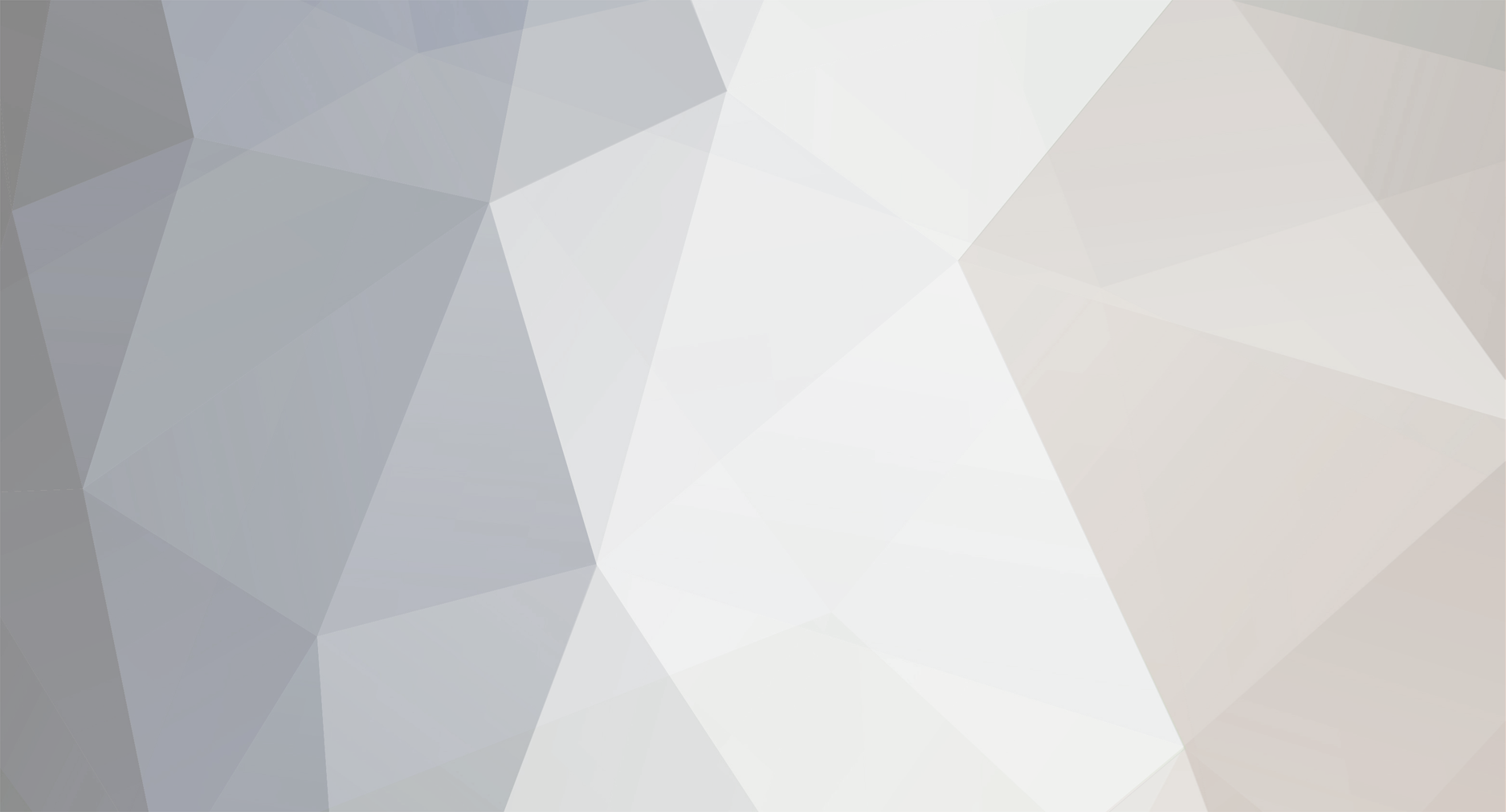
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
how can I arrange an array I got from database?
roopurt18 replied to shedokan's topic in PHP Coding Help
You do know that you can calculate data in the MySQL query and ORDER BY calculated fields, right? -
Dot is not special when present inside a character class. Therefore if you want to match dot in a character class, use: [.] and not: [\.]
