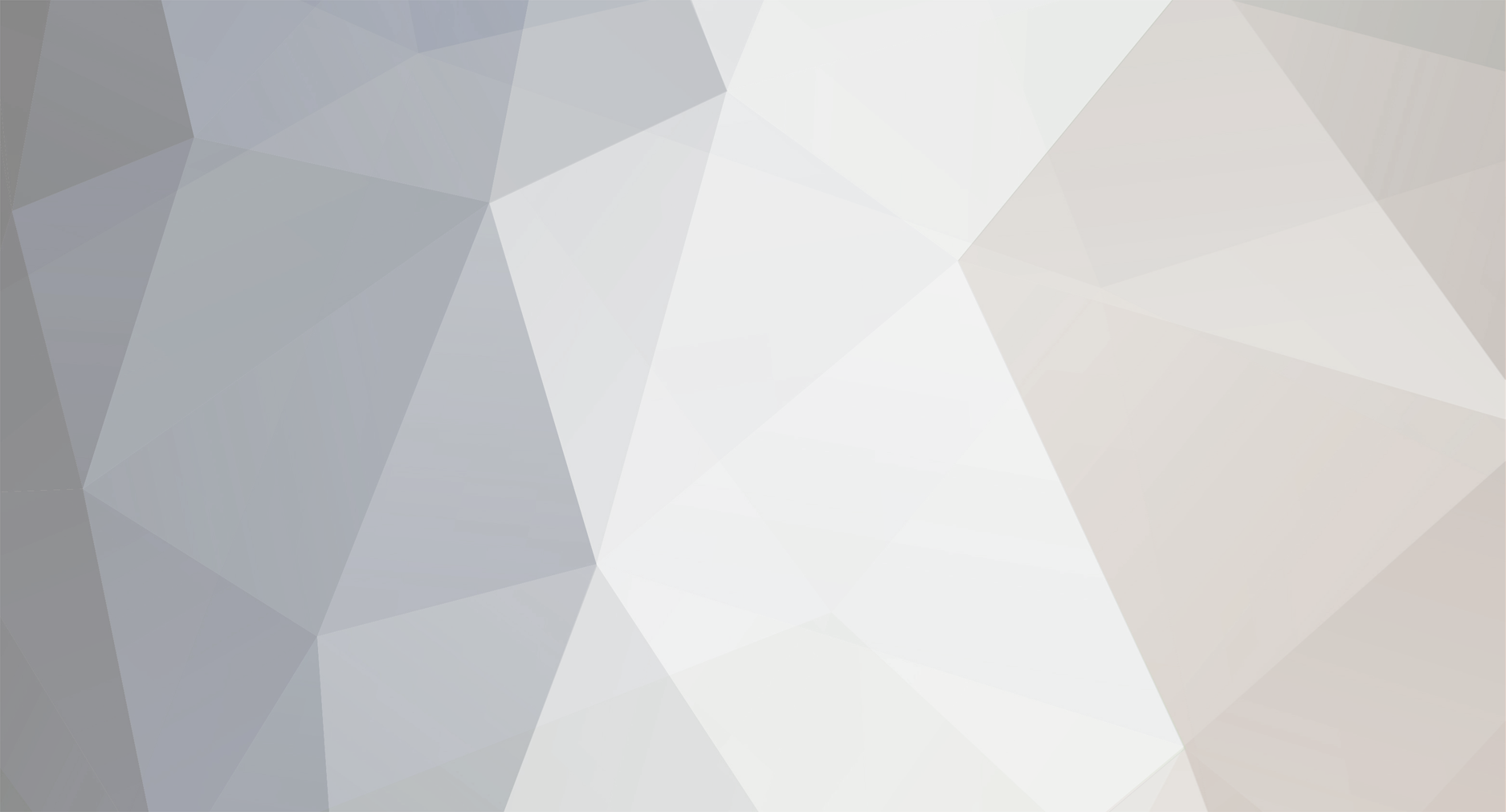
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
[SOLVED] select where now() between start and end
roopurt18 replied to bschultz's topic in MySQL Help
Is the MySQL server in a timezone that is earlier in the day than the one you are physically in? -
Programming is all in the details!
