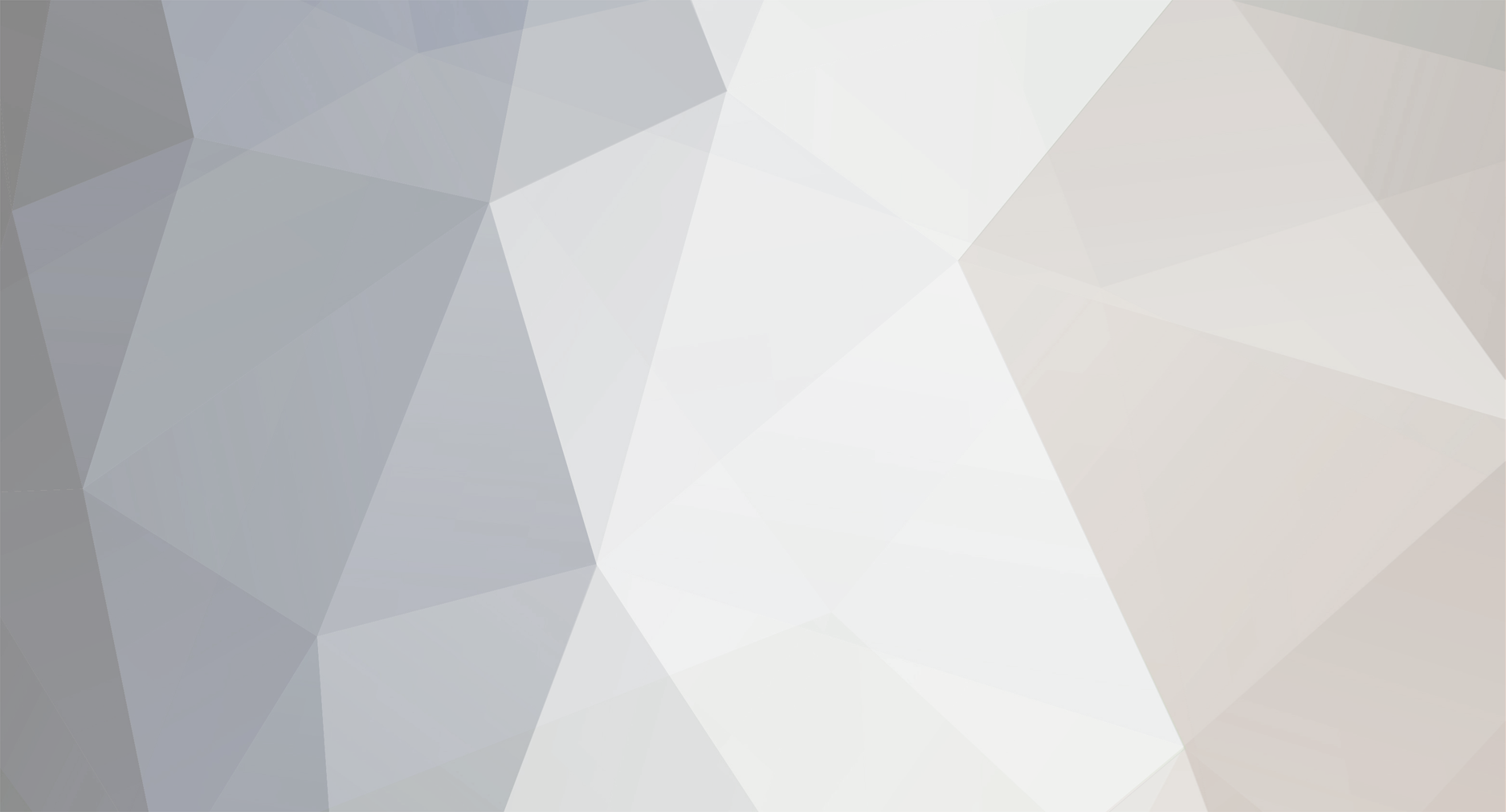
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
This should be locked.
