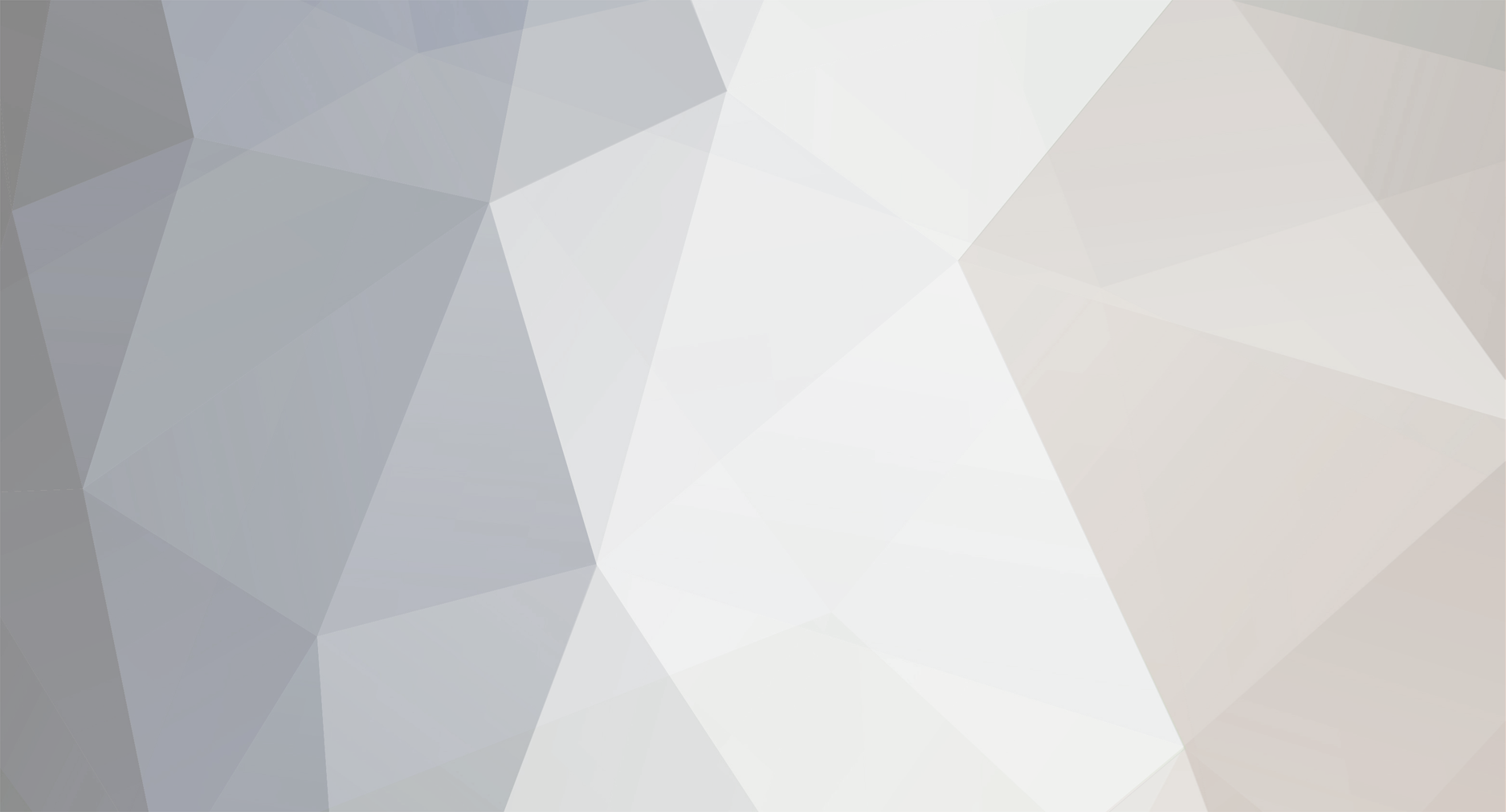
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Are you trying to explain this to a friend or to a teacher? In any case, let's do this the Socratic way. Why would you drive a car from Los Angeles to San Diego as opposed to riding a bicycle? After all they both get you there, they're the same right?
