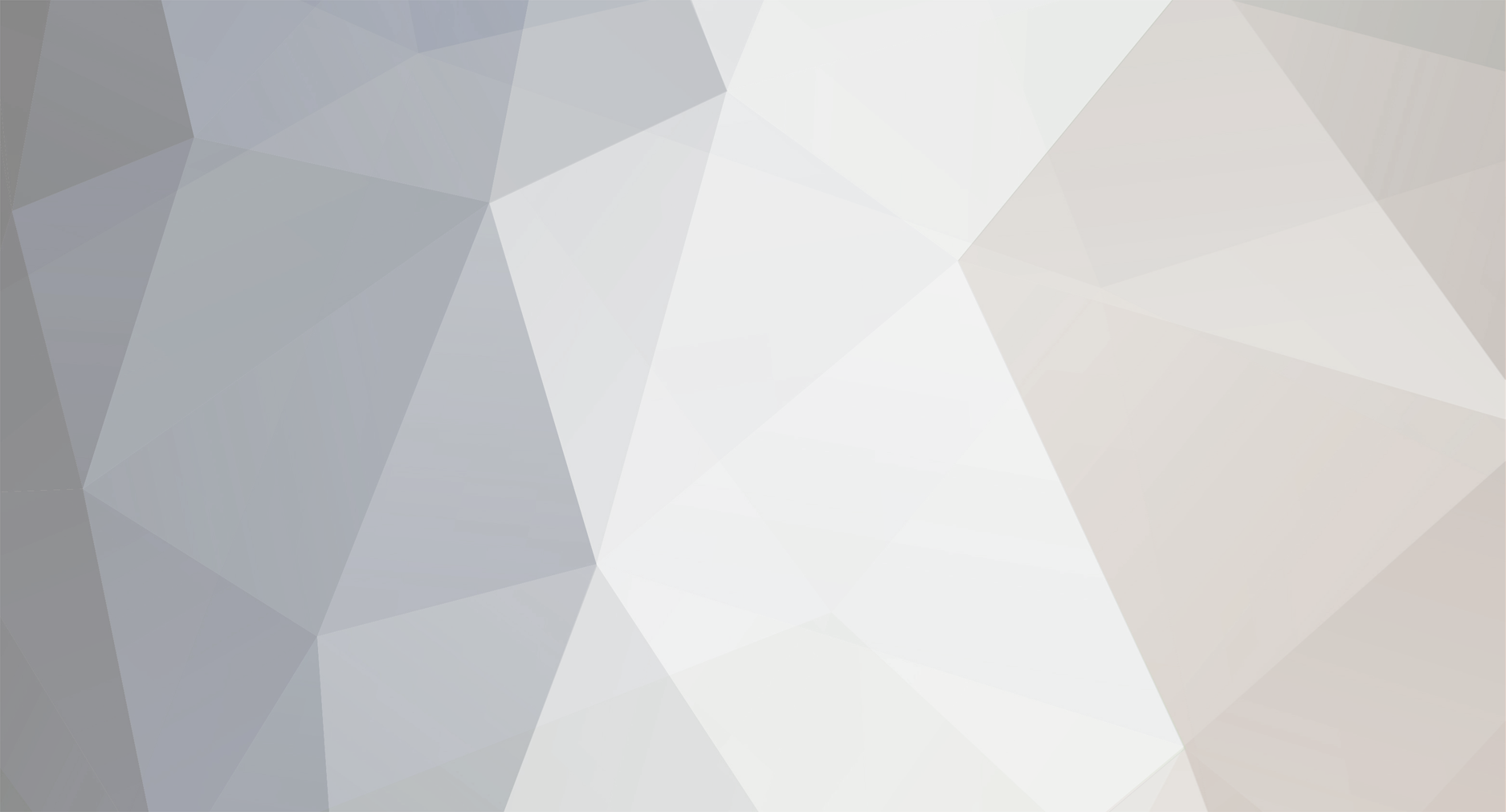
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
is unobtrusive JS/AJAX in personal dev tools necissary?