-
WEird Combination of fwrite and simplexml
roopurt18 replied to cardio5643's topic in PHP Coding Help
<?php // Change to absolute paths, relative paths have a chance of burning you if you're not careful $inFile = '/etc/input.raw.txt'; $xmlFile = '/etc/input.xml'; $in = file_get_contents( 'php://input' ); file_put_contents( $inFile, $in ); // This is your raw input, run it through an XML validator and make sure it's valid $xmlObj = new SimpleXMLElement( $in ); $xmlObj->asXML( $xmlFile ); // Should be the same XML as $inFile, but not necessarily same indenting ?> I'm not even sure why you need to use SimpleXML at all though, unless you are interested in validating. Seems you could just as easily do: <?php file_put_contents( '/etc/someXml.xml', file_get_contents( 'php://input' ) ); ?> -
You're better off leaving the rows numerically indexed; otherwise you'll have to loop over the columns in a row more than once. When you say update a database, do you mean you are inserting or are you inserting and updating duplicate records? Also, which database are you talking about here? Many databases provide a way to bulk insert records. In that case it's often easier to bulk insert into a temporary table and then perform the updates with a couple of SQL statements rather than writing a PHP script.
-
Do file* functions accept full directory-paths?
roopurt18 replied to Goldeneye's topic in PHP Coding Help
There's some stuff in php.ini that controls whether or not PHP will open files given as URLs, so you might look into that. Otherwise full paths work, for example: c:\a\path\to\this\file.txt /home/users/joeboo/myfile.txt -
You're the first one to bring up confusing the server, so I'm not sure what you're talking about.
-
Since you're new I'll give you a small piece of heads up in terms of security. Do not send sensitive information over GET because the web server will typically log requested URLs, including the GET variables. Because logs are plain-text and all sorts of crazy things happen to them (they get archived, they're sent to dev teams for debugging and error fixing, etc.) it's best to not have anything sensitive in them. I hope that makes sense.
-
Which PHP-Editor do you think is the best?
roopurt18 replied to briananderson's topic in Miscellaneous
[quote]I prefer tabs over spaces personally (and if I'm not mistaken, I think it saves on filesize that way as well).[/quote] A file with tabs should be smaller than one with spaces. But who is really concerned if the file is 1.4kb or 1.5kb in an age of TB servers? I prefer spaces over tabs because there are occasions when you have to view a file in something other than your favorite editor. When this is the case, not all viewers use the same width for tabs and some of them use extraordinarily wide tab characters, which make it harder to see all the code at a glance. Spaces are almost always the same size though, which means my code always looks the same no matter what I open it in. -
Which PHP-Editor do you think is the best?
roopurt18 replied to briananderson's topic in Miscellaneous
I've used: Zend Studio The last version I used actively for development was version 5 I believe, or the last compiled version right before they switched to an Eclipse IDE. It was solid and I liked it, but not without some problems. I did play briefly with their Eclipse version and at first glance was impressed, but never did any heavy development in it. PDT 2.0 Slow, slow, slow. There are a lot of things I like about this editor but the performance on my dev machine at work was terrible and unusable at times. PDT 1.03, 1.05 These versions of PDT are much better than the 2.0 to date. In fact the Zend IDE is based on PDT 1.05 from what I hear. I used these for a long while at work until my projects must have hit a size barrier for my dev machine or something because the performance eventually started to degrade. NuSphere's PHPED I use this exclusively at work and it's very fast and snappy. The debugging is very easy to set up with a 3rd party web server, which is a big plus. It'll even break on XHR requests which is also nice. There are a couple of features I really dislike about this editor, most notably the default options in the 'Find and Replace' dialog; they make me want to pull my hair out! NetBeans I just installed this a few hours ago because there is a project I want to work on at home. I must say I am greatly impressed. + It defaults to spaces over tabs for indents + Debugging worked right out of the box, I didn't have to do anything (not even to get it to work with a 3rd party web server) + I like the phpDoc hints with extended descriptions about what they mean (yah yah I could look it up elsewhere but I'm lazy) + I like that it will copy my source files into another directory when I save them (one of my favorite features actually) Give me some more time with it an I might find some things I dislike about it. I'd say for me it's currently a tie between NetBeans and PHPED and if you didn't want to shell out any cash then NetBeans all the way. Now if the PDT team could get some better performance out of that monster I might change my mind. -
What frameworks does the majority of phpfreaks use?
