make two calls to mysql_query(). #1 SET @DVAR=0, @CVAR=0, @JVAR=0; #2 @DVAR:=(`nat_d` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin}))) AS `d_score`, @CVAR:=(`nat_c` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin}))) AS `c_score`, @JVAR:=(`nat_j` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin}))) AS `j_score`, @DVAR + @CVAR + @JVAR AS `score` This creates some variables in MySQL (just like PHP variables) that you can use in your queries. So each of those column values is computed and stored in a variable, and then all three variables are used to compute the last column. I'm not 100% sure this will work, but it might.
-
Run this in phpMyAdmin or other such tool to make sure the inner-most SELECT is working correctly: SELECT `name`, `nat_d` + FLOOR( 0 + RAND() * (10 - 0)) + `nat_j` + FLOOR( 0 + RAND() * (10 - 0)) + `nat_c` + FLOOR( 0 + RAND() * (10 - 0)) AS `score` FROM `users` WHERE 1=1 If I had to guess, you're column type for score is a float or double. Those types of numbers are usually displayed in scientific notation, which is what you have there. Where exactly are you seeing those values? In phpMyAdmin? In another script? What do your CREATE TABLE statements look like?
-
http://www.yourdomain.com/index.php?f=../../database.config If you have a file, database.config, two directories up from where you index.php is (or whatever the current directory is when Apache runs the file), then you'll include() it and give me your database credentials. Of course I have to be good at guessing such things, but why leave it to chance? And what if you installed third party plugins / scripts on your site? I might know how those work and where they keep files and I could get your index.php to deliver to me configuration for all sorts of stuff.
-
I'm not an SEO buff, but I believe, amongst other things, search engines prefer pretty URLs, but do not necessarily require them. As long as your links render correctly in an agent with JavaScript disabled, it will find them. You can use mod_rewrite to clean your URLs up; it's an apache module. And make sure you white list things coming in off of $_GET for which PHP files to include or you're vulnerable to attacks.
-
Also, if you later need to know what the random numbers were, then this solution will have to be modified as it loses them.
-
Oops. I had the columns for the INSERT and SELECT in the wrong order. I must have brain damage. Change that part to: // SELECTS, APPLIES RANDOM, ORDERS, RANKS, AND INSERTS ALL IN ONE $qInsert = " INSERT INTO `event` ( `user`, `score`, `rank` ) SELECT a.`name`, a.`score`, @MYCOUNTER:= @MYCOUNTER + 1 AS `rank` FROM ( SELECT `name`, `nat_d` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) + `nat_j` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) + `nat_c` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) AS `score` FROM `users` WHERE 1=1 ) AS `a` WHERE 1=1 ORDER BY a.`score` ; ";
-
Hey they're just getting ready to kill off FoxPro support. Some things take a while.
-
not attach but imbed image within the actually email
roopurt18 replied to jasonc's topic in PHP Coding Help
About as good as you're gonna get I'm afraid. http://www.campaignmonitor.com/blog/post/1759/embedding-images-revisited/ -
oni-kun gave you most of what you need. If you look real carefully at how you phrased your original question and how he provided his answer, you just might be able to figure out what you need to change to get it to work...
-
I don't have the time to help you with your URL regexp, but I can offer a piece of advice. Whenever I try to right a real nasty regexp I break it apart into pieces. $protocol = '(http|https)'; $domain = '(regexp_to_match_domain)'; $url_regexp = "/{$protocol}{$domain}/"; // then combine them together It will take a bit of time to get it right, but in doing it this way you divide and conquer and can easily test any one of the individual parts. Also, read the specification from W3C on URIs.
-
[SOLVED] loading mysql data into a variable
roopurt18 replied to wargolchaos's topic in PHP Coding Help
Take a 5 minute break every hour. Walk around and stretch. It helps. -
My solution might be waaaay over your head, so ask questions. If you can walk away understanding it, then you'll have learned a lot in very short time.