roopurt18 replied to Derleek's topic in Miscellaneous
It depends on how big your audience is intended to be. If your code is meant to be distributed in the widest possible channel, then yes it must be unobtrusive and work both with and without JavaScript. If you are developing a product, then you can simply say one of the product's requirements is that JavaScript is turned on. -
If you added Dojo to your application, then you might achieve your validation with the following JavaScript: /** * Called when the form is sumitted. * * @param Event */ function myFormSubmitHandler( e ) { var valid = true, possible_rows = dojo.query( 'tr', dojo.query( 'form[action="roster.php"]' )[0] /* You can probably replace this query with some property of argument e, such as e.target */ ), i, k, input_qry, count_empty, count_not_empty; for( i = 0; i < possible_rows.length; i++ ) { // For each possible TR row, make sure it is one with immediate input[name=player*] children input_qry = dojo.query( 'td > input + input[name^="player["]', possible_rows[i] ); if( input_qry.length === 0 ) { continue; } // input_qry[i] is a valid TR row with inputs. input_qry = dojo.query( 'input[name^="player["]', possible_rows[i] ); if( input_qry.length === 0 ) { continue; // This should never happen } count = 0; count_empty = 0; count_not_empty = 0; for( k = 0; k < input_qry.length; k++ ) { if( dojo.attr( input_qry[k].name ).indexOf( '[customer_id]' ) ) { continue; // Skip hidden inputs of customer ID } if( dojo.trim( input_qry[k].value ).length === 0 ) { count_empty++; }else{ count_not_empty++; } } if( count_empty > 0 && count_not_empty > 0 ) { alert( 'you must fill in all of the rows!' ); valid = false; } } if( valid === false ) { e.preventDefault(); } } // When the page loads, we add an onSubmit handler to the form dojo.addOnLoad( function() { var qry = dojo.query( 'form[action="roster.php"]' ); if( qry ) { dojo.connect( qry[0], 'onsubmit', 'myFormSubmitHandler' ); } } ); It's not the best written and probably won't even work as-is, but it may give you an idea. That's about as much as I can help you with JS validation though. You may think about posting a topic in the JS forums.
-
I actually didn't even know keys could be disabled. Learn something new all the time!
-
Can you post the XHTML that PHP produces for the form? Or is this page live so that I can just view it?
-
PHP doesn't keep the values of variables from page to page. Every page request from the user starts with a "clean slate" in terms of variables and their values. The only way to save values from page to page is to use some form of persistent storage; for web applications that is typically done with sessions or with the database. I hope that explanation helps somewhat. My apologies for not being as helpful the past few days; I had less downtime at work and I've been enjoying every moment of my free time away from the computer.
-
I'd recommend downloading a JavaScript library. I'm the most familiar with Dojo so if you added that to your project it's a breeze. Otherwise, yah, you got a bit of work in for yourself.
-
Well the answer is obvious: They're not the same. Even looking at the source in two different Google Chrome tabs I can see that one jumps down a line and has an extra character in it. Try using WinMerge to see where the difference is; it's an open source file compare utility.
-
And you'd be right. I guess I could have been more clear and said within a particular type or class of programming language, the general concepts don't change.
-
You should get smashed tonight.
-
My wife has Vista Home and it works just fine. I turned off her UAC because it bothered her. The only real complaint I have when I've used it is the smaller tree hierarchy in Windows Explorer because they added that extra crap on top. I imagine if it were my OS though I'd be used to it by now. MS is only a giant in terms of workstations and personal computers. In the server market they're still way behind and likely always will be. Their online presence is trumped by Google. A large section of Microsoft's PC market would disappear if games were released on *nix systems. The business world is moving back towards the model of server-run software that is connected to by dumb clients (i.e. web browsers) so it could be in another five or ten years it doesn't matter what OS is installed throughout a company. In terms of their products, no company, none whatsoever, can compete with MS programming IDEs. If MS made a PHP IDE you can bet I'd buy that in a heartbeat.