-
<?php $width = 2; // width of printed value for( $i = 0; $i <= 10; $i++ ) { echo sprintf( "%0{$width}s", $i ) . '<br />'; // or "\n" } ?>
-
[SOLVED] how to return variable variable?
roopurt18 replied to diamondnular's topic in PHP Coding Help
The original code you posted looks ok to me. What were the errors you received? I ran your code and it works fine: <?php function test() { // get the array name $array_name = 'foo'; // initiate all elements to be zeros for ( $i=1;$i<=10;$i++ ) { ${$array_name}[$i] = ''; } // assign each element of array a value for ( $i=1;$i<=10;$i++ ) { ${$array_name}[$i] = 'bar' . $i; } return ${$array_name}; } $new_array = test(); print_r($new_array); ?> -
Display the value as: sprintf( "%02s", $value );
-
how can I arrange an array I got from database?
roopurt18 replied to shedokan's topic in PHP Coding Help
You can't change your database query to have an ORDER BY column and just get the data out of the database already sorted? That's usually the easiest and most efficient method. If you insist on the array_multisort() method, you want to model your code on example #3 in the array_multisort documentation. <?php $combinedNum = array(); foreach( $your_array as $key => $val ) { $combinedNum[$key] = $val['combinedNum']; } array_multisort( $combinedNum, SORT_ASC, $your_array ); ?> -
On the confirm page, you can create a hidden input whose value is a delimited list of the selected values. Use a delimiter that does not exist in one of the values. <!-- on the confirm page --> <input type="hidden" name="selected" value="1,2,4,7,11" /> <?php // Processing page $selected = explode( ',', $_POST['selected'] ); echo '<pre style="text-align: left;">' . print_r( $selected, true ) . '</pre>'; ?>
-
I'm guessing that last_id is a user_id of the person who viewed the topic. I'm curious why you wouldn't always want to update it to the current value?
-
You don't need AJAX for this. As someone else suggested use an IMG tag. Your client inserts an IMG tag to a script on your page, complete with $_GET arguments. <!-- your client's html code --> <img style="display: none;" alt="Something or other" src="http://www.yoursite.com/clients/img.php?client_id=1&something_else=Larry" /> On your site you create the img.php script: <?php // Grab $_GET['client_id'] and $_GET['something_else'] and do something with them. // doing something with the data... // This is supposed to be an image, so your script should dump out a 1x1 white img header( 'Content-type: image/gif' ); // set more headers as appropriate fpassthru( 'path/to/1x1.gif' ); ?> (edit) If you need to get more fancy, think about creating a web service, which is very easy to do. Do not rely on JavaScript (aka AJAX) solutions as the user can just disable JavaScript.
-
<?php // Your first attempt $gcs = array($_POST['checkbox']); // If $_POST['checkbox'] is already an array, then you do not // need to modify it before using your foreach. Just use it // as-is. foreach( $_POST['checkbox'] as $gc ) { // Do something with $gc } ?> <?php // Your second attempt $gcs = array(implode(',', $_POST['checkbox'])); // Implode *returns a string*. With the above line you have // turned $gcs into a string and you *can not* use it in a // foreach. If you want to get a comma-delimited list of // the elements in an array: echo "These were selected: " . implode( ', ', $_POST['checkbox'] ); ?>
-
I disagree with having to know how it works before you set it up. I knew how to program long before I even knew what PHP was and when I started learning PHP I just installed xampp on a windows box. I didn't have to configure anything. Double click the installer, next, next, next, finish. I only started learning how to install and configure this stuff as the need arose. It starts small. "Ah I need to change php.ini" "Ah I need to change .htaccess" "Ah it's better to put items in .htaccess in httpd.conf instead. What's all this other stuff do in this file?" "Ah I need to recompile to enable or disable something." Shared hosting still has lots of uses; I use it for my personal site as the site's requirements don't warrant a VPS or dedicated server.