-
JavaScript is inappropriate for this kind of thing because the user may have it turned off. The solution here, I think, is to change your code that renders the form. <tr> <td align="center"><input type="text" name="rows[{$id}][name]" value="{$name}" /></td> <td align="center"><input type="checkbox" name="rows[{$id}][check]" value="{$id}" {$checked} /></td> </tr> Notice the subtle difference? Now you can loop through $_POST['rows'] and each item will itself be an array with indexes name and check. <?php foreach( $_POST['rows'] as $id => $row ) { echo '<pre>' . print_r( $row, true ) . '</pre>'; } ?>
-
Getting the PHP file to download without being executed
roopurt18 replied to makoinater's topic in PHP Coding Help
Sounds like you're SOL. -
Getting the PHP file to download without being executed
roopurt18 replied to makoinater's topic in PHP Coding Help
Does your old site or host provide a backup feature? If it does you can back it up and download it. -
At the most abstract level, you'll want to research MVC and there's plenty of topics on this in the Application Design board. Read up on it just a little bit and then, since you're new, hit a middle ground. Create a base db.php: <?php class MyDB { function connect() { // connects to database } function select( $sql ) { // runs a select query } function insert( $insert ) { // runs an insert query } // etc... } ?> You should have a config.php that establishes a DB connection, among other things: <?php // Database init require_once 'MyDB.php'; MyDB::connect(); ?> Then say your site has users, have DB access functions in users.php: <?php class Users { function add( $username, $pass, $otherVar ) { $insertSql = 'INSERT INTO `users` ...'; return MyDB::insert( $insertSql ); } function authenticate( $username, $pass ) { $select = 'SELECT COUNT(*) AS `n` FROM `users` WHERE ...'; return MyDB::select( $select ); } } ?> You can create an html.php for common XHTML elements you constantly render, or a session.php, etc. Also, don't mix functionality. Don't put all of your user-related functions in user.php. Put the user database ones in user_db.php and the user XHTML ones in user_display.php or something like that. That would be a good beginning step for code organization.
-
DarkWater, that is what I've already recommended he do.
-
Does this help clarify: <?php // Select items from the database $sql = " SELECT `value_column` AS `value`, `display_column` AS `display` FROM `your_table` WHERE 1 ORDER BY `display` "; // Get the items $item_query = mysql_query($sql) or die('Error: ' . mysql_error()); /** * GETTING THE DEFAULT SELECTED ITEM */ $default_item = null; // initially no item // If you are here from another page in your site, you have to pass the // default either via $_GET or $_SESSION; use the appropriate line $default_item = isset($_GET['default_item']) ? $_GET['default_item'] : $default_item; // $default_item = isset($_SESSION['default_item']) ? $_SESSION['default_item'] // : $default_item; // However, if 'theName' is set within $_POST, then you are here from // a form submittal. In this case you should use the $_POST value because // it is the one the user selected $default_item = isset($_POST['theName']) ? $_POST['theName'] : $default_item; /** * END - GETTING THE DEFAULT SELECTED ITEM */ // create a dropdown echo '<select name="theName">'; // open tag while( $item = mysql_fetch_assoc($item_query) ) { echo sprintf('<option value="%s"%s>%s</option>', $item['value'], $item['value'] == $default_item ? ' selected' : '', $item['display'] ); } echo '</select>'; // close tag ?>
-
For future reference, MySQL provides a LOAD DATA which can load a CSV file into a table. If the file has values you'd like to edit you could load them into a temp table first, modify the values, and then INSERT ... SELECT from the temp table into the final destination.
-
No problem. If you plan on having boards nested within boards you'll want to read the hierarchical data link I provided though.
-
I'm not sure I understand why you couldn't just do it with either the query I wrote or the one you wrote. Did you try either of them? What did they return? If they didn't return the set you wanted, what should have changed in the result set?
-
If you follow the links in my signature, I have written some basic form tutorials that explain how to create a single script to handle the display, validation, and processing of a form on a single script. You can adapt them to your situation by doing the following: The form in a.php should submit to a.php so that the script that renders the form can also validate it. If the form is invalid you can re-display the form with the previous values set. Change the processing portion of a.php to set session variables and then redirect to b.php. b.php can then do whatever it normally does. And if b.php needs any of the values from a.php they are in the session.