-
You may have to enable it in the BIOS? Most hardware manufacturers have support forums on their websites these days; I'd probably ask this question over there. These guys also know their stuff: http://forums.extremeoverclocking.com/ Every 2 to 4 years I build myself a brand-new PC without any recycled parts. PC hardware changes rapidly and I don't really keep up with what's the latest and greatest. So every time I'm about to build one I head over to those forums and do some research. I'd be surprised if someone over there couldn't answer your question.
-
Ditto to what waynewex said. I've been programming for close to 15 years now and I think I can accurately outline the learning hurdles you need to overcome: When initially learning you are presented with programming concepts in general and a specific programming language. Programming concepts are things like functions, arguments, return values, pass by value, pass by reference, memory management, references, pointers (maybe not so much any more), algorithms, and then finally more advanced topics like object oriented programming, abstract data types, advanced algorithms, etc. It will take some time before you can effectively use these concepts to solve a particular problem; you actually have to train your brain to think a little differently about things. A specific programming language is all about how it implements those general concepts. An example would be all PHP programs start with <?php or PHP functions begin with function theName() { or that PHP uses references rather than pointers. Really it's about the syntax, which is what you have to type, where you have to type it, and what it has to look like. The beautiful thing about programming is the general concepts do not change from language to language, only the syntax. A real programmer can easily learn new languages and write the same programs in many languages because all that changes from language to language is syntax (and a few other silly things sometimes). I've known a few people that could program one language but were unable (or maybe just unwilling) to learn a new language, saying it was "too difficult" to get around the different syntax. IMO they're not really programmers. As a beginner the general concepts you need to be familiar with are: variables, functions, loops, conditional statements, input and output. Once you know how those all work in PHP, start writing small programs. After it's finished, think about how you could have written it differently. Ask yourself, "What design decisions did I make in this program that made programming it harder than it needed to be?" Ask yourself if the program is easy to maintain. Do you think you could open the file in 6 months and follow your own code? Then actually open the file 6 months later and look at your old program and critique it then. As I said I've been doing this for a while. Once you overcome the hurdle of learning about general concepts, the rest is just how a specific language accomplishes a specific task. The only real way to get better is to write lots of programs and constantly try to make them better!
-
Halo 3 Saved Film HD Recording Service - Testers Needed
roopurt18 replied to HaLo2FrEeEk's topic in Beta Test Your Stuff!
You should always validate your XHTML and CSS and run your JavaScript through JSLint. You don't have to do it after every single change, but you should do it once per hour or so. Small errors in XHTML, CSS, and JavaScript can affect a page's behavior quite significantly. Screw up your XHTML and the CSS rules may not be followed properly. Goof up in your JavaScript and it may do all sorts of weird things. Have you ever added a CSS rule that didn't work properly? So you end up having to add more and more CSS rules or maybe extra markup to get it working? Perhaps the original rule didn't work because of your invalid XHTML. So you write all this extra crap trying to get it pretty when if you'd just validated all your stuff it probably would have worked from the get-go. But sure. You can validate at the very end after you've already wasted countless hours "hacking" around the results of your own carelessness. -
Just curious, did you consider any of that?
-
Might you post the full code; it might be easier to solve that way.
-
Make sure your table stats are up to date; I'm not sure what the exact command in MySQL would be but in general the database system keeps analytical stats on its tables. It then uses these stats to determine if the use of an index may be appropriate. If the stats become too far out of sync with the index, then the indexes are not used. You may also have too many indexes and may be better off combining them. MySQL will also skip using the table if all of the data it needs lies within the index. For example, in your query: SELECT post_id, topic_id, forum_id FROM phpbb_posts WHERE topic_id = 51544 Sure, MySQL can use your topic_id index, but it's still going to have to pull post_id and forum_id from the actual table. For that particular query, an index like this may be more appropriate: create index idx_topic_forum_post on `thetable` (topic_id, forum_id, post_id ) Since the three requested columns exist entirely in the index, MySQL can skip looking at the actual table altogether. Also keep in mind that the more indexes you have, the slower inserts and updates become.