-
Are you talking about the XML-based spreadsheets (the OOXML or XMLOO or whatever MS calls it)? 1) Open Excel 2007 (or later if there is one) 2) Create a worksheet, modify some column widths, add some data 3) Save as xlsx format 4) Close Excel 5) Rename file to have zip extension 6) Unzip the file 7) Reverse engineer!
-
I don't know any reliable hosts in the Middle East, but I'm sure if you check in certain channels you can locate one.
-
If you are hashing the password, then it's irrelevant to escape_string() the password before hashing it as the hashing function will likely remove the harmful characters anyways. All that matters is the data as it is going to be entered into the database is escaped(), which means: $password = mysql_real_escape_string( md5( $salt . $password ) ); as opposed to: $password = md5( mysql_real_escape_string( $salt . $password ) ); Note that in the second example, it will work as I don't think md5() will create values that need to be escaped for the database. But if you are using a hash function which may output: ' \ or other harmful characters, then you still need to: $password = mysql_real_escape_string( some_hash( mysql_real_escape_string( $salt . $password ) ) ); Long story short is data needs to be escaped when it's in the form or state that it will be entered into the database, escaping it at any other point in time is irrelevant.
-
Does not compute!
-
You have to be very careful when parsing XHTML. SimpleXML and DOMDocument are the best tools to use if they'll work. I've never used DOMDocument, but I can say the last time I used SimpleXML it barfs all over itself if the file is not valid XML to start with. If you can trust the validity of the document then either should work fine. I've never used regexps to parse markup, but I have a coworker who tried (after SimpleXML failed due to the document being invalid markup). He basically ran into the problem of being unable to write a regexp that matches an opening tag with the appropriate closing tag. The problem is with nested elements, such as tables or divs. Say you try to write a regexp to match a div with id="foobar"; that is trivial. Now try to match it's appropriate closing div-tag when: 1) You don't know how deep in the page div::id="foobar" is (i.e. it has div parents) 2) You don't know how many div children div::id="foobar" has The last resort (and most reliable) is to program a parser of your own. I mean a real parser that is a FSM that grabs either a character or a token at a time and switches between states to determine how the current token (or character) should be handled.
-
Your markup is invalid. Does the DOM API work with invalid markup? I don't know the answer to that, but if it doesn't then that's why it's not working for you.
-
Ubuntu 7.10 was the last GNU Linux distro I played around with that I felt had almost enough polish for the masses to use comfortably. They're on version 8 or maybe even 9 now so I imagine it's just gotten better. Once you know what you're doing though, they're all pretty much the same.
-
SELECT CONCAT_WS(" ", a.`first_name`, a.`last_name`) as `name` FROM `employees` as a LEFT JOIN `out_board` as b ON a.`id`=b.`id` WHERE b.`id` IS NULL; Alias the tables to save yourself some typing. In the SELECT portion, you just identify which columns from which table you want, just like you always do.
-
$username = mysql_real_escape_string($_POST["username"]); $sql="SELECT * FROM $tbl_name WHERE username = '$username'"; $result=mysql_query($sql); $count=mysql_num_rows($result); If all you're doing here is checking if the name is available, then change the query to: $username = mysql_real_escape_string( $_POST["username"] ); $sql = "select count(*) as n from `$tbl_name` where username='{$username}'"; $result = mysql_query( $sql ); if( !$result ) { throw new Exception( "Count username." ); } $row = mysql_fetch_object( $result ); if( !$row ) { throw new Exception( "No row." ); } if( $row->n > 0 ) { // user exists }else{ // add user }
-
Just host it in the Middle East.
-
"All we are is dust in the wind." Doesn't really matter what we do!