-
Without know which queries you've tried and what they returned, or without an example of the data and the set you'd like returned, it's hard to answer your question. Have you tried anything like: SELECT DISTINCT `Field2` FROM `myTable` WHERE `Field3`=1 AND /* You could use BETWEEN here, I didn't fill like */ /* doing all the casting /shrug */ `Field5`>=timestamp1 AND `Field5`<=timestamp2 AND `Field3`=4 AND `Field4`='Yes'
-
For the database part, you'll want to understand normalization, the WHERE clause, and table joins. It might also be beneficial to understand hierarchical data as well. For the PHP part, you just need to know how to connect to the database (mysql_connect()) and run SELECT queries (mysql_query()). When you select data you loop over it and generate XHTML. You should be able to Google some of those topics and get at what you want. Or you might try one of these: Google Search Results
-
I bet it was a great icebreaker.
-
Just yesterday I made some mistakes in the worst place possible, during an interview. I was asked three simple CS questions. I answered the first one. Froze on the second like a goober, and was given one slightly less challenging, which I answered. The first interviewer left and arranged with me to meet a manager; while waiting for the manager, I thought about the one that stumped me. I had the beginnings of a solution in my head by the time I met with the manager, and afterwards when I'd made it home, I checked my solution and it was correct. Then later that night in the shower I realized my response for the last question, while correct, was the "dumb" way to do it. Overall a disappointing experience, but I'd like to think that since I was able to meet with a manager after the first interview that they might still be interested.
-
On a somewhat related note, I was hankering for some RTS action and installed war3. I haven't played that in like 2 or 3 years so I was ready to get my butt handed to me. I log into b.net and win 3 in a row. It was sad really.
-
when/why would a user need to imagecreatefrompng
roopurt18 replied to dsdsdsdsd's topic in PHP Coding Help
Another common use for the image libraries is to confirm that a file is in-fact an image. For example, if you provide an upload script and I rename a php script to have a .jpg extension, depending on how you perform your validation your site may accept my file, even though it is not an image. To validate that the file is actually an image, you might try and open it with the various image manipulation functions; if the functions fail then the file is not a valid image. That said, I use finfo (or fileinfo) for the same functionality. -
And here I thought youtube was going to become the new lolcat.
-
[SOLVED] Calculating two thirds of a value?
roopurt18 replied to rareness's topic in PHP Coding Help
I want you to think long and hard about what that simplifies to mathematically. -
Oops. That should have said regarded.
-
When to 'shared->virtual->dedicated' hosting?
roopurt18 replied to Gath's topic in Application Design
Use virtualization to create a host on your current machine and restrict the memory and cpu usage to be the same as common hosting packages. Then nail the crap out of it with a program that simulates user actions. If you can afford it a VPS should be able to handle your site, at least in the initial stages. -
Allow the user to choose their template style. If you already have a class for each, then when the user is entering their template have them select which of the two the template should be retarded as. Done and done.
-
The best place to disable / enable error reporting is in php.ini. If you have a shared host they might provide a means for you to provide your own php.ini; if you're on a dedicated or local environment then it shouldn't be a problem. I was adjusting my error reporting levels today in php.ini and they didn't take affect until I restarted the web server, so try that as well if it looks like your changes are being ignored.
-
Have you made any real effort to figure out why this isn't working yourself? Or are you just looking for copy and paste solutions? Whenever you have a syntax error, you can always, always figure out where the error is by commenting out chunks of code at a time. This is where a good editor's automatic commenting (usually ctrl + /) comes in handy. Start around where the error is reported. Start at the most nested piece of code and comment it out. If you get the error move up on level on your indentation and comment that section. Repeat until the error goes away. It's repetitive but you can find the error that way. Also, syntax errors will always come up in coding so you might as well learn how to fix them yourself now.
-
Creating computer software (applications)
roopurt18 replied to therealwesfoster's topic in Miscellaneous
In today's world, I'd suggest learning how to make applications in C# using .NET with Microsoft's free Visual C# Express. Then I'd spend a bit of time learning how to make platform-independent applications with Java. Then maybe you should try and write a windows program in assembly. After a few hundred lines of code you can get a blank window to appear. There's no harm in hard-coding a few times through to get the hang of how things work under the hood, but once you understand it there's no sense in wasting countless hours on mundane tasks. On a side note, I'd recommend not learning Visual Basic for two main reasons: 1) The language is unnecessarily verbose, which is an annoyance to me 2) Basic style languages are not as common IMO as c-style languages, so having solid roots in c-style syntax gives you a head start in moving from one language to another