-
If you have MySQL version 4.1 or higher, then this should get you there. <?php /** * THE TEST TABLE I USED TO MAKE THIS create table testjunkin ( name varchar( 12 ), nat_d integer, nat_j integer, nat_c integer ); insert into testjunkin ( name, nat_d, nat_j, nat_c, score_final ) values ( 'Fred', 8, 10, 12, null ), ( 'Bob', 9, 7, 11, null ), ( 'Alice', 11, 2, 4, null ); */ require_once("inc/config.php"); include("inc/header.php"); $connection = mysql_connect($dbHost,$dbUser,$dbPass); if (!$connection) { die("Database connection failed: " . mysql_error());} $db_select = mysql_select_db($dbName,$connection); if (!$db_select) { die("Database selection failed: " . mysql_error()); } // SETS UP A RANDOM VALUE BETWEEN 0 AND 10 $natModifierMin = 0; $natModifierMax = 10; // SETS A MYSQL VARIABLE $qCounter = " SET @MYCOUNTER=0; "; // SELECTS, APPLIES RANDOM, ORDERS, RANKS, AND INSERTS ALL IN ONE $qInsert = " INSERT INTO `event` ( `rank`, `score`, `user` ) SELECT *, @MYCOUNTER:= @MYCOUNTER + 1 AS `rank` FROM ( SELECT `name`, `nat_d` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) + `nat_j` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) + `nat_c` + FLOOR( {$natModifierMin} + RAND() * ({$natModifierMax} - {$natModifierMin})) AS `score` FROM `users` WHERE 1=1 ) AS `a` WHERE 1=1 ORDER BY a.`score` ; "; $success = false; $reason = null; do { if( !mysql_query( $qCounter ) ) { $reason = 'Counter...'; break; } if( !mysql_query( $qInsert ) ) { $reason = 'Insert...Select'; break; } $success = true; } while( false ); echo '<p>'; if( $success === true ) { echo 'Success!'; }else{ echo 'Failed at ' . $reason; } echo '</p>'; include("inc/footer.php"); ?>
-
Here are some general comments on your code, I'll come back later with something else. <?php require_once("inc/config.php"); include("inc/header.php"); // // die() IS JUST ABOUT THE WORST WAY TO HANDLE ERRORS, ESPECIALLY WHEN // COMBINED WITH mysql_error() OR OTHER MESSAGES LIKE YOU HAVE THERE. // WHEN YOUR SCRIPT DIES, IT'S GOING TO DUMP INFORMATION TO THE BROWSER THAT // WILL MAKE YOUR SCRIPT VULNERABLE TO ATTACKERS. // INSTEAD, YOU SHOULD USE set_error_handler() TO REGISTER YOUR OWN ERROR // FUNCTION. WITHIN YOUR ERROR FUNCTION, LOG THE ERRORS TO A FILE SILENTLY AND // KILL THE SCRIPT IF NECESSARY. WHEN YOU NEED TO CAUSE AN ERROR, YOU CAN USE // SOMETHING LIKE: // // if( !$connection ) { trigger_error( "Failed Connection: " . mysql_error() ); } // $connection = mysql_connect($dbHost,$dbUser,$dbPass); if (!$connection) { die("Database connection failed: " . mysql_error()); } $db_select = mysql_select_db($dbName,$connection); if (!$db_select) { die("Database selection failed: " . mysql_error()); } // requesting $result = @mysql_query("SELECT * FROM users"); if (!$result) { // // THIS IS JUST AS BAD AS DIE() // exit('<p>Error performing query: ' . mysql_error() . '</p>'); } // // HERE YOU HAVE A LOOP THAT ITERATES ONCE FOR EACH USER, NOTHING WRONG WITH // THAT. while ($row = mysql_fetch_array($result)) { /* here is where I think I need to add the random score, but then it adds the same score to each user, and I want each user to have their own randomized number */ //I have plugged 4 in for now, but would like the next three lines to read + $random instead of + 4 $score_d = $row ['nat_d'] + 4; $score_j = $row ['nat_j'] + 4; $score_c = $row ['nat_c'] + 4; $score_final = $score_d + $score_j + $score_c; // YOU HAVE NOT DECLARED $assocArray. PHP IS SMART ENOUGH TO FIGURE OUT WHAT // YOU MEANT, BUT YOU SHOULD NOT RELY ON SUCH FUNCTIONALITY. $assocArray[$row['name']] = $score_final; // REMEMBER THAT WE ARE IN A LOOP AND EACH TIME THROUGH THE LOOP WE ARE // ADDING AN ITEM TO $assocArray. IT IS A GREAT WASTE OF COMPUTING POWER // TO SORT SOMETHING WHEN WE KNOW IT IS AUTOMATICALLY GOING TO CHANGE THE // NEXT TIME WE COME THROUGH HERE. THEREFORE THE arsort() CALL SHOULD COME // **AFTER** THE LOOP HAS FINISHED. arsort($assocArray, SORT_NUMERIC); // // THERE IS NO PURPSE TO SETTING THIS VARIABLE. IT IS NOT USED WITHIN THIS // LOOP. IT **IS** USED IN THE FOREACH() LOOP BELOW, IN WHICH CASE YOU SHOULD // MOVE THIS STATEMENT TO JUST BEFORE THE FOREACH() LOOP BEGINS. $i = 1; }; // I CAN ONLY ASSUME HERE YOU ARE ATTEMPTING TO LOOP OVER THE RESULTS // IN THE CORRECT ORDER TO INSERT THEM INTO THE FINAL TABLE. // HOWEVER... foreach ($assocArray as $key => $value) { // ARRRRGH! // YOU'VE ALREADY SELECTED ALL OF THE USERS ABOVE AND NOW YOU ARE GOING // TO SELECT EACH ONE AGAIN? ONE AT A TIME? THERE'S NO NEED TO SLAM THE // DATABASE WITH THIS MANY QUERIES IF YOU'VE ALREADY SELECTED THEM ABOVE. $result = @mysql_query("SELECT * FROM users WHERE name='$key'"); if (!$result) { exit('<p>Error performing query: ' . mysql_error() . '</p>'); } while ($row = mysql_fetch_array($result)) { // ARRRRGH! x2 // YOU'VE ALREADY CALCULATED THESE VALUES ABOVE -- WHY NOT JUST SAVE THEM // THE FIRST TIME YOU CALCULATE THEM? // ALSO, SINCE YOU WANT THESE TO BE REPLACED BY RANDOM NUMBERS, RECALCULATING // THEM HERE WOULD GIVE YOU DIFFERENT RESULTS SINCE RANDOM NUMBERS AREN'T // THE SAME TWICE IN A ROW (UNLESS YOU RESEED THE GENERATOR). $score_d = $row ['nat_d'] + 4; $score_j = $row ['nat_j'] + 4; $score_c = $row ['nat_c'] + 4; echo $i . ' <b>' . $row ['name'] . '</b> | '; printf("%05.2f", $score_d); echo ' | '; printf("%05.2f", $score_c); echo ' | '; printf("%02.0f", $score_j); echo ' | '; echo $value . ' |<br><br>'; /*$sql = "insert into event (rank, score, user) VALUES ('$i', '$value', '$key')"; $query = mysql_query($sql); */ $i++; }; }; include("inc/footer.php"); ?>
