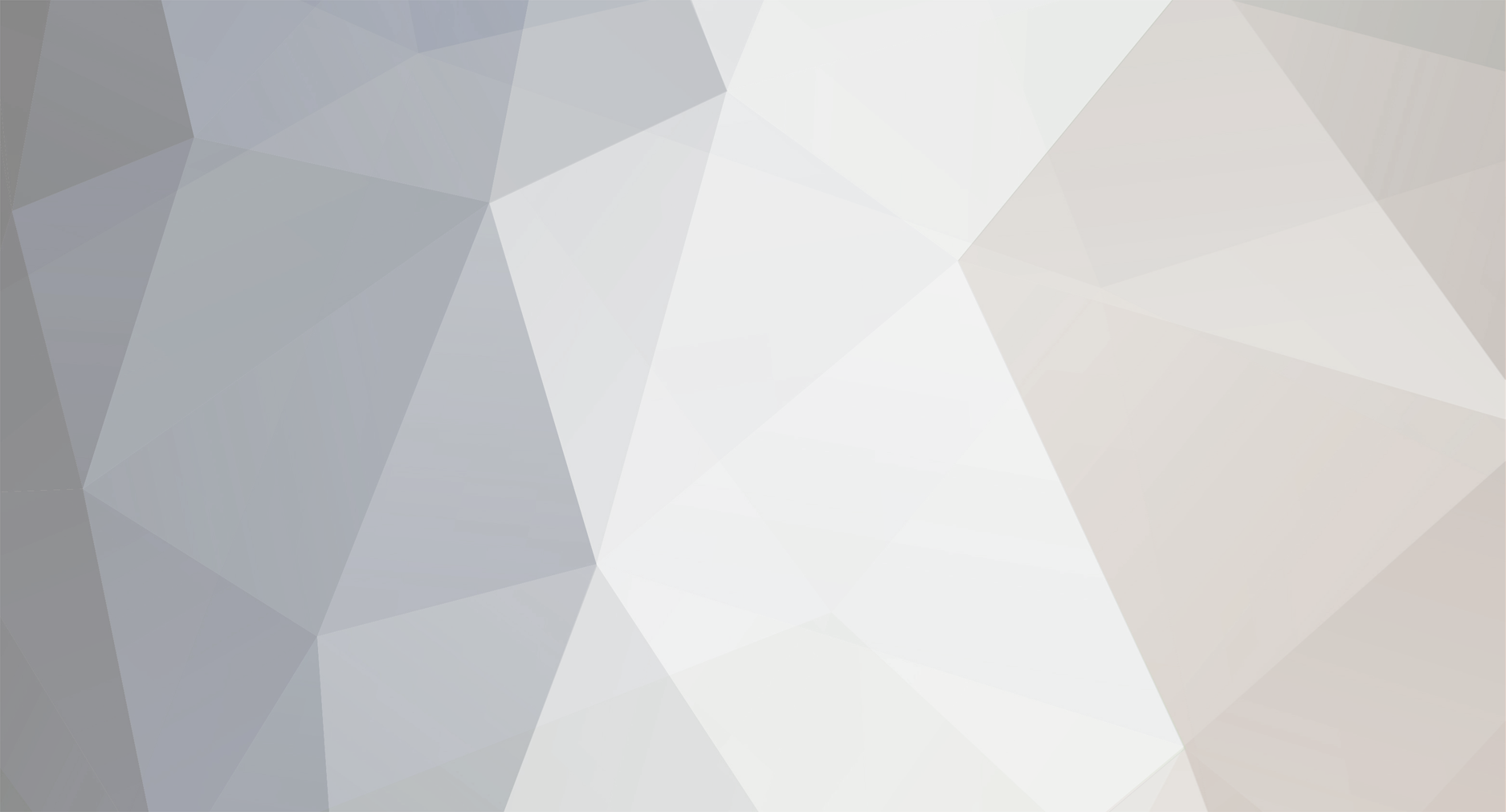
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
If you do some searching for "display users online" then you'll find any number of solutions on how to accomplish this. It deserves it's own topic really.