-
What rhodesa said. In all of my programs I use set_error_handler() to define my own error handler that logs errors to a file. When a piece of code becomes deprecated, I perform the following: function DoSomething() { trigger_error( 'DEPRECATED: ' . __FUNCTION__ . ' is deprecated, use DoSomethingNew() instead.' ); DoSomethingNew(); } function DoSomethingNew() { // Replaces DoSomething() // Function body follows... } Then once per day, week, or month I have the error log e-mailed to me and start a new one.
-
Returning a different varable for every stage in a loop
roopurt18 replied to ldb358's topic in PHP Coding Help
Just to expand: $output$i = "<input type='button' value='$name' onClick=\"mainImage.src='" . $username . "/" . $name . "' \"/><br/>"; return $output$i; Surely that gives you syntax errors. You can't have code like $output$i and expect it to work. Secondly, return immediately ceases execution for the current function and returns control back to the code that called the function. So if you want to return a bunch of things from a function, combine them into an array or an instance of stdClass() and return that. Some things to remember: 1) A function can have any number of return statements 2) It's considered good practice (by some) to limit your functions to a single return statements 3) Regardless of how many return statements you have, once your function reaches one, it's done 4) You can only return a single value from a function, but that single value can be a single collection of items, like an array. -
<td align="center"><?php if ($recordsFound){ echo '<a href="update_general.php?recordID=$row_current['cls_id'] &cls_item_var=$row_current['cls_item']">Update</a>';}?></td> If you want to embed PHP variables inside a string, then the string must be enclosed with double quotes, not single quotes like you have there. Whatever you start your string with (single or double quotes), then that same character must be escaped with a backslash within the string. I.E: You can output a single double quote: echo '"'; // single-quoted string, so no escape sequence necessary for the enclosed double-quote echo "\""; // double-quoted string, so escape sequence is necessary for the enclosed double-quote <td align="center"><?php if ($recordsFound){ echo "<a href=\"update_general.php?recordID={$row_current['cls_id']}&cls_item_var={$row_current['cls_item']}\">Update</a>";}?></td> When placing variables in a double-quoted string, it is good programming practice to enclose the variable in curly brackets. $name = 'Joe'; echo "Hello, $name\n"; // BAD practice echo "Hello, {$name}\n"; // GOOD
-
1) User requests a login link 2) Unique token is generated and attached to user's database record. 3) Unique token is mailed (as a link) to the user's e-mail 4) User logs into their e-mail and clicks the link 5) Your site validates the user's token, logs them in, and removes the token from their database record (making the token one-time use). Yes that should be ok. It's the same type of system most sites use for password recovery. However, I still don't see why you jump through all these hoops. This is inherently no more secure than if the user just logged into the site using a "regular" login system. Look at step #4 above: User logs into their e-mail. So they still have to log into something, regardless of it being your site or their e-mail or whatever, they still have to go through the step of typing in a username and password. Again, if it really needs to be secure, use HTTPS. Consider this, regardless of the login mechanism, once the user is logged in if someone is sniffing network traffic then the sniffer can still hijack the session. The only way they can't hijack the session is if the packets are encrypted with HTTPS.
-
Yes, but this is more unlikely. In this case the e-mail is sent from the hosting server to your client's e-mail server, which is probably not his home machine. I believe a packet sniffer would have to be installed on either of those machines or on a machine on the same network; this is unlikely to occur if the companies hosting those services know their business. It would be much more likely that the client's machine becomes infected with a virus or trojan that sniffs his internet traffic (and thus intercepts the e-mail message as he's reading it). But that's always a risk.
-
Please mark the topic as solved.
-
You could put in a call to mysql_error() and see what the actual error is; I mean, how do you expect to solve it if you don't know what the actual message is? Otherwise your question is like someone saying, "I'm having a problem with my car...any ideas???" More information is required!