-
[SOLVED] loading mysql data into a variable
roopurt18 replied to wargolchaos's topic in PHP Coding Help
You mistyped your variable name: $row = mysql_fetch_assoc($gUser); $_SESSION["level"] = $_row["level"]; // VARIABLE $_row IS NOT DEFINED -
[SOLVED] pulling my hair out over a mysql query
roopurt18 replied to evanct's topic in PHP Coding Help
No problem. Just remember this simple step for future reference: You always have tools to help you locate problems. These tools are typically front-ends to the technologies that we write specialized applications for. In most cases we can be reasonably confident that the tools (php, pgAdmin, phpMyAdmin, jslint, validators, etc) are solid and work correctly. In other words, it's much more likely there is a problem with YOUR | OUR code than there is MySQL, PHP, etc. Whenever you have a problem, try and break it down into solvable steps. In your case you had a query that appeared to not work. Whenever I'm faced with a similar situation (a non-working query), I immediately run it directly in the database. By doing so I accomplish the following: 1) I remove all of my PHP code, logic, and settings from the problem. 2) I immediately see if the query has errors (from the database front-end) 3) If the query runs without errors, I immediately see the results I should be getting 4) If the results are not what I expected, then I know my query is wrong, although it is syntactically correct. So in your case the query worked (no errors) in phpMyAdmin and returned an empty result set. At that point you don't even need to look for errors in your PHP code because the query itself is broken. Once the query is fixed in phpMyAdmin, you can put it back in your code. And then you are likely to encounter more errors that may be PHP-related, at which point you need to use more tools to find the problems. -
If you have output buffering turned on, then PHP will internally buffer the file before sending it to the client. If that is the case, it doesn't matter how you try and break the file up in your code because PHP will still be holding onto it until you run out of memory. I've seen, but do not have handy, solutions where all output buffering is turned off and in your file read/echo loop you constantly echo and flush so it is all sent immediately. However, there may be a more simple solution if you're on a *nix OS, which may not work but could be worth a shot. Apache should resolve links to the actual resources, so when a file is requested just create a new link to it and redirect the user to the link. Then create a cron job or something else to periodically remove links. Like I said, it may not work at all, but I'd try it if it's appropriate to your environment.
-
[SOLVED] pulling my hair out over a mysql query
roopurt18 replied to evanct's topic in PHP Coding Help
Alternatively you could give `status` a default value, such as 'good' -
[SOLVED] pulling my hair out over a mysql query
roopurt18 replied to evanct's topic in PHP Coding Help
You have misunderstood, or not been told, about how MySQL treats NULL. In most SQL database system, NULL is not equal to anything, not even to itself. Further, performing operations against NULL values such as math, concatenation, date operations, or, in your case, comparisons, will always result in NULL. So your for those records where `status` is null, your WHERE clause is becoming: WHERE `parent`=0 AND `status`=NULL -- leads to WHERE `parent`=0 AND NULL -- leads to WHERE NULL Since NULL is not equal to anything and it is certainly not equal to TRUE, the query does not return them. Try this: SELECT * FROM posts WHERE `parent`=0 AND (`status`!='deleted' OR `status` IS NULL) ORDER BY votes DESC LIMIT 0, 10 -
[SOLVED] pulling my hair out over a mysql query
roopurt18 replied to evanct's topic in PHP Coding Help
If you ran it in phpMyAdmin and got zero rows and zero errors, then there's only two conclusions I can draw: 1) Your query is syntactically correct 2) There are no rows that match your WHERE criteria -
IS NOT NULL does not return null fields. They are either empty strings in the database or you are some how converting them from empty strings to NULL values.
-
[SOLVED] pulling my hair out over a mysql query
roopurt18 replied to evanct's topic in PHP Coding Help
Have you run that in phpMyAdmin or a similar tool to ensure it's working as expected? Did you remember to connect to the database? Still need to see more code.