-
Perhaps there's a better way, but: <?php $foo = 38.88; $foo = 38.880001; $places = 2; $mult = (int)pow( 10, $places ); if( ((double)$foo) === ( (double)(((int)(((double)$foo) * $mult)) / $mult) ) ) { echo 'no ceil!' . "\n"; }else{ echo 'ceil' . "\n"; } ?>
-
Programs execute sequentially starting at the first statement and thereafter executing a single statement at a time. Therefore your code always executes in the order that you have written it in the file. Unless! The exception is when you use code branching features, such as if-else statements or loops. In the case of if-else statements, some pieces of code will execute and others will not. In the case of loops, the code will execute sequentially (just as programs always do) starting with the first statement of the loop body, then the next, and the next, until it hits the loop-body end and starts the loop over again. Eventually the loop itself comes to a finish and code continues executing sequentially one statement at a time as it always does. When PHP loads your program, it reads the entire file one time to parse it. This is where PHP checks the syntax of your file for errors and determines the locations of loops, if statements, and other constructs. It only notes their location, it does not execute them. Then your program begins actual execution. Only lines that need to be executed are actually executed. If you have blocks of code that are not accessible, even though they're there, they do nothing. Example: if( false ) { include( 'somefile.php' ); // PHP will note that this line of code is here, but it will NEVER execute it }
-
I believe PHP may be doing something funny because you are using a string in your arithmetic. I know my debugger showed a wrong calculation. Try this: $uscos = 9.6000; $a = 4.0500; $aRate = $uscos * $a; $aTotal = ceil(100 * $aRate) / 100; echo number_format( $aTotal, 2, '.', ',' );
-
Just to make sure I understand. You have a `topics` table with N records, where N is an arbitrary integer. For a given user, U, you want to duplicate the existing N topics records but with U's user id? If topics is: UserID TopicID done Joe ABC 0 Joe XYZ 0 Joe LMNO 1 And the user ID is 'Betty', then you want topics to look like: UserID TopicID done Joe ABC 0 Joe XYZ 0 Joe LMNO 1 Betty ABC 0 Betty XYZ 0 Is this correct?
-
This is from my immediate window in my debugger: ceil( "3888" ) : double = 3888 100 * "38.88" : double = 3888 ceil( "3888" ) : double = 3888 ceil( "3888.00000" ) : double = 3888 100 * "38.88" : double = 3888 ceil( 100 * "38.88" ) : double = 3889 Very strange IMO.
-
I'm surprised it works at all since you have a syntax error and all.
-
A code profiler can give you a better estimation of where the big slow down is more than anything else. I don't suppose you have anything nifty like that?
-
UPDATE `completed` SET UserID='theuserid' WHERE `done`=0;
-
Why don't you just use a UNION in MySQL and have the database sort it? Then you only need to make one database call.
-
I need to grab a specific array item without using key and value
roopurt18 replied to scrubbicus's topic in PHP Coding Help
$_POST is already an array. Why exactly do you not want to have to use the array indexes? It sounds like you're solving something the wrong way. -
lol @ the 'linux windows' I'll give credit where credit is due, at least they picked a good name. And by that I mean the name does not describe in any way the function, which seems to be the trend in the largest and most successful endeavors.
-
$total = '1,244.70'; PHP will convert this to a number before subtracting. '1,244' converted to a number is 1. http://www.php.net/language.types.type-juggling
-
Because he's building a PHP string and concatenating the value of variables to it: <?php $sql = "UPDATE member SET paydate=CURDATE(), status='regular', payamount=payamount+" // DOUBLE QUOTED PHP STRING . $amount // CONCAT A PHP VARIABLE . " , validitydate='" // DOUBLE QUOTED PHP STRING . $validitydate // CONCAT A PHP VARIABLE . "' WHERE id=" // DOUBLE QUOTED PHP STRING . $_SESSION['userid'] // CONCAT A PHP VARIABLE ; // END OF PHP STATEMENT ?> Everything I said was matter-of-fact, no sarcasm included.
-
Have a look at creating a pivot table. You can turn your data into a format that has columns: teamname, week1, week2, week3, week4, ... weekN Then you can build an HTML table as you normally would with a result set. http://en.wikibooks.org/wiki/MySQL/Pivot_table
-
Sounds like you answered your own question. If you understand correctly how an SQL UPDATE statement should look, how to give money and datetime data to a database, and how string concatenation in PHP works then it shouldn't be confusing at all.