-
When I started programming my goal was to get into game development. Lo and behold I landed in database development. Having known a few veterans of the gaming industry, I hear the entry level people work insane hours, make almost nothing, and are supposed to just take because they work IN THE GAMING INDUSTRY!!1!!!!!ONE!1!! Suckers.
-
You might do something like this for processing then: <?php if( !empty( $_POST ) ) { /** * If you print_r( $_POST ) you will find that you have an array named 'players'. * Each index into this array will be POSITIVE and the players database ID if they already exist in the database * The index will be NEGATIVE if the player is new and needs to be inserted. * * And then each player is an array where the associative names should match your column names, * so that you can easily generate your insert / update statements based on what I * showed you earlier. */ foreach( $_POST['players'] as $player_id => $player_info ) { foreach( $player_info as $k => $v ) { $player_info[$k] = "'" . mysql_real_escape_string( $v ) . "'"; } if( $player_id < 0 ) { $player_info['primary_key'] = "'" . mysql_real_escape_string( $player_id ) . "'"; $stmt = "insert into `yourtable` ( " . implode( ', ', array_keys( $player_info ) ) . " ) values ( " . implode( ', ', $player_info ) . " )"; }else{ foreach( $player_info as $k => $v ) { $player_info[$k] = '`' . $k . '`=' . $v; } $stmt = "update `yourtable` set " . implode( ', ', $player_info ) . " where " . "`primary_key`='" . mysql_real_escape_string( $player_id ) . "'"; } mysql_query( $stmt ); // check for errors and success } exit(); return; // whatever is appropriate to stop processing } ?>
-
[SOLVED] How to calculate two dates into a percentage
roopurt18 replied to DirtySnipe's topic in PHP Coding Help
My code takes two dates, calculates the number of seconds between them, multiplies by a percentage, and then adds that percentage to the first date. Finally it outputs the result as a date. Otherwise what you are asking for makes no sense mathematically so you'll need to be more clear. -
date and time that are auto inserted into my database are wrong
roopurt18 replied to sandrine's topic in PHP Coding Help
Postgre's timestamp fields support with timezone or without timezone. If the database you are using does the same, then you can just insert it with the correct timezone and display it with the user's timezone. Then it will all be correct! -
[SOLVED] How to calculate two dates into a percentage
roopurt18 replied to DirtySnipe's topic in PHP Coding Help
Untested but should get you close... <?php // three inputs $start = '2009-01-01'; $end = '2008-01-01 12:00:48'; $percentage = .3; // order them correctly $start = strtotime( $start ); $end = strtotime( $end ); if( !$start || !$end ) { throw new Exception( 'Invalid dates' ); } $min = min( $start, $end ); $max = max( $start, $end ); // $min is the begin date in seconds, $max is the end date in seconds echo date( 'Y-m-d H:i:s', $min + ($percentage * ($max - $min)) ); ?> -
He just wants to modify it to search on fields other than firstname and lastname. In addition to his search form, he'll need to modify his SQL statement: $sql="SELECT ID, FirstName, LastName FROM staff WHERE FirstName LIKE '%" . $name . "%' OR LastName LIKE '%" . $name ."%'";
-
[SOLVED] Recursion: How to display data hierarchically from a table
roopurt18 replied to sbrinley's topic in PHP Coding Help
Have you read this: http://dev.mysql.com/tech-resources/articles/hierarchical-data.html -
It would be a good idea if you removed your database credentials from your script before posting it on a public forum.
-
A pre-tag with print_r() is almost all you ever need to debug stuff. In the case where it's saying Array, do this: <?php echo '<pre>' . print_r( $_POST, true ) . '</pre>'; ?> And see if you can't make sense of how to access your data.
-
foo.php?id=1&qwerty=hello&hi2u=ohnoes
-
Your HTML should look like this: <input type="text" name="screen[]" value="" /> <input type="text" name="screen[]" value="" /> <!-- etc. -->