-
I've always used and recommended ServerPowered and WebHostFreaks, the company behind these forums. Not sure about the pricing but the hosting is top notch as far as I'm concerned.
-
You can only learn the benefits of OOP by writing OOP and writing lots of it. It also helps to work in steps. 1) Stop writing procedural code. Just write everything as classes even if it seems like more work with no benefit. This will get you familiar with the syntax and "structural" components of OOP. Write a small project in this way. It doesn't have to be a web site. It can be a command line script that runs a simulation or anything else. But write something. 2) Read about inheritance. Think about how you could have used inheritance in your project from step #1 to decrease the amount of work you performed. Rewrite the project from step #1 to take advantage of this. 3) Introduce a new object or component into your project. For example if your program calculates miles per gallon for cars, introduce buses, planes, boats, etc. Write them as separate classes. 4) Read about polymorphism. Think about how you could have applied that in your project to decrease the amount of work and rewrite your project again. 5) Keep writing OOP code. Start using it everywhere. Eventually you will start to see some re-emerging problems and road-blocks. At this point pick up a book on design patterns and the light bulbs should start really firing off.
-
This a take home mid-term or something? The only reason I'm not locking / deleting it is you have a history of posts that look like genuine questions; so you're getting the benefit of the doubt.
-
Just query it on each page load. If you leave it in the session or memory or anywhere else, then it can be changed and your session (or whatever it is) may become stale. In order to determine if the data is stale, you will have to run a query to determine if the data in the database is newer than the data you have stored anyways. So since you have to hit the DB with a query and process the results anyways, it might as well be a select query with a proper index that gets the data you need. Here's a helpful hint, database indexes are a compromise between size and speed. IF all of the data in the select query happens to be stored in the index, then the DB engine will only use the index for your select; this is the best possible case. If the select statement has columns that are not part of the index, then the DB will use the index to prune the rows and then pull the remaining columns from the actual table file on disk. So my suggestion is to keep the amount of information you need on each page down to the bare minimum and create an index on those columns.
-
You can create a redirect rule in httpd.conf or an .htaccess file to redirect all requests without a www to the domain with www. You will have to do this for each of the sub-folders of your site that contain URL-accessible scripts unless you are sending all requests through a single index.php via mod_rewrite.
-
No. I got it on your very first post. In fact the reason I responded to the thread was because people were constantly not understanding what you want to do and trying to stir you down the wrong path. Help doesn't always have to be "This is how you solve the problem." Sometimes help can be "You're assumptions are right and you're stuck." BTW, I did tell you one method of solving the problem. You can create an applet (either Java or .NET) that can run on the client's machine and break it apart before it ever reaches the server. I have to make (educated) guesses because I don't know as much about your environment as either you or your server's administrators. There is nothing about this topic I can say for absolute certain. I'm also not trying to circumvent your server's restrictions. You are trying to do that yourself by breaking a large file into multiple pieces. I'm telling you what I might do in that same situation. Here again I am making an educated guess. The file is being uploaded to a target URL handled by either apache or IIS most likely. When apache / IIS realize they have an incoming file, they receive the entire file and only after they have received it do they bother to tell your PHP script that a file has been uploaded. Thus, using PHP and your web server (apache or IIS), you have no way of detecting even where the temp file is until the upload is complete (which won't happen because your server disallows it). Now we both know that there are sites that have upload progress meters so there must be a way of detecting and monitoring the temp file as it grows during the upload. I have no experience with these but here are some more educated guesses. First off, PHP has no built-in mechanism to accomplish this that I'm aware of, but there are probably PHP extensions that allow it. However, I'm guessing you are unable to modify your PHP installation since you are likely not the admin of the machine. I'm guessing that most other solutions to upload progress scripts are uploading to perl or python scripts and are maybe not being handled by apache / IIS either (although I have no idea really). You could probably play around with examples of those scripts to get an idea of what to do. Now, let's say that you did have an idea of what to do. Let's say you could monitor the upload and watch the file's size and once it reaches a certain barrier copy and move it. You will be competing with whatever process is handling the upload in the first place. This process might have the file opened exclusively, in which case there is nothing you can do. Or it may not be exclusive, but then how can you be sure your process that "chunks" the file doesn't interrupt a read or write by the owning process? You can't. In all likelihood you will just corrupt the data. Now you could write your own small web-server that can break files as they come in. Depending on your experience level this might be very difficult and then you'd have to talk your web host into installing it on their machine. That is not likely to happen either. So now we're back to you creating a Java or .NET client component that can break the file on the client's end and send it to you in pieces. Or upgrade your hosting plan. Or get a new host.