-
echo tep_draw_input_field( "player[{$player['player_id']}][{$c}]", $row[$c], $extra ) ."</td>"; Should have been: echo tep_draw_input_field( "player[{$player['player_id']}][{$c}]", $player[$c], $extra ) ."</td>"; Notice the change from $row[$c] to $player[$c]. I'm used to calling my database rows $row in PHP, but you had named yours $player. I was simply using the wrong variable. <?php require('includes/application_top.php'); // if the customer is not logged on, redirect them to the login page if (!tep_session_is_registered('customer_id')) { $navigation->set_snapshot(); tep_redirect(tep_href_link(FILENAME_LOGIN, '', 'SSL')); } ?> <!doctype html public "-//W3C//DTD HTML 4.01 Transitional//EN"> <html <?php echo HTML_PARAMS; ?>> <head> <meta http-equiv="Content-Type" content="text/html; charset=<?php echo CHARSET; ?>"> <title><?php echo TITLE; ?></title> <base href="<?php echo (($request_type == 'SSL') ? HTTPS_SERVER : HTTP_SERVER) . DIR_WS_CATALOG; ?>"> <link rel="stylesheet" type="text/css" href="stylesheet.css"> <?php require('includes/form_check_coach.js.php'); ?> </head> <body marginwidth="0" marginheight="0" topmargin="0" bottommargin="0" leftmargin="0" rightmargin="0"> <!-- header //--> <?php require(DIR_WS_INCLUDES . 'header.php'); ?> <!-- header_eof //--> <!-- body //--> <?php mydbg( 'POST: ' ); mydbg( $_POST );//%% if( !empty( $_POST ) ) { /** * If you print_r( $_POST ) you will find that you have an array named 'players'. * Each index into this array will be POSITIVE and the players database ID if they already exist in the database * The index will be NEGATIVE if the player is new and needs to be inserted. * * And then each player is an array where the associative names should match your column names, * so that you can easily generate your insert / update statements based on what I * showed you earlier. */ foreach( $_POST['player'] as $player_id => $player_info ) { // ADDED A CHECK FOR IF THE PLAYER IS EMPTY! $emptyplayer = $player_info; // Copy the array unset( $emptyplayer['customer_id'] ); // Remove customer_id from empty player $emptyplayer = trim( implode( " ", $emptyplayer ) ); $emptyplayer = !strlen( $emptyplayer ); // true if empty, false otherwise foreach( $player_info as $k => $v ) { $player_info[$k] = "'" . mysql_real_escape_string( $v ) . "'"; } if( $player_id < 0 ) { if( $emptyplayer ) { mydbg( 'Skipped player' );//%% continue; // SKIP TO NEXT PLAYER } // OOPS! SINCE 'player_id' IS AUTO-INCREMENTING, WE DO NOT HAVE TO INSERT IT. // THEREFORE THE FOLLOWING LINE IS COMMENTED OUT (i.e. you can remove it from your code). //$player_info['player_id'] = "'" . mysql_real_escape_string( $player_id ) . "'"; $stmt = "insert into `rosters` ( " . implode( ', ', array_keys( $player_info ) ) . " ) values ( " . implode( ', ', $player_info ) . " )"; mydbg( 'Insert player' );//%% }else if( !$emptyplayer ){ // player_id GREATER THAN ZERO, SO PLAYER EXISTS IN DATABASE. PLAYER IS NOT // EMPTY, SO WE UPDATE HIM! foreach( $player_info as $k => $v ) { // SLIGHTLY MORE READABLE $player_info[$k] = "`{$k}`={$v}"; } $stmt = "update `rosters` set " . implode( ', ', $player_info ) . " where " . "`player_id`='" . mysql_real_escape_string( $player_id ) . "'"; mydbg( 'Update player' );//%% }else{ // player_id GREATER THAN ZERO SO HE EXISTS IN DATABASE. PLAYER IS EMPTY // SO WE DELETE HIM! $stmt = "delete from `rosters` where `player_id`='" . mysql_real_escape_string( $player_id ) . "'"; mydbg( 'Delete player' );//%% } $r = mysql_query( $stmt ); // check for errors and success if( !