-
I'm pretty sure daniel was just picking on laffin's lazy typing skillzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzzz. What laffin meant is how do you intend to know when a user has logged out? It's easy when they actually click a Logout link, but what if they just walk away from the computer and their session expires? You have to detect that. Likewise if you keep adding features or optimizations that you have not found to be necessary. The rule in software design is add only what is necessary and if a certain feature may need to be changed later, then encapsulate it's functionality so that when you do change it, the rest of your code keeps working on top of it.
-
We need to see a little bit more code and a little bit more of your program's output to help, because it sounds like it should be working. But it's not, so you've made a mistake elsewhere.
-
Read the user comments on: http://www.php.net/htmlspecialchars They will help explain when to use one or the other. If you have further questions feel free to post them afterward.
-
Hehe What I meant was I'm not helping you fix the problem you asked for help with until you fixed your other more serious problems.
-
There's your problem right there. You're "optimizing" without any evidence that it's necessary; I surround optimizing with quotes because you aren't even sure the performance gains will be worth it in your case. You should just write it now with the database as the solution and use it that way until it breaks, which may never happen. And when it does you may be able to fix it by adjusting some configuration parameters somewhere, a small hardware upgrade, or something else. If you're worried that fixing it later will require a code rewrite, then this is where encapsulation is your friend. Write a PHP class to encapsulate the storage mechanism of this page, call it UsersOnXYZPage. Then in your xyz.php page, whenever you need to know which users are online, edit which users are online, or delete users online, you use methods exposed by your UsersOnXYZPage class. Your UsersOnXYZPage class will handle the storage mechanism, be it database, file system, RAM disk, cache, telekinesis, or fibble-fobbles. Should you later need to rewrite the storage mechanism, just rewrite the underlying structure of your UsersOnXYZPage class to no longer use the database, but to use whatever storage solution is deemed most appropriate at that time. Since your xyz.php page only access data through the UsersOnXYZPage class and the methods in UsersOnXYZPage don't change (i.e. the interface remains constant), then everything else should just keep working. That's how I'd do it anyways. You could spend endless hours optimizing or adding features that you don't even know are necessary. Or you could write flexible and expandable code and only work on what's necessary to get the job done knowing you can easily modify it later.
-
I'm not telling you anything until you properly escape your database inputs: mysql_real_escape_string()
-
Good point, although I took it on assumption that he's not writing anything that actually processes credit card information. Someone who was probably wouldn't have posted this topic in the first place.
-
I'm really not sure why you're against using the database for this. A single table with 500 to 1000 rows with one or two columns and no indexes should have decent enough performance; you don't need the index because on tables that small I think many database systems will just perform a full table scan anyways. Plus with the database you don't have to worry about users fighting over a file. Perhaps the APC solution will work for you; I don't know anything about it though. Lastly, you could create a RAM disk, which works in both Windows and *nix. This will eliminate the need to read and write from the HDD constantly so performance should be greater. Also, since the disk lives in RAM, if the server goes down or is rebooted all of the files there will go away so you're not left with crap all over the place from a crashed system.
-
That's essentially it. INSERTING INTO THE DATABASE 1) Does the data need to be protected (i.e: passwords, credit cards)? a) If yes, encrypt or hash the data using your appropriate algorithm. 2) Call the appropriate escape function for your database (i.e: mysql_real_escape_string()) 3) Insert into the database EXTRACTING DATA FROM THE DATABASE TO DISPLAY IN A WEB PAGE 1) Select the data from the database 2) Does the data need to be protected (i.e: show XXXX-XXXX-XXXX-1234 for credit cards, show ********* for passwords) a) If yes, protect the data however necessary 3) Call the appropriate escape function for display as HTML (i.e: htmlentities(), striptags()) 4) Display the HTML EXTRACTING DATA FROM THE DATABASE TO DISPLAY IN AS PLAIN TEXT, OR IN A NON-HTML REPORT (PDF, DOC, XLS) 1) Select the data from the database 2) Does the data need to be protected (i.e: show XXXX-XXXX-XXXX-1234 for credit cards, show ********* for passwords) a) If yes, protect the data however necessary 3) We no longer have to escape for output 4) Produce the output (i.e: plain text, XLS, DOC, PDF)