-
I'm assuming Little Guy's solution will not work because: I'm guessing that if a user tries to create a file, even in the /var/tmp that is an upload, the server stops it from happening. AFAIK, there is nothing you can do to get around this, otherwise the server admins wouldn't even have it in place. This is one of those situations where you would probably need a Java or .NET component on your site that the user installs. This component would be responsible for allowing the user to select a file to upload and if the file is larger than a certain size, the component would break it into chunks and upload them individually.
-
First, don't use $HTTP_COOKIE_VARS as it is deprecated. Use $_COOKIE instead. You should also be aware that cookies are set for the domain and that http://yoursite.com and http://www.yoursite.com are two different domains. So make sure you are not setting the cookie on a yoursite.com URL and then trying to read it on a www.yoursite.com URL. The best thing to do when you are having problems with something is to simplify it into a "proof of concept" script. Create a folder called test and create individual scripts inside it, where each script is a single proof of concept. set_cookie.php - sets a cookie value read_cookie.php - looks for the value and displays it delete_cookie.php - removes the cookie You can create those as a single script if you think you can handle it. But the point of a proof of concept is to keep it simple. If all you are trying to do is set a cookie and it's not being set, you don't have a lot of other crap in your way to bog you down. To implement a "remember me" feature you have to do two things. When a user logs into the site: Does the user have "Remember me" checked? No - Log in as normal (via sessions) Yes - Create a unique key for the user. Store this unique key in the user's row in the database. Store encrypt(user_name) and encrypt(unique_key) in the cookie. Log the user in as normal (via sessions) Anytime a user visits your site and is not already logged in: Does the user have a cookie with the user_name and unique_key values?( No - This is not a remember me user, treat as a guest. Yes - This is a remember me user. Get the cookie values and decrypt. Try to SELECT the matching row from the database. If no row is found, do not log the user in. If a row is found, log the user in via sessions.
-
JavaScript is extremely powerful and the web wouldn't be what it is today without it. That said, there are plenty of bad implementations (from sites using it to actual interpretors) to give it a bad name. However, most people who knock JavaScript simply do not understand how it works. Also, it's completely irrelevant to piss and moan about "cross-platform" problems, which web programmers do constantly. If you don't want to deal with cross-platform problems, become a Windows, *nix, or a Java developer. It's like hearing a psychological therapist say he hates his job because people whine all the time. It comes with the territory. Deal with it or get out.
-
I can understand why someone might want to do this, but it really is a superficial thing. Unless there is a technical restriction, who cares if the table is called users or old_system_users.
-
It's $this->something Read the PHP manual on object oriented programming. It's a big topic so I'm just pointing you in the right direction.
-
http://www.google.com/search?q=mysql+curdate
-
[SOLVED] Help with validating form email addresses
roopurt18 replied to Sportbob's topic in PHP Coding Help
I notice you're using ereg where I normally use preg, so perhaps the rules are different. But you have domain.edu in your regexp. That dot does not match a dot, it matches anything. What you want is: domain[.]edu or domain\.edu Also, if you want multiple domains, try changing +domain1.edu to: +(domain1)|(domain2)[.]edu -
There is a good chance the session attached to that ID no longer exists on the server. If you want to create a remember me function, the best thing to do is to store the user's ID or username in a cookie along with a unique token. You store this same unique token in the user's row in the users table. When a guest visits your site, you check for the username and token in the cookie and see if they match in the database as well. If they do, you can auto-login that user. Do not store the user's password or an easily guessed value in the cookie as clever people will figure it out. You want the token to be unique and constantly changing so the chances of someone guessing the proper token for a username is slim to none.