$r ) { mydbg( $stmt );//%% mydbg( mysql_error() );//%% } } } echo "<form action=\"roster.php\" method=\"post\"><table>"; // The reason your players are not selected from the database and displayed in the form // is because $sqlplayers is NULL; you are not running any query. $sqlplayers = "select * from `rosters` where `customer_id`='" . mysql_real_escape_string( $customer_id ) . "'"; $result = mysql_query( $sqlplayers ); $maxrows = 15; $insid = -1; // create a blank player template $cols = array( 'fname' => 'size="10"', 'lname' => 'size="10"', 'address' => 'size="15"', 'city' => 'size="15"', 'state' => 'size="2"', 'zip' => 'size="10"', 'phone' => 'size="10"', 'email' => 'size="20"', 'number' => 'size="2"', 'gradyear' => 'size="4"', 'height_feet' => 'size="1"', 'height_inches' => 'size="4"' ); $blankplayer = array(); foreach( $cols as $c => $extra ) { $blankplayer['player_roster_' . $c] = ''; // MODIFIED TO ADD player_roster_ prefix } // we now have a blank player template for( $i = 1; $i <= $maxrows; $i++ ) { echo "<tr>"; if( $result ) { $player = mysql_fetch_assoc( $result ); mydbg( $player );//%% } if( !$player ) { // We've run out of players, so create a blank one to insert $result = null; // stop trying to access result $player = $blankplayer; $player['player_id'] = $insid--; // first blank player is id -1, second is -2, third is -3, etc. } // dump the fields $firstcol = true; foreach( $cols as $c => $extra ) { $c = 'player_roster_' . $c; // MODIFIED TO ADD player_roster_ prefix echo "<td>"; if( $firstcol === true ) { echo "<input type=\"hidden\" name=\"player[{$player['player_id']}][customer_id]\" value=\"{$customer_id}\" />"; } echo tep_draw_input_field( "player[{$player['player_id']}][{$c}]", $player[$c], $extra ) ."</td>"; } $player = null; // important! echo "</tr>"; } ?> </table><input type="submit" name="editplayers" value="Submit" /> </form> <?php mydbg( null, false ); /*%%REMOVE ME dump debugging */?> <!-- footer //--> <?php require(DIR_WS_INCLUDES . 'footer.php'); ?> <!-- footer_eof //--> </body> </html> <?php require(DIR_WS_INCLUDES . 'application_bottom.php'); ?> <?php /** * Simple debugging function. If $add is true, it adds debugging message. If $add is * is false, it dumps debugging messages that were added. * * @param mixed $msg * @param bool $add */ function mydbg( $msg, $add = true ) { static $msgs = array(); if( $add === true ) { $msgs[] = $msg; }else{ echo '<pre style="text-align: left; font-weight: bold; font-size: 10px; background-color: #ececec;"> DEBUG:'; foreach( $msgs as $msg ) { if( is_bool( $msg ) ) { $msg = 'BOOLEAN [' . ($msg ? 'T' : 'F' ) . ']'; }else if( is_null( $msg ) ) { $msg = '__NULL__'; }else if( is_string( $msg ) && !strlen( $msg ) ) { $msg = '__EMPTY_STRING__'; }else if( is_array( $msg ) || is_object( $msg ) ) { $msg = print_r( $msg, true ); } echo $msg . "\n\n"; } echo '</pre>'; } } ?>
-
<?php // http://www.domain.com?page=home => home.php // http://www.domain.com?page=contact => contact.php // http://www.domain.com?page=spywareintrusion => home.php $allowed = array( 'home', 'contact', 'users', 'addresses' ); $inc_file = in_array( isset( $_GET['page'] ) ? $_GET['page'] : 'home', $allowed ) ? $_GET['page'] : 'home'; include( $inc_file ); ?>