roopurt18 replied to littlevisuals's topic in Frameworks
I spent a little time looking at cakephp and zend framework, but to be honest I didn't like either of them. There's too many ways to accomplish the same task and not enough clear examples on which is the best supported, which is deprecated, etc. Also since portions of the framework are designed by different people they don't always follow the same code conventions. I spent probably a week or two on each one and really just found them confusing. When I started at my current job I wrote my own framework in a couple of days that accomplishes everything I need. It uses a Controller-View design and no Model. I've slowly added onto it over time and I'd say it's probably got 10 or 12 classes and half a dozen libraries (static classes). Setting up a new site takes me about half an hour and from then on out I only have to work on the site at hand. Basically I couldn't justify spending weeks of my time learning ever-changing existing frameworks when I knew I could write one better suited to my needs in less time. Lately I've been toying with the idea of incorporating Propel or Doctrine into my projects, but I really can't seem to justify it. -
I'm going to blow you all out of the water with my development machine at the office. Steady... Steady now... Wait for it... Pentium 4 3.06Ghz 1GB Ram GeForce 6600 LE 2x Samsung SyncMaster 2053 BW (20inch?) 150 GB HDD, Probably a single IDE @ 7200RPM, maybe 5400RPM though because it struggles sometimes WinXP It actually had 512MB of RAM when I started working here, but I brought in 2x512MB sticks I had at home. Shame the motherboard only has 2 slots though. I keep telling my boss to give me a small budget and I'll cherry pick a machine off NewEgg that'll shame every workstation in the office, but he won't do it. Every time I tell him what a turd it is he says, "We bought you a new motherboard before you got here for the dual monitors!"
-
I don't use any AV. As far as the OS debate, I'd prefer to run Linux but I'm stuck running Windows because I like to play games and stream movies over NetFlix. Dual-booting is out because it's just a PITA after a while. I ran Photoshop CS2 on Ubuntu for a while and it did perform acceptably; however there were some GUI glitches that were annoying. A Linux OS with Windows VM is out of the question for gaming as well; at least last I checked there is no VM that can give full hardware control to the virtual installation. Windows will run its application better in a VM from my experience though. I believe this has to do with Linux having better memory management and only creating a swap file when it's actually out of RAM, as opposed to the Windows OS which creates a swap file and uses it no matter what!
-
Going further, NULL isn't equal to anything, not even NULL. If you want to prevent NULLs and empty strings, then: declare the column not null do not specify a default value add one of the following, depending on what your database implementation supports: check constraint insert and update triggers
-
session_start() hangs randomly only for a few users
roopurt18 replied to fats's topic in PHP Coding Help
If you ever get this entirely resolved could you please remember to update this thread with the problem and solution. I like to tuck little tidbits of experience like this away for future reference. -
Holy crap! It looks like the puppet from Tales from the Crypt.
-
Dogs are really only scary if you have more than one. A determined and unarmed invader / attacker could get through one dog but probably not two. Some people say pit bulls are good guard dogs, but I just don't see how with how short they are. By far the best dogs my family ever owned growing up were a pair of rottweilers. They were easily the most obedient and calm dogs of any we owned. They are protective as well and their size puts them at small enough to be controllable by their owner and large enough to be a deterrent. Lastly, don't go hanging any "Beware of Dog" signs around your property or vehicles.
-
I always tell people to learn C when they ask these things.
-
Make sure you know some dirty songs with clean language! Kids love those.
-
[SOLVED] Question about speed mysql storing vs php array storing
roopurt18 replied to pkedpker's topic in PHP Coding Help
If there's a PHP optimizer installed it may just optimize that kind of stuff away; it's hard to say exactly what the real impact could be without benchmarking. If he's always using all of the strings then having them in a PHP array is probably more efficient than always pulling all 500 out of the DB. My concern is why are you pre-optimizing your code? Write it so that it works and let it go. If you encounter problems down the road then do some profiling and fix the actual problems. -
is unobtrusive JS/AJAX in personal dev tools necissary?
roopurt18 replied to Derleek's topic in Miscellaneous
Oops. Rereading my post I see I didn't say what I wanted to correctly. Yes JS should be unobtrusive. If you want to reach the widest possible audience, then JS should be optional. If you are developing a product, then JS can be made a product requirement but it should sill be unobtrusive. -
select a.*, (select user_email from phpbb_users b where a.topic_poster=b.user_id) as first_poster_email, (select user_email from phpbb_users c where a.topic_last_poster_id=c.user_id) as last_poster_email from phpbb_topics a Or maybe: select a.*, b.user_email as first_poster_email, ifnull( c.user_email, b.user_email ) as last_poster_email from phpbb_topics a inner join phpbb_users b on a.topic_poster=b.user_id left join phpbb_users c on a.last_poster_id=c.user_id
-
You could also try: select a.* from ( -- 25 random records select *, (floor( 1 + rand() * 60))) as randomtimer from downloads order by rand() limit 25 ) as a order by a.randomtimer Note sure if that could be written any better.
-
I didn't even know what Twitter is until I read this. Thank you CV